To rename a Git branch both locally and remotely, first rename the local branch using `git branch -m old-branch-name new-branch-name`, then push the renamed branch to the remote repository with `git push origin new-branch-name`, and finally delete the old branch from the remote with `git push origin --delete old-branch-name`.
git branch -m old-branch-name new-branch-name
git push origin new-branch-name
git push origin --delete old-branch-name
Understanding Branch Naming Conventions
What is a Branch in Git?
A branch in Git allows you to diverge from the main line of development and continue to work without affecting that main line. This is particularly useful in collaborative environments, where multiple developers are working on different features or fixes. Each branch acts as an independent space where changes can be made, tested, and perfected before being merged back into the main branch (commonly referred to as `main` or `master`).
Common Branch Naming Patterns
When creating branches, it’s crucial to adopt a consistent naming convention. Common patterns include:
- Feature branches (e.g., `feature/new-user-registration`)
- Bugfix branches (e.g., `bugfix/fix-login-issue`)
- Hotfix branches (e.g., `hotfix/urgent-fix`)
Descriptive names provide clarity and help others understand the purpose of the branch at a glance. Consistency in naming also simplifies branch management over time.
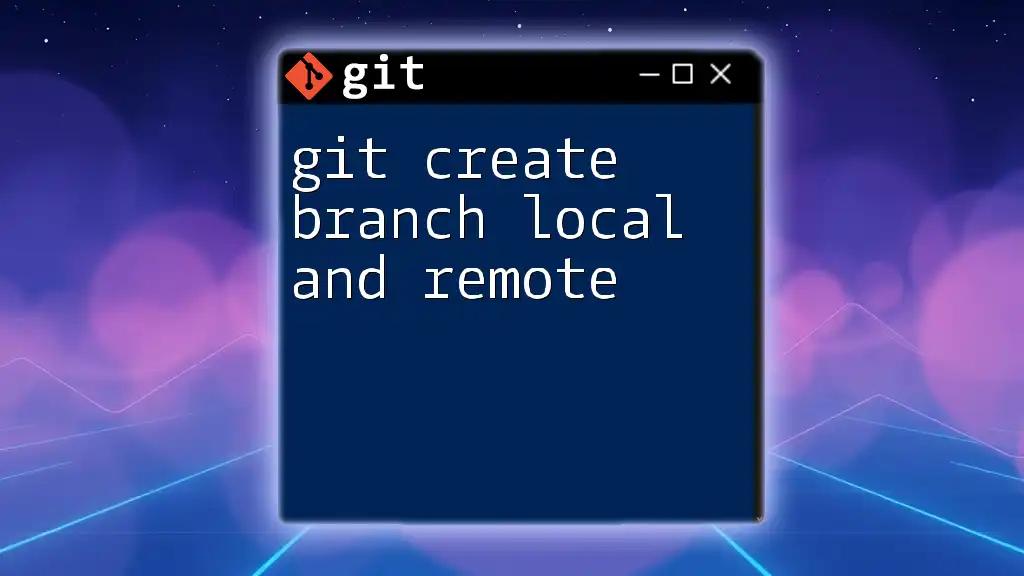
Renaming Local Git Branches
How to Check Current Branch
Before renaming a branch, it's important to know what branch you are currently on. You can check your current branch with the command:
git branch
This will list all local branches, with the current branch highlighted.
Steps to Rename a Local Branch
If You Are On the Branch
You can easily rename the branch you’re currently working on (the checked-out branch) using the following command:
git branch -m new-branch-name
Here, the `-m` flag stands for "move" and allows you to rename the branch seamlessly.
If You Are Not On the Branch
If you wish to rename a branch that you are not currently on, you can do so with:
git branch -m old-branch-name new-branch-name
In this case, you explicitly specify the old and new branch names.
Verifying the Rename
To ensure that the branch has been renamed successfully, you can run the command again to list the branches:
git branch
This will show you the updated list of branch names, confirming that your rename was successful.

Renaming Remote Git Branches
Understanding Remote Branches
Remote branches represent branches stored on a remote repository (like GitHub, GitLab, or Bitbucket). They allow multiple collaborators to work together seamlessly. Renaming a remote branch is a bit more complex than renaming a local branch, as it involves multiple steps: deleting the old branch and pushing the new one.
