To move a commit from one branch to another in Git, you can use the `git cherry-pick` command followed by the commit hash, after checking out to the target branch.
git checkout target-branch
git cherry-pick <commit-hash>
Understanding Git Commits
What is a Git Commit?
A Git commit is a snapshot of your project's file system at a specific point in time. It serves as a record of changes you've made to the codebase. Each commit contains not only your changes but also a unique identifier (commit hash), author information, and a commit message. This information is crucial for understanding the rationale behind changes and allows for efficient tracking and referencing throughout the project.
Branching in Git
What is a Branch?
In Git, a branch is essentially a lightweight movable pointer to a commit. When you create a branch, you're creating a new path of development within the repository. Branches allow developers to work on new features or bug fixes in isolation, without affecting the main codebase.
For instance, the primary branch in most repositories is commonly called `main` or `master`. Feature branches can be created to implement new functionalities, and they can diverge from the main line of development. This encourages collaboration and avoids potential conflicts from simultaneous changes.
How Branches Work Together
Branches can diverge and later be merged back into one another. This flexibility allows for effective team collaboration, as multiple developers can work on different features concurrently. A clean branching strategy helps maintain project organization and commit integrity.
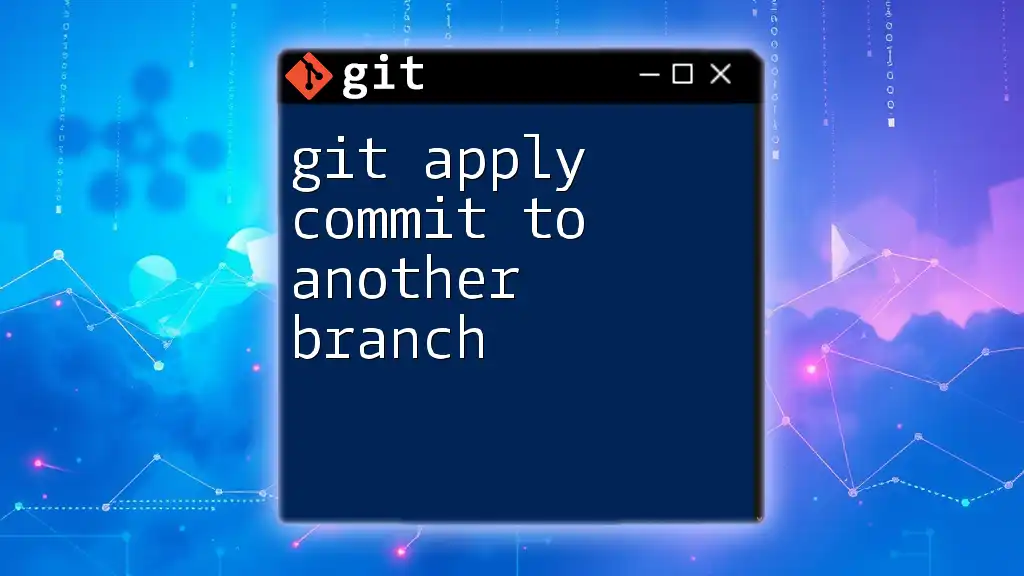
Moving Commit to Another Branch
Reasons to Move a Commit
There can be several reasons for needing to move a commit to another branch:
- Accidental Commits: Occasionally, you might commit code on the wrong branch, which necessitates moving it to the intended branch.
- Feature Development Errors: If you've been working on a specific feature but inadvertently developed it on the wrong branch, you may want to relocate that commit.
- Cleaning Up Commit History: For a more organized and understandable commit history, you might want to relocate commits to their respective branches.
Prerequisites
Before proceeding with moving commits, ensure you have a basic understanding of Git commands and that your Git environment is set up properly. Familiarity with `git log`, branch creation, and checkout commands will be beneficial.
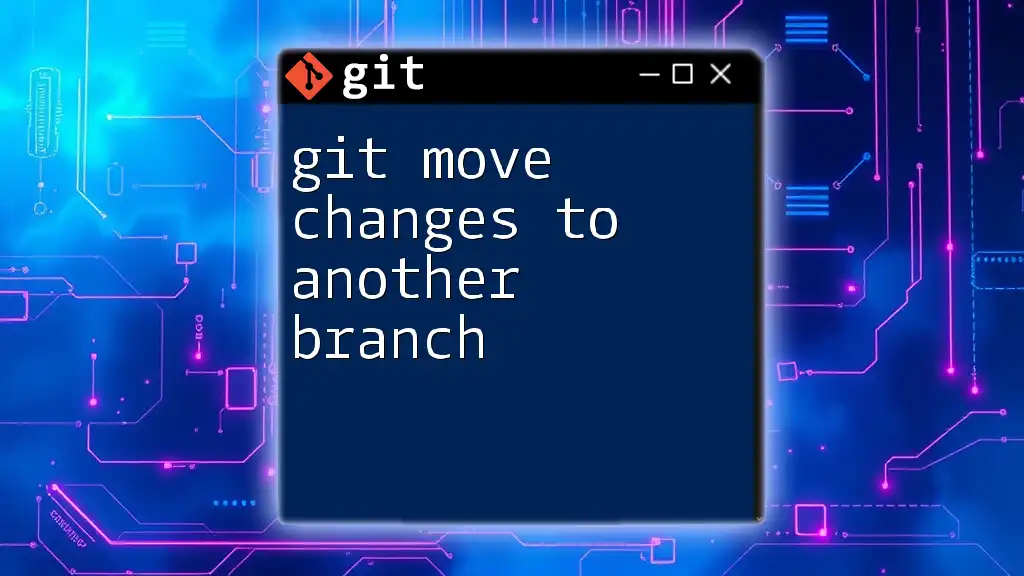
Methods to Move Commits
Using Git Cherry-Pick
What is Cherry-Picking?
