To switch to a remote branch in Git, you can use the following command, which checks out the branch from the remote repository to your local environment.
git checkout -b <branch-name> origin/<branch-name>
Understanding Remote Branches
What is a Remote Branch?
A remote branch in Git is a reference to the state of branches in a remote repository. Unlike local branches that exist in your local repository, remote branches represent the work done by other collaborators. They are prefixed with the name of the remote (e.g., `origin/branch-name`) and can be thought of as bookmarks to the last known state of those branches.
Remote branches play a crucial role in collaborative development, allowing multiple developers to work on the same project while maintaining their changes in isolated environments.
How Remote Branches Work
Git tracks branches that exist in remote repositories, providing a mechanism to incorporate changes made by others. Tracking branches are local branches that are linked to a remote branch, allowing you to push and pull changes easily.
Before switching to a remote branch, it’s essential to understand a few key commands:
- `git fetch`: This command updates your local copy of the remote repository, fetching new commits and branches.
- `git pull`: This command fetches updates from a remote branch and merges them into your current branch.
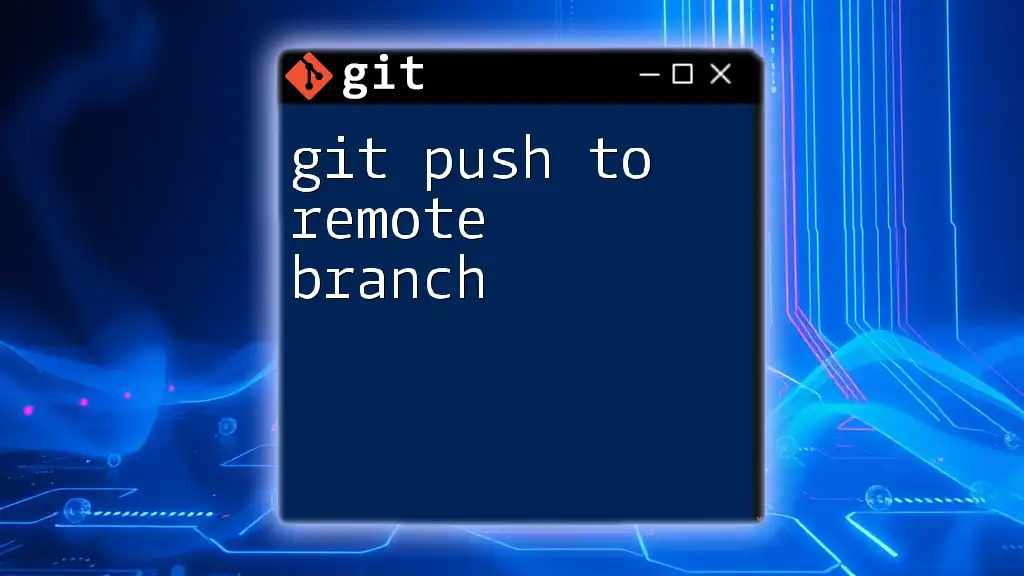
Prerequisites
To effectively switch to a remote branch, you should have:
- Basic knowledge of Git commands: Familiarity with commands like `git clone`, `git fetch`, and `git branch`.
- A local clone of the repository: Ensure that you have the repository cloned to your machine before starting.
- Awareness of available remote branches: Knowing what branches exist will make it easier to switch to the desired one.
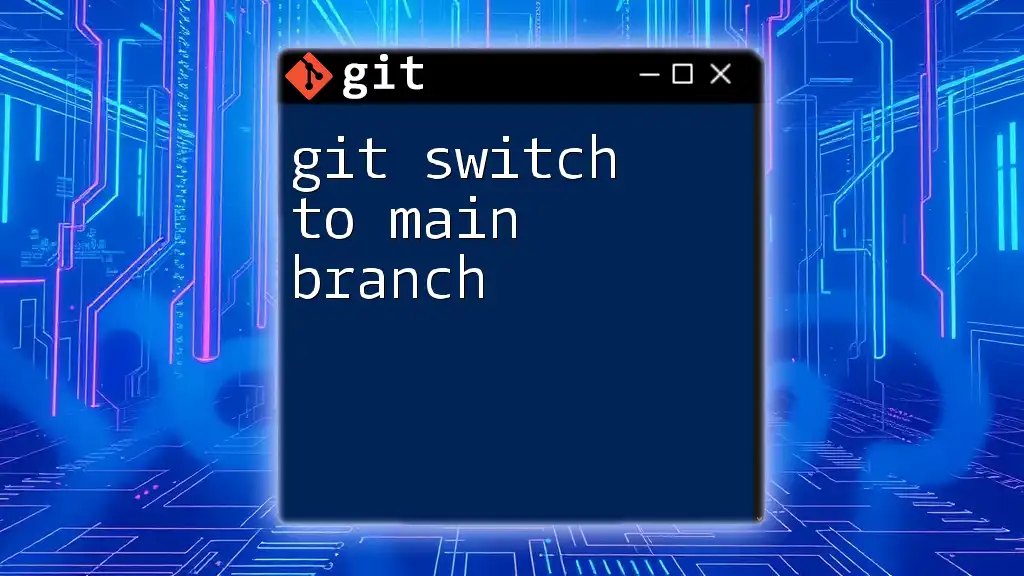
Switching to a Remote Branch with Git Switch
What is `git switch`?
