To create a branch locally and then push it to the remote repository, you can use the following commands:
git checkout -b new-branch-name && git push -u origin new-branch-name
Understanding Git Branches
What is a Git Branch?
A branch in Git represents an independent line of development in your project. It allows you to isolate changes, experiment with new features, and collaborate with others without affecting the main codebase.
Why Use Branches?
Branching is a powerful feature of Git for managing your project effectively. It enables multiple developers to work on different features without stepping on each other's toes. Here are a few scenarios where branching shines:
- Feature Development: Develop new features in isolation so that they can be thoroughly tested before integration.
- Bug Fixing: Address bugs while ensuring that the main branch is stable and deployable.
- Experimentation: Try out new ideas or make significant changes without fear of breaking existing functionality.
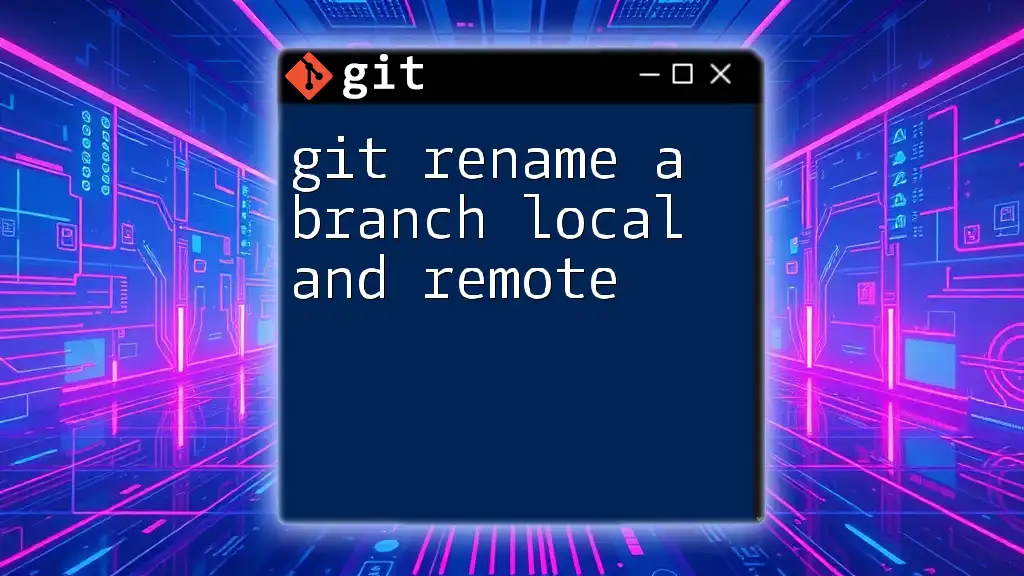
Creating a Local Branch
Step-by-Step Guide to Creating a Local Branch
Before creating a local branch, ensure you have a cloned repository to work with. You can start with the following commands:
git clone <repository-url>
cd <repository-name>
Once inside your project directory, it’s a good practice to check your current branch to understand your starting point:
git branch
Creating a New Local Branch
To create a new branch, you can use the following command:
git branch <branch-name>
For example, to create a branch for developing a new feature:
git branch feature/new-feature
Switching to the New Branch
Once the branch is created, switch to it using the checkout command:
git checkout feature/new-feature
This command changes your working directory to the new branch, allowing you to start making changes immediately.
