To pull all branches from a remote repository in Git, you can use the following command to fetch updates and set up tracking branches for all of them:
git fetch --all && git pull --all
Understanding Git Branches
What are Git Branches?
In Git, a branch represents an independent line of development in your project. It allows multiple team members to work on features, fixes, or experiments without interfering with the main codebase. Branching is essential for managing different versions of your project and facilitates organized collaboration.
Default Branches vs. Feature Branches
Git repositories typically have default branches: `master` or `main`—these usually contain stable code ready for production. On the other hand, feature branches are created to develop new features or perform bug fixes. They allow developers to isolate their changes until they’re ready to be merged back into the main branch, reducing the risk of introducing bugs into the primary codebase.
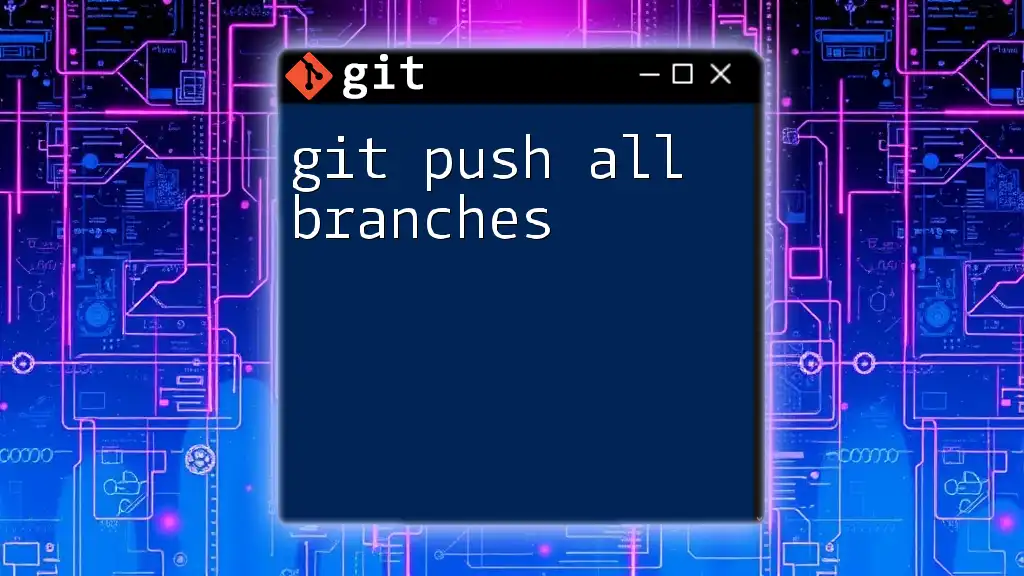
The `git pull` Command
What Does `git pull` Do?
The `git pull` command is a fundamental part of using Git that updates your local repository with changes from a remote repository. The command essentially combines two commands: `git fetch` and `git merge`. First, it retrieves new data from the remote repository, and then, it merges those changes into your current branch.
Basic Usage of `git pull`
The syntax of the `git pull` command is as follows:
git pull [options] [repository] [refspec]
For example, to pull updates from the main branch of the origin repository, you would use:
git pull origin main
This command automatically fetches and merges the remote changes into your current branch, keeping everything up to date.