The `git switch` command is specifically designed to facilitate the process of switching branches in Git. It offers a more user-friendly approach compared to the older `git checkout` command, which is used for both switching branches and other purposes like checking out files.
Advantages of using `git switch`:
- Clearer intent: It is intuitive; you know that it’s only for switching branches.
- Reduces confusion: You won't accidentally use it to check out files.
Steps to Switch to a Remote Branch
-
Fetching Remote Branches
Before switching to a remote branch, ensure you have the latest information about remote branches. This is done using the fetch command:git fetch origin
This command fetches updates from the remote repository and updates your local references to the remote branches. Always fetch before switching to ensure you're working with the most current data.
-
Listing Remote Branches
To see all available remote branches, you can list them using:git branch -r
This command displays your remote branches prefixed by the remote's name (e.g., `origin`). This list enables you to identify the branch to which you wish to switch.
-
Switching to the Remote Branch
To switch to a remote branch, you utilize the `git switch` command. Assuming you want to switch to a branch called `feature-branch`:git switch --track origin/feature-branch
By using the `--track` option, you create a local tracking branch that follows the remote branch. This means any future `git pull` or `git push` commands will know which remote branch to interact with.
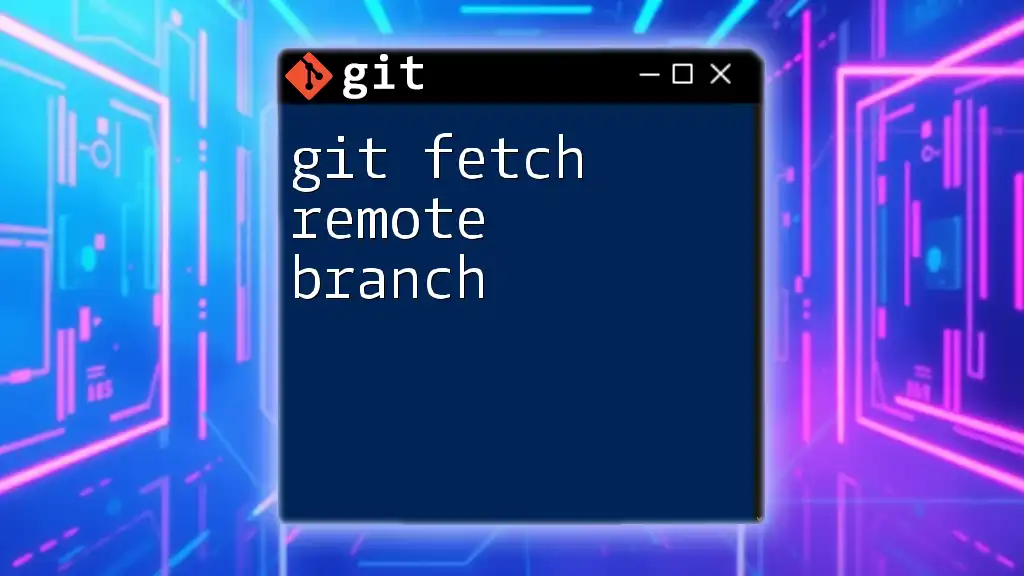
Example Scenario
Imagine you're collaborating on a project where a teammate has created a feature branch called `feature-xyz`. To switch to this branch, follow these steps:
- Fetch updates from the remote repository:
git fetch origin
- List available remote branches to confirm its existence:
git branch -r
- Switch to your teammate's branch:
git switch --track origin/feature-xyz
Upon executing these commands, you are now on `feature-xyz` and can begin contributing to the project.
