To merge changes from another branch into your current branch in Git, use the `git merge` command followed by the name of the branch you want to merge. Here's the command in a code snippet:
git merge <branch-name>
Understanding Branches in Git
What is a Branch?
In Git, a branch is essentially a pointer to a snapshot of your changes. The primary branch (often called `main` or `master`) represents the production-ready state of your project. Other branches allow developers to work simultaneously on different features, fixes, or experiments without interfering with one another's work.
Common Use-Cases for Branches
Branches serve multiple purposes in Git. Here are some common scenarios:
-
Feature Development: When developing a new feature, a developer can create a new branch to isolate their work. This ensures that the new code doesn’t affect the stability of the main branch until it is ready.
-
Bug Fixing: Similar to feature development, when a bug is identified, developers can create a dedicated branch to address it, allowing for focused and safe changes.
-
Experimentation: Creating experiments in separate branches enables developers to try out new ideas without any risk to the main codebase.
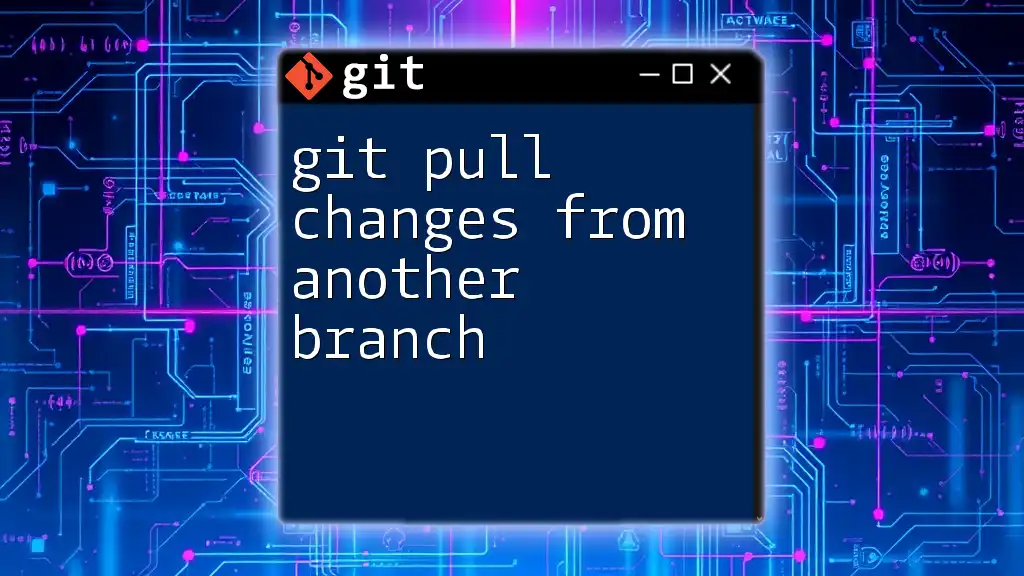
The Git Merge Command
What is Git Merge?
The `git merge` command is the tool used to integrate changes from one branch into another. It’s vital in collaborative environments, where multiple developers work on separate branches and need to bring their changes together regularly.
Merging is often preferable to rebasing because it preserves the history of the work done. This can be particularly beneficial for code reviews or understanding the evolution of the code when looking back over time.
Types of Merges
Fast-Forward Merge
In a fast-forward merge, the branch being merged has all the latest commits from the target branch. When you perform a fast-forward merge, Git simply moves the branch pointer forward to the latest commit. This is generally straightforward and does not create a new merge commit.
For example, if you are on the `main` branch and you want to merge a feature branch `feature-branch`:
git checkout main
git merge feature-branch
If `main` has not diverged from `feature-branch`, this will result in a fast-forward merge.
Three-Way Merge
A three-way merge occurs when both branches have diverged and contain distinct commits. Git carves out a common ancestor, taking the union of the changes from the two branches and then combining them.
To perform a three-way merge, the command is the same as with a fast-forward merge, but when the branches diverge, Git will create a merge commit.
