The `git diff` command allows you to compare differences between your local repository and its remote counterpart, highlighting any changes that have not yet been pushed or pulled.
git diff origin/main
Understanding Git Diff
What is Git Diff?
`git diff` is a powerful command in Git that allows you to see the differences between various states of your files. It highlights changes made in your working directory compared to the index (staging area) or the last commit. This function is essential for understanding precisely what has changed at each stage of your development process.
How Git Diff Works
Git tracks changes in files using a mechanism that involves snapshots, which are stored as commits. Each time you make a change to a file, Git calculates a “diff” by comparing the previous commit to the current state of your files. This allows you to see precisely what lines of code have been added, removed, or modified.
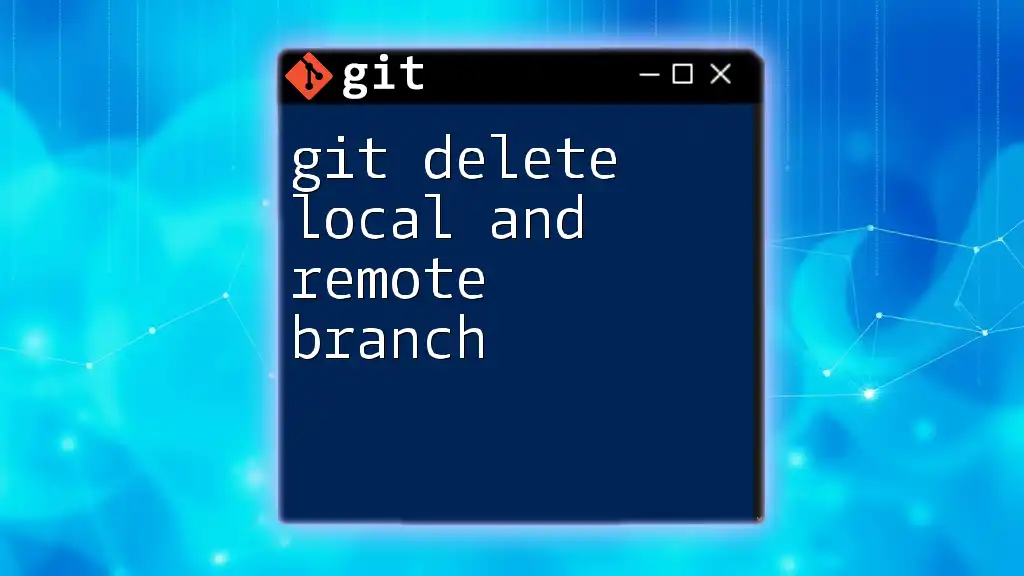
Setting Up Your Git Environment
Prerequisites
Before you can effectively work with `git diff`, ensure you have Git installed on your system. Familiarize yourself with basic Git concepts, including repositories, commits, and branches, as these are crucial for understanding how to use the command effectively.
Cloning a Repository
To start using Git, you may often need to clone a repository. Cloning creates a local copy of a remote repository, enabling you to modify and commit changes locally. Use the following command to clone a repository:
git clone [repository-url]
This action initializes a new directory containing your local copy of the specified repository, allowing you to work independently of the original source.
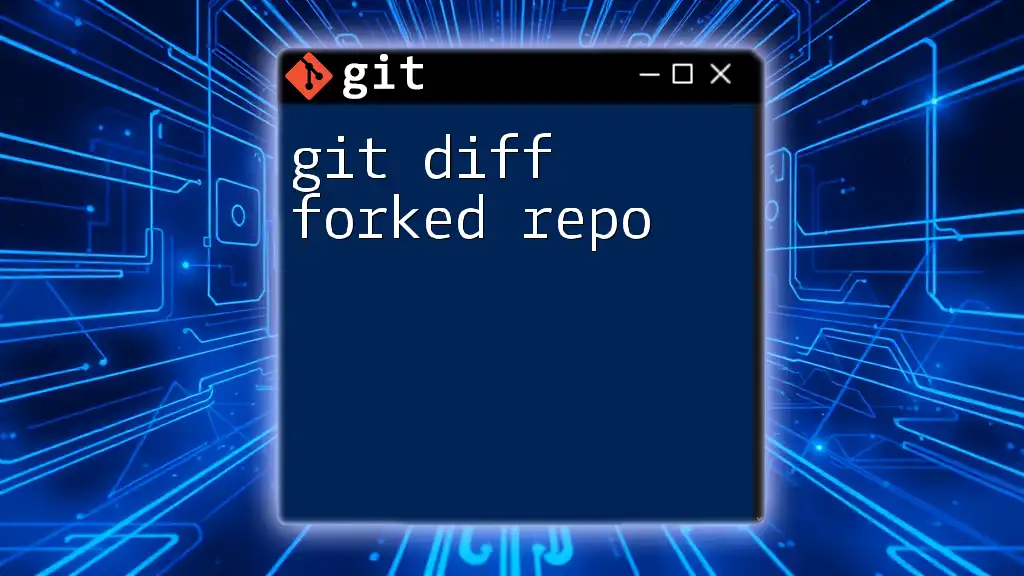
Comparing Local and Remote Changes
Purpose of Comparing Local and Remote Repositories
Comparing local and remote repositories is vital, especially in collaborative environments. It helps you understand how your changes stack up against those made by other developers, ensuring you are not overwriting or conflicting with their work.