Combining Creating and Switching
To streamline your workflow, you can combine the creation and checkout steps using:
git checkout -b <branch-name>
This command creates the branch and switches to it in a single step, as shown below:
git checkout -b feature/new-feature
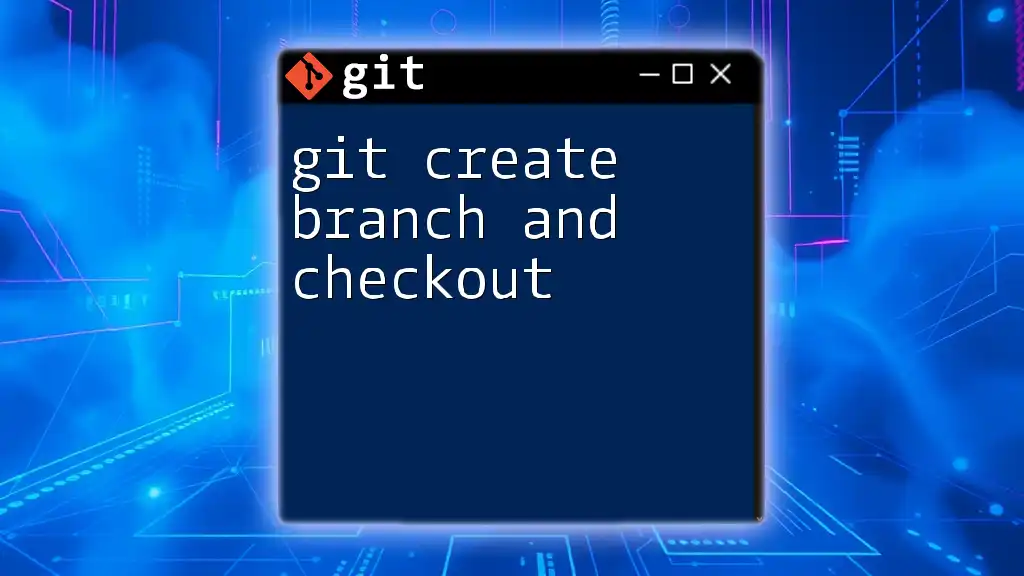
Working with Local Branches
Listing Local Branches
To view all local branches in your repository, use the following command:
git branch
This will display a list of branches, highlighting the branch you are currently on.
Deleting a Local Branch
If a branch is no longer needed, deleting it is straightforward to avoid clutter. Use the following command:
git branch -d <branch-name>
For example, to delete the "feature/new-feature" branch:
git branch -d feature/new-feature
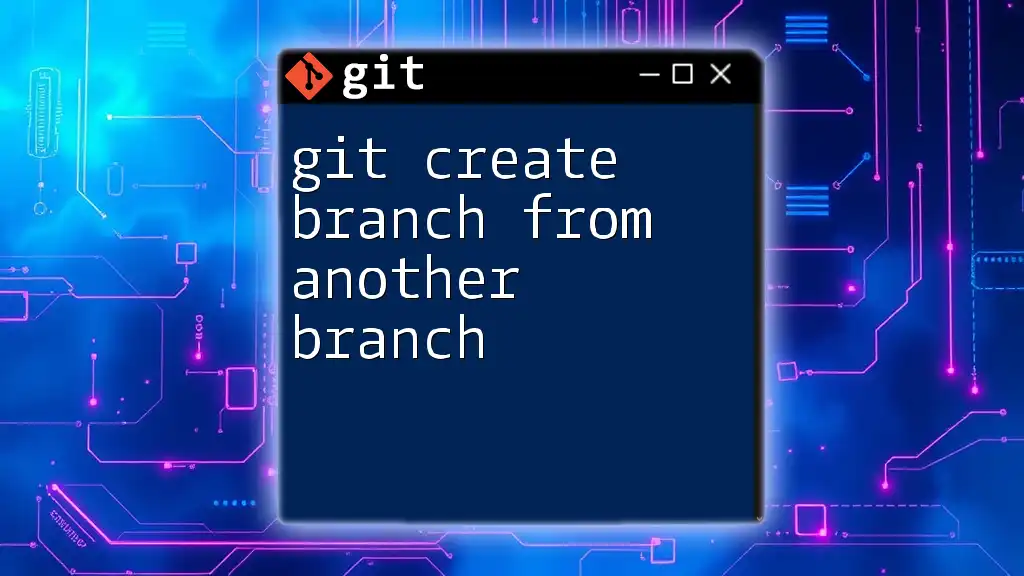
Creating a Remote Branch
Understanding Remote Repositories
Remote repositories are hosted versions of your project that enable collaboration. They allow multiple developers to share changes conveniently.
How to Push a Local Branch to Remote
Step-by-Step Guide to Pushing a Branch
Before pushing a local branch to the remote repository, ensure you are on the desired local branch:
git checkout feature/new-feature
To push the branch to a remote, use:
git push <remote-name> <branch-name>
For example, if your remote is named `origin` and your branch is `feature/new-feature`, execute:
git push origin feature/new-feature
Setting Upstream Branch
Setting upstream branches is a best practice that simplifies future pushes and pulls. Use the following command to set the upstream for the new branch:
git push -u <remote-name> <branch-name>
For example:
git push -u origin feature/new-feature
This command associates your local branch with the remote branch, allowing you to use just `git push` in the future.
