The `git remote add -f` command adds a new remote repository and fetches its branches immediately, allowing you to work with them right away.
git remote add -f <remote-name> <remote-url>
What is `git remote add -f`?
`git remote add -f` is a powerful command in Git that combines adding a remote repository and immediately fetching its content. Understanding this command is crucial for any developer who interacts with remote repositories, as it streamlines the process of syncing your local repository with remote changes.
When using `git remote add`, you'd typically specify a name for the remote and the corresponding URL, allowing you to reference this remote later for commands like push and pull. The `-f` flag stands for "fetch" and instructs Git to fetch all the branches and commits from the newly added remote immediately after it's added, ensuring your local repository is up-to-date.
Use Cases
You should consider using `git remote add -f` in situations such as:
- Setting up a new collaboration environment: When starting to work on a new project that already has a remote repository, adding the remote and immediately syncing gets you up to speed right away.
- Tracking upstream changes: If you’re forking a repository and want to keep your fork updated with the upstream, this command can be a quick way to establish a connection and fetch updates.
- Working with remote tracking branches: When you need to start work on a branch that exists in a remote repository, you can quickly fetch it using this command combination.
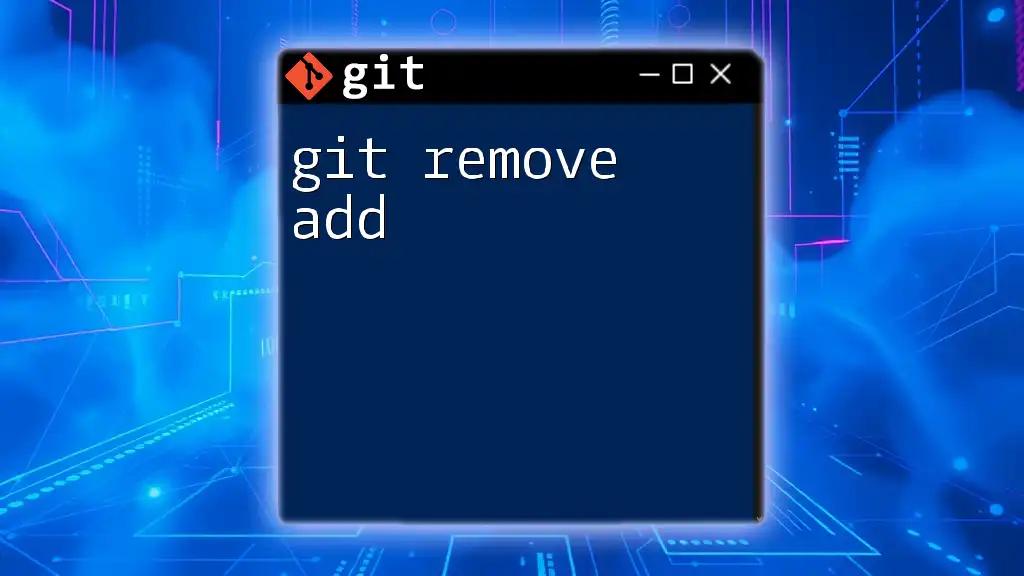
Setting Up Your Repository
Initializing a Git Repository
Before you can add a remote, you need to have a Git repository set up. You can create a new repository using the following command:
git init my-new-repository
This command initializes a new Git repository in the specified directory. You can navigate into this repository to begin working.
Adding a Remote Repository
Once your repository is initialized, you can add a remote. A remote repository is essentially a version of your project that is hosted elsewhere, making collaboration easier. The command syntax for adding a remote repository is as follows:
git remote add <name> <url>
Here, `<name>` is a reference you choose (often `origin` for the main remote), and `<url>` is the location of the remote repository. For instance:
git remote add origin https://github.com/user/repo.git
This command sets up a connection to the specified GitHub repository.
