To push your local commits to a specific remote branch in Git, use the following command:
git push origin your-branch-name
Understanding Git Remote
What is a Remote Repository?
A remote repository is a version of your project that is hosted on the internet or another network, allowing multiple people to collaborate on it. This can be contrasted with a local repository, which is the version that exists on your own machine. Remote repositories are important for collaboration among team members who may be working in different locations.
Common Remote Repositories
Popular platforms for hosting remote repositories include GitHub, GitLab, and Bitbucket. Each of these platforms provides tools for version control, issue tracking, and collaborative workflows. Knowing where your code resides and how to manage it on these platforms is crucial for effective collaboration.
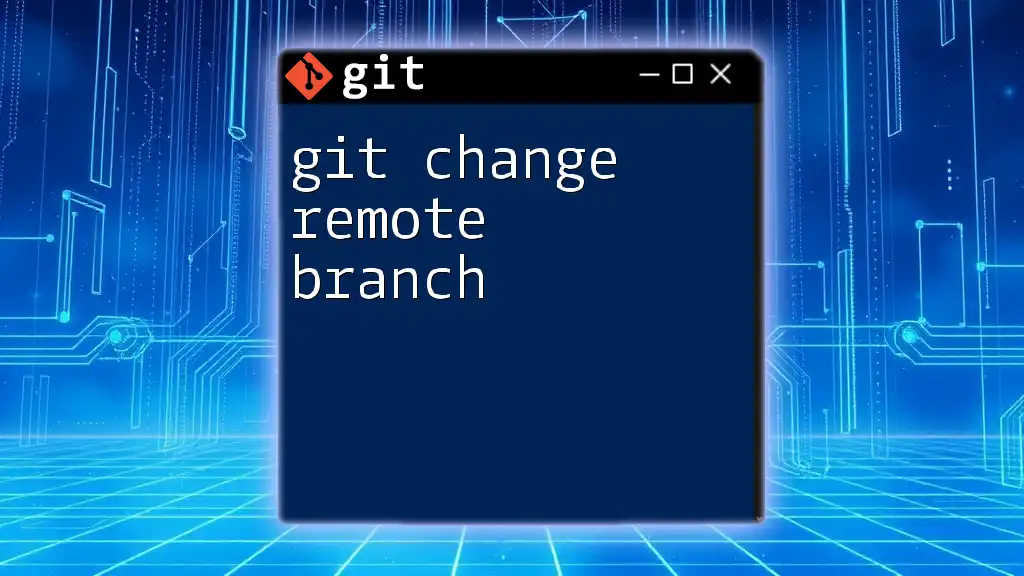
Setting Up Your Remote Repository
Creating a Remote Repository
Creating a remote repository is straightforward. On GitHub, for example, you can follow these steps:
- Sign into your GitHub account.
- Click on the “New” button to create a new repository.
- Enter a name for your repository, add an optional description, choose its visibility (public or private), and initialize it with a README if desired.
- Click “Create Repository” to complete the setup.
Adding a Remote Repository to Your Local Repository
Once your remote repository is created, you need to link it to your local repository. This is done using the following command:
git remote add origin <repository-url>
Replace `<repository-url>` with the actual URL of your remote repository. This command tells Git where to push your changes.
Verifying Remote Configuration
To ensure that your remote repository is correctly configured, you can verify your setup with:
git remote -v
This command displays a list of all remote branches associated with your local repo. You should see your remote repository URL here, confirming that it's configured properly.
