To update your current branch with the latest changes from the remote repository, you can use the following command:
git pull origin <branch-name>
Replace `<branch-name>` with the name of the branch you want to update.
Understanding Git Branches
What is a Git Branch?
A branch in Git is essentially a pointer to a specific commit in your project history. Branching allows developers to diverge from the main codebase to work autonomously on features, bug fixes, or experiments without affecting the main code. This results in a cleaner and more organized development process.
As an analogy, think of branches as different paths on a hiking trail. While one group goes left (the main branch), another group can explore right (the feature branch) without obstructing each other's journey.
The Role of Master/Main and Feature Branches
In Git, the default branch is often named master or main. This branch typically represents the source of truth in a project. On the other hand, feature branches are created to develop new features or fix bugs.
Maintaining a clear distinction between these branches helps to ensure a smoother development workflow and also facilitates code reviews and integrations.
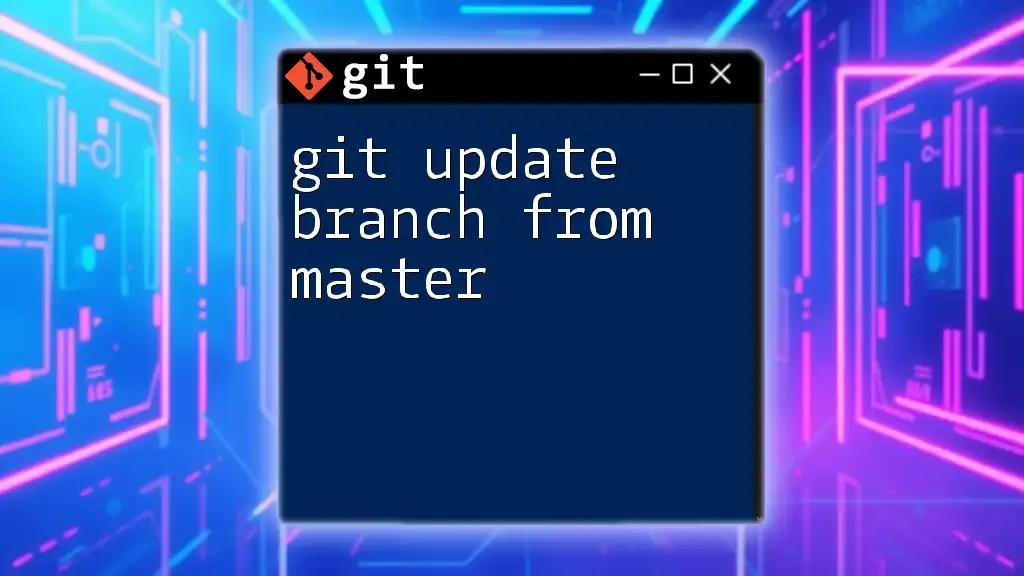
Why You Need to Update Your Branch
Keeping Up with Changes
When collaborating on a project with multiple team members, the code can change rapidly. Each time someone else pushes updates to the repository, it becomes essential to keep your branch current.
If you neglect to update your branch, you may end up facing merge conflicts when you try to integrate your changes. This can lead to wasted time and frustration.
Synching with the Remote Repository
Updating your branch is crucial for syncing with remote branches (the versions of your branch in an online repository). Regularly syncing helps you stay informed about what changes have been made by other team members, keeping your codebase consistent and up to date.