Cherry-picking in Git enables you to apply specific commits from one branch onto another branch. This method is particularly helpful when you want to apply a commit without merging the entire branch or manipulating the code history.
Steps to Cherry-Pick a Commit
-
Identify the Commit Hash To begin, you need to find the hash of the commit you wish to move. You can do this using the `git log` command. Example:
git log --oneline
This displays a concise list of commits along with their hashes.
-
Checkout to the Destination Branch Before you can apply the cherry-pick, switch to the branch where you want to move the commit. Example:
git checkout target-branch
-
Execute Cherry-Pick After checking out to the target branch, execute the cherry-pick command:
git cherry-pick <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you wish to move. Git will apply the changes associated with that commit to your current branch.
Handling Conflicts During Cherry-Picking
If the changes in the commit conflict with the current state of the target branch, Git will notify you of the conflict. In this case, you'll need to manually resolve the conflicts, stage the resolved files, and complete the cherry-pick process with:
git cherry-pick --continue
If you want to abort the cherry-pick process at any point, you can use:
git cherry-pick --abort
Using Git Rebase
What is Git Rebase?
Git rebase is another powerful tool for rewriting commit history. It allows you to move or combine commits from one base to another, thus creating a cleaner, linear commit history. Unlike cherry-picking, rebasing can be used to transfer a series of commits.
Steps to Move a Commit with Rebase
-
Checkout to the Branch Containing the Commit First, navigate to the branch that contains the commit you wish to move. Example:
git checkout branch-with-commit
-
Initiate Interactive Rebase Start an interactive rebase with the command:
git rebase -i HEAD~n # where n is the number of commits back
Here, `n` would be the number of recent commits you'd like to view for possible rebasing.
-
Modify the Rebase Instructions An editor will open displaying a list of commits. Here, you can mark the desired commit to be moved by prefacing it with `edit` instead of `pick`.
-
Checkout to the Destination Branch and Apply After marking the commit, check out to your target branch:
git checkout target-branch
Finally, apply the selected commit with the cherry-pick command as previously described:
git cherry-pick <commit-hash>
Advantages and Disadvantages of Rebasing
Rebasing provides a cleaner project history and can make collaboration easier. However, it can be a bit more complex than cherry-picking, and misuse may lead to a messy commit history. It’s essential to understand when to utilize each method effectively—cherry-picking for individual commits and rebasing for a series of commits.
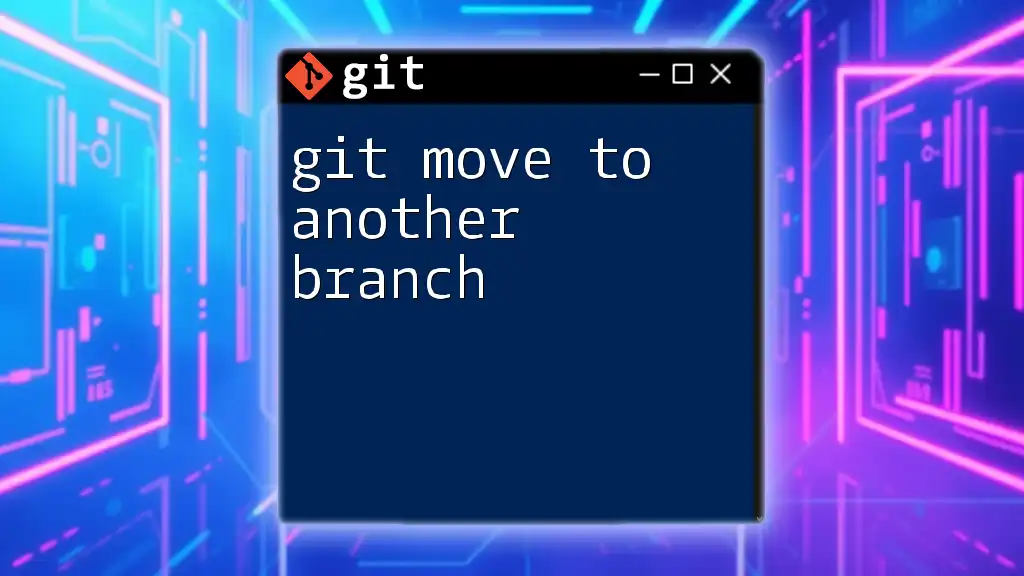
Conclusion
Mastering how to move commits between branches is vital for maintaining a clean and understandable project history in Git. By utilizing methods like cherry-picking and rebasing, you can ensure that your code organization reflects your development workflow accurately. As you become more familiar with these commands, you will find that your ability to manage and collaborate on projects will improve significantly.