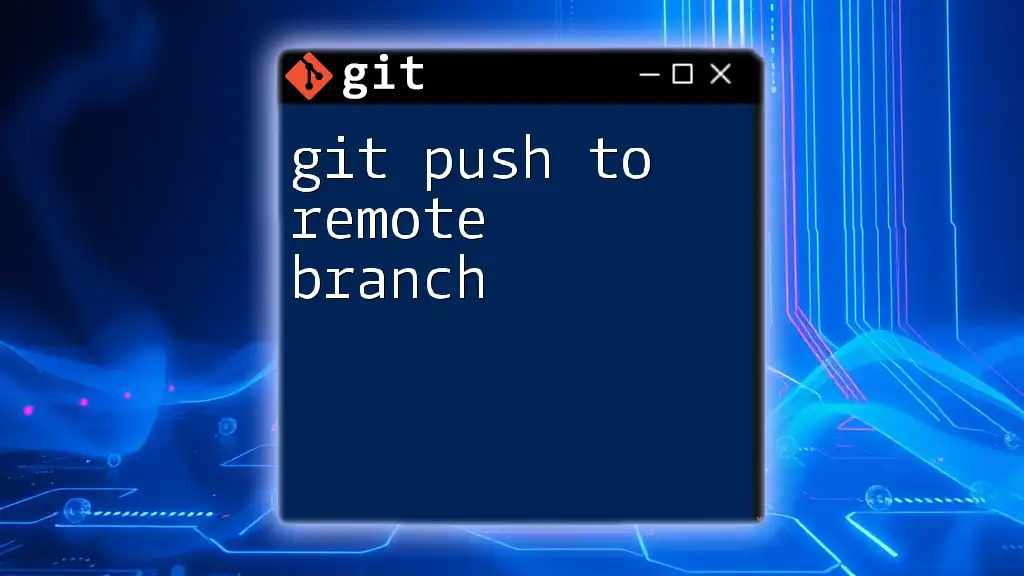
Making Changes Locally
Staging Changes
Before pushing changes, you need to stage them. Staging involves marking changes that you want to include in your next commit. This can be accomplished by using the command:
git add <file>
or, to stage all files:
git add .
Staging allows you to selectively choose which changes are committed, providing flexibility in version control.
Committing Changes
Once changes are staged, you need to commit them to your local repository. Committing is essential as it records the changes in your version history. Use the following command:
git commit -m "Your commit message here"
The commit message should be clear and concise, describing the changes made. A good practice is to use the imperative mood, e.g., "Fix bug" or "Add feature".
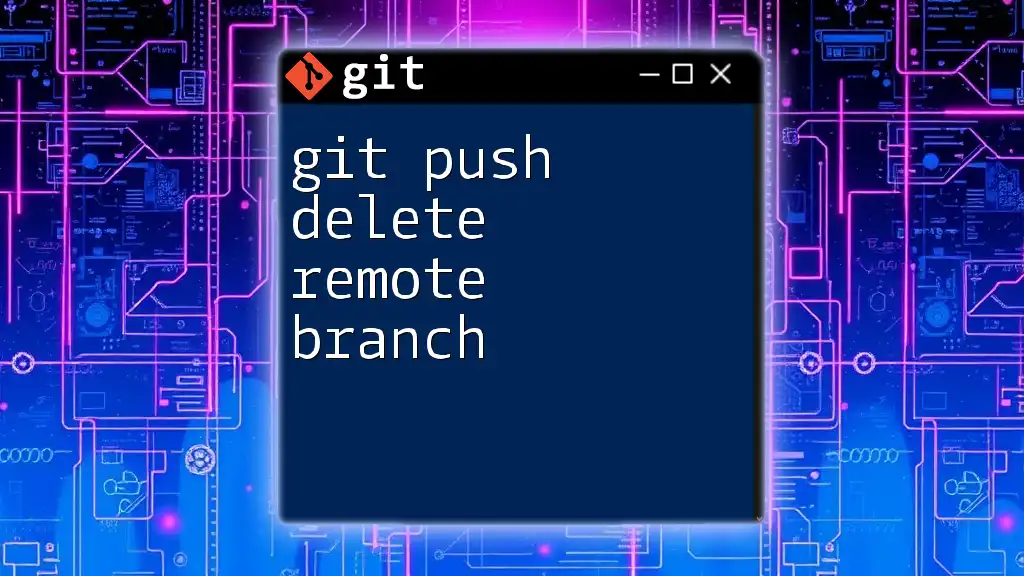
Pushing Changes to a Remote Branch
Preparing to Push
Before pushing your changes to the remote branch, it's vital to ensure your local branch is in sync with the remote. This is particularly important if other collaborators are also making changes. Execute:
git pull origin <branch-name>
This command fetches and integrates changes from the remote branch to your local branch. If there are conflicts, you'll need to resolve them before proceeding to the push.