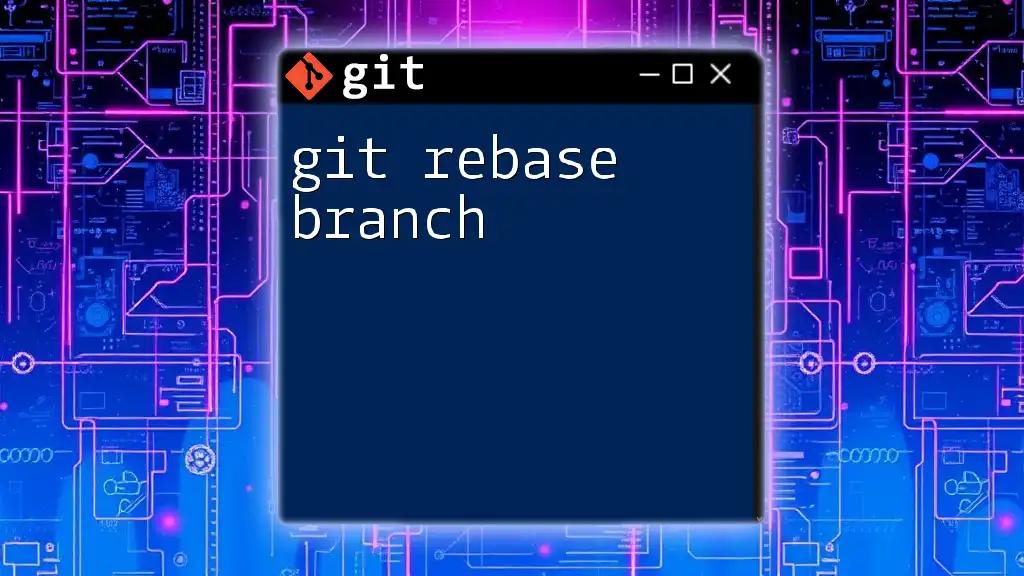
The Command: `git update-branch`
Introduction to the Command
The `git update-branch` command is specifically designed to update the tip of your current branch to match the latest changes available on a specified remote branch, usually referred to as “upstream”.
Syntax and Usage
The basic syntax for the command is as follows:
git update-branch <branch-name>
Here, `<branch-name>` refers to the name of the branch you wish to update.
Practical Example
Imagine a developer is working on a feature branch called `feature-branch` while the main branch `main` has seen several updates. To pull those changes into their feature branch, they would execute:
git update-branch feature-branch origin/main
This command tells Git to fetch the latest changes from `main` and apply them to `feature-branch`, thereby keeping the branch current.
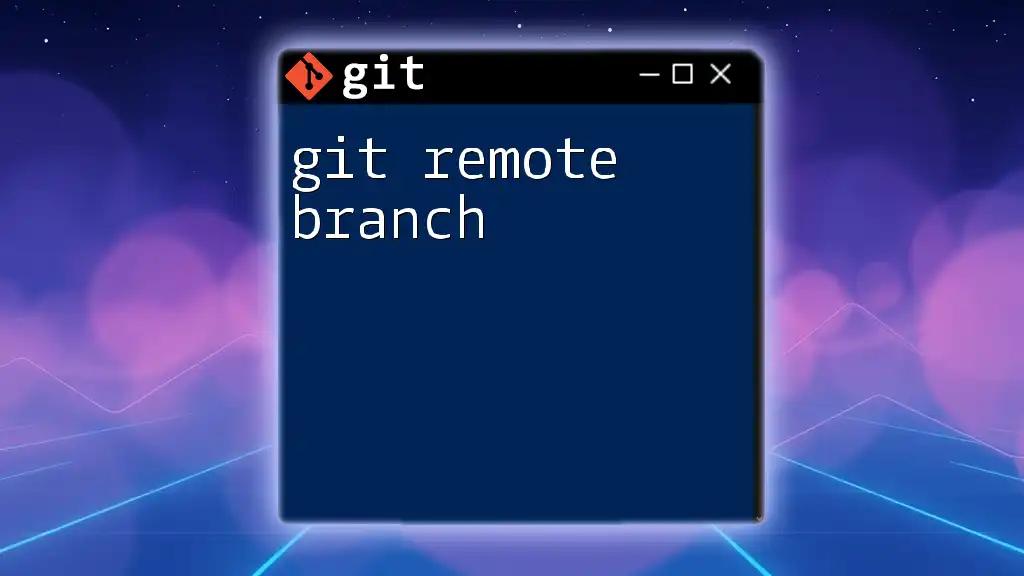
Alternative Ways to Update a Branch
Using `git pull`
While `git update-branch` is effective, many developers prefer using `git pull`, which performs both fetching and merging in a single command. This means you can quickly get the latest updates that others have pushed.
Here’s how you could use it:
git pull origin main
This command fetches the latest changes from the `main` branch in your remote repository and tries to merge them into your current branch.