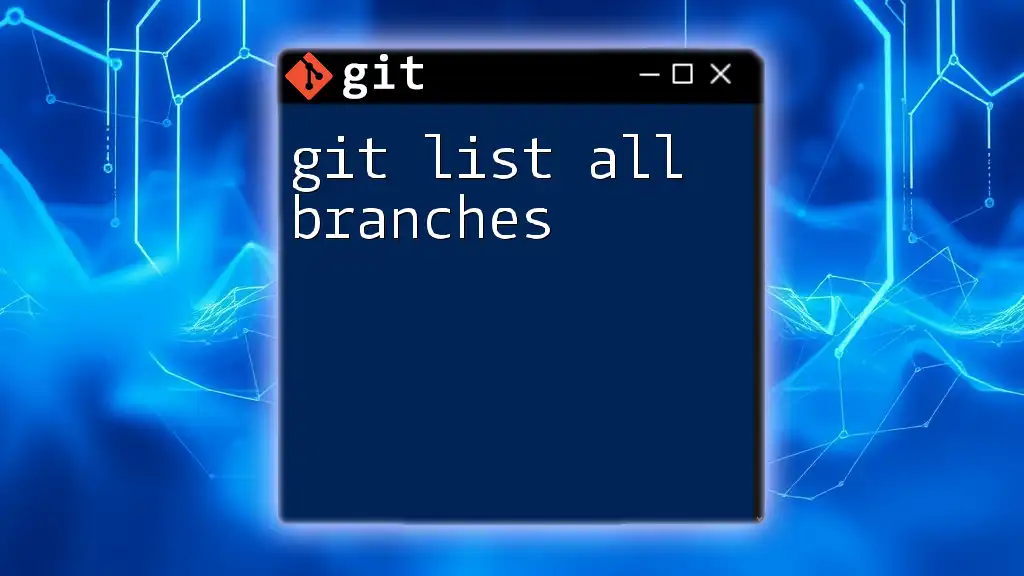
Pulling All Branches: Concept and Methods
Why Pull All Branches?
In a collaborative development environment, it’s crucial to keep all your local branches up-to-date with their corresponding remote branches. This practice minimizes the risk of merge conflicts when working on different branches and ensures that everyone on the team has the latest updates, fostering smoother collaboration.
Method 1: Using `git fetch` followed by `git merge`
Steps to Pull All Branches
To pull all branches, you can start with the `git fetch` command, which retrieves all changes from the remote repository without merging them immediately. This allows you to see what’s new in all branches.
You can then switch to each branch and merge the changes individually. Here’s how:
git fetch --all
This command fetches changes from all branches. Next, check out each branch and merge changes one at a time. For example:
git checkout branch_name
git merge origin/branch_name
Example Codes
In a scenario where you have multiple branches to update, you would do this for each branch that you have checked out locally to ensure you are up to date with the remote copies.
Method 2: Using Git Scripts to Automate the Process
Writing a Simple Shell Script
To streamline the process, you can write a script that automates pulling changes for all branches. Here’s a helpful example of a shell script:
#!/bin/bash
git fetch --all
for branch in $(git branch -r | grep -v '\->'); do
git checkout ${branch#origin/}
git pull
done
This script starts by fetching all updates and then iterates over each remote branch. By switching to each branch and pulling the updates automatically, you save time and reduce manual errors.
Explanation of How This Script Works
- `git fetch --all`: Fetch all changes from all remote branches.
- `git branch -r`: Lists all remote branches.
- `grep -v '\->'`: Filters out symbolic references (such as `HEAD`).
- `git checkout ${branch#origin/}`: Switches to the local version of the branch stripped of the `origin/` prefix.
- `git pull`: Updates the local branch with the latest changes from the remote branch.
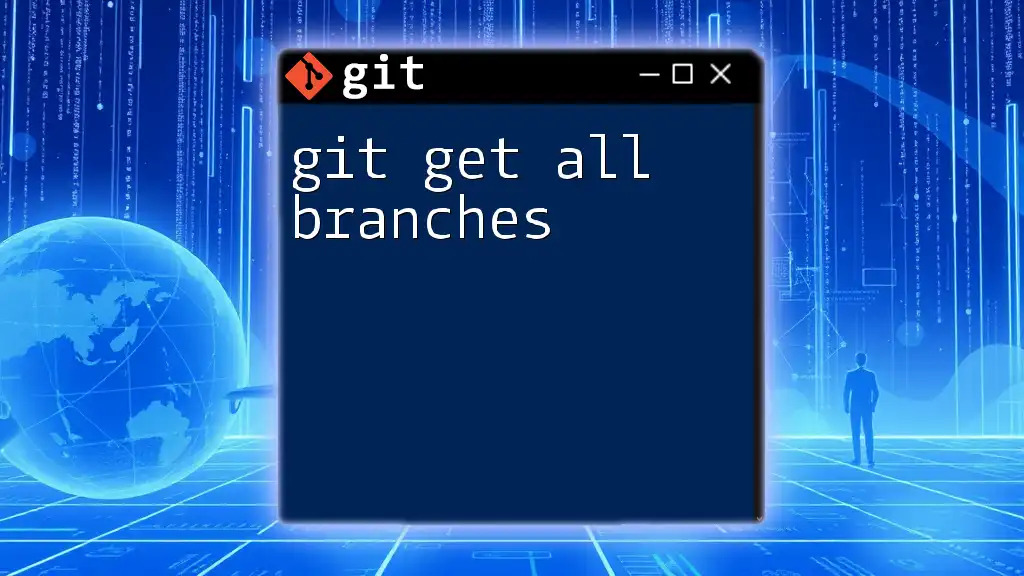
Best Practices for Pulling All Branches
Regular Updates
Regularly pulling all branches can prevent merge conflicts from building up over time. A habit of frequent updates helps you stay in sync with your team, ensuring that you always work with the latest code.