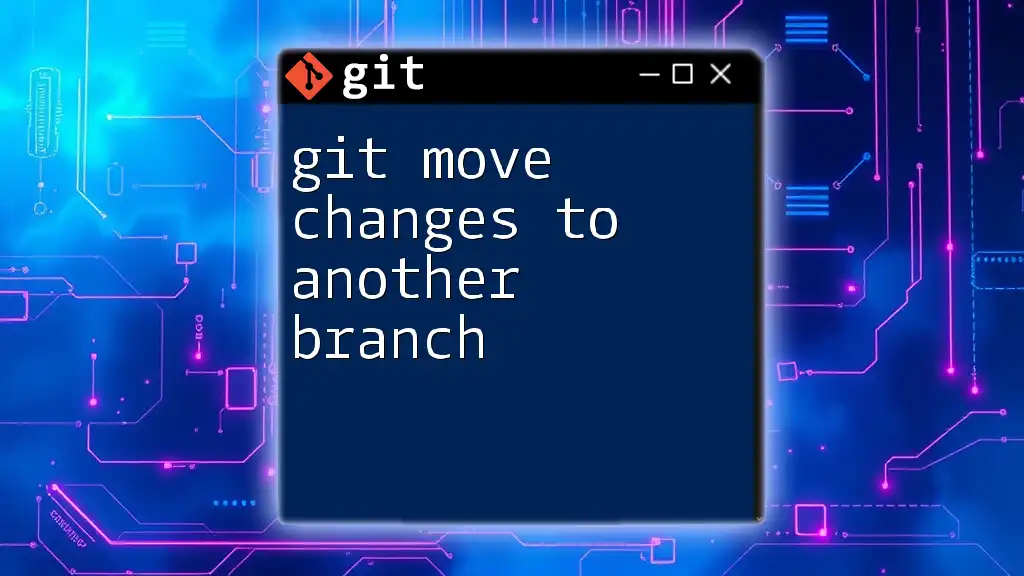
Preparing to Merge
Check Your Current Branch
Before merging, it is crucial to check which branch you are currently on, as you cannot merge changes into the wrong branch. You can do this using the `git status` command:
git status
If you see a message indicating you are on `main`, this means any merges will be directed into the `main` branch.
Fetching the Latest Changes
To avoid merging outdated changes, it's essential to ensure your branches are fully updated. The difference between `git fetch` and `git pull` is noteworthy:
- `git fetch` only downloads updates from the remote repository without merging them into your local files.
- `git pull` combines fetching and merging in one command. It updates your local branch immediately.
To fetch changes from the remote:
git fetch origin
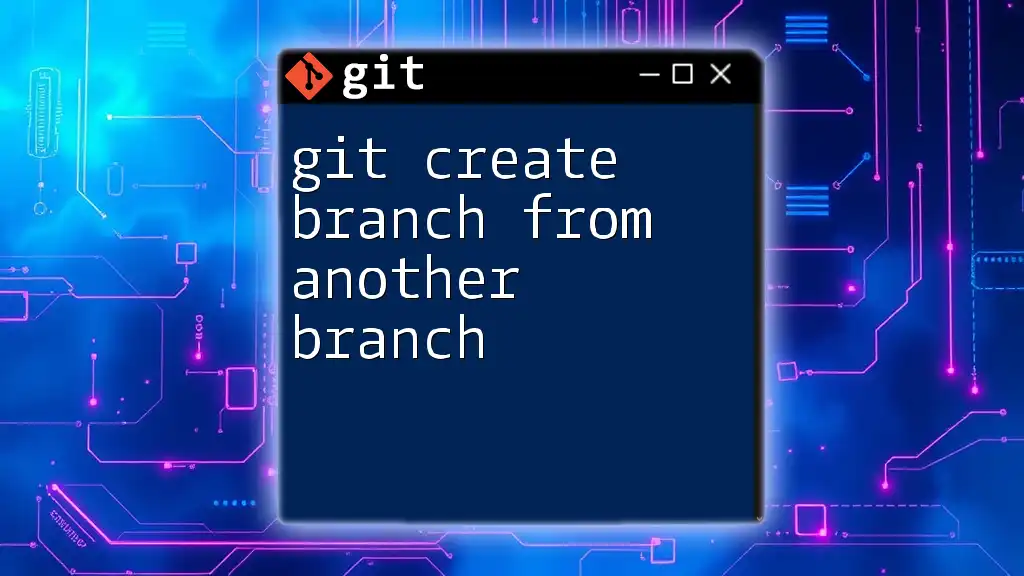
Merging Changes from Another Branch
Basic Merge Syntax
To merge changes from one branch into another, you need to check out the target branch (where you want to bring the changes) and run the merge command:
git checkout main
git merge feature-branch
This command integrates the changes from `feature-branch` into `main`. If there are no conflicts, Git will create a merge commit.
Handling Merge Conflicts
What are Merge Conflicts?
Merge conflicts occur when changes in different branches affect the same lines of code or the same files, and Git cannot determine how to automatically combine them.
When a conflict arises, Git will pause the merge and mark the conflicted files in your working directory.
How to Resolve Merge Conflicts
Resolving merge conflicts involves several steps:
-
Identify Conflicted Files: After a merge conflict, Git will notify you of files with conflicts. You can see the status using:
git status
-
Manual Resolution: Open the conflicting files, and you will see conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`). Edit the files to resolve the conflicts. After making your decisions on what to keep, save the files.
