To pull changes from another branch into your current branch in Git, use the `git pull` command followed by the source branch name, as shown in the example below:
git pull origin feature-branch
Understanding Branches in Git
What is a Branch?
In Git, a branch is essentially a lightweight movable pointer to a commit. It allows developers to diverge from the main line of development and continue to work independently without affecting the main project. Branching facilitates a more organized workflow, ensuring that new features, bug fixes, or experiments can be conducted without disrupting the stability of the main codebase.
Why Use Multiple Branches?
Using multiple branches provides several advantages:
- Feature Development: Each feature can be developed in isolation to avoid interference with others.
- Collaboration: Team members can work on different tasks simultaneously, reducing the likelihood of major conflicts.
- Stability: The main branch (often called `main` or `master`) remains stable and deployable since developers work on separate branches until they're ready to merge back.
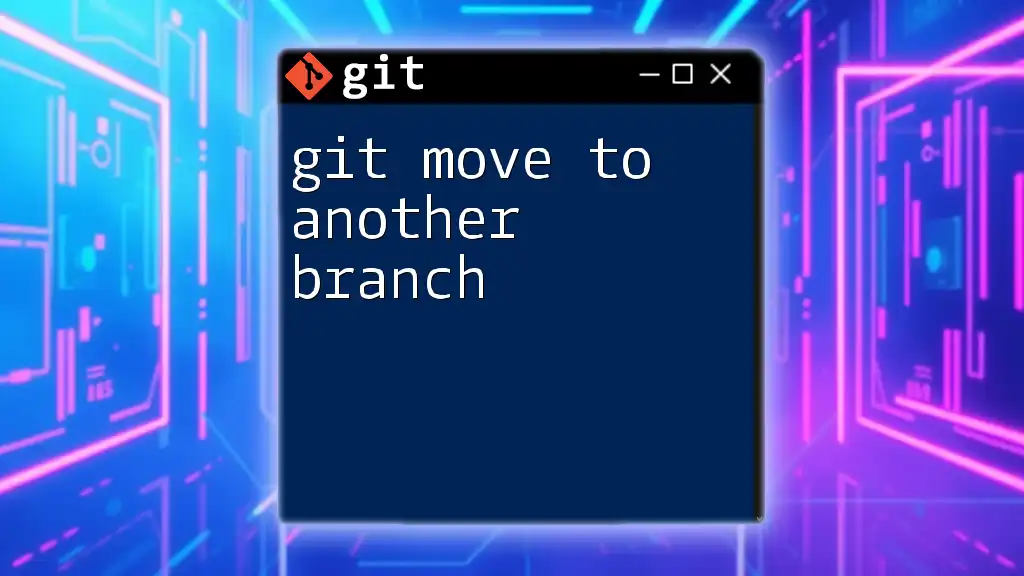
The `git pull` Command
What is `git pull`?
The `git pull` command is a vital Git function used to fetch and merge changes from a remote repository into your local branch. It combines two operations:
- Fetch: Downloads the latest changes from the remote repository, saving them in your local branches without merging.
- Merge: Automatically integrates the fetched changes into the current branch.
The difference between `git pull` and `git fetch` is crucial. While `git fetch` only downloads the changes, leaving the merge action to the user, `git pull` completes both actions in one command.
Example of a simple `git pull` command:
git pull origin main
This command pulls the latest changes from the `main` branch of the remote repository defined as `origin`.
When to Use `git pull`
You should use `git pull` when you want to sync your local branch with changes made by others. It’s best practice to pull frequently to avoid complicated merges later. Ensure that your current working branch is in a clean state before executing the command to prevent unwanted complications.
