To pull changes from a remote repository into a new local branch, first checkout a new branch and then use the `git pull` command with the specified remote and branch name.
git checkout -b new-branch-name
git pull origin remote-branch-name
Understanding Git Branching
What is a Git Branch?
A branch in Git is essentially a separate line of development, allowing multiple features to be worked on concurrently without affecting the main codebase. Branches enable developers to experiment with new ideas, fix bugs, or develop features in isolation. This practice is crucial in collaborative environments where multiple developers might be modifying the same files or functionality.
For instance, you might have:
- Feature Branches for developing new features.
- Bugfix Branches for addressing bugs or issues.
- Release Branches to prepare for a new deployment.
Why Use a New Branch for Pulling?
When pulling changes from a remote repository, creating a new branch provides several advantages:
- Safety: By operating in a new branch, you ensure that the stable code on the primary branch (e.g., `main` or `master`) is untouched. This is especially crucial for showcasing the latest working version of your application.
- Isolation: Pulling changes into a dedicated branch allows for testing new features or updates without impact. You can examine how new updates merge with your changes without affecting your main branch.
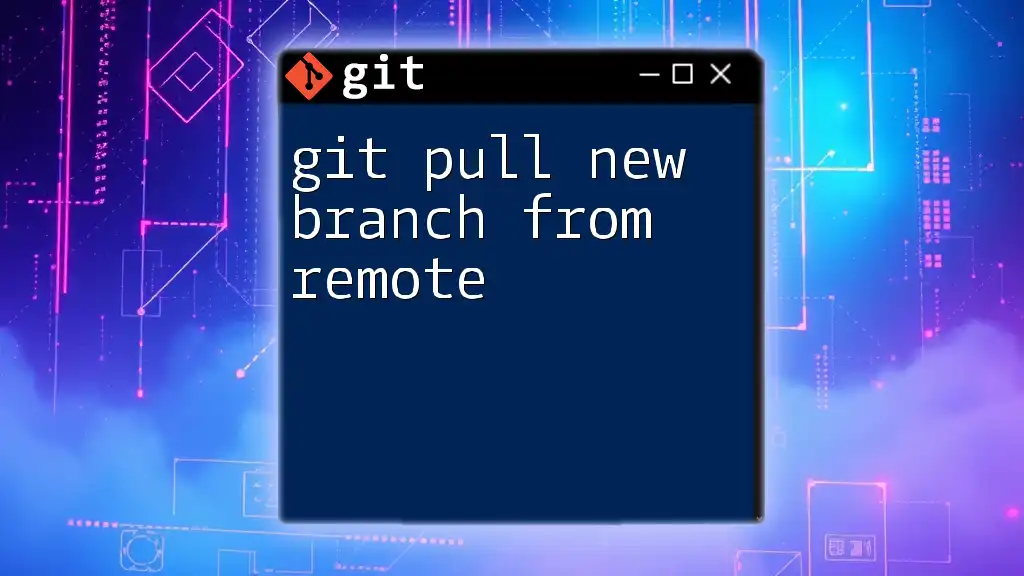
Preparing for a Pull Request
Creating a New Branch
Before pulling changes, you first need to create a new branch. This helps to organize your work effectively. To create and switch to a new branch, execute the following command:
git checkout -b new-feature-branch
Here, `checkout` is used to switch branches, and the `-b` option signifies that a new branch should be created. After running this command, you’ll be in the context of the newly created branch.
Fetching Updates from Remote
Before pulling in changes, it is crucial to fetch updates from the remote repository first. This ensures that you have the latest changes available. You can accomplish this with the following command:
git fetch origin
In this command, `origin` refers to the default remote repository. Fetching updates pulls in any changes from the remote without merging them into your current branch, allowing you to review updates before applying them.