-
Add the Resolved Files:
After resolving conflicts, stage the resolved files with:
git add <file-name>
-
Commit the Merge:
Finally, commit the merge to complete the process:
git commit -m "Resolved merge conflicts"
Finalizing the Merge
After merging and resolving any conflicts, it’s essential to review all changes carefully before finalizing the merge with a commit. Commit messages should be clear and informative, reflecting which branches were merged and any significant changes made.
git commit -m "Merged feature-branch into main"
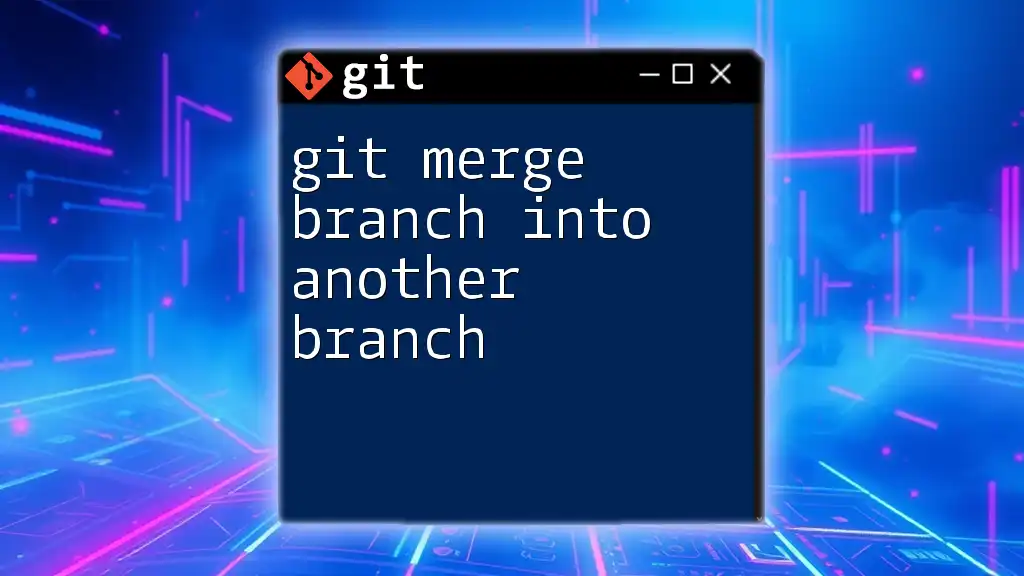
Best Practices for Merging
Keeping Your Branches Up-to-Date
To avoid complex conflicts, consider merging your main branch back into your feature branches regularly. This practice helps ensure that your feature branch remains compatible with the latest changes from the main codebase.
Documenting Merges
Documenting your merges is vital for clarity and accountability. Use descriptive commit messages to summarize what changes were merged and why. This practice will be extremely helpful for team collaborations in the future.
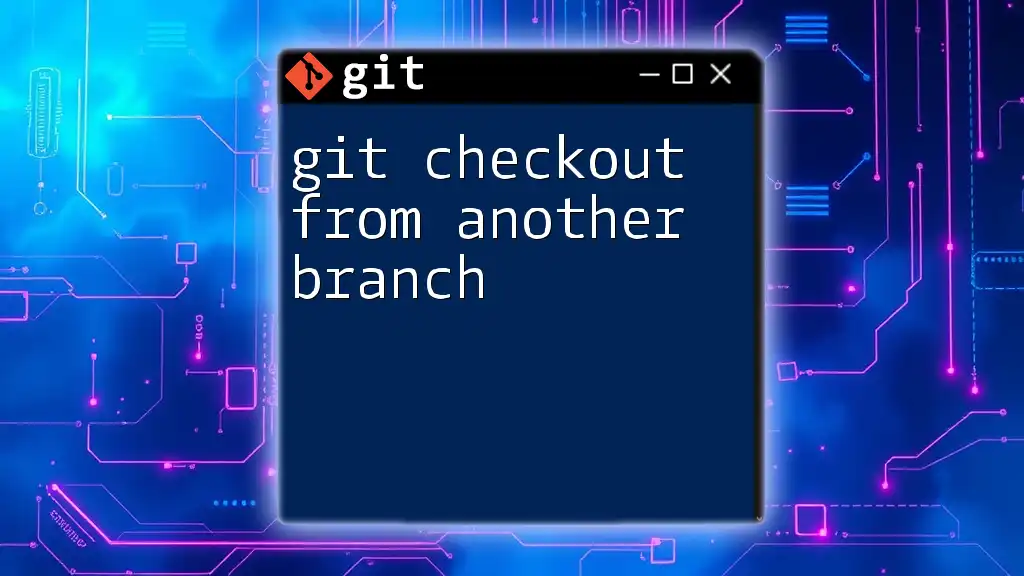
Advanced Merge Strategies
Using Merge Tools
Git offers the flexibility to use external merge tools that can simplify the conflict resolution process. Tools like Meld or KDiff3 provide visual interfaces to resolve conflicts.