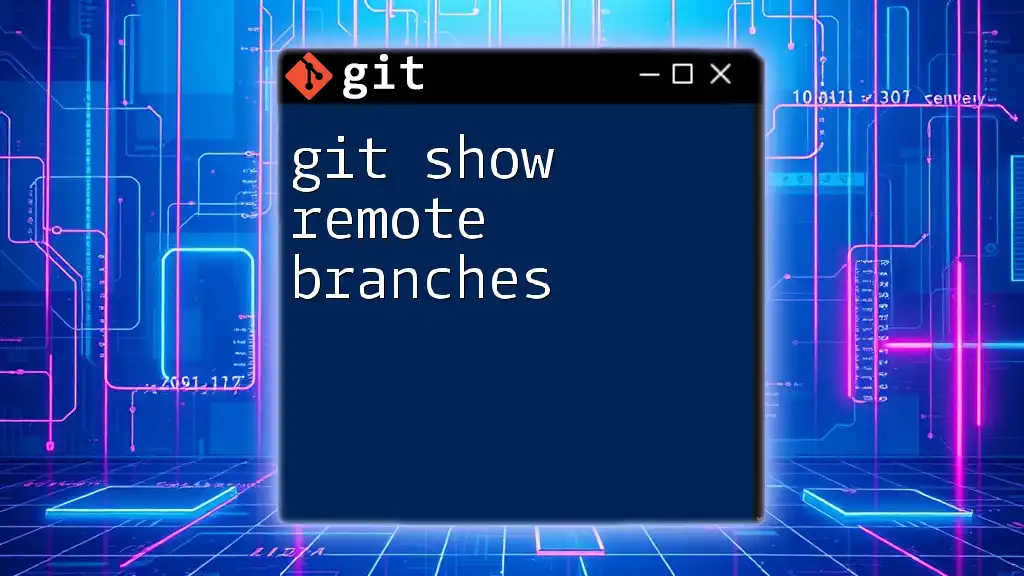
Common Issues and Troubleshooting
Error Messages While Switching
You might encounter errors like "branch not found." This typically indicates either a typing error in the branch name or that the branch does not exist on the remote. To solve this:
- Double-check the branch name using `git branch -r`.
- Ensure you have already executed `git fetch` to update your local references.
Working with Deleted Remote Branches
If a remote branch has been deleted, attempting to switch to it will produce an error. Regularly perform cleanup of your local branches to reflect the status of remote branches:
git fetch --prune
This command removes references to remote branches that no longer exist, ensuring a cleaner local branch list.
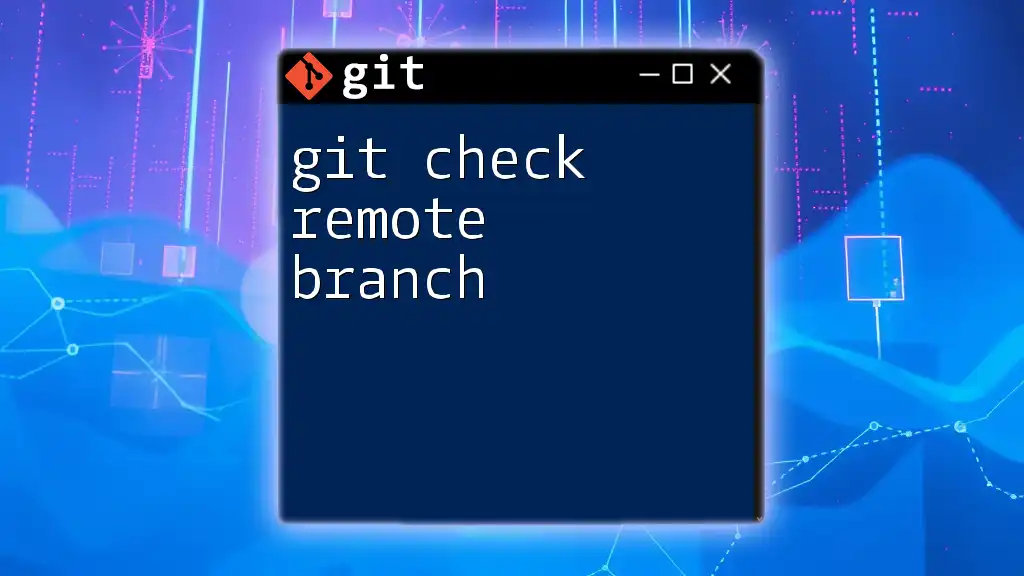
Best Practices for Working with Remote Branches
To manage remote branches effectively, consider these best practices:
- Regularly fetch updates from the remote to stay informed about changes made by collaborators.
- Use tracking branches strategically to minimize discrepancies between your local work and remote branches.