Collaboration and Communication
Effective collaboration involves strong communication among team members about branch changes. Make it a practice to inform others when you push significant changes so they can pull updates accordingly.
Cleanup and Maintenance
Keeping your local repository tidy is essential for productive development. Delete any local branches that are no longer needed to avoid confusion:
git branch -d branch_name
This command deletes a local branch that has been merged. Always make sure you're not on the branch you want to delete.
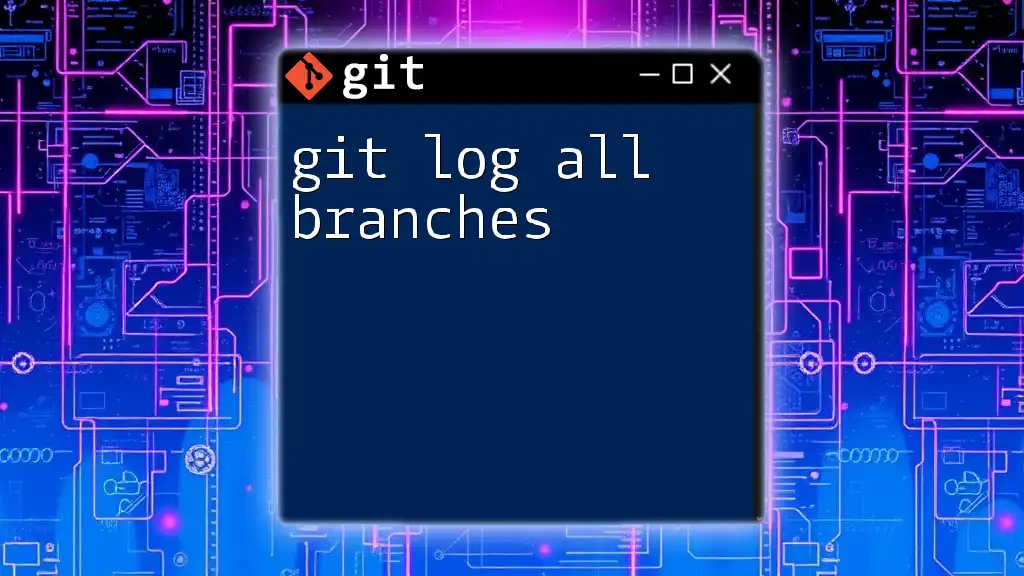
Troubleshooting Common Issues
Merge Conflicts
Merge conflicts can occur when multiple developers change the same part of the codebase. When you encounter a conflict during a pull, Git will inform you, and you’ll need to resolve it before you can complete the merge. To resolve conflicts, manually merge the changes in your code editor and then stage them for a commit.
Handling Detached HEAD State
A detached HEAD state occurs when you check out a specific commit that is not a branch, meaning your changes won't be saved to any branch unless you create one. To recover, you can create a new branch from your current state:
git checkout -b new_branch_name
This command establishes a new branch based on your current changes, thereby saving your progress.
