To merge another branch into your current branch in Git, use the following command to ensure that all changes from the specified branch are integrated.
git merge <branch-name>
What is a Branch in Git?
A branch in Git is essentially a pointer to a specific commit in the repository's history. It allows developers to diverge from the main line of development without affecting the main project. This isolation is critical when working on features, bug fixes, or experiments simultaneously.
Purpose of Branching
Branching serves several important purposes:
- Isolation: Each branch can contain different versions of the code, allowing multiple features or fixes to be developed in parallel without interference.
- Collaboration: Developers can work independently on feature branches and share their progress without disrupting others.
- Experimentation: New ideas can be tried out in a separate branch, and if they don’t pan out, they can be discarded without affecting the stable codebase.
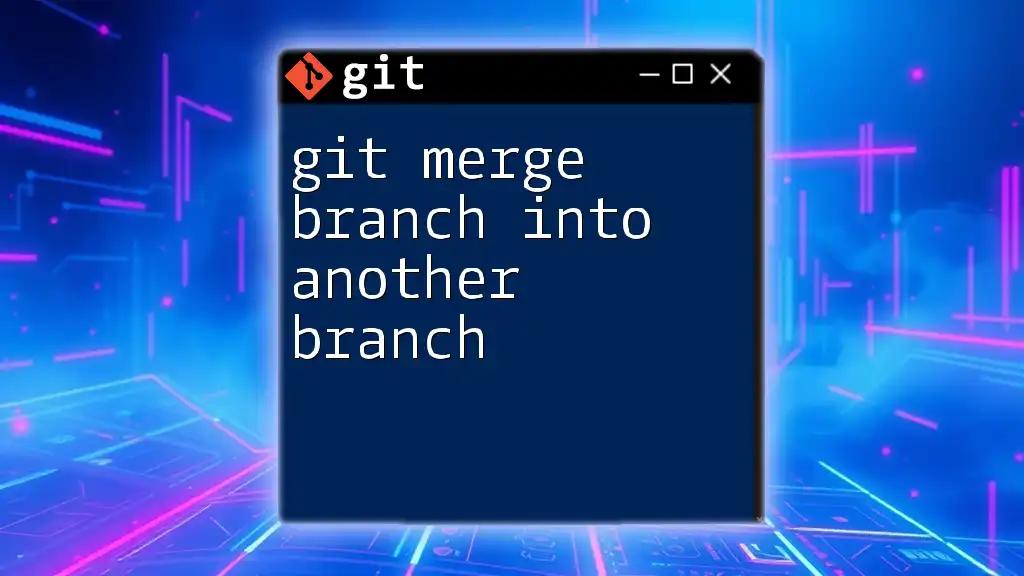
Preparing for a Merge
Before performing a merge, it's essential to ensure that your current branch is up to date and ready for changes.
Checking Your Current Branch
To check which branch you're currently on, you can use the following command:
git branch
This will display a list of all branches, with an asterisk (*) next to the current branch. Knowing your current branch is vital for understanding where the changes will be merged.
Pulling the Latest Changes
It’s best practice to ensure your current branch is updated with the latest changes from the remote repository before merging. You can do this by running:
git pull
This command fetches and integrates any changes from the remote branch into your current branch, reducing the risk of conflicts during the merging process.