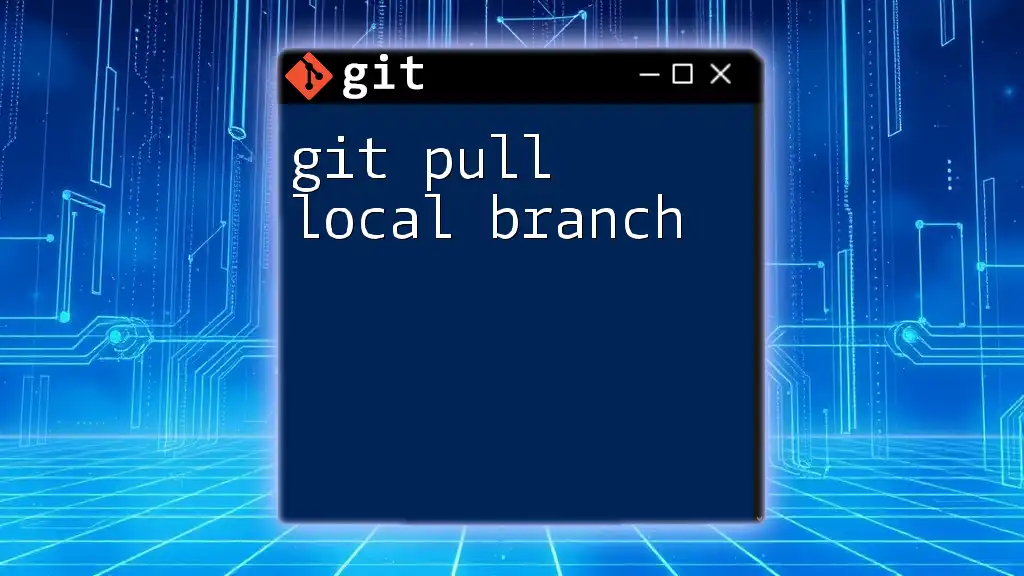
Pulling Changes into a New Branch
Using Git Pull Command
Once your new branch is created and you have fetched the updates, you can use the git pull command to integrate the changes into your new branch. The command generally looks like this:
git pull origin main
Here, `origin` represents your remote repository, and `main` refers to the branch from which you want to pull the updates. This action merges the specified branch into your current branch, which is your new feature branch.
Merging Changes
During a `git pull`, two actions take place: fetching updates and merging them. When changes from the remote branch are pulled in, Git will attempt to automatically merge these modifications. In cases where two sets of changes conflict—i.e., they affect the same lines in a file—Git will indicate a merge conflict that requires manual resolution.
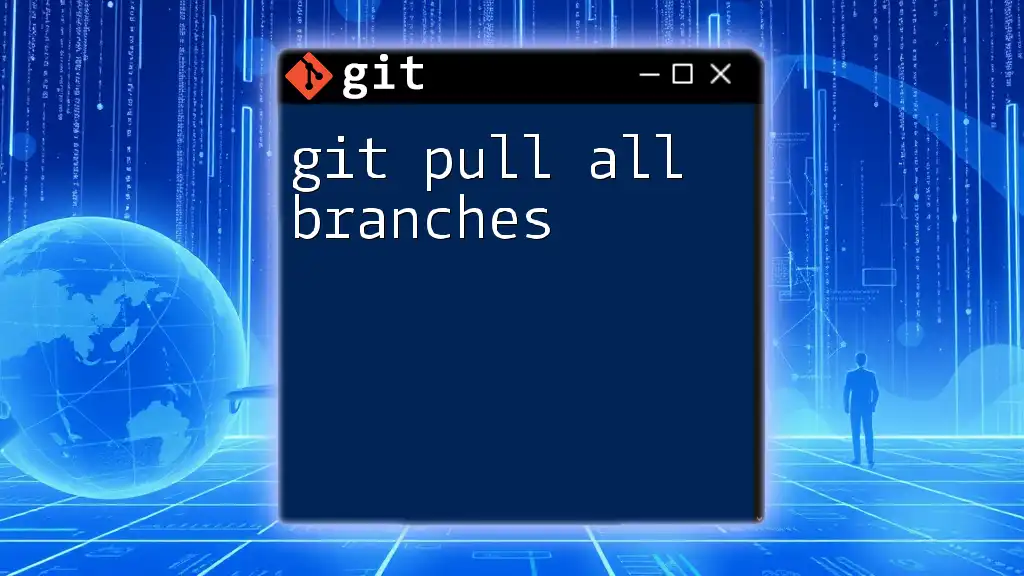
Resolving Merge Conflicts
Understanding Merge Conflicts
Merge conflicts occur when changes in your branch and the branch you are pulling from cannot be merged automatically. This situation typically arises when several developers modify the same part of a file. Recognizing these conflicts is crucial, as they can prevent your pull from completing successfully.