Using Git Diff to Compare Local and Remote
To compare your local changes with those on the remote repository, you can use the `git diff` command followed by the reference to the remote branch. The following command will show you the differences between your local branch and the remote branch, typically called `origin/main`:
git diff origin/main
This command provides a side-by-side view of your changes relative to what is currently available on the remote. It’s essential to understand that `origin` refers to your default remote repository, while `main` is the primary branch in many projects.
Showing Changes in a Specific File
There may be times when you only want to check the differences made to a specific file. You can specify the file path along with the `git diff` command, as shown below:
git diff origin/main -- path/to/file.txt
This command will output the differences for `file.txt` only, making it easier to focus on specific modifications without being overwhelmed by other changes.
Visualizing Differences with Git Diff
Adding Colors for Better Clarity
Having clear visuals can significantly improve your understanding of code changes. You can configure Git to display diffs in color for better clarity by using the following command:
git config --global color.ui auto
This setting helps you distinguish between additions, deletions, and unchanged lines, making it easier to navigate through your diffs.
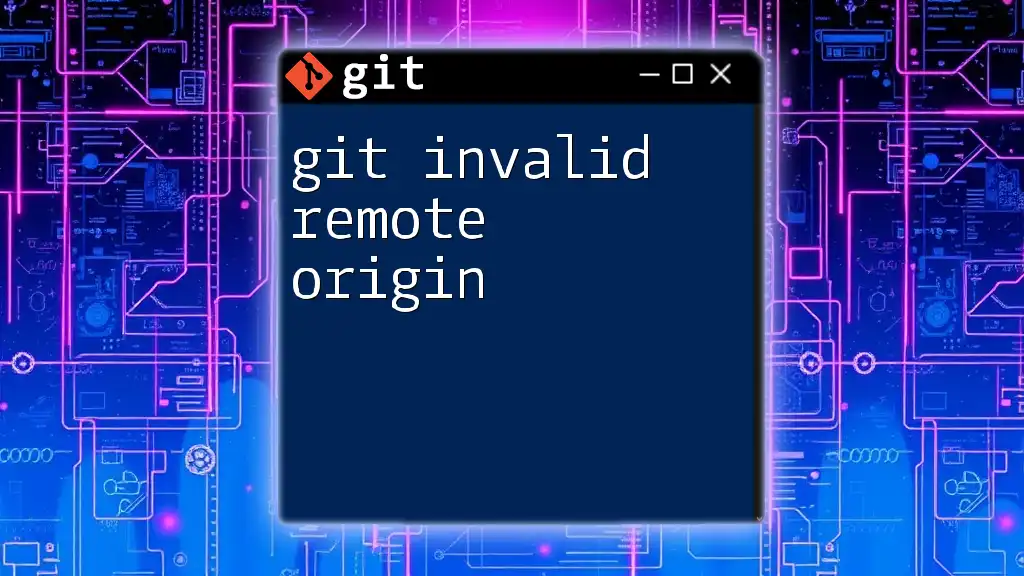
Advanced Diff Techniques
Comparing Local Changes with a Specific Remote Branch
To gain insight into how your local branch compares with a specific remote branch, you can use the following command:
git diff local-branch..origin/remote-branch
This format allows for more precise comparisons by explicitly stating which local branch you wish to compare against which remote branch, thus narrowing down the changes you want to analyze.
Using Diff for Staged and Unstaged Changes
Understanding the state of your changes—whether they are staged or unstaged—is crucial for effective version control. You can use `git diff` to see unstaged changes and `git diff --cached` for staged changes.
git diff
This command shows you the changes in your working directory that haven’t been staged yet.
git diff --cached
This command reveals any changes that are in the staging area and ready to be committed.
Exporting Diffs for Review
If you need to share your changes with teammates or prepare for a code review, exporting a diff into a patch file can be highly beneficial. You can create a patch file by executing:
git diff origin/main > changes.patch
Here, all the changes between your current state and that of `origin/main` are saved into `changes.patch`. This file can then be shared, enabling reviewers to see precisely what modifications you are proposing.
