To merge one branch into another in Git, you can use the `git merge` command followed by the name of the branch you want to merge.
Here’s a code snippet demonstrating how to merge a branch named `feature-branch` into your current branch:
git merge feature-branch
Understanding Git Branches
What is a Git Branch?
A branch in Git represents an independent line of development. It allows developers to work on isolated changes without affecting the main codebase. Each branch can contain its own set of changes, and once development is stable, these changes can be integrated back into the main branch.
The Importance of Merging
Merging is the process of combining the changes from one branch into another. It’s crucial in collaborative workflows, especially when multiple developers are working simultaneously on different features or bug fixes. Merging enables you to incorporate finished features from feature branches into the main branch, keeping the project organized and up to date.
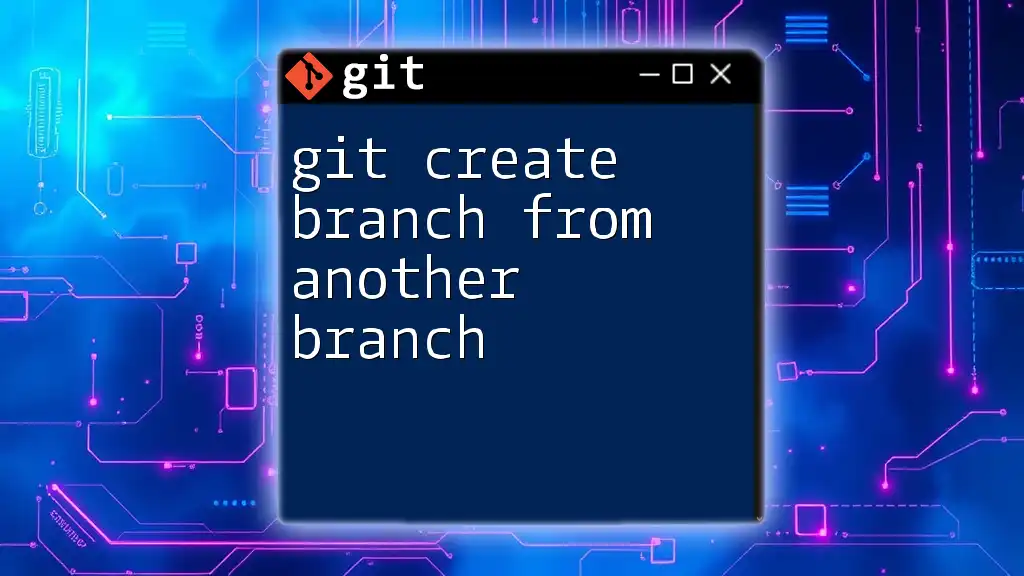
Preparing for a Merge
Ensuring a Clean Working Directory
Before merging, it's essential to have a clean working directory. A clean state means all changes are either committed or stashed.
To check the status of your working directory, use:
git status
This command will outline any uncommitted changes, helping you confirm whether you are ready to proceed with the merge.
Committing Changes
If you have uncommitted changes, you’ll need to commit or stash them first. Committing ensures that any modifications you’ve made are saved, while stashing can temporarily store your modifications for later use.
To commit your changes, follow these commands:
git add .
git commit -m "Your commit message here"
This sequence of commands stages all changes and commits them with a clear message summarizing the changes made.
Identifying the Branches to Merge
Before merging, identify which branches you are working with. Make sure you have a clear naming convention for your branches such as feature/ for new features or bugfix/ for bug fixes. This helps maintain organization and clarity across your project.
