The `git show --remote` command allows you to display the details of a remote reference, such as a branch or tag, including its commit information and changes.
Here's how to use it:
git show --remote <remote>/<branch>
Understanding Git Show
What is `git show`?
The `git show` command is a versatile tool within Git that allows users to display information about various Git objects such as commits, tags, and trees. It’s particularly useful for inspecting details about your codebase at a specific point in history. When you run `git show <object>`, Git retrieves and presents relevant information about that object, including commit messages, author details, and the actual changes made to the code.
Importance of Remote Repositories
In Git, a remote repository serves as a central hub where multiple collaborators can push and pull changes. Unlike local repositories, which are on your own machine, remotes are hosted externally (often on platforms like GitHub, GitLab, or Bitbucket). Understanding the concept of remote repositories is essential for working in teams, allowing for seamless collaboration and version tracking.
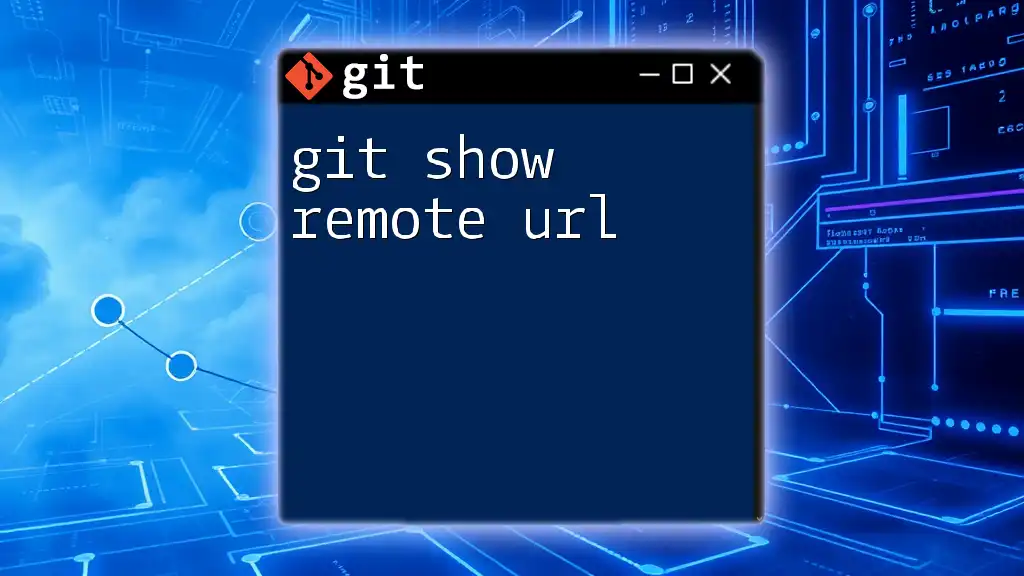
Introduction to `git show --remote`
What Does `git show --remote` Do?
The command `git show --remote` serves to specifically access and display information about the commits present in a remote branch without needing to first check them out locally. This command is particularly beneficial for developers needing a quick review of changes without switching branches or pulling in the entire history of a remote branch.
How It Works Under the Hood
When you run `git show --remote`, Git communicates with the specified remote repository to retrieve the data on the commits for the requested branch. It sends a request for information and receives a response containing the latest commit information, which it then formats for display. This ensures that you always get the most up-to-date data from the remote branch.