Using `git fetch` and `git merge`
Another approach is to use `git fetch` followed by `git merge`. This gives you more control over the process, allowing you to review changes before merging them.
- Fetching changes:
git fetch origin
This command retrieves changes from the remote repository but does not merge them immediately.
- Merging changes:
git merge origin/main
This merges the fetched changes into your current branch.
Using this two-step process is particularly useful if you want to check what changes you are integrating without immediately applying them.
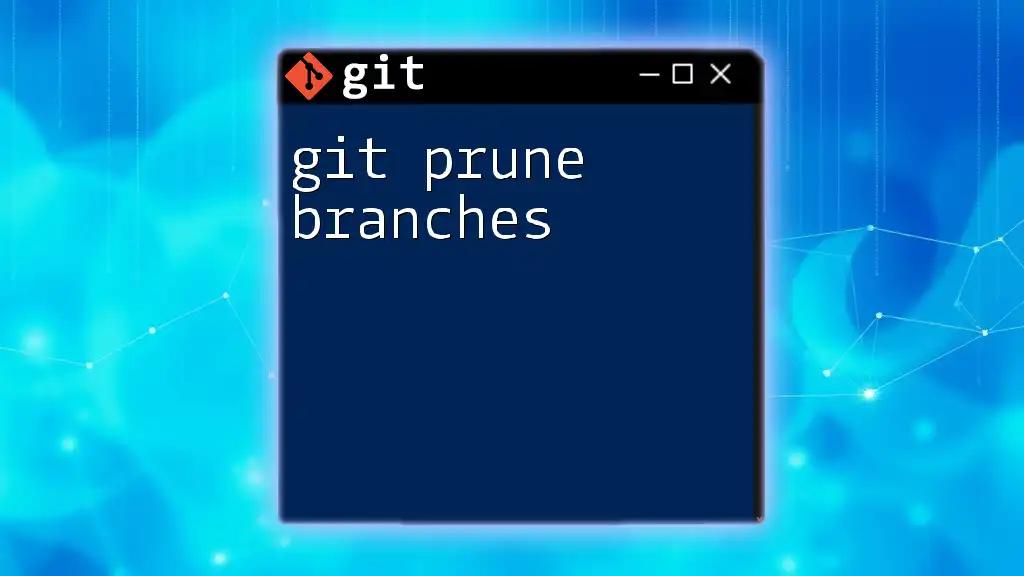
Resolving Merge Conflicts
Understanding Merge Conflicts
Merge conflicts occur when two branches have changes to the same parts of the code, and Git can't automatically reconcile them. The most common scenarios include changes made in both the main branch and the feature branch.
When conflicts arise, you will typically see messages in the terminal indicating which files are in conflict. Understanding how to identify these conflicts is key to maintaining an efficient workflow.
How to Resolve Merge Conflicts
When you encounter merge conflicts, Git marks the conflicting sections in the affected files. You’ll need to open these files, look at the conflicting code, and manually decide how to resolve the disagreement. Here’s a general workflow:
- Identify files with merge conflicts using `git status`.
- Open the conflicting files to resolve the issues manually.
- After resolving the issues, mark the conflicts as resolved:
git add <file-name>
- Finally, complete the merge process:
git commit
Utilizing GUI tools can also facilitate this process. Many Git clients come with integrated diff views which make it easier to visualize the changes and resolve conflicts intuitively.