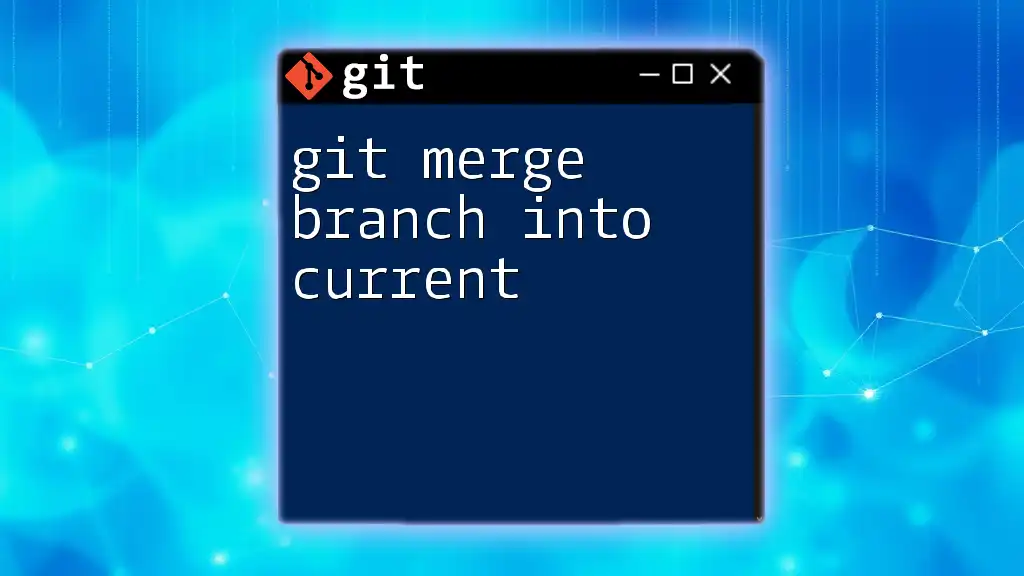
Performing the Merge
Basic Merge Command
The basic command to merge one branch into another is straightforward. First, you need to switch to the branch you want to merge into (the target branch) and then run the merge command.
Here’s how to do it:
git checkout target-branch
git merge source-branch
In this command, target-branch is the branch you want to merge changes into, and source-branch is the branch you want to merge from. After executing these commands, Git will combine the changes and update the target branch accordingly.
Handling Merge Conflicts
What are Merge Conflicts?
A merge conflict arises when changes made in two branches are incompatible. For example, if two developers change the same line in a file differently, Git can't automatically decide which version to keep, leading to a conflict.
Resolving Merge Conflicts
When conflicts occur, Git will notify you, and it’s crucial to resolve these before continuing. To identify conflicts, you can use:
git status
Git will mark the files with conflicts. You will need to open these files, and you’ll see conflict markers that indicate the different changes. For instance:
<<<<<<< HEAD
Your changes here
=======
Changes from the source branch here
>>>>>>> source-branch
To resolve the conflict, manually edit the file to choose the desired version of the changes, removing the conflict markers. Once resolved, stage the file to mark it as resolved:
git add resolved-file
Finally, commit your merge:
git commit -m "Resolved merge conflict"
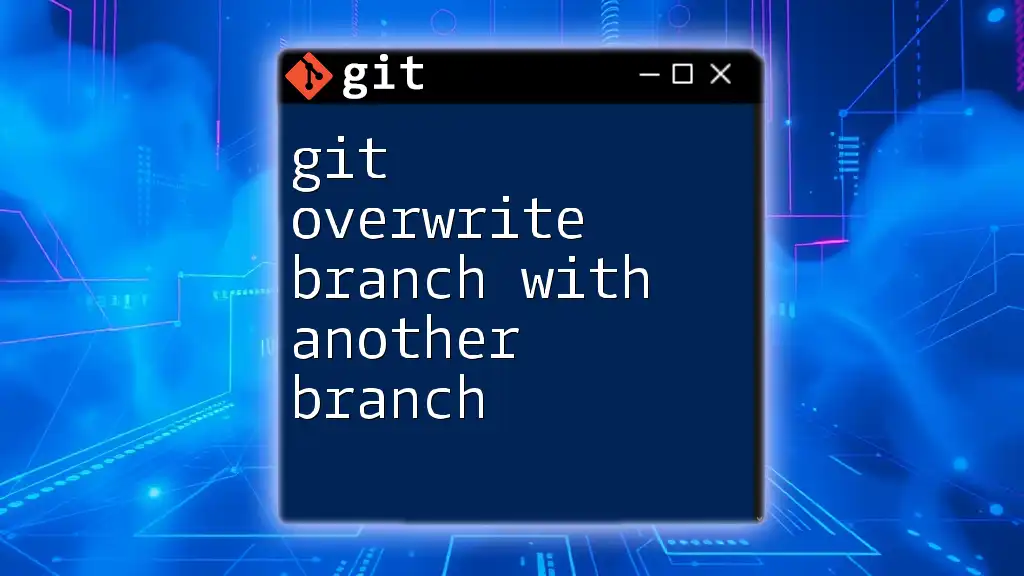
Post-Merge Practices
Verifying the Merge
It is essential to verify the outcome of your merge. After merging, test your project to ensure that everything is functioning as expected. Review the commit history using:
git log
This command will show you a list of commits, including your latest merge commit.