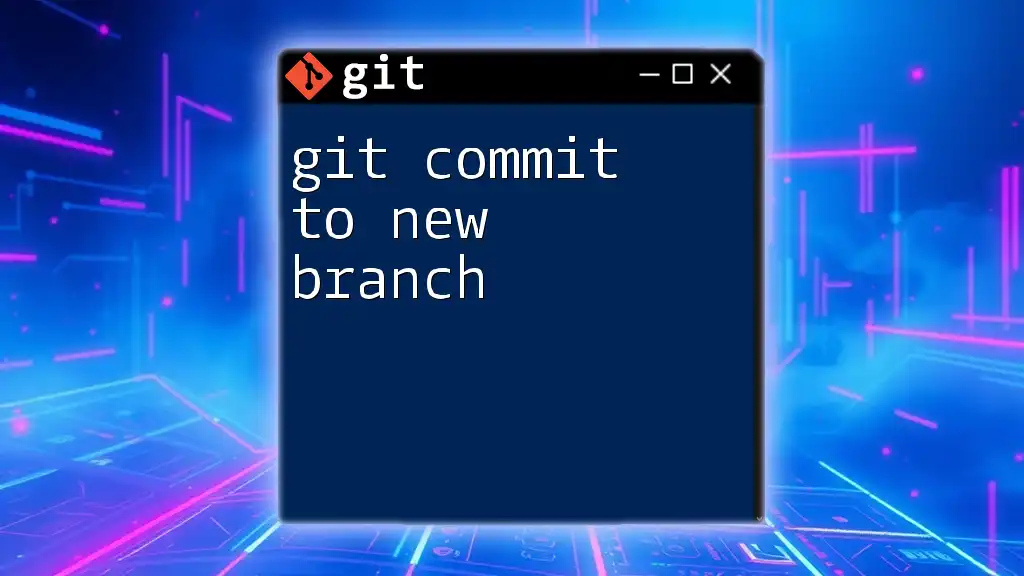
Additional Resources
For further learning, consider exploring articles on Git workflows, interactive rebasing, and commit message best practices. Engaging with community forums can also help you refine your Git skills.
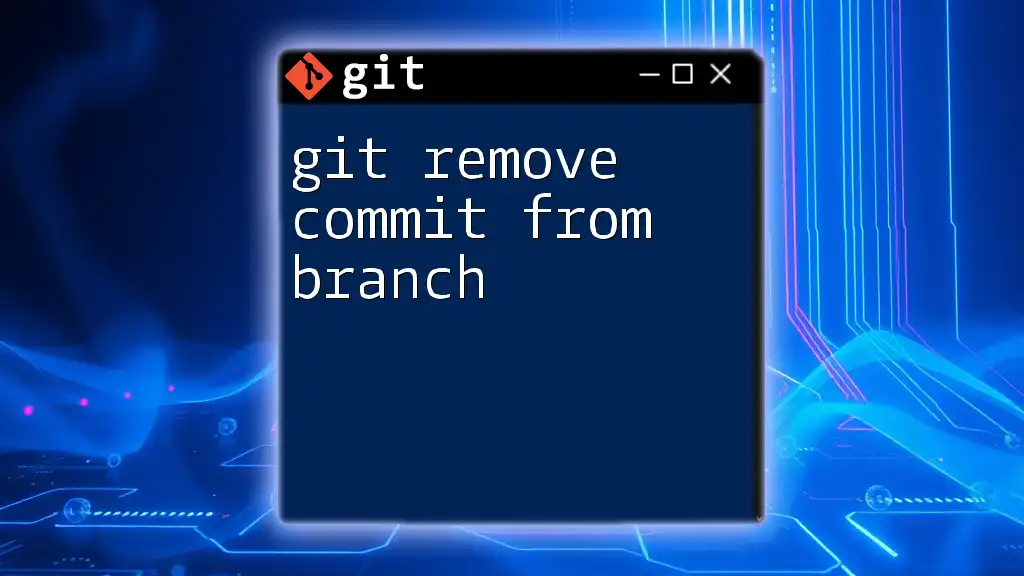
Call to Action
Have you had experiences moving commits between branches? Share your stories in the comments below! Don’t forget to check out our courses to elevate your Git command knowledge and become an expert in version control.