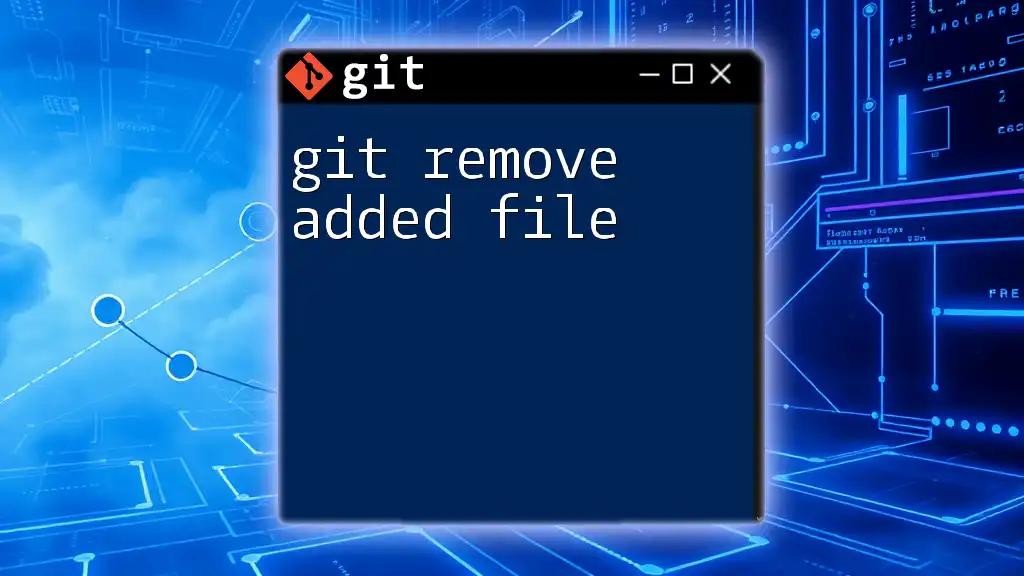
How to Use `git remote add -f`
Step-by-Step Guide
To utilize `git remote add -f`, simply include the `-f` flag in your command when adding the remote. The command structure is as follows:
git remote add -f <name> <url>
For example:
git remote add -f origin https://github.com/user/repo.git
What happens here is two-fold:
- Git adds the remote named `origin`.
- It fetches all branches and commits from `https://github.com/user/repo.git`, updating your local repository immediately.
Fetching From the Remote
When you execute `git remote add -f`, the fetch operation pulls in all the remote-tracking branches and objects. This means you don’t have to run an additional fetch command afterwards, ensuring your local repository reflects the latest state of the remote.
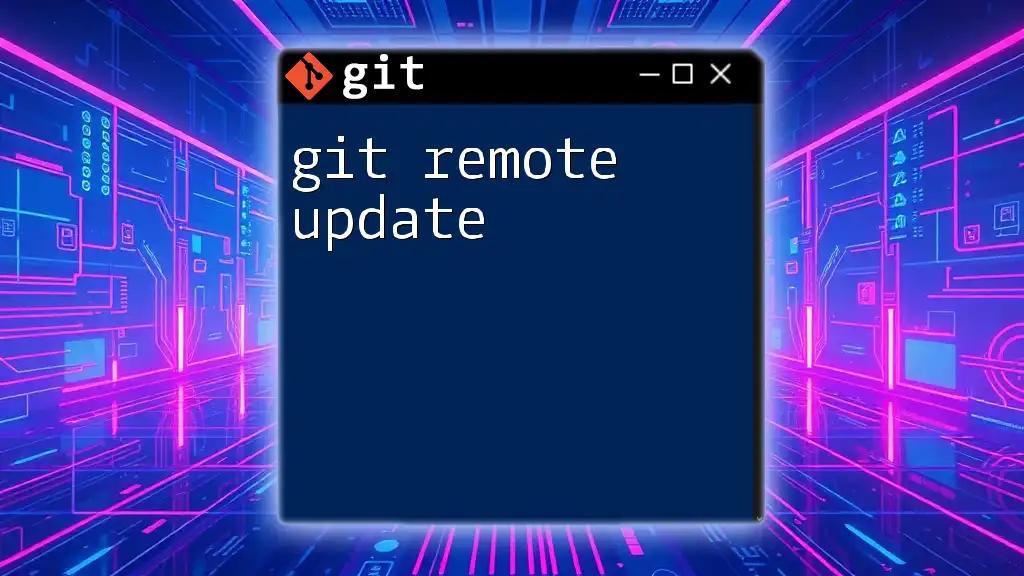
Practical Examples
Example 1: Adding a Remote Repository
Let's walk through an example to illustrate how this works in practice. Suppose you are collaborating on a project hosted on GitHub.
git remote add -f origin https://github.com/user/project.git
After running this command, you will have created a remote connection and simultaneously fetched the data. You can verify this by running:
git branch -r
This command lists all remote-tracking branches, which would now include branches from the newly added remote.