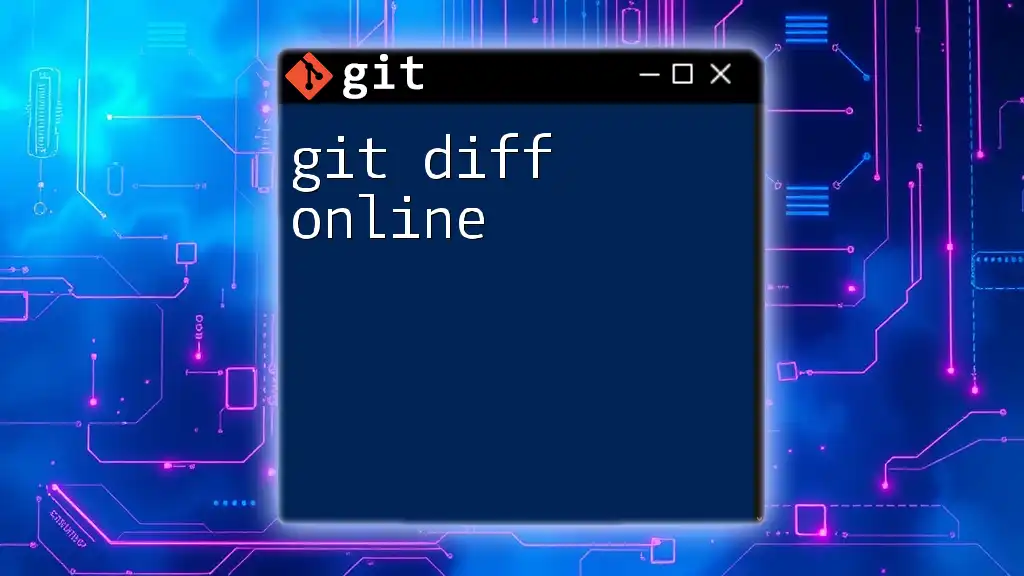
Best Practices for Using Git Diff
Keeping Your Local Branch Updated
To minimize potential conflicts and ensure you’re developing with the latest code, regularly pull updates from the remote repository. Use the following command to accomplish this:
git pull origin main
This command fetches changes from the remote and merges them into your local branch, keeping your codebase fresh.
When to Use Git Diff in Your Workflow
Make it a habit to use `git diff` before committing or merging changes. It allows you to review and verify modifications, ensuring that you are not unintentionally including any unwanted changes. This step is critical to maintaining clean and organized commits in your project history.
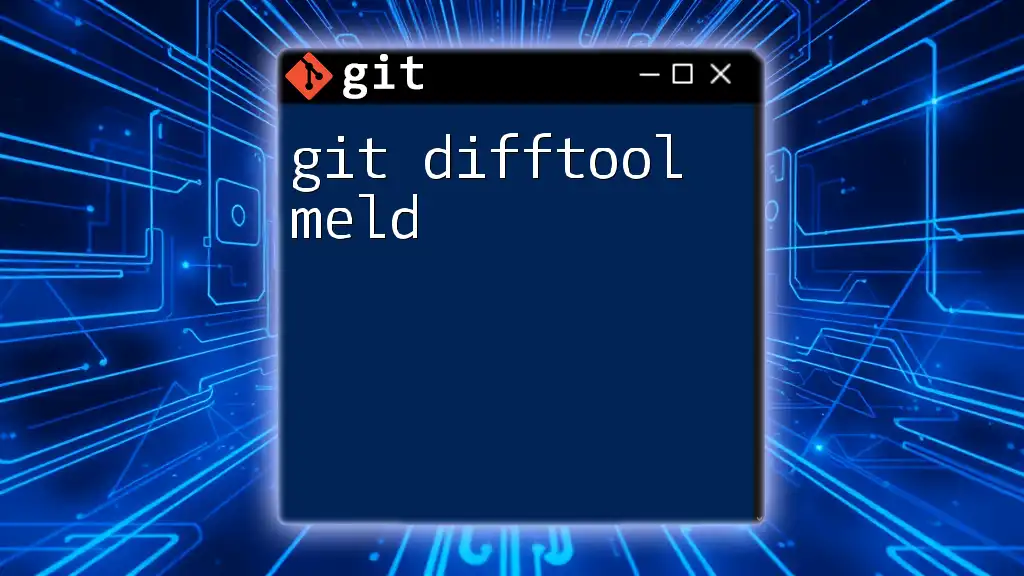
Troubleshooting Common Issues
No Changes Found
Sometimes, you may run the `git diff` command and find no changes available. Confirm that you are comparing the correct branches or that you have uncommitted changes in your local repository. Using `git status` can often reveal useful information about your current working state.
Handling Merge Conflicts Using Diff
When multiple contributors are working within a project, merge conflicts can arise, particularly when using `git diff`. Understanding how to interpret these conflicts can help you resolve them effectively. Use `git diff` to review conflicting areas in the code, and then manually edit the files to address the conflicts according to the project requirements.