Steps to Rename a Remote Branch
Delete the Old Branch from Remote
First, you need to delete the old branch from the remote. You can use the following command to do this:
git push origin --delete old-branch-name
This command tells Git to remove the specified branch from the remote repository. Note that this action will affect all collaborators accessing this branch.
Push the New Branch to Remote
After deleting the old branch, you must push the renamed branch to the remote repository:
git push origin new-branch-name
This creates the new branch on the remote and makes it available for everyone to work with.
Reset Upstream Branch for New Local Branch
To establish the upstream connection for the renamed branch, you’ll need to set the remote tracking:
git push --set-upstream origin new-branch-name
The `--set-upstream` option enables your local branch to track the remote branch, allowing you to use commands like `git pull` and `git push` easily.
Verifying the Rename on Remote
Finally, to check if the rename was successful on the remote repository, you can list all remote branches with:
git branch -r
This command will show you the updated list of remote branches, confirming the new name is now in place.
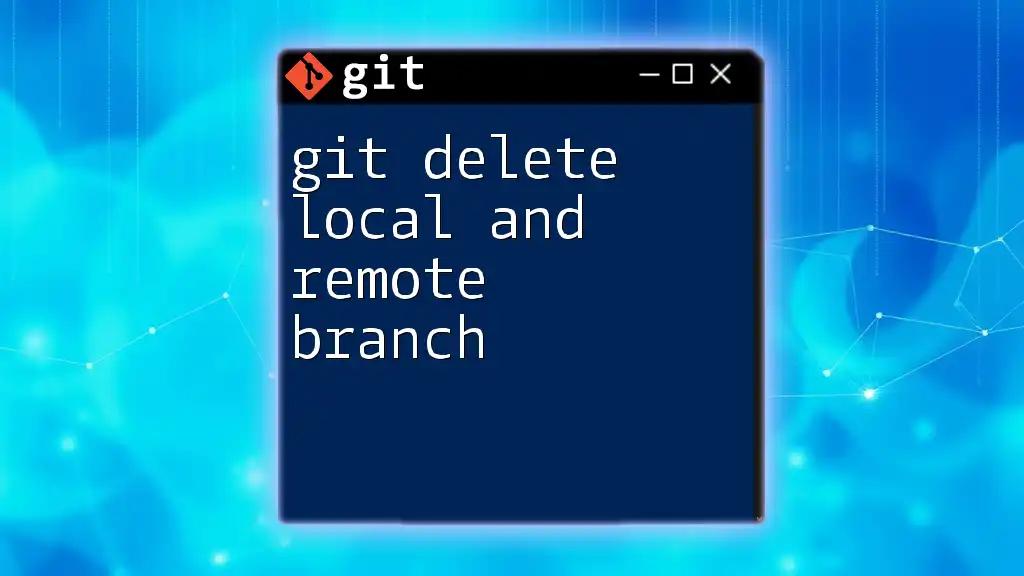
Handling Common Issues
What if the Branch is Already Present Remotely?
If you try to push a branch with a name that already exists on the remote, you will receive an error. To resolve this, you must either delete the existing remote branch first (as described earlier) or choose a new, unique name for your branch.
Recovering a Mistakenly Deleted Branch
Accidentally deleting a branch can be stressful, but Git provides a safety net through its reflog. You can access the reflog with:
git reflog
This command shows the history of your Git actions, including deleted branches. You can find the commit reference for a deleted branch and recover it using:
git checkout -b branch-name commit-ID
Replace `branch-name` with what you want to call the restored branch and `commit-ID` with the reference of the last commit in that branch.