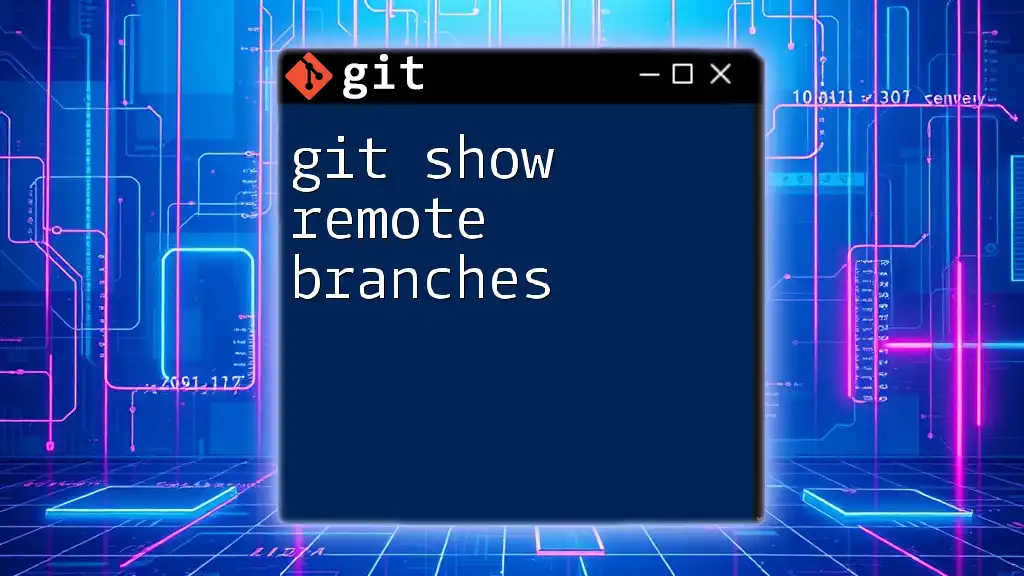
Prerequisites Before Using `git show --remote`
Setting Up Your Remote
Before you can run `git show --remote`, it's crucial to have a remote repository set up. You can check the remote configuration by running:
git remote -v
This command lists the remotes associated with your local repository along with their URLs. If you don’t see the expected remote, you may need to add it using:
git remote add <remote-name> <remote-url>
Ensuring You Have the Latest Data
Before using `git show --remote`, it’s a good practice to fetch the latest changes from the remote repository. This can be done with the following command:
git fetch origin
Fetching updates pulls in any new commits from the remote branches, ensuring that the data displayed with `git show --remote` is current.
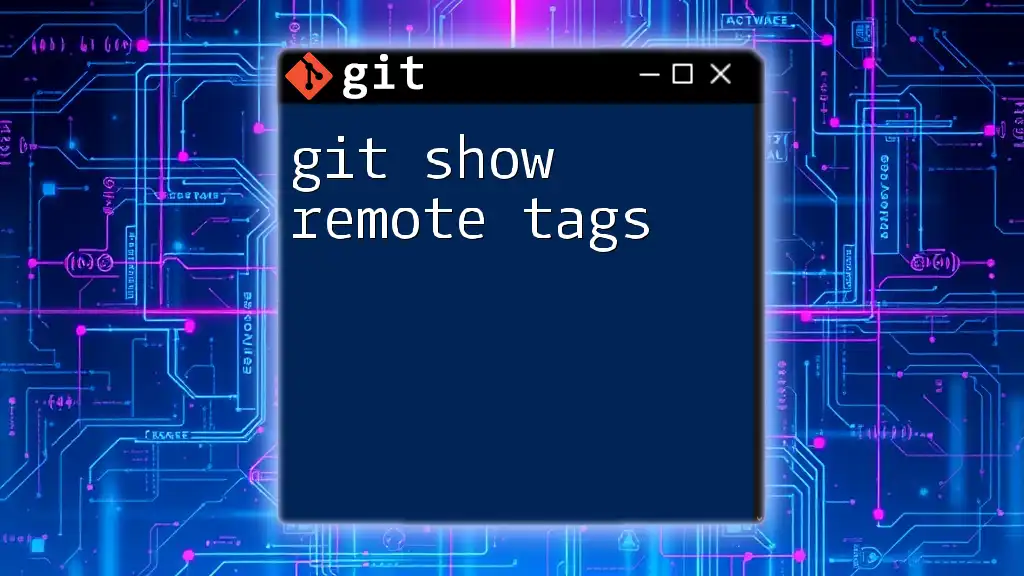
How to Use `git show --remote`
Basic Syntax
The basic syntax for the `git show --remote` command is:
git show --remote <remote-name>/<branch-name>
This format directs Git to access the specified remote and branch to display commit information.