- Communicate with your team regarding branch naming conventions and updates to prevent confusion during collaboration.
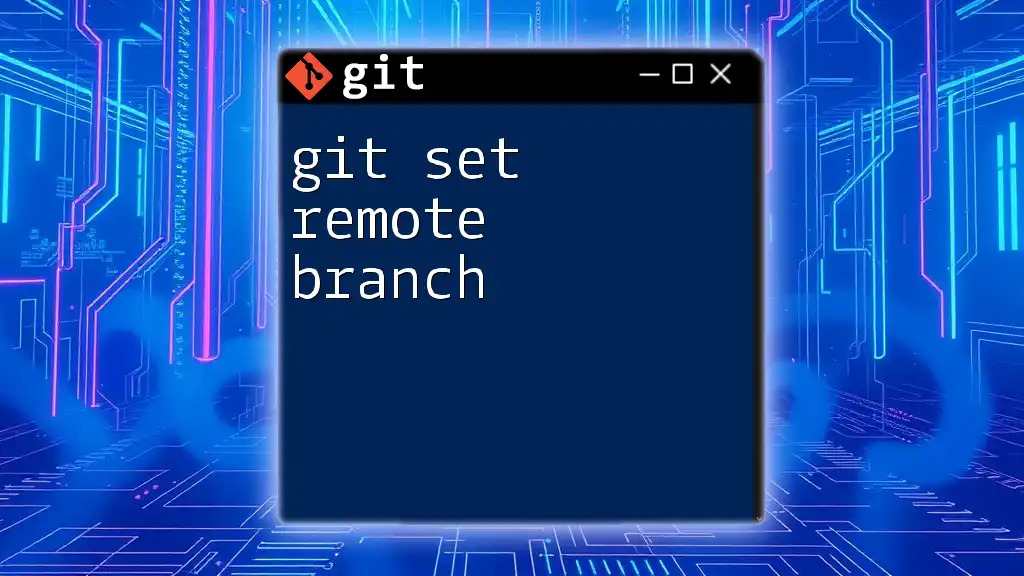
Conclusion
Switching to a remote branch using the `git switch` command is a fundamental aspect of collaborating efficiently in Git. By understanding how to fetch branches, list available options, and establish tracking, you can streamline your workflow and enhance productivity.
Practicing these commands will empower you to work more effectively with your team, ensuring that your project development remains organized and efficient. For more concise tutorials and tips on Git commands, be sure to follow our updates!
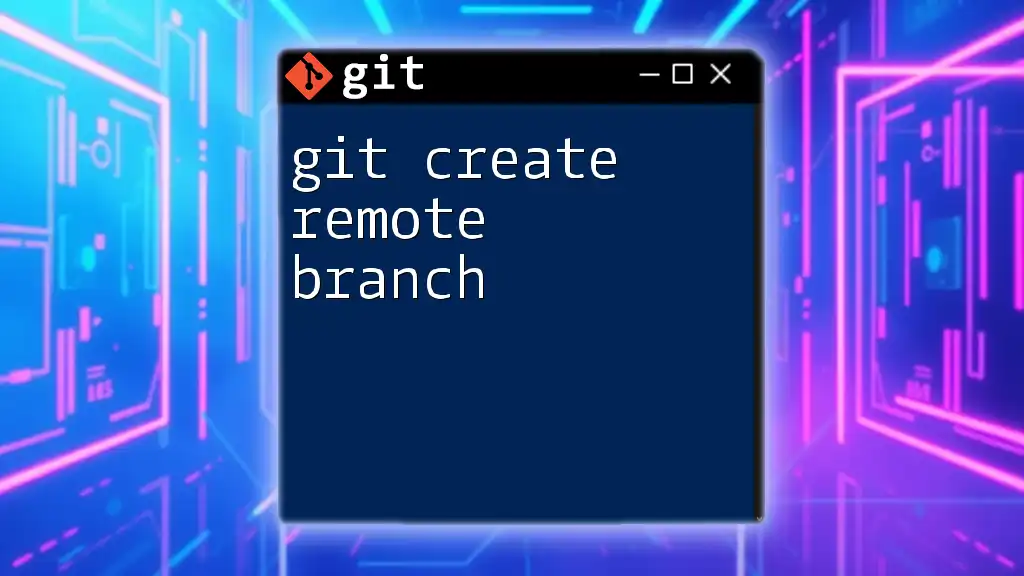
Additional Resources
- [Git Documentation](https://git-scm.com/doc)
- Recommended books: "Pro Git" by Scott Chacon and Ben Straub
- Explore our other informative articles on Git workflows and commands for deeper insights.