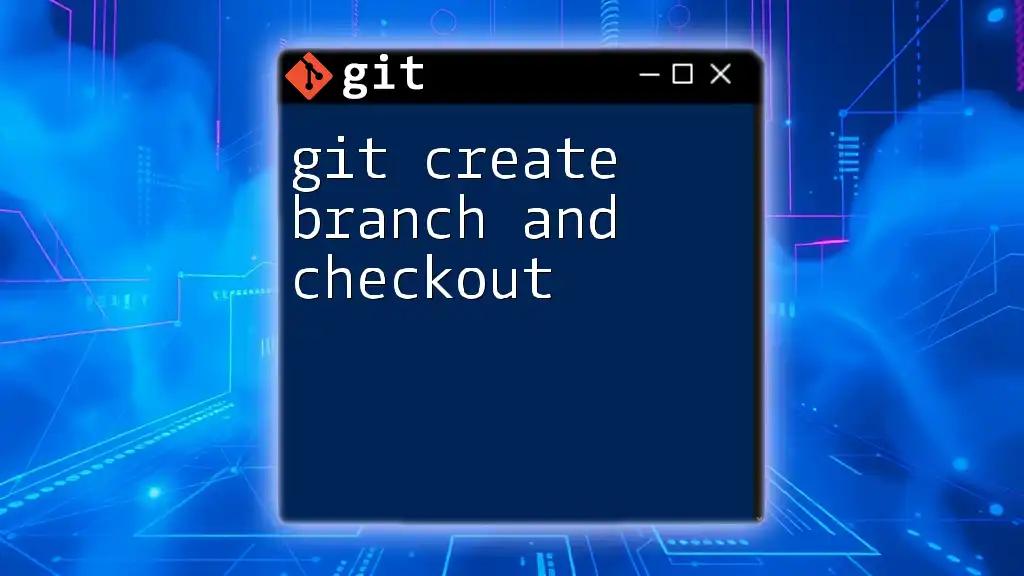
Performing the Merge
Now that you’re prepared, let's dive into the actual merging process.
Basic Merge Command
To merge another branch into your current branch, you use:
git merge <branch-name>
This command consolidates the changes from `<branch-name>` into your current branch. For instance, if you want to merge a feature branch called `feature-branch`, you would run:
git merge feature-branch
This command brings all the commits from `feature-branch` and integrates them into your current working branch.
Fast-Forward Merges
A fast-forward merge occurs when the current branch is directly behind the branch being merged without any additional commits. In such cases, Git simply moves the pointer of the current branch forward to the last commit of the merged branch.
To perform a fast-forward merge, use:
git merge --ff feature-branch
If a fast-forward merge is possible, it keeps the commit history linear without additional merge commits.
Three-Way Merges
When the branches have diverged, Git employs a three-way merge strategy. This combines the snapshots of both branches and their common ancestor. When you want to ensure a merge commit is created, you can use:
git merge --no-ff feature-branch
This command creates a merge commit even if a fast-forward is possible. It provides a clear record in your commit history of when the branches were merged, which can be helpful for reviewing project history.
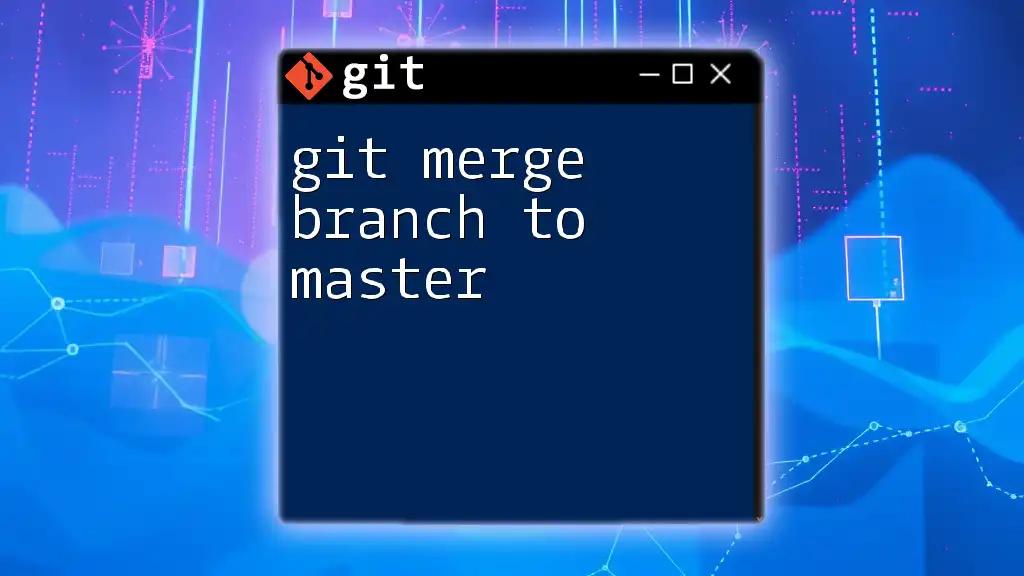
Handling Merge Conflicts
What is a Merge Conflict?