Examples of `git show --remote`
Example 1: Viewing the Latest Commit on the `main` Branch
To view the latest commit on the `main` branch of the `origin` remote, you can use the following command:
git show --remote origin/main
This command retrieves and displays the most recent commit's details, such as the commit hash, author, date, and the actual changes made.
-
Output Example: The output may look like this:
commit 123abc456def789ghi Author: John Doe <johndoe@example.com> Date: Mon Oct 10 14:00:00 2023 -0400 Fixed bug in login feature --- src/login.js +++ src/login.js @@ -1,5 +1,5 @@ // some code
-
The output shows critical information including the author's name and the specific changes made.
Example 2: Viewing a Specific Commit from a Remote Branch
If you're looking to view a specific commit within a feature branch, you can specify it directly:
git show --remote origin/feature-branch
This command retrieves the latest commit for `feature-branch`, allowing you to understand the most recent updates before merging or collaborating further.
Common Use Cases
- Quick Inspections Before Merging Changes: Use `git show --remote` to preview commits before integrating them into your local branches.
- Reviewing Pull Requests: This command aids in examining changes associated with pull requests in a clear and concise manner.
- Understanding the Current State of a Remote Branch: Ensures you are well-informed about the progress of emergency branches used in collaborative projects.
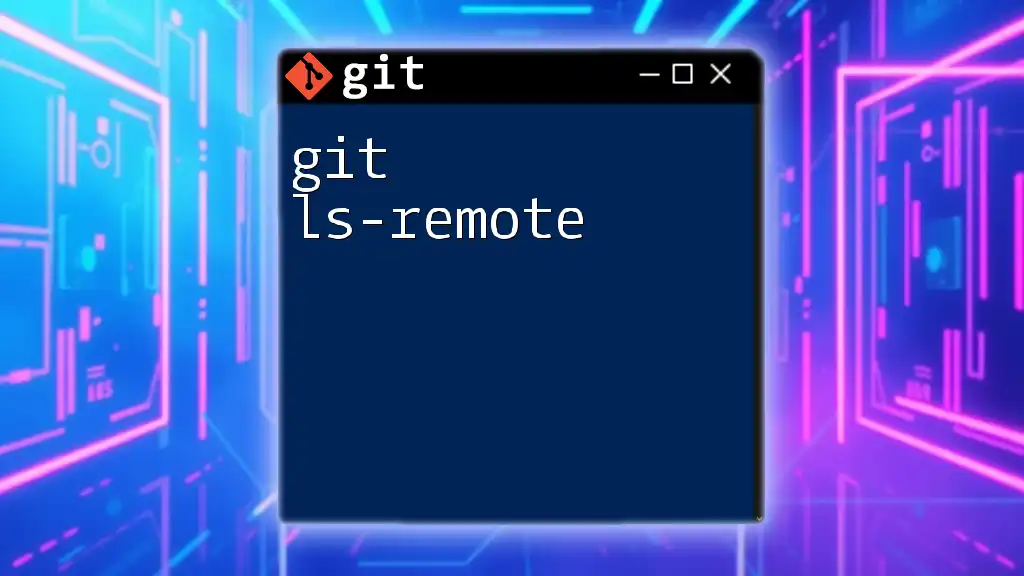
Customizing Output with `git show`
Formatting Options
You can further customize the output format of `git show --remote` by using several options. For instance, by modifying how commit details are displayed:
git show --remote --pretty=oneline origin/main
This command will format the output in a single line, displaying just the commit hash and message, making it easier to sift through multiple commits quickly.
Filtering Commits
If you wish to find commits with specific messages, the `--grep` option can be very useful:
git show --remote --grep="fix" origin/main
This command filters the displayed commits to show only those containing the word "fix" in the commit message, providing a targeted view of relevant changes.