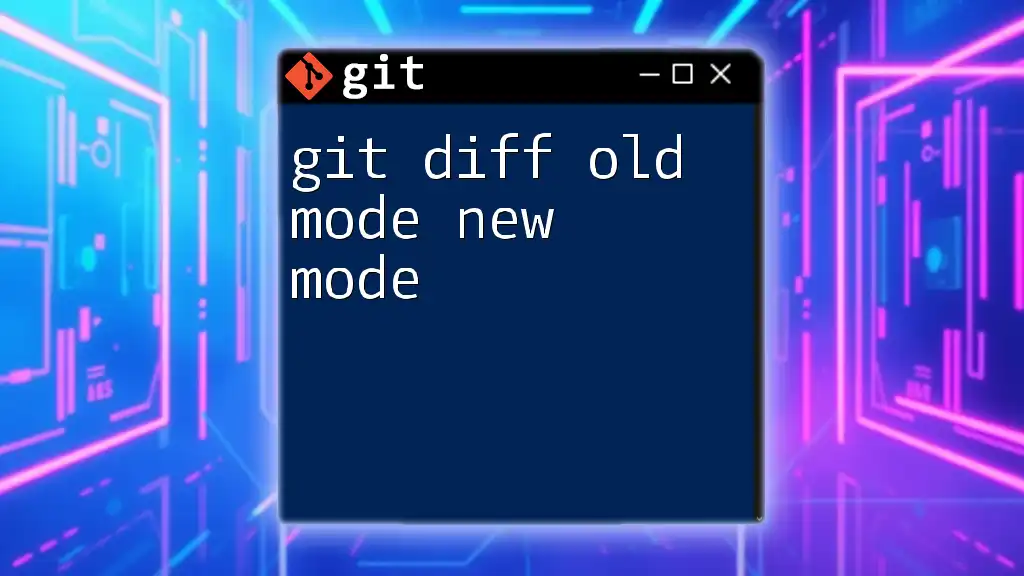
Conclusion
Utilizing `git diff` to compare local and remote changes is a fundamental practice in modern software development. By mastering this command, you can greatly enhance your workflow, maintain a healthy codebase, and collaborate more effectively with your colleagues. Make it a point to incorporate `git diff local and remote` comparisons into your daily development routine, and watch as your command over version control deepens.
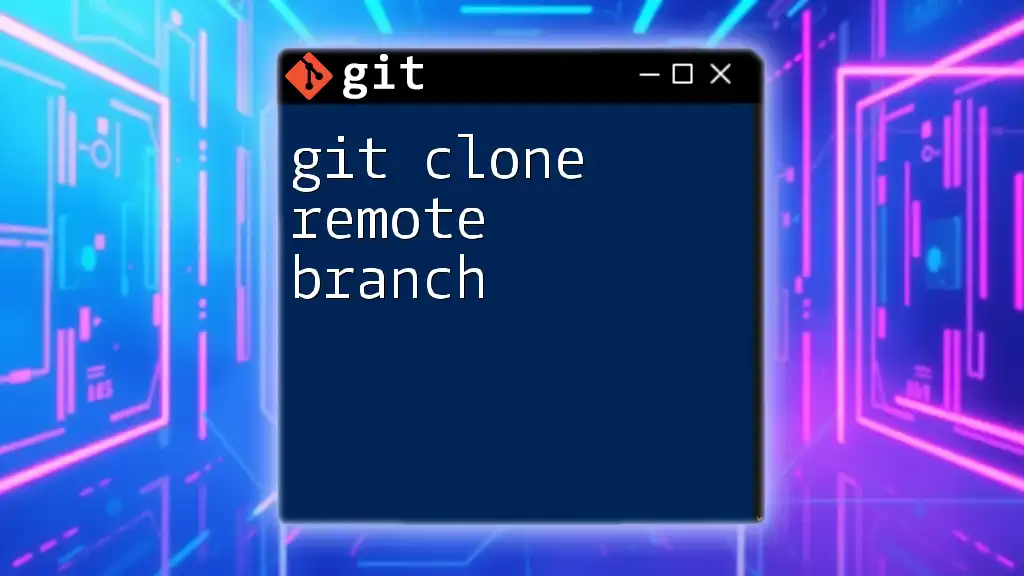
Additional Resources
Recommended Further Reading
- Explore the [official Git documentation](https://git-scm.com/doc) for in-depth explanations and additional commands.
- Consider online learning platforms like Coursera or Udemy, which offer courses specifically for mastering Git.
Frequently Asked Questions (FAQs)
-
What is the difference between `git diff` and `git status`?
`git status` provides an overview of the working directory and staging area, while `git diff` shows specific changes to files. -
Can I see differences for multiple files at once?
Yes, simply use `git diff origin/main` to see changes across all files modified in the working directory.