Executing the Push Command
Once your branch is up to date, you can push your changes to the remote branch using:
git push origin <branch-name>
Replace `<branch-name>` with the name of the local branch you want to push. This command uploads your committed changes to the corresponding branch on the remote repository.
Handling Authentication
When pushing changes, you may encounter authentication prompts. Remember, there are two primary methods: HTTPS and SSH. HTTPS may require you to enter your username and password, whereas SSH uses key-based authentication which is generally more secure. Ensure you have set up your authentication method correctly to avoid interruptions.
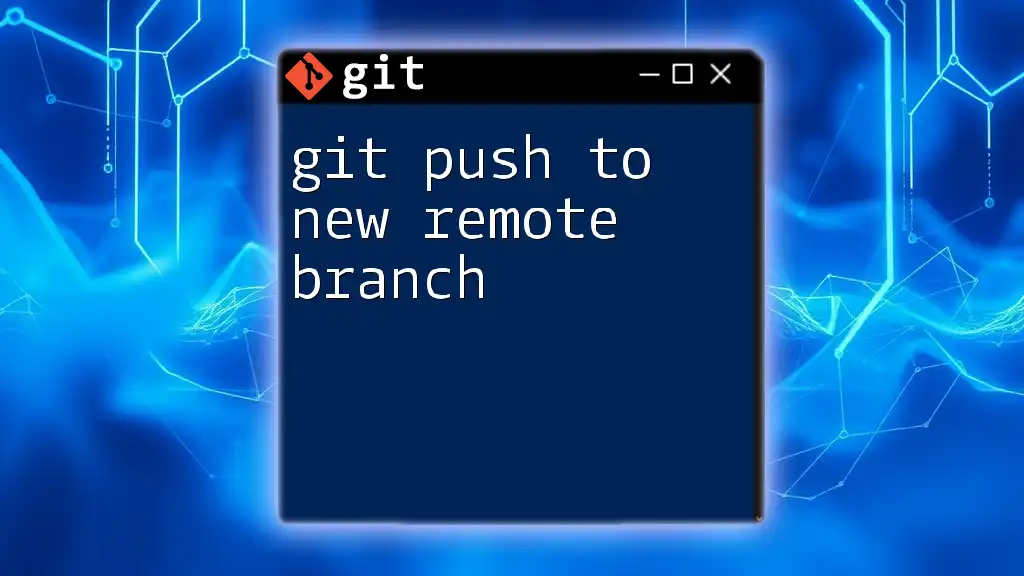
Understanding Push Options
Push Specifications
If you need to push changes from a local branch to a different remote branch, you can do so with:
git push origin <local-branch>:<remote-branch>
This command allows you to specify which local branch should be pushed to which remote branch, giving you control over your code deployment.
Force Pushing with Caution
Sometimes, you may need to overwrite the remote branch with your local changes. This is achieved via a force push, but you must use it wisely as it can lead to lost changes:
git push --force
Force pushing rewrites the history of the remote branch, potentially discarding others' committed changes. Always communicate with your team before doing this to avoid conflicts.