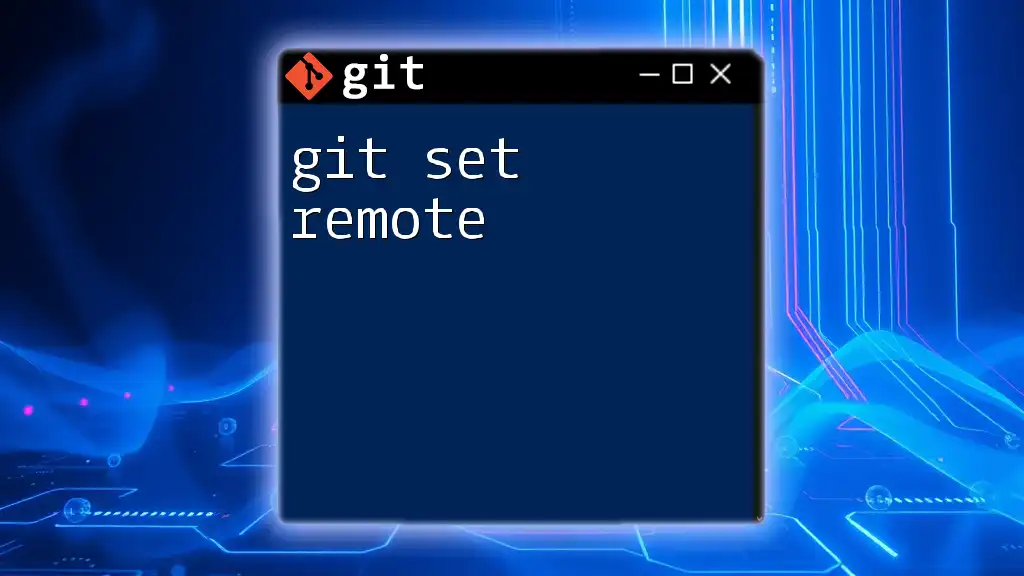
Troubleshooting Common Issues
Remote Not Found
If you encounter an error stating that the remote doesn’t exist, ensure that you have the correct remote name. You can check configured remotes with:
git remote -v
If the remote is absent, you will need to add it using the aforementioned `git remote add` command.
Permission Denied Errors
When facing permission denied errors while using `git show --remote`, it typically indicates an issue with your access credentials. Ensure that your SSH keys or HTTPS login credentials are correctly configured. Consult your Git host's documentation for specific instructions on troubleshooting these authentication issues.
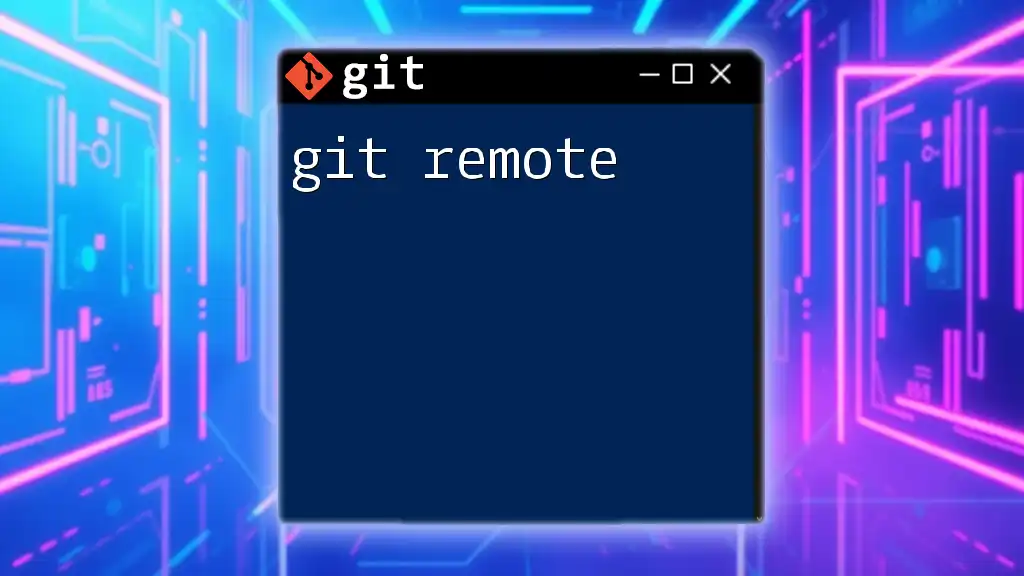
Best Practices for Using `git show --remote`
-
Regularly Check for Updates in Remote Branches: Frequent use of `git show --remote` helps you stay updated with the latest changes made by your team, allowing for better coordination.