Cleaning Up
Once the merge is done and verified, consider deleting the source branch if it is no longer needed. This helps keep the repository tidy and manageable. To delete a merged branch, use:
git branch -d source-branch
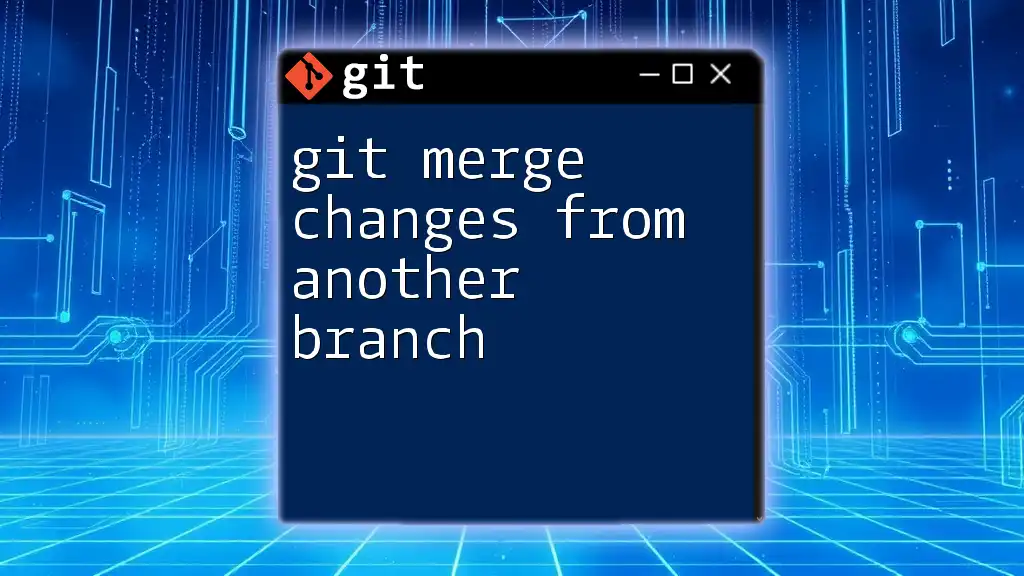
Best Practices for Merging
Keeping Branches Up-to-Date
Regularly update your feature branches with changes from the main branch to minimize the chances of conflicts when it comes time to merge. This can be done using:
git checkout feature-branch
git merge main-branch
Squashing Commits
When merging, you might find it beneficial to squash commits. Squashing combines multiple commits into a single commit, making the project history cleaner and easier to read. To do this, you would run:
git merge --squash source-branch
This command merges the changes but doesn’t create a new commit, allowing you to finalize the commit message as needed.
Documenting Merges
Always document your merges in the commit messages. A clear note on what changes were made and why will help other collaborators understand the project history better and navigate future merges with ease.