A merge conflict occurs when two branches have competing changes to the same line in a file, or one branch modifies a file while the other deletes it. In such cases, Git cannot automatically reconcile these differences, and it requires manual intervention to resolve them.
Identifying Conflicts
To identify files with merge conflicts after attempting to merge, use:
git status
This command will show a summary of files and highlight any that have conflicts, indicated as "unmerged paths."
Resolving Merge Conflicts
-
Open the Conflicted File: For instance, if you are alerted to a conflict in `conflicting-file.txt`, open this file in your text editor.
-
Edit the Conflicts: You will see conflict markers indicating the differing changes. They look like this:
<<<<<< HEAD
Your changes here
======
Changes from feature-branch
>>>>>> feature-branch
Manually choose how to combine the changes and delete the conflict markers.
- Stage the Resolved Files: After resolving the conflicts, you need to add the file back to the staging area:
git add conflicting-file.txt
- Complete the Merge: Finally, finish the merge process with:
git commit
This creates a new commit that finalizes the merge after all conflicts have been resolved.
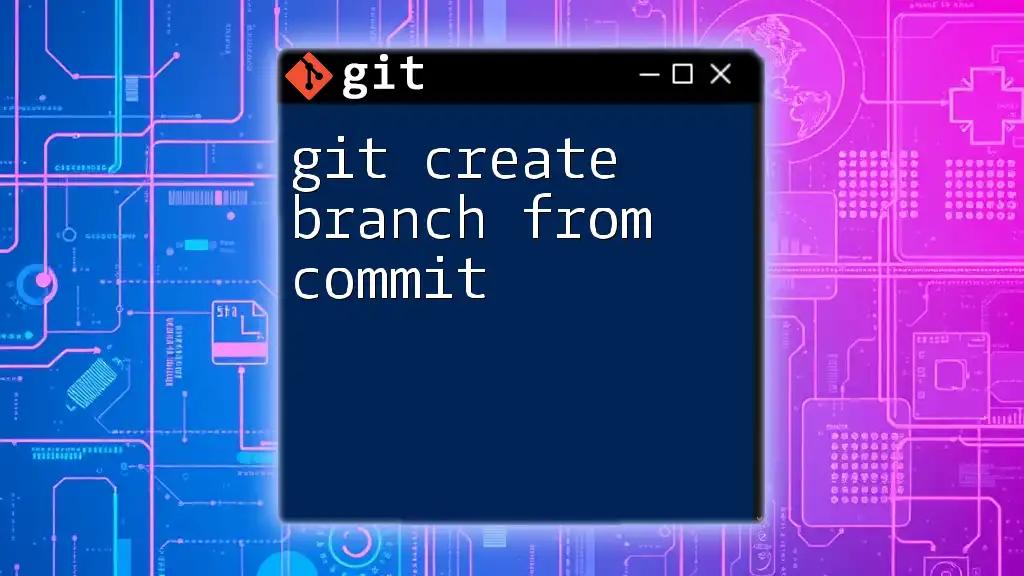
Best Practices for Merging
-
Commit Before Merging: Always commit your changes to avoid losing work. If you are working in a clean state, Git can handle merges more efficiently.
-
Frequent Merges: Regularly merging branches can minimize conflicts. Instead of merging once a large feature is complete, merge often to keep changes integrated smoothly.
-
Use Descriptive Commit Messages: When completing a merge, include clear commit messages to explain what has been merged and why. This aids in tracking changes over time.