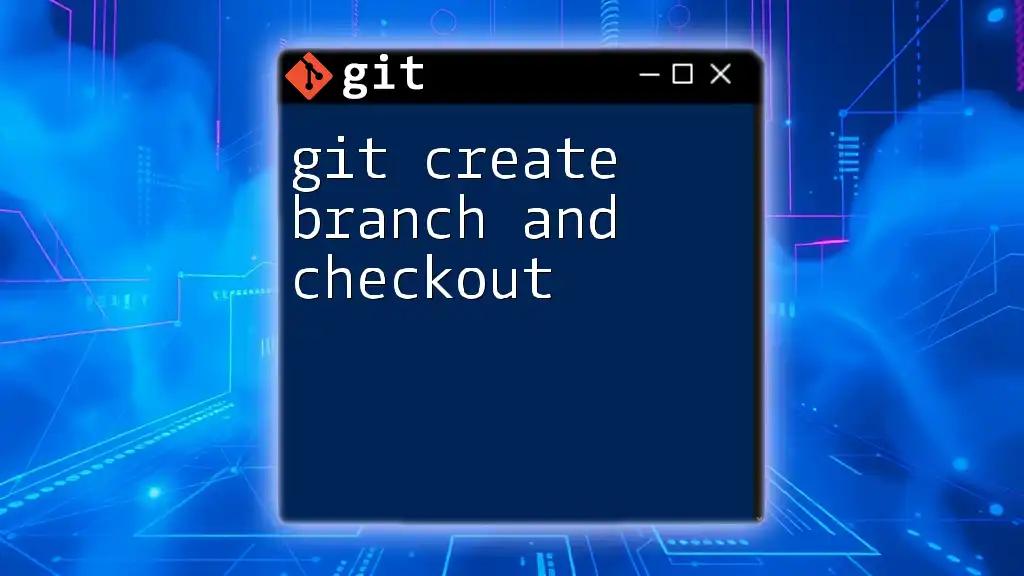
Best Practices for Branch Naming and Management
Consistency in Naming
Establishing a consistent naming convention for your branches significantly improves organization and collaboration within any project. When all team members adhere to established naming conventions, confusion is minimized, and work can proceed more smoothly.
Regular Maintenance of Branches
It’s good practice to regularly check and clean up branches that are no longer in use or that have been merged. To delete a local branch after merging, use:
git branch -d branch-name
Maintaining a tidy branch structure helps reduce clutter and keeps focus on ongoing development efforts.
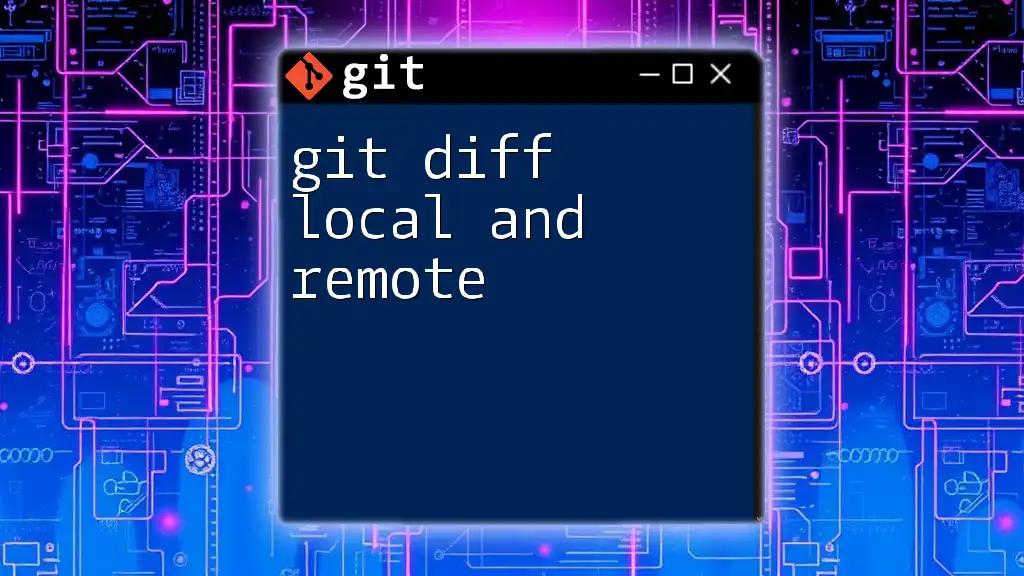
Conclusion
Renaming a Git branch, whether local or remote, is a straightforward process once you understand the steps involved. By employing best practices in branch naming and regularly maintaining your branches, you can create a more cohesive and manageable development environment. With practice, you will become adept at using Git commands efficiently, strengthening your skills in version control and collaborative software development.