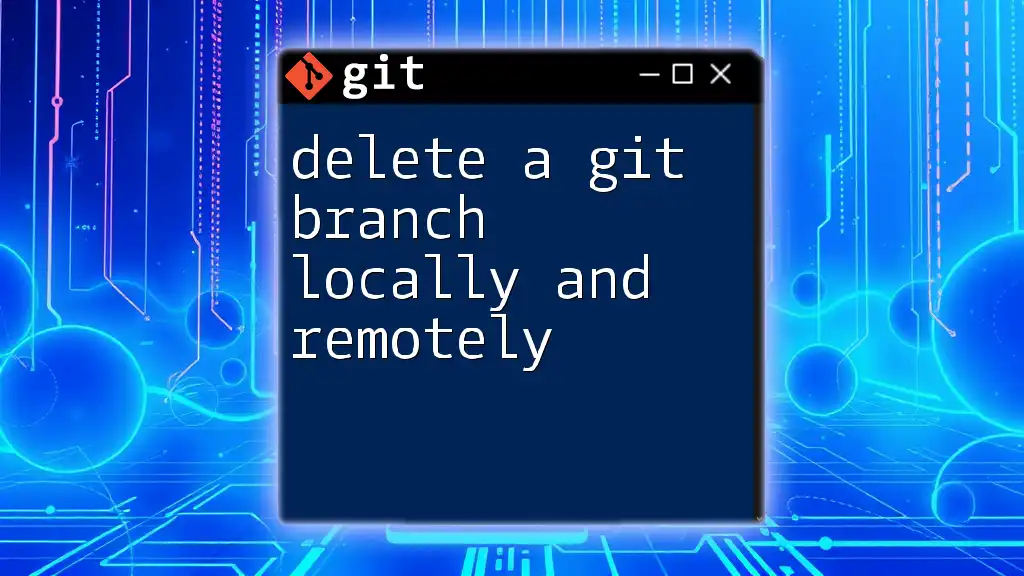
Managing Remote Branches
Listing Remote Branches
To list all branches in the remote repository, use:
git branch -r
This command provides an overview of which branches are available remotely.
Deleting a Remote Branch
If a remote branch is no longer needed, you can delete it using:
git push <remote-name> --delete <branch-name>
For example, to delete the remote branch `feature/new-feature`:
git push origin --delete feature/new-feature
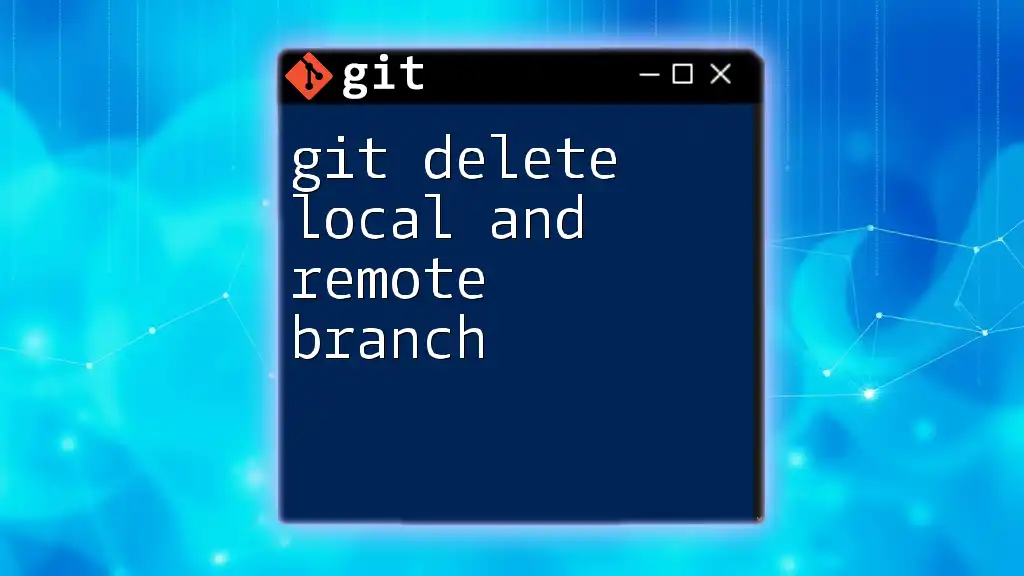
Best Practices for Branch Management
Naming Conventions
When creating branches, use clear and descriptive names that convey the purpose of the branch. Consistent naming conventions can help improve clarity for your team. For instance, prefix feature branches with `feature/`, bug fix branches with `bugfix/`, and hotfix branches with `hotfix/`.
Keeping Your Branches Clean
Regularly review your branches and delete those that are no longer in use. This practice helps maintain a clear project history and simplifies branch management.