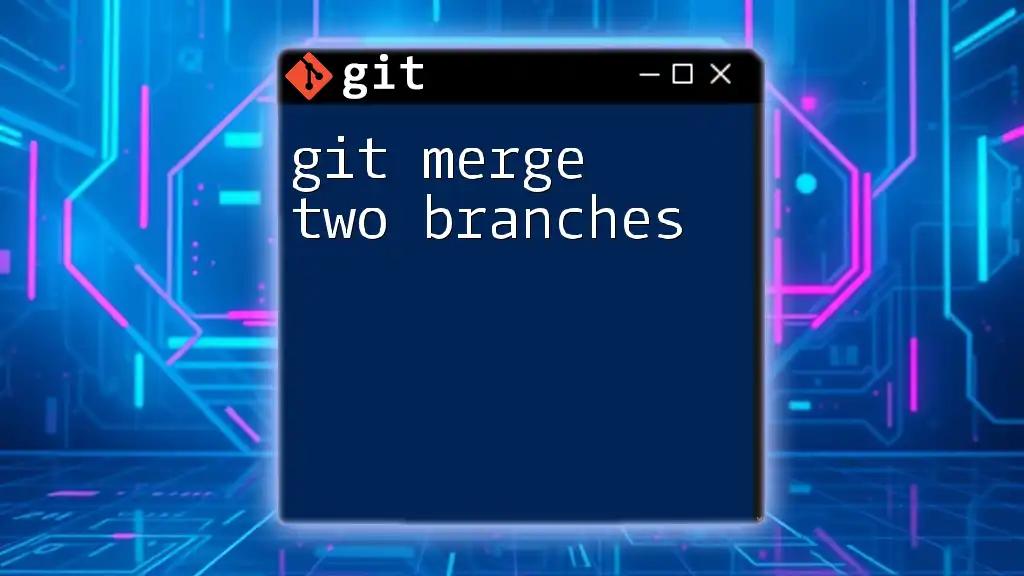
Post-Merge Actions
Verifying the Merge
After merging, it's essential to verify that the merge has gone successfully:
- To view the commit history and ensure the merge is recorded:
git log
- To check the actual changes introduced by the merge, you can also run:
git diff HEAD~1 HEAD
This shows the differences introduced by the merge.
Pushing Changes to Remote
Once you are satisfied with the merge, don’t forget to update the remote repository. Use:
git push origin <branch-name>
This command pushes your changes to the specified branch on the remote repository, making your merged work accessible to others.
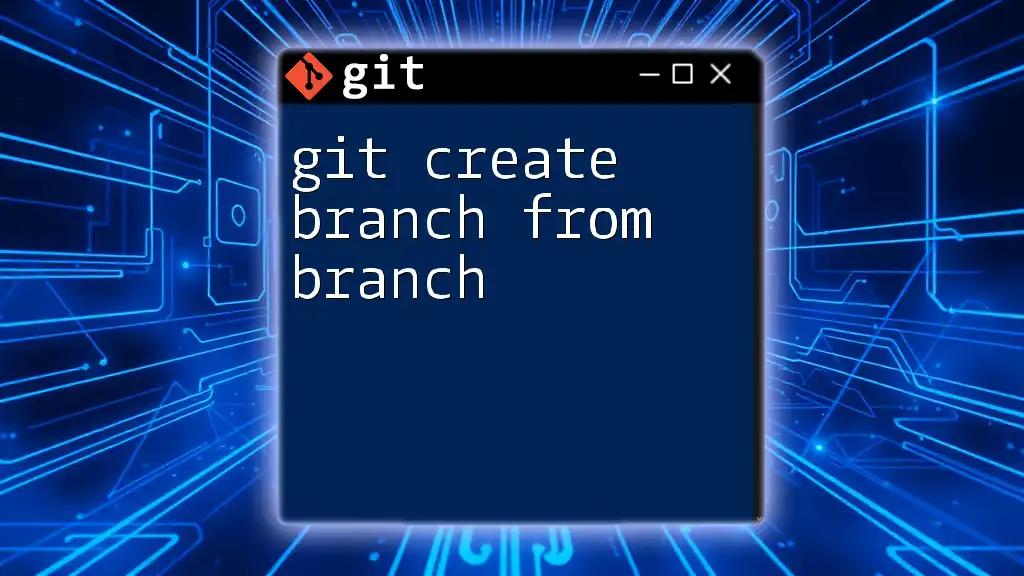
Conclusion
Merging branches in Git is a critical skill for any developer working in collaborative environments. Understanding how to successfully merge another branch into your current one, manage conflicts, and follow best practices can significantly improve your workflow. Remember, practicing these merging techniques in real projects will solidify your comprehension and confidence in using Git commands.
Engage more with your skills and consider joining us for further learning experiences with Git and version control!