In Git, "remote" refers to a remote repository where your code is stored, and "origin" is the default name given to that remote repository when you clone it.
Here's a code snippet to show how to add a remote repository named "origin":
git remote add origin https://github.com/username/repository.git
Introduction to Git Remote
Git Remote allows your local Git repository to communicate with remote repositories. A remote repository serves as a centralized location where your Git project can be stored, allowing collaboration with other developers. Understanding how to work with remotes is essential for any collaborative development workflow.
Understanding the Concept of Origin
In Git, the term origin refers to the default alias Git gives to the main remote repository. When you clone a repository, Git automatically names the remote repository you cloned from as origin. Using this convention simplifies commands and promotes consistency across teams.
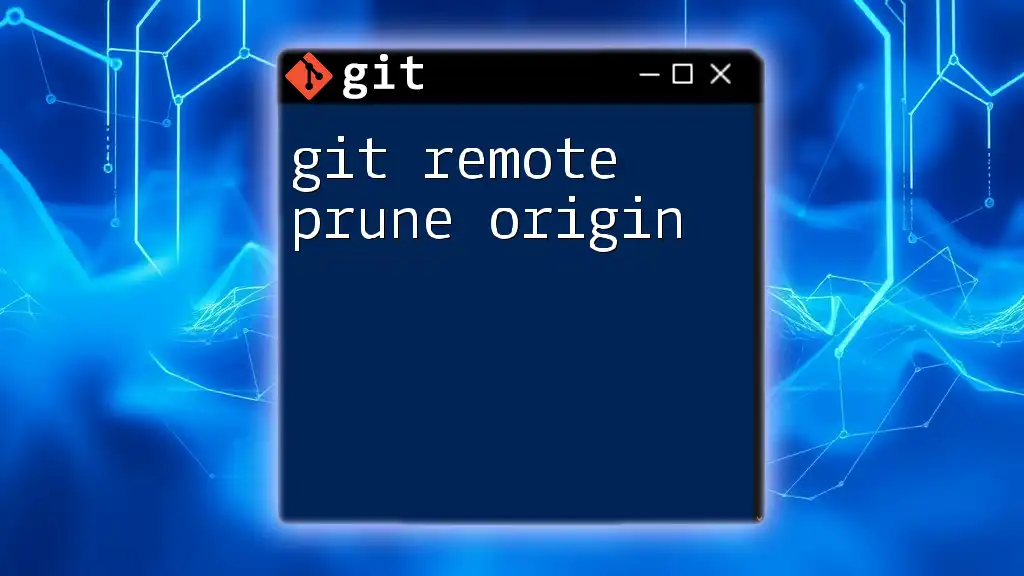
Setting Up Remote Repositories
Creating a Remote Repository
To collaborate effectively, you first need to establish a remote repository. Platforms like GitHub, GitLab, and Bitbucket provide easy ways to create remote repositories. Generally, follow these steps:
- Sign in to your preferred Git hosting service.
- Click on "New Repository".
- Fill out the necessary details such as the repository name and visibility (public/private).
Once you've established your remote repository, you can initialize a new remote repository directly on your command line if you’re using your own server:
git init --bare <repository-name>.git
This command initializes a bare repository, suitable for hosting a Git repository.
Linking Local Repository to Remote
Next, you need to connect your local repository to the remote repository. Use the following command to add a remote repository:
git remote add origin <repository-url>
For example, if you’re linking to a GitHub repository, your command might look like this:
git remote add origin https://github.com/username/repository.git
This command creates an alias (in this case, origin) for your remote repository, allowing you to use a simple name to reference it in subsequent Git commands.
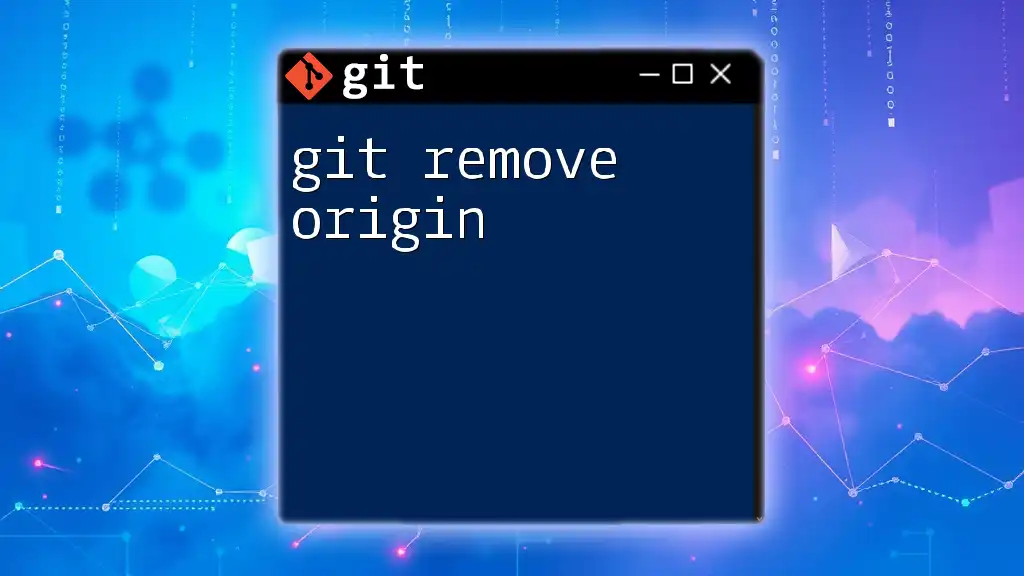
Managing Git Remotes
Checking Existing Remotes
To see all remote repositories linked to your local repository, execute:
git remote -v
This command will display the names and URLs of each remote, helping you confirm that you've correctly linked your remote repository.