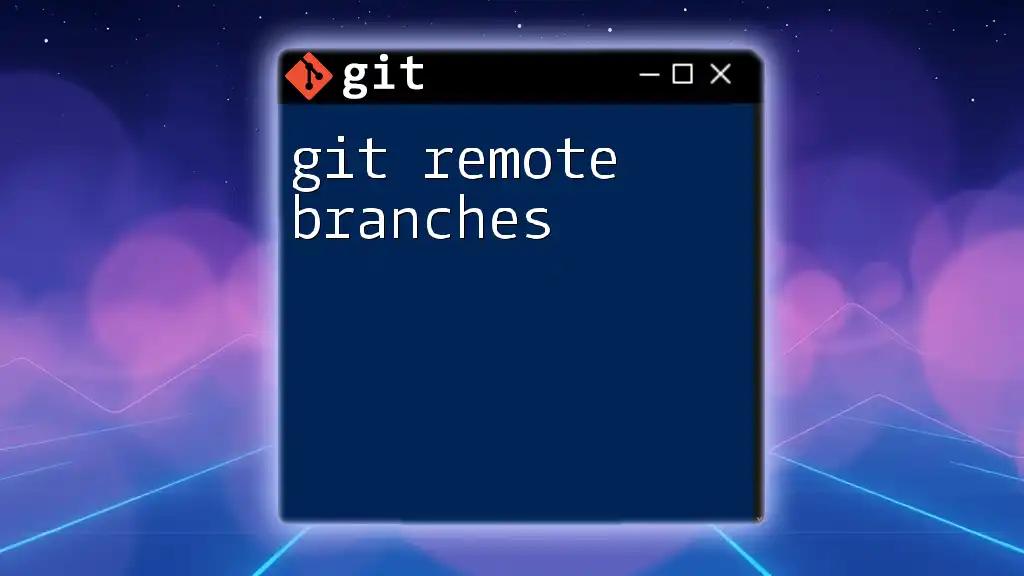
Best Practices for Keeping Your Branch Updated
Regular Updates
To maintain a seamless workflow, frequently update your branches. This can prevent large and complex merge conflicts and keeps everyone on the same page. Communication between team members is vital—ensure everyone is aware of ongoing updates.
Leveraging Rebase
Rebasing is another powerful technique that allows you to incorporate upstream changes by moving your feature branch to the new base. You can do this with the following command:
git rebase main
This command will replay your changes in `feature-branch` on top of the latest changes in `main`, creating a clean project history.
Utilizing Git Hooks for Automation
Git hooks can automate certain tasks before and after commits. By setting up a pre-commit or post-merge hook, you can ensure that your branch remains updated automatically whenever you make a commit or merge changes.
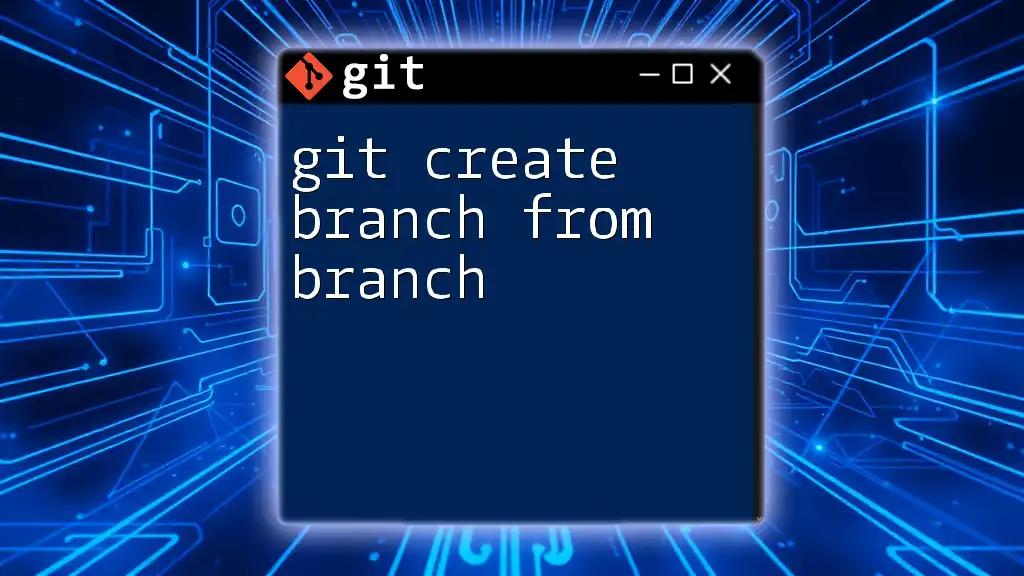
Conclusion
The ability to effectively update your branch in Git is essential for maintaining a consistent and efficient workflow in collaborative projects. Keeping branches up to date helps mitigate merge conflicts and ensures that everyone is working with the latest version of the code. By following the best practices outlined in this guide, you can make your Git experience smoother and more productive.
Stay informed, keep experimenting with commands, and don't hesitate to reach out for further learning resources to enhance your Git skills!