Steps to Resolve Merge Conflicts
To resolve merge conflicts, follow these steps:
-
Identify Conflicts: After a pull attempt results in conflicts, you can run the following command to see which files are affected:
git status
-
Edit Files: Open the files in conflict with your text editor. Git will insert conflict markers (e.g., `<<<<<<< HEAD`, `=======`, `>>>>>>> branch-name`) to show conflicting changes. Review the changes and edit decisions you need to make.
-
Mark as Resolved: After editing conflicts out of the file, you need to mark those files as resolved. First, stage the changes by running:
git add resolved-file
Following resolution, finalize the merge with a commit that documents the resolution.
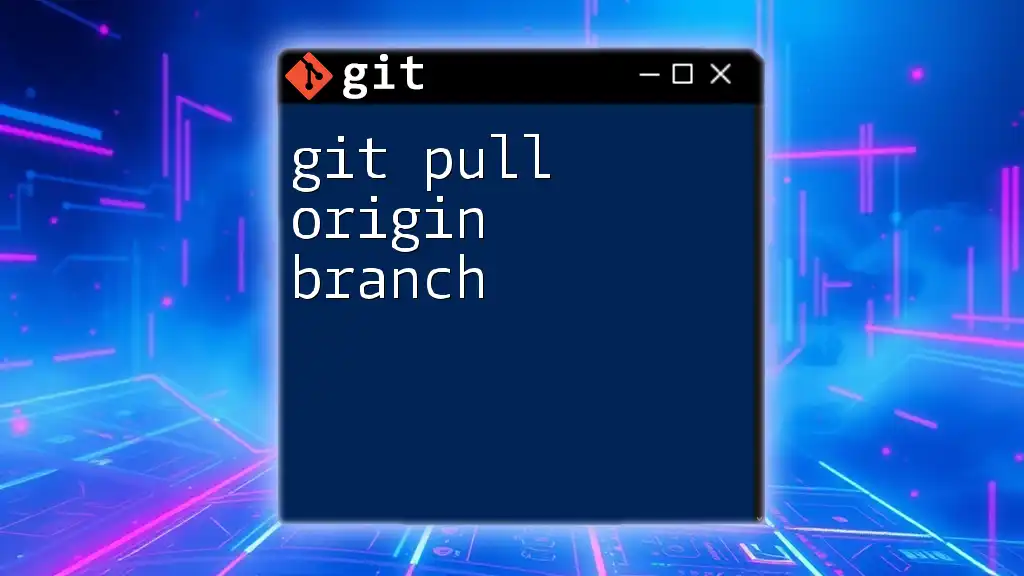
Best Practices for Pulling into a New Branch
Keeping Your Branch Updated
Make a habit of regularly pulling changes into your branch from the main or development branch. Staying updated helps prevent larger conflicts when you're ready to merge your feature branch back into the main code. When pulling updates, consider using rebasing at times, as it can help keep a cleaner project history.
Naming Conventions for Branches
Using descriptive branch names is important for clarity. It helps the entire team understand what is being worked on and organizes tasks better. Here are a few examples:
- `feature/new-login`
- `bugfix/fix-header-issue`
- `release/v1.2.0`
Pull Requests and Code Reviews
Once you've completed your work on the new branch, submit a pull request. This facilitates code reviews, allowing team members to examine the changes before they are integrated into the main branch. Code reviews are vital for maintaining code quality and fostering knowledge sharing among developers.