To configure an external merge tool, you typically adjust your Git config like so:
git config --global merge.tool meld
Interactive Merging
The `git merge --squash` option allows you to combine multiple commits from the feature branch into one single commit on the target branch. This is particularly useful for keeping a clean history.
git checkout main
git merge --squash feature-branch
This combines all changes without retaining individual commit history, giving you the option to create a cleaner commit message.
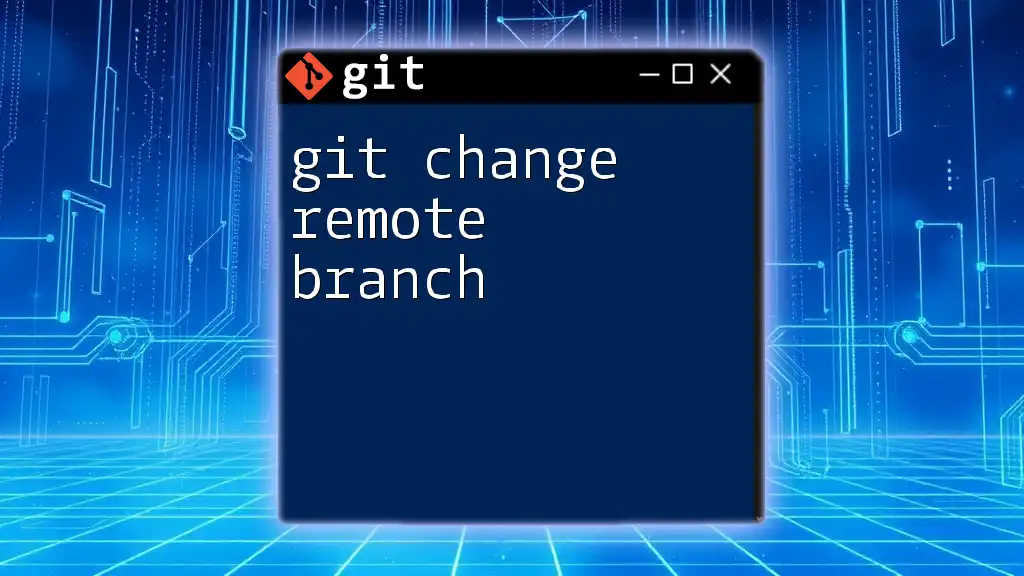
Conclusion
Merging changes from another branch in Git is a fundamental skill for effective collaboration in software development. By understanding how merging works, knowing how to handle conflicts, and adhering to best practices, you can streamline your development workflow.
Practicing these skills will enhance your proficiency with Git and contribute to more efficient coding practices. As you grow more comfortable with merging, you’ll find that it becomes an integral aspect of your coding routine.
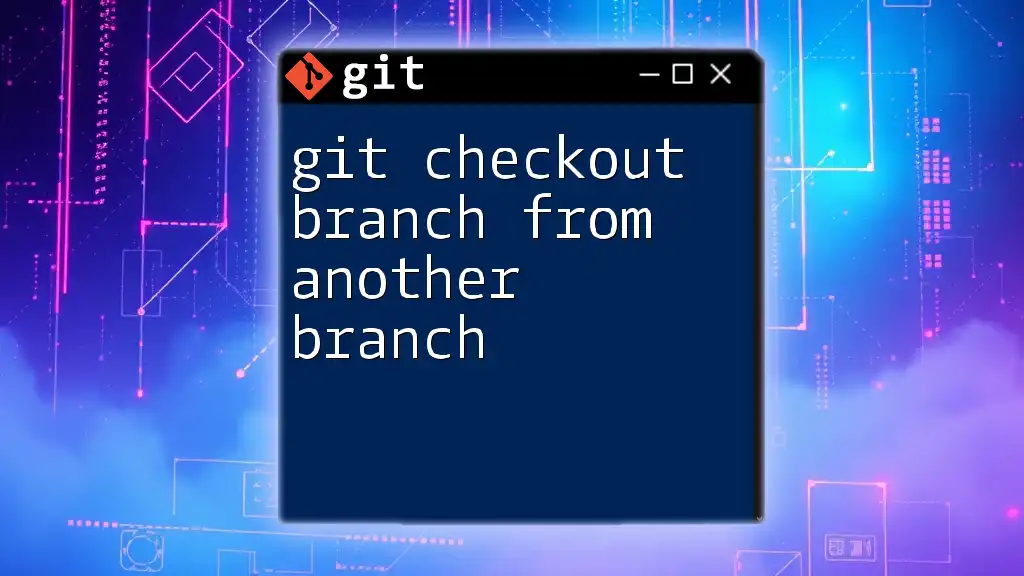
Call to Action
We invite you to share your experiences with merging in Git! What strategies have you found most effective? Consider signing up for more detailed guides and courses for bulk improvements to your Git muscle.