Collaboration Tips
Effective communication is key when working with branches in a team. Make sure to inform your fellow developers about the purpose of branches and any significant changes made. Use pull requests to facilitate discussion and review before merging.
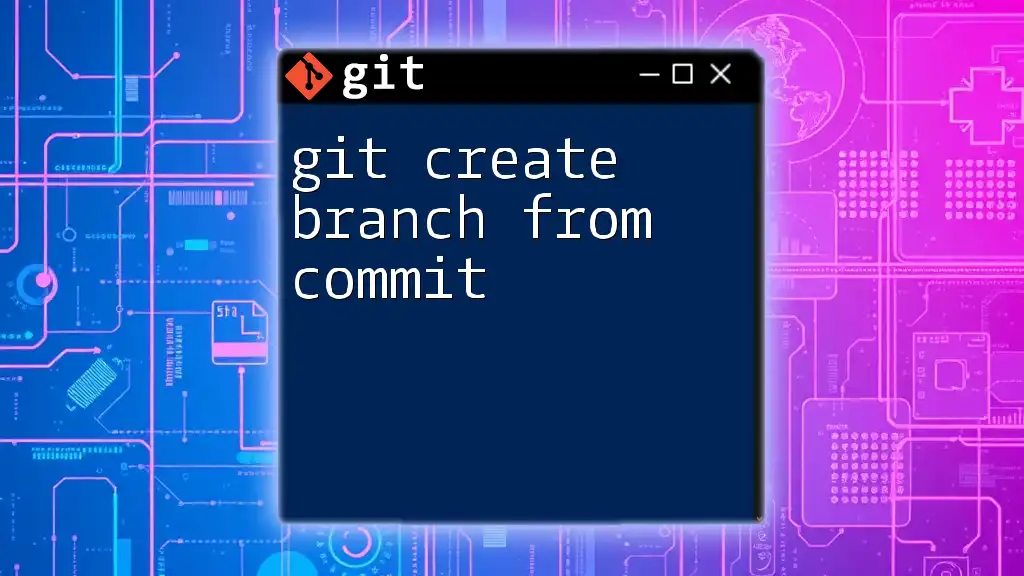
Conclusion
Understanding how to git create branch local and remote is a foundational skill in Git that significantly enhances your version control capabilities. By effectively utilizing branches, you’ll make your development process more manageable and conducive to collaboration. We encourage you to practice branching and venture deeper into Git's powerful features for a more structured development approach.
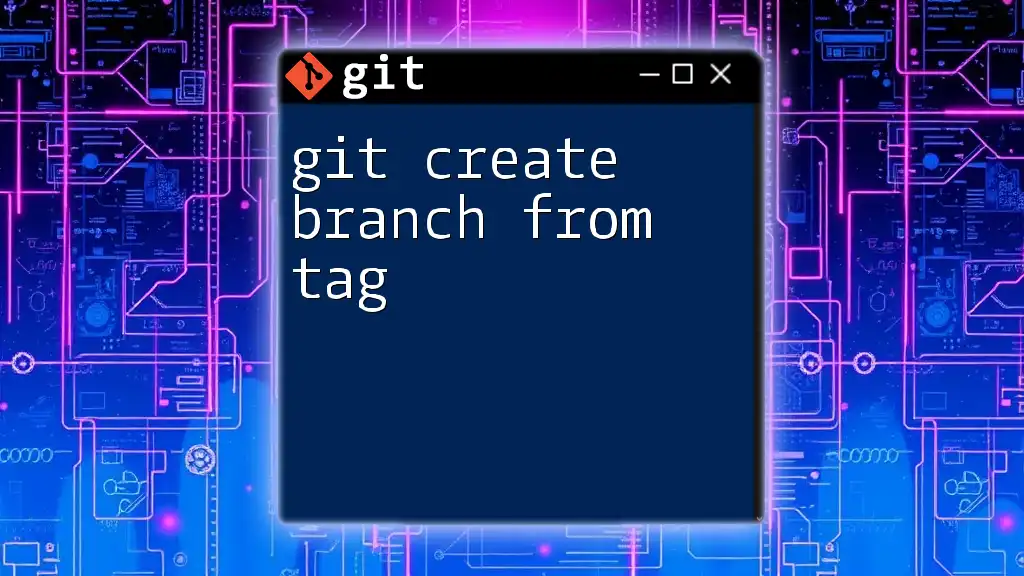
Call to Action
Start implementing what you’ve learned today! Test your knowledge by creating and managing branches in your projects. For more detailed tutorials, resources, and training sessions, visit our website and elevate your Git skills.