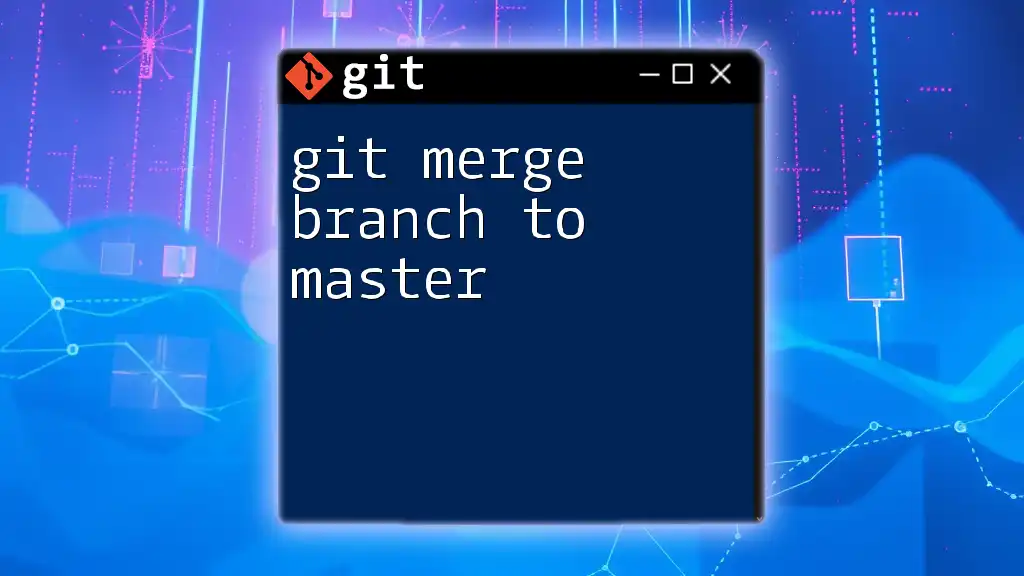
Conclusion
Merging in Git is a critical skill that, when mastered, can significantly enhance collaboration and project management. By understanding branches, preparing for merges, and following best practices, you can effectively manage changes and maintain a clean project history. Remember that practice makes perfect; the more you work with merges, the more comfortable you will become. Happy merging!
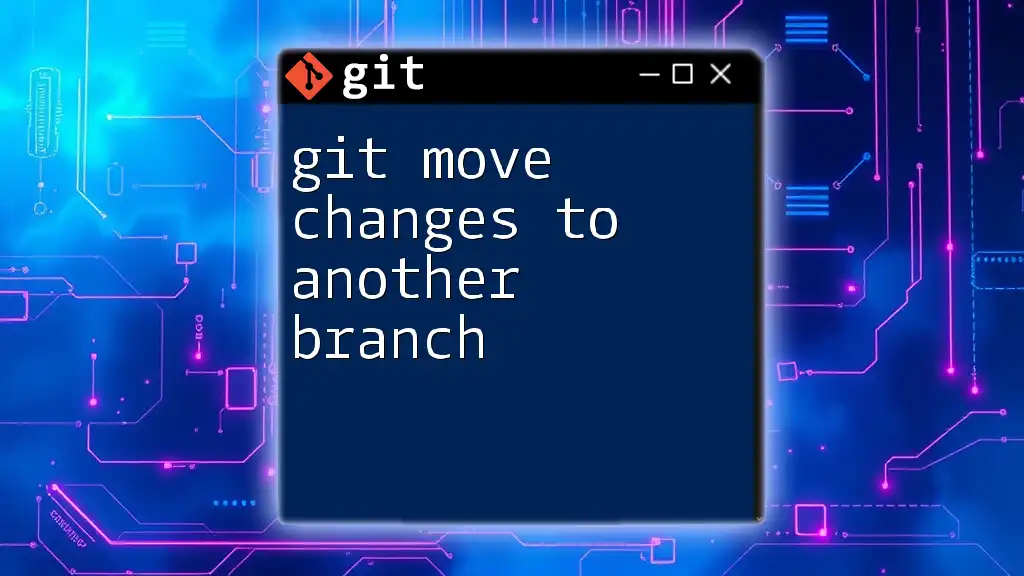
Additional Resources
Recommended Reading
For further insights into Git and version control practices, explore additional literature and online resources tailored for developers.
Tutorials and Tools
Engage with interactive platforms that provide hands-on experiences to practice Git commands proficiently. These tools can help reinforce your Git skills and prepare you for real-world scenarios.