-
Use Alongside Other Git Commands for Efficient Workflow: Combine `git show --remote` with commands like `git fetch` to maintain an organized workflow and ensure you're informed about the state of both local and remote branches.
-
Note the Importance of Context: When reviewing commits with `git show --remote`, don’t just focus on the code changes but also consider the context, such as commit messages and related issues or pull requests. This holistic view will enhance your understanding of the project’s evolution.
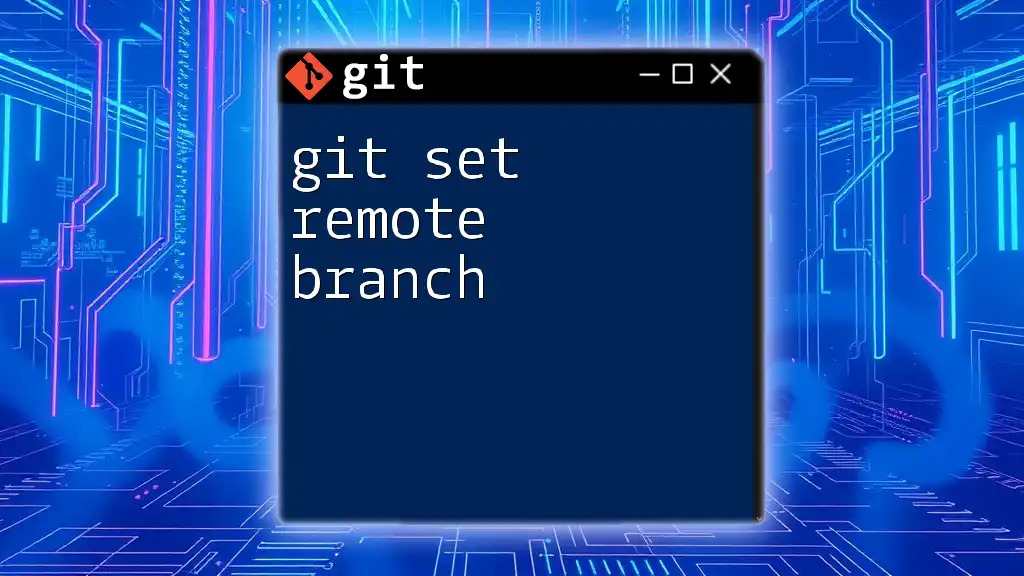
Conclusion
The `git show --remote` command is a powerful tool for developers working with version control in a team setting. By allowing quick access to remote branch information, it facilitates better collaboration and enhances workflow efficiency. Practicing its usage will significantly benefit your Git mastery, empowering you to manage collaborative projects with confidence and precision.