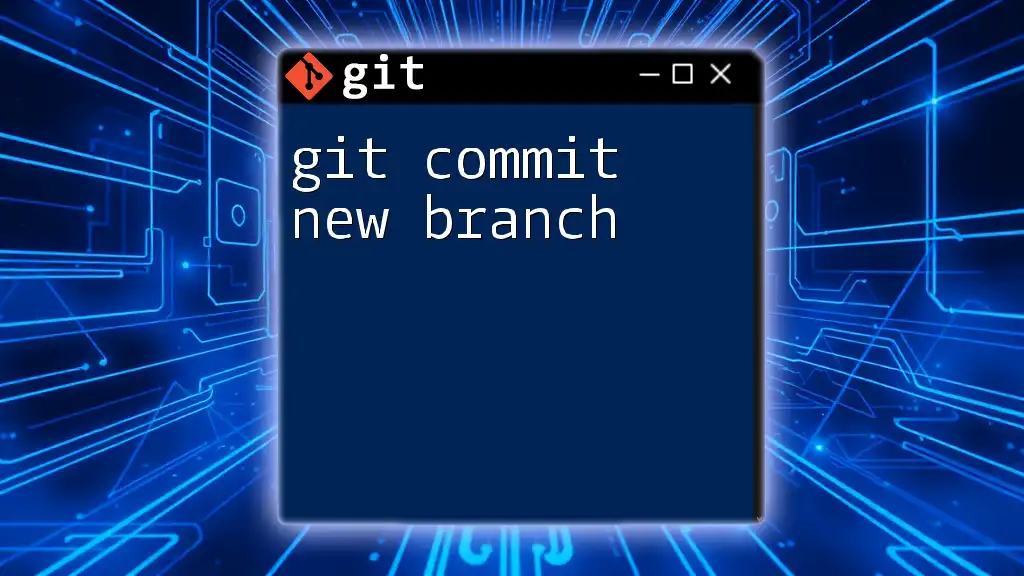
Conclusion
Pulling changes into a new branch is an organized approach to integrating updates while safeguarding the stability of the main codebase. By understanding the fundamentals of branching, pulling, and resolving conflicts, you ensure a smoother collaboration process and maintain high code quality. Don't forget to practice these commands regularly to aid your learning curve in Git.
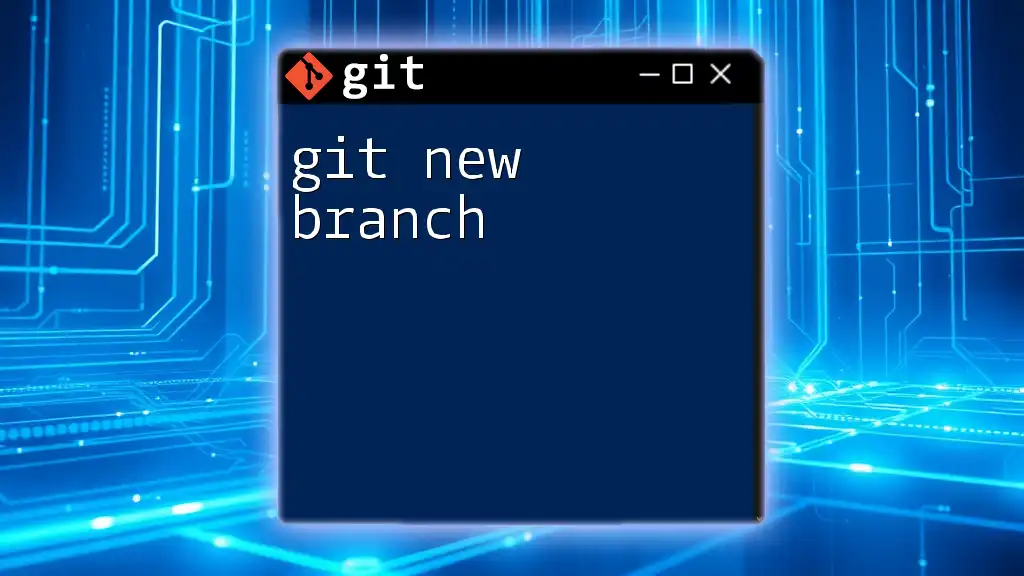
Additional Resources
To deepen your understanding of Git commands and best practices, consult various cheat sheets and recommended articles. Additionally, the official Git documentation is an excellent resource for mastering complex commands and functionalities.