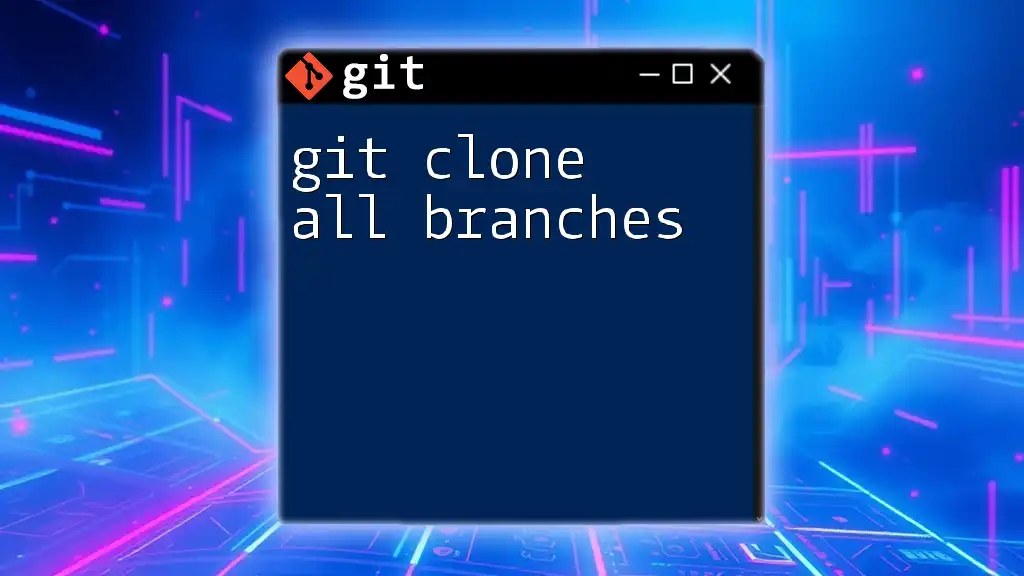
Conclusion
In summary, mastering the `git pull all branches` command is essential for effective collaboration in a Git-managed project. Regularly updating all branches strengthens teamwork, reduces the potential for conflicts, and maintains the integrity of your project. Embrace these practices and scripts to streamline your workflow, enhance collaboration, and stay efficient in your Git operations.
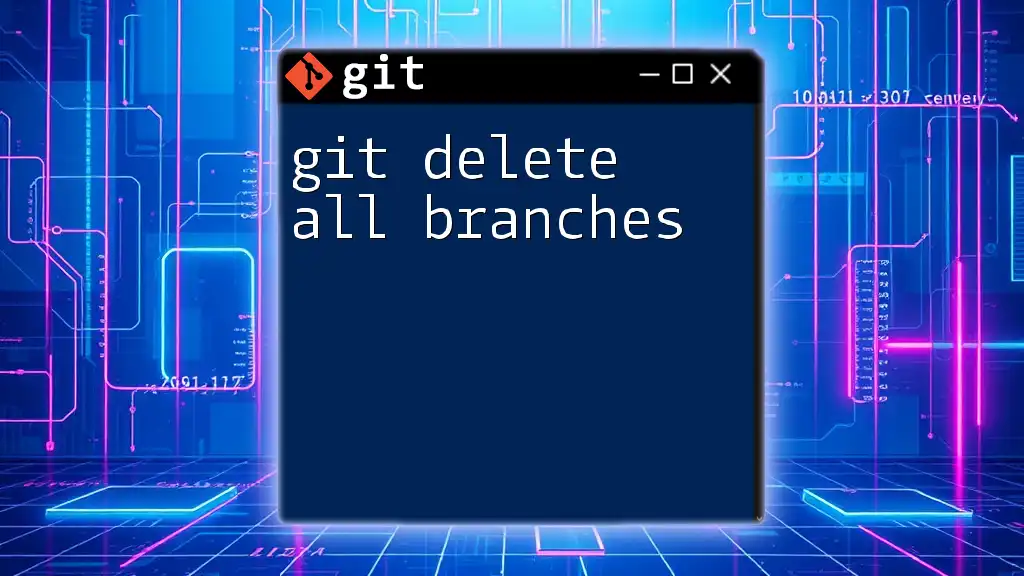
Additional Resources
For more information, consider reading the [Git documentation](https://git-scm.com/doc) or enrolling in online courses that offer practical exercises and deeper insights into Git commands and best practices.