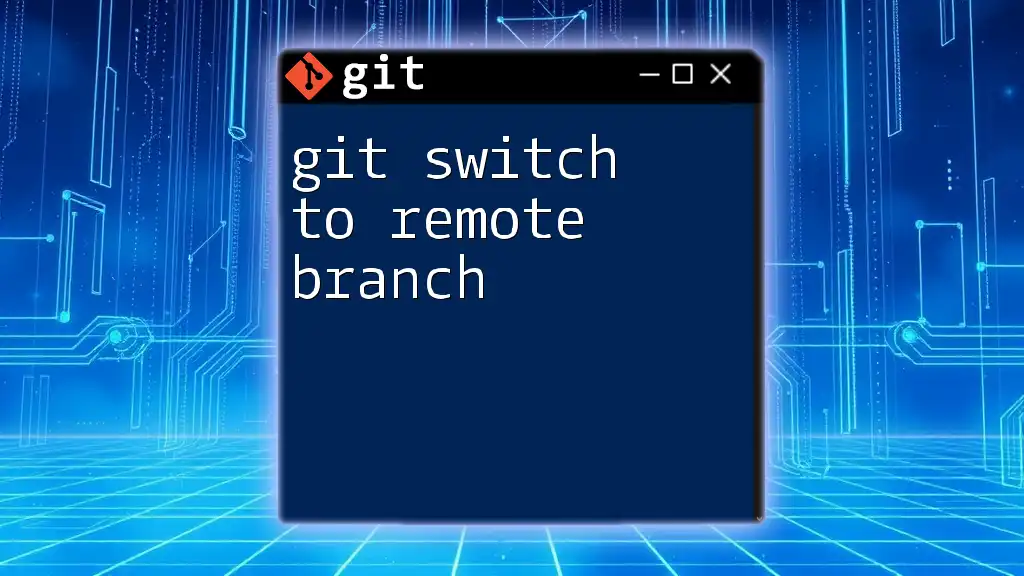
Common Issues and Troubleshooting
Push Rejections
You might encounter a push rejection. This typically happens if your local branch is behind the remote branch. In this case, you need to pull changes first (as explained earlier) before you can successfully push.
Authentication Errors
Authentication errors can occur frequently. These can stem from incorrect usernames/passwords or SSH key issues. Make sure to check your credentials, and if using SSH, verify that your SSH keys are correctly configured on your remote platform.
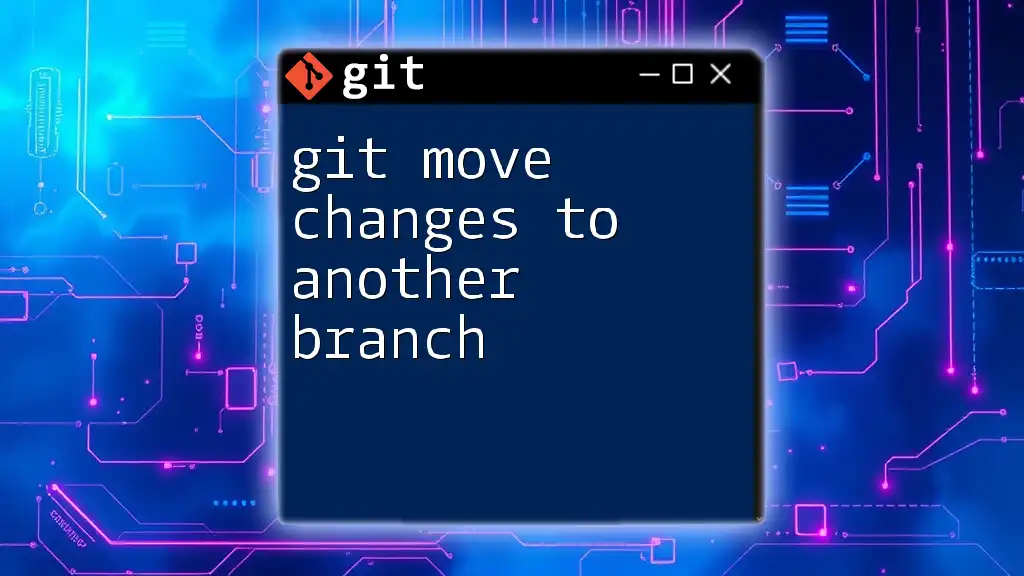
Best Practices for Pushing Changes
Commit Often, Push Often
Adopting the practice of committing your changes frequently and pushing them often keeps your work in sync with the team, reducing the risk of conflict and ensuring that everyone has access to the latest code.
Use Branches Wisely
Working in feature branches rather than directly on the main branch facilitates organized collaboration. By segmenting changes, you reduce the chances of introducing bugs into the production code and maintain a clean project history.
Regularly Syncing with Remote
Regularly syncing with the remote repository helps you stay aligned with your team's work. This habit is crucial for avoiding large merge conflicts that can arise from prolonged periods of divergence between your local and remote branches.