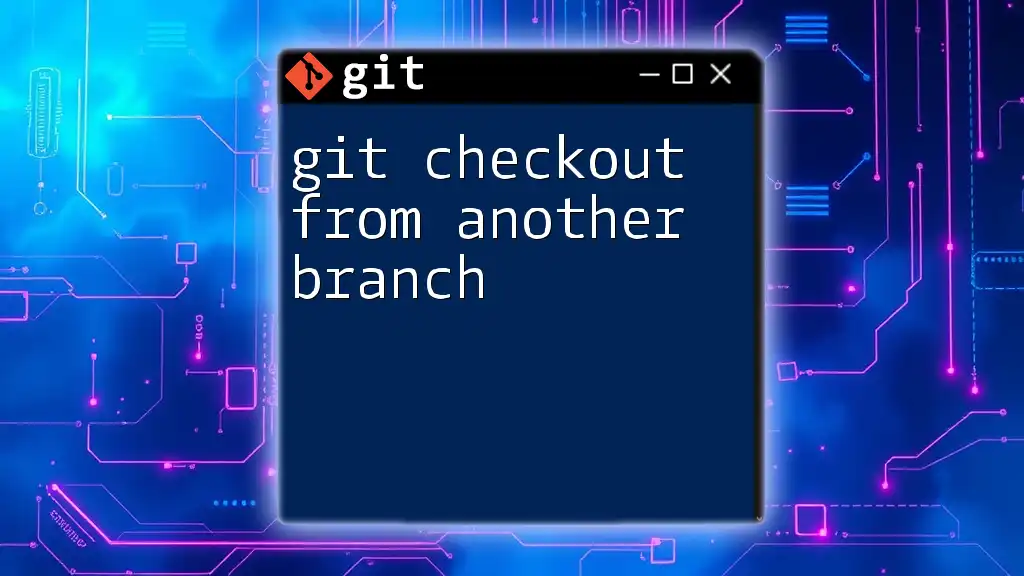
Pulling Changes from Another Branch
The Basic Syntax of `git pull`
The syntax for the `git pull` command includes specifying the remote name and the branch you want to pull from. The fundamental structure is as follows:
git pull <remote> <branch>
In this command:
- `<remote>` is typically `origin`, which refers to your remote repository.
- `<branch>` is the name of the branch from which you wish to pull changes.
How to Pull from a Specific Branch
Step 1: Checkout the Target Branch
Before pulling changes, you need to switch to the branch where you want to integrate those changes. You can do this using the `git checkout` command:
git checkout my-feature-branch
This command ensures that you're on the correct branch before executing the pull.
Step 2: Execute the Pull Command
Now, you can pull changes from the desired branch. For instance, if you want to pull updates from a branch called `another-branch`, you would execute:
git pull origin another-branch
This command will fetch changes from `another-branch` and merge them into `my-feature-branch`, allowing you to stay up to date with the latest changes.
Example Scenario
Consider a practical scenario where you're working on a `feature-branch`. You need the recent changes made in the `develop` branch. The process would look like this:
- First, check out your feature branch:
git checkout feature-branch
- Next, pull the latest changes from the develop branch:
git pull origin develop
This sequence will help align your feature branch with the latest work done in the develop branch, ensuring that you’re building upon the most recent code.