Renaming and Deleting a Remote
If you need to rename a remote, you can use the following command:
git remote rename origin upstream
In this case, you’re changing the name from origin to upstream. This can be useful when you want to differentiate between multiple remotes.
To remove a remote from your project, use:
git remote remove origin
This command deletes the connection to the remote specified.
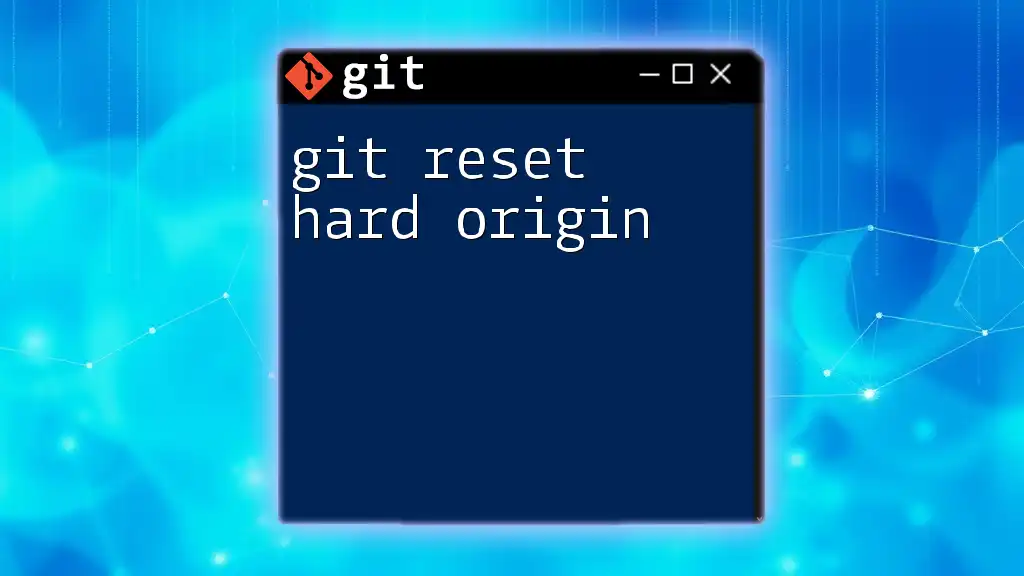
Fetching and Pushing Changes
Fetching from a Remote
Fetching allows you to retrieve changes made in the remote repository without merging them into your local repository immediately. To fetch changes from origin, you would use:
git fetch origin
When you run this command, Git retrieves the latest commits from the remote but does not incorporate them into your current branch. This is useful for reviewing changes before integrating them.
Pushing Local Changes to Remote
Once you’ve made changes locally, you want to push these changes back to the remote repository. The command for this is:
git push origin main
This command pushes the changes from your local `main` branch to the `main` branch in the origin remote. It’s crucial to understand that the name of the branch might differ based on your repository setup (e.g. master instead of main).
Understanding Push and Fetch vs. Pull
While fetching gets changes from the remote, pushing sends your local changes to the remote. In contrast, pull combines both operations—fetching and merging. To pull changes from origin, you can simply use:
git pull origin main
This command updates your local repository with the latest changes from the remote, integrating those updates in your working branch.
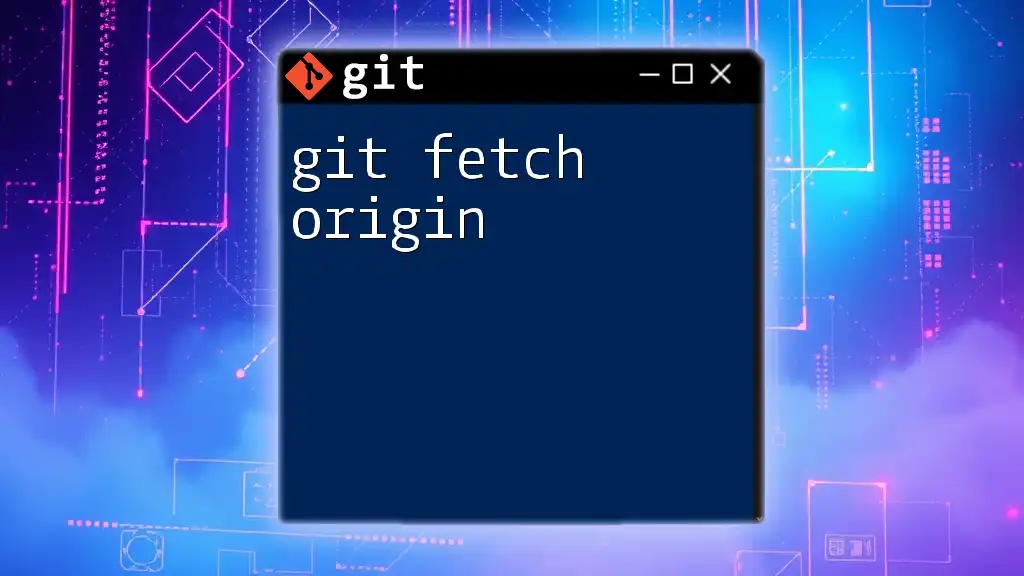
Collaborating with Teams
Using