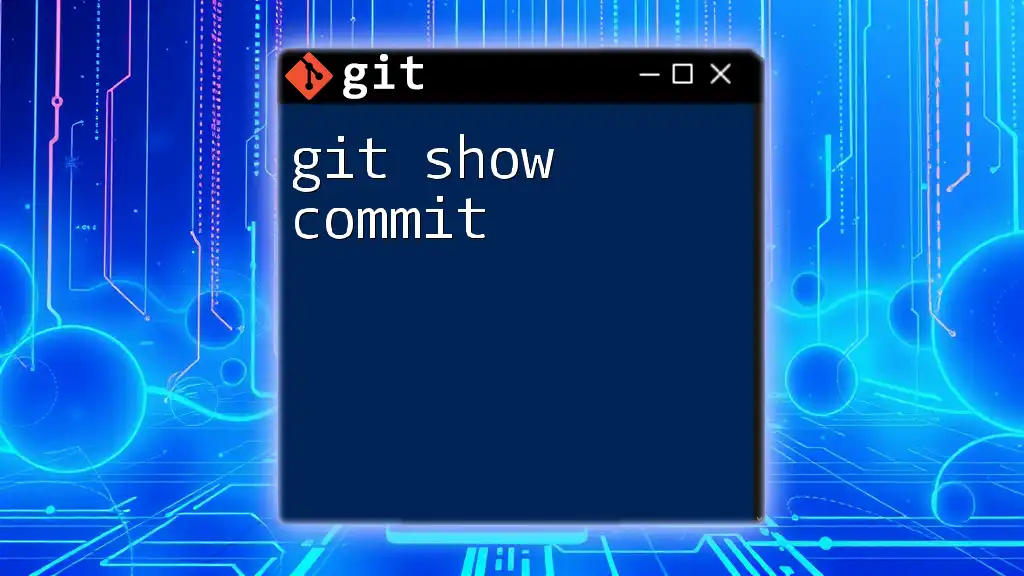
Additional Resources
For further reading and more detailed exploration of Git commands, including `git show` and remote repositories, refer to:
- [Official Git Documentation](https://git-scm.com/doc)
- [Git Branching and Remote Repositories Guide](https://git-scm.com/book/en/v2/Git-Branching-Remote-Branches)
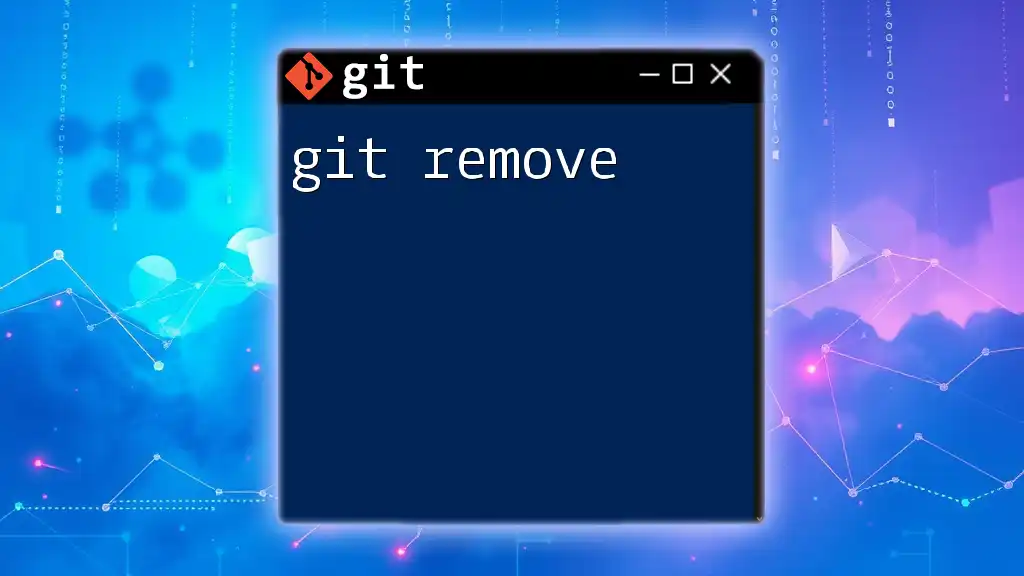
FAQ Section
What happens if the remote branch does not exist?
If you try to run `git show --remote` on a branch that does not exist, you will receive an error message indicating that the remote branch could not be found. Double-check the remote name and the branch name to ensure that they are correctly specified.
Can I use `git show --remote` for multiple remotes?
While `git show --remote` is primarily designed to access a specific remote repository, you can execute the command for different remotes by specifying their respective names. However, you cannot retrieve information from multiple remotes in a single command execution.
Is `git show --remote` available in all Git versions?
Yes, `git show --remote` is available in all modern versions of Git. However, it's always a good idea to ensure that you are using an updated version of Git to take advantage of the latest features and improvements.