Example 2: Fetching Updates
In a situation where your remote repository gets updated, you want to ensure that your local copy is synchronized. If you had added your remote using `git remote add` without the fetch option, you'd need to run the fetch command separately:
git fetch origin
However, having used `git remote add -f`, your local repository is already updated, meaning there’s no need for this additional fetch unless new updates occur later.
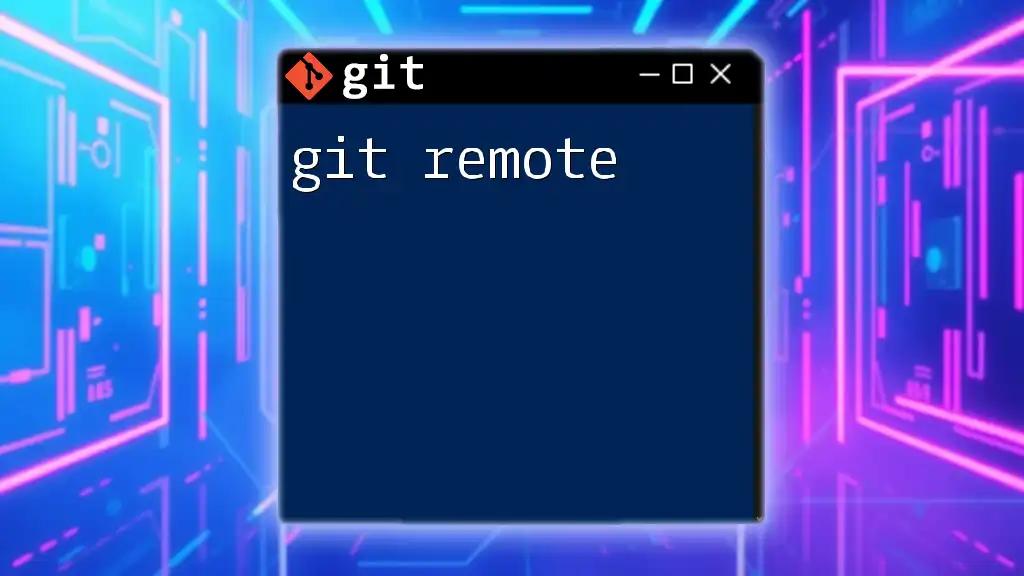
Understanding the Benefits
Why Use the `-f` Option?
Using the `-f` option is advantageous because it eliminates an extra step in your workflow. When you add a remote without the fetch option, you must remember to run `git fetch` afterwards. This can lead to inconsistencies if you forget, especially if you’re eager to start working on the latest changes.
Comparison with Other `git remote` Actions
While `git remote add -f` is unique in its immediate fetching aspect, it’s useful to be aware of similar commands:
- `git remote update`: This fetches updates from all remotes you've configured. It’s useful if you’re working with multiple remotes.
- `git fetch`: This command retrieves updates from a specific remote but requires the remote to be already defined.

Common Issues and Troubleshooting
Common Errors When Using the Command
While using `git remote add -f`, you might encounter some common issues:
- Duplicate Remote Names: If you try to add a remote with a name that's already in use, you'll see an error. To fix this, you can either rename the existing remote or remove it:
git remote remove <name>
- Invalid Repository URL: Make sure that the URL you're entering is correct. A simple typographical error can lead to access issues.
How to Troubleshoot
If you run into problems, checking your remote URLs can resolve many issues. You can list all remotes and their URLs with:
git remote -v
This command provides a clear view of your configured remotes, making it easier to spot any inconsistencies.