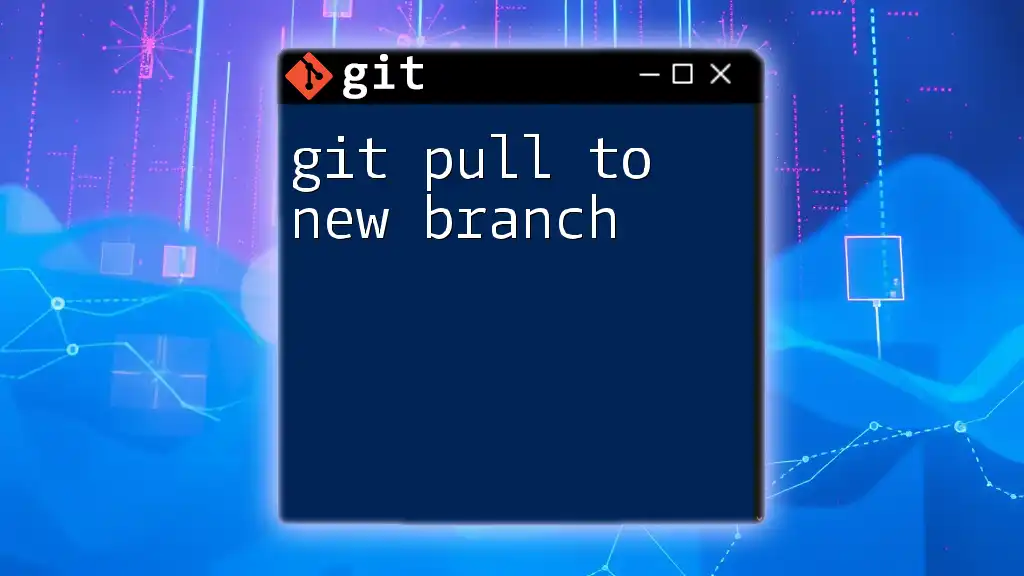
Handling Merge Conflicts
What Are Merge Conflicts?
A merge conflict occurs when Git cannot automatically resolve differences between two branches. This situation arises when changes have been made to the same line of a file or if a file has been deleted in one branch and modified in another.
How to Resolve Merge Conflicts
Resolving merge conflicts involves a few key steps:
-
Identify Conflicts: After attempting a pull that results in conflicts, run:
git status
This command will list all files with conflicts.
-
Manual Resolution: Open the conflicted files and look for conflict markers (`<<<<<<<`, `=======`, and `>>>>>>>`). Edit the file to finalize the changes that should be kept.
-
Finalize Your Changes: After resolving conflicts in all affected files, use:
git add . git commit -m "Resolved merge conflicts"
This commits the changes with a message indicating that conflicts were resolved.
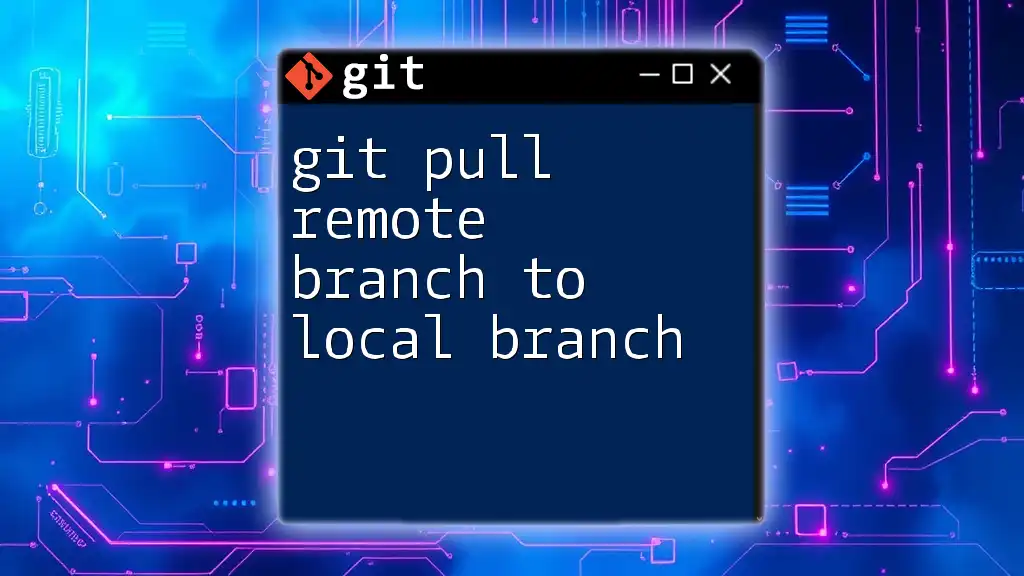
Best Practices for Pulling from Another Branch
Keep Your Branch Updated
Regularly pulling changes from main branches helps mitigate the risk of conflicts. Frequent updates ensure that your local branch remains in sync with the ongoing project developments. If you stay informed about changes being made by your teammates, you can address potential conflicts proactively.
