The `git remote` command is used to manage and manipulate the set of remote repositories that your local Git repository can track and interact with. Here's a code snippet to add a remote repository:
git remote add origin https://github.com/username/repository.git
Understanding Git Remote
What is a Remote in Git?
In Git, a remote repository serves as a central hub where team members can collaborate on the same project. Unlike a local repository, which resides on your machine, a remote is typically hosted on platforms like GitHub or GitLab, allowing multiple users to access the same codebase from different locations. Understanding the relationship between local and remote repositories is crucial for effective collaboration.
The local repository is where you perform operations such as commits and branching, while the remote repository is where those changes are shared and stored. This structured approach facilitates teamwork and version control, keeping the history of changes clean.
Key Concepts of Git Remote
When working with a remote repository, you interact primarily with three fundamental operations:
- Fetch: Retrieves the latest changes from the remote but does not merge them into your local branch.
- Push: Sends your committed changes to the remote branch, updating the remote repository.
- Pull: Combines fetching and merging, allowing you to get changes from the remote and apply them to your local branch efficiently.
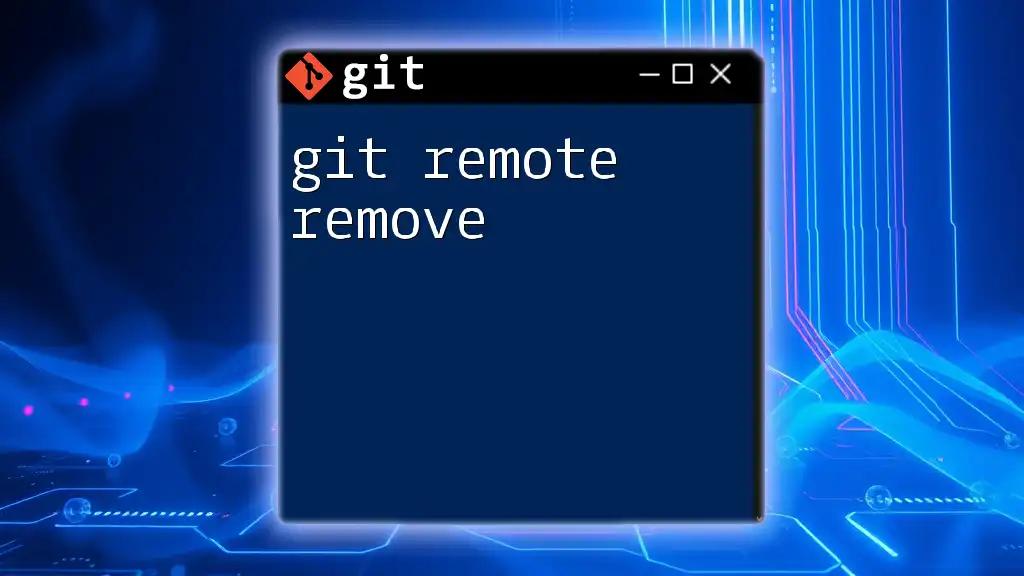
Common Git Remote Commands
Viewing Remotes
To view the remotes that have been configured for your repository, you can use the following command:
git remote -v
This command lists all configured remotes along with their respective URLs, giving you a quick overview of where your local repository can send its changes and where it can fetch updates from.
Adding a Remote
When you want to connect your local project to a remote repository, you will use the add command:
git remote add <name> <url>
For example, if you want to connect your local repository to a GitHub repository, you'd execute:
git remote add origin https://github.com/user/repo.git
In this example, origin is a conventional name that represents the primary remote repository. Naming remotes appropriately helps maintain clarity in larger projects.
Removing a Remote
If, for any reason, you need to disconnect from a remote repository, you can use:
git remote remove <name>
For instance, to remove the previously added origin, the command will be:
git remote remove origin
This is useful if you are changing the hosting service or if the remote repository is no longer relevant to your project.
Renaming a Remote
If you need to change the name of a remote for better organization, use:
git remote rename <old-name> <new-name>
Example:
git remote rename origin upstream
Renaming remotes can increase the clarity of your working environment, especially if you have several collaborators or multiple repositories.
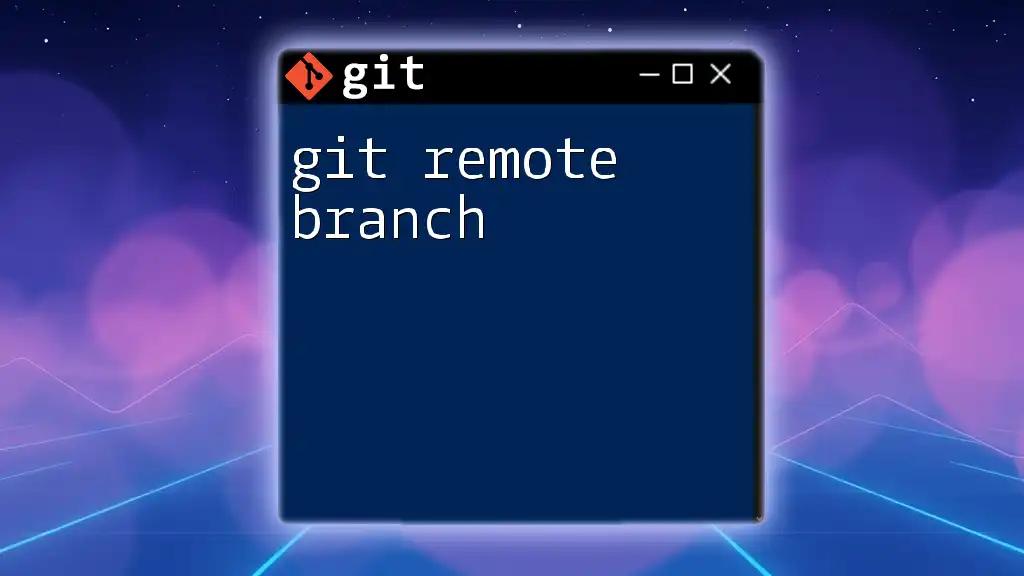
Working with Remote Branches
Understanding Remote Branches
Remote branches act as pointers to the state of branches in the remote repository. By fetching or pulling from a remote, you can update your local copy of these branches without making any changes to your local branches. This separation allows you to freely explore changes without the risk of accidentally overwriting your own work.
Listing Remote Branches
To see all remote tracking branches, you can run:
git branch -r
This command provides you with a complete list of remote branches, allowing you to understand what branches are available for collaboration.
Fetching Changes from a Remote
Fetching updates your local repository with the latest changes from the remote, without merging them:
git fetch <name>
For example, you can fetch changes from origin with:
git fetch origin
This command retrieves new commits from the remote repository but will not modify your working directory or current branches.
Pulling Changes from a Remote
If you want to incorporate changes from a remote branch directly into your current branch, use:
git pull <name> <branch>
For instance:
git pull origin main
This operation fetches the changes and immediately merges them, integrating recent updates into your current branch.
Pushing Changes to a Remote
To share your committed changes, you'll push them to the remote repository with:
git push <name> <branch>
For example:
git push origin main
Understanding best practices about when to push is critical. It’s advisable to pull the latest changes before you push to avoid conflicts and ensure you are working on the most up-to-date version of a branch.
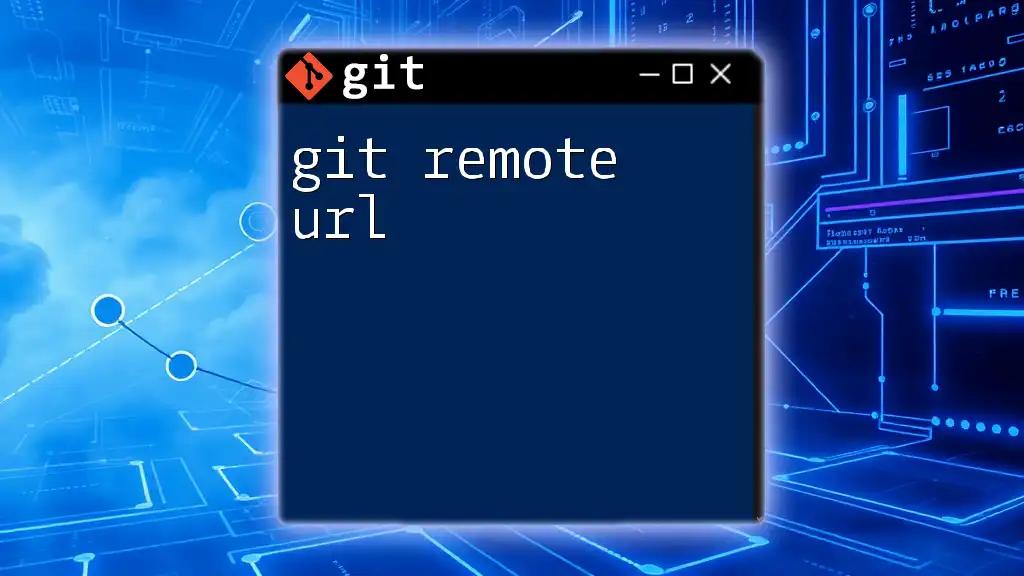
Advanced Git Remote Commands
Working with Multiple Remotes
In some scenarios, it may be beneficial to configure multiple remotes, for example, when you want to push to one repository and fetch from another. This allows for greater flexibility in your workflow.
Understanding how to reference and manage these remotes is crucial. You can simply run `git remote -v` to see all remotes and their URLs. Use appropriate commands (add, remove, rename) as needed to maintain your remote connections.
Configuring Remote Git Repositories
Sometimes it's necessary to change the URL associated with a remote, particularly if a repository moves or if you switch to using SSH instead of HTTPS. The command to do this is:
git remote set-url <name> <newurl>
For example, switching URLs might look like this:
git remote set-url origin https://github.com/user/new-repo.git
This command ensures the remote reference points to the correct repository.
Viewing and Modifying Remote Configuration
To get detailed information about a remote, including its fetch and push URLs:
git remote show <name>
You will receive output describing the remote, including information about which branches are tracked. This is especially helpful when managing a larger repository with many collaborators.
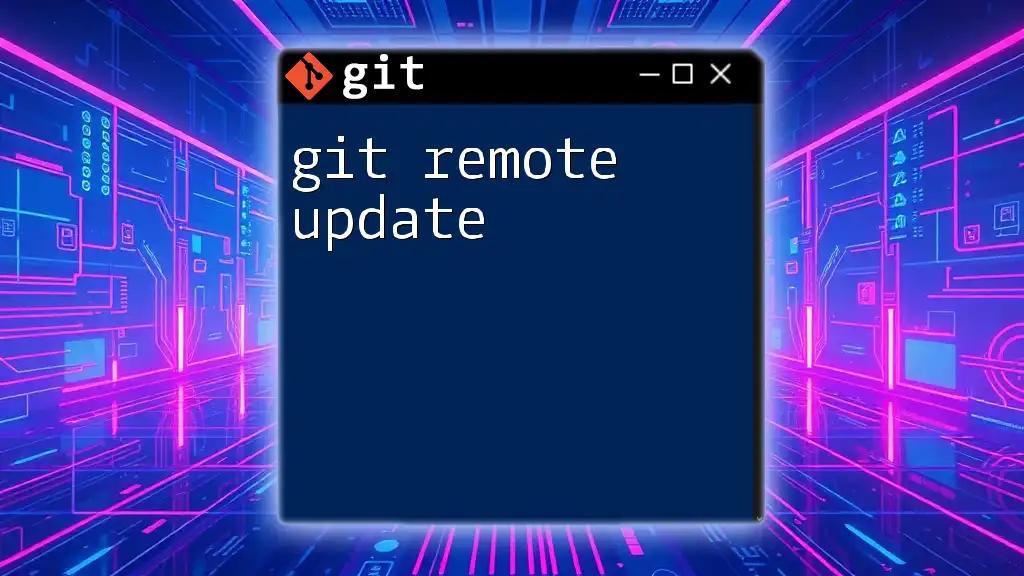
Troubleshooting Common Issues with Git Remote
Remote Connection Issues
When working with remotes, you may encounter connection issues due to various reasons, including incorrect URLs or loss of internet connectivity. Always check your remote URL using:
git remote -v
If the URL is incorrect, update it using the configuration command mentioned earlier.
Resolving Conflicts during Fetch/Pull/Push
Conflicts can occur when changes made on the remote are incompatible with your local changes. Here are strategies to resolve conflicts:
- Before Pulling: Regularly pull changes from the remote to minimize conflicts.
- Merge Conflicts: If conflicts arise, Git will indicate the files that need resolution. Open the files, resolve the conflicts manually, then stage and commit the changes.
Understanding how to handle these scenarios will keep your workflow running smoothly.
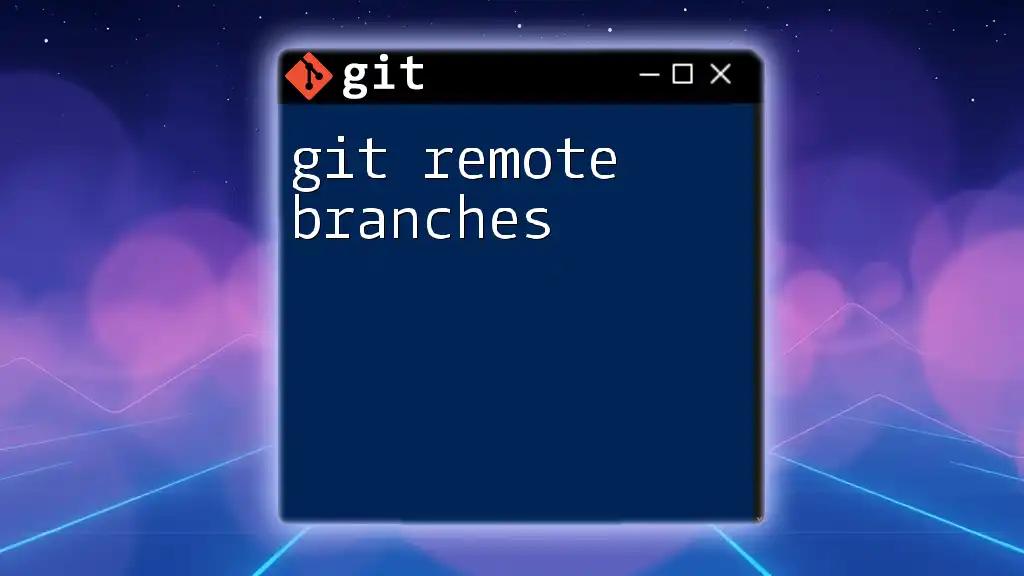
Conclusion
In summary, understanding and effectively using git remote is vital for collaboration and managing your codebase in a distributed environment. By mastering commands such as adding, removing, and manipulating remotes, as well as fetching and pushing changes, you'll enhance your productivity and streamline your development processes.
Engagement with remote repositories is essential in any team-based development workflow, and continual practice will help you become proficient. Consider exploring additional courses and resources to deepen your Git knowledge and enhance your skills in version control.