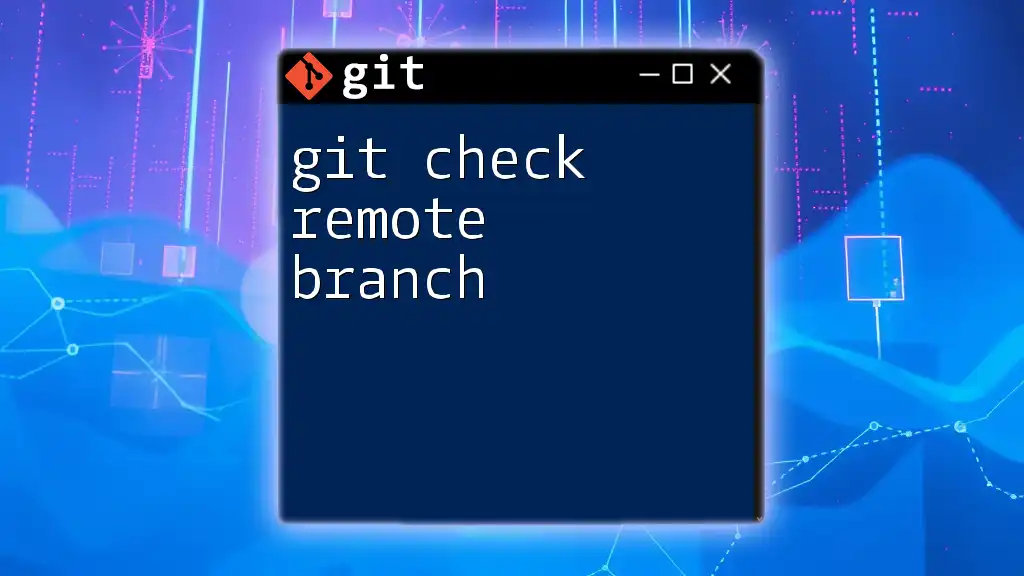
Conclusion
Successfully using the command git push changes to remote branch is essential for modern software development practices. Mastering this process enhances collaboration and ensures that all team members are on the same page. Regular practice and adherence to best practices will make you proficient in using Git and contribute more effectively to your projects.
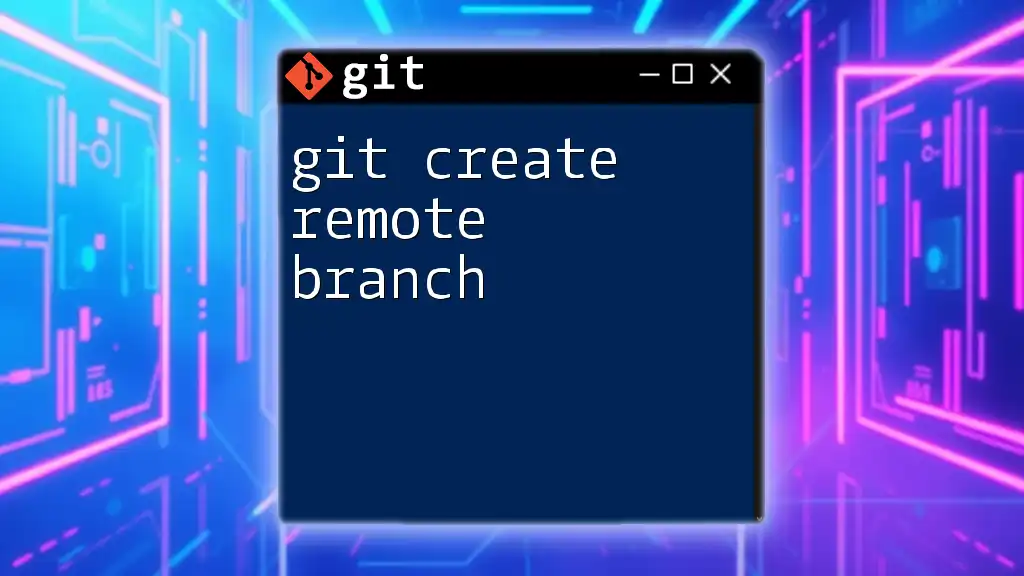
Additional Resources
For a deeper dive into Git, consider exploring the official Git documentation and various online courses that provide insights into advanced Git features and collaborative practices.