Clear Communication in Teams
Coordinate with your team about the branches being worked on. Utilize tools such as pull requests, which provide a structured way to discuss and review changes before they are merged into other branches. Encouraging feedback through code reviews can also help maintain code quality and foster collaboration.
Use of Rebase
In some scenarios, you might prefer to use rebase instead of merging when pulling from another branch. Rebasing reorganizes your local commits to be applied on top of the latest changes from the target branch, leading to a linear project history.
For example, to pull changes using rebase, you would run:
git checkout my-feature-branch
git pull --rebase origin another-branch
This command rewrites the history to create a cleaner project log, but be cautious with rebasing if you’ve already shared your branch with others.
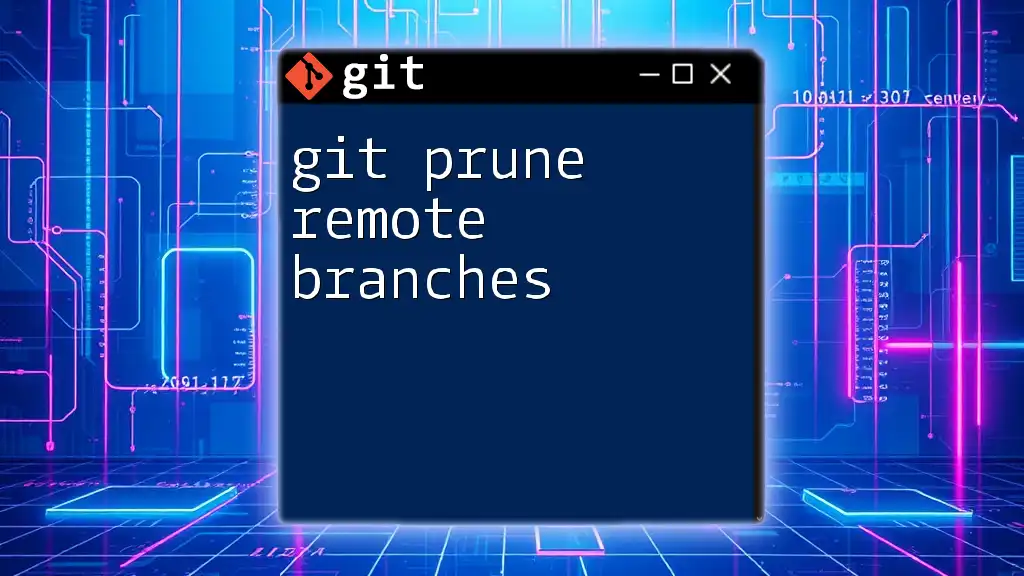
Conclusion
Understanding how to git pull from another branch is essential for effective teamwork and version control in software development. By following the practices outlined in this guide, you will improve your collaboration skills and maintain a smoother workflow. Keep learning and exploring other Git commands to enhance your proficiency and agility in managing your projects.
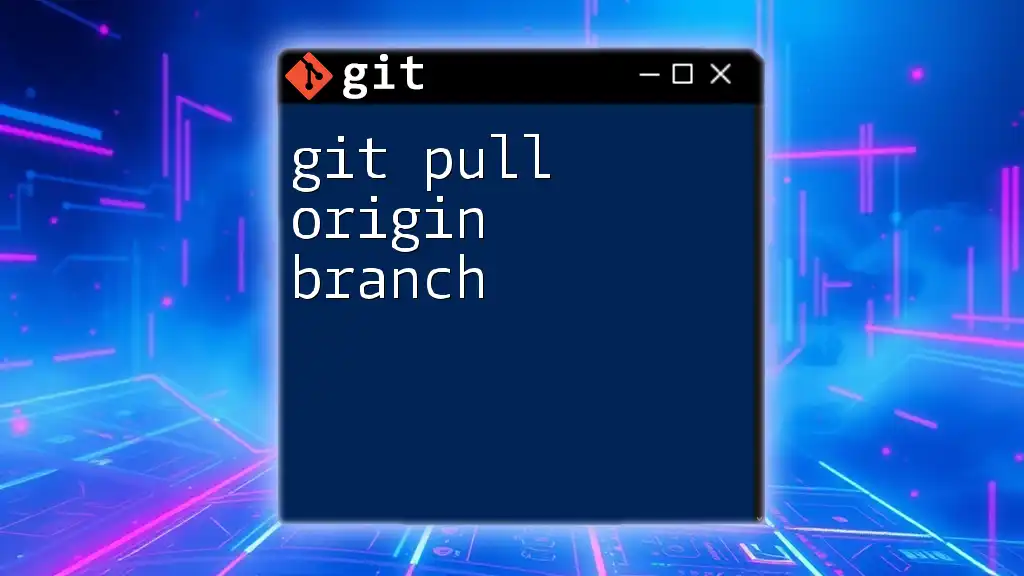
Additional Resources
For further exploration of Git, refer to the official Git documentation. There, you will find in-depth explanations and guides on a variety of commands and workflows. Additionally, many online tutorials and courses can provide valuable insights into mastering Git. Embracing these resources will bolster your development skills and enhance your capabilities in using Git for version control.
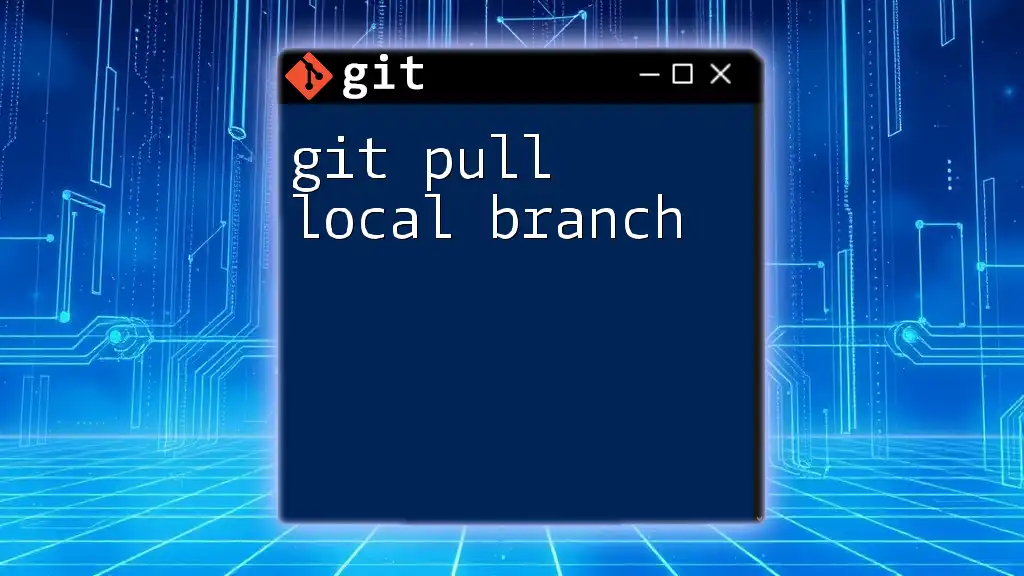
FAQs
What happens if I don’t specify a branch while pulling?
If you run `git pull` without specifying a branch, Git will pull from the default upstream branch for your current branch. It’s vital to ensure that your local branch is properly set up to track the correct remote branch to avoid unintended consequences.
Can I pull from two branches simultaneously?
No, Git does not allow you to pull from two branches at the same time. If you want changes from multiple branches, you need to pull each branch sequentially.
Do I need to commit my changes before pulling from another branch?
It is highly advisable to have a clean working directory before pulling from another branch. If you have uncommitted changes, Git may flag these changes when you attempt to pull, complicating the merge process. Always commit or stash your changes before performing a pull to maintain a smooth workflow.