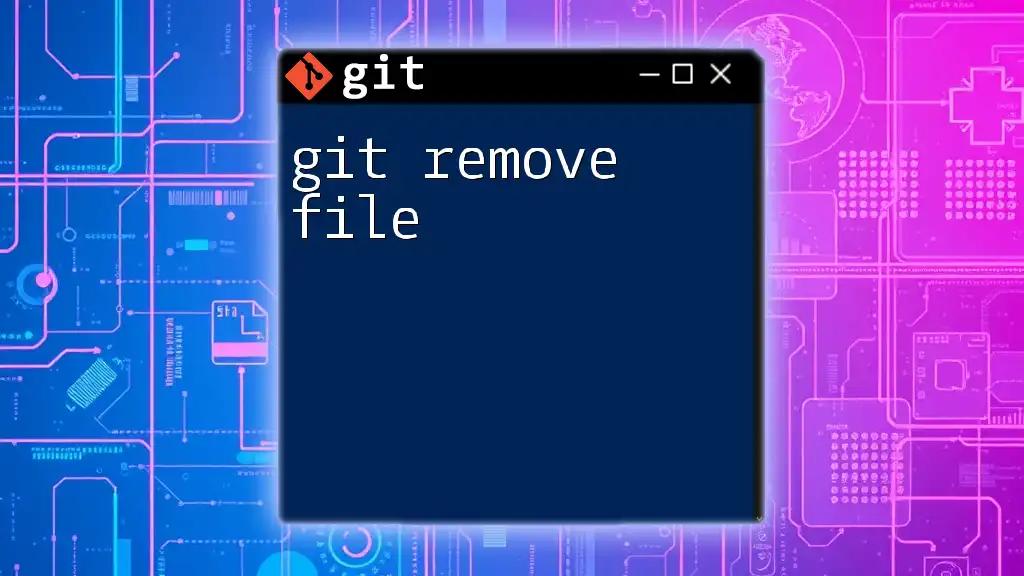
Conclusion
In summary, `git remote add -f` is a highly effective command for streamlining your workflow when dealing with remote repositories. By integrating both the addition of a remote and immediate fetching, it ensures that you're working with the most up-to-date information right from the start. This command is truly essential for anyone looking to enhance their Git skills and maintain a seamless development process.
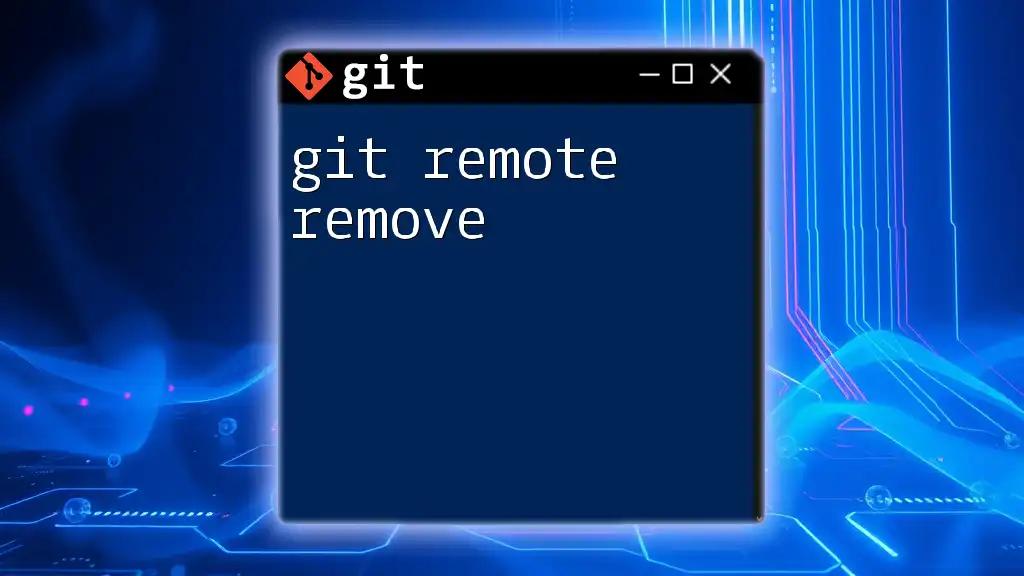
Call to Action
Ready to become a Git expert? Join our Git Learning Community today and access a wealth of resources, tutorials, and courses tailored to elevate your proficiency with Git commands. Embrace the world of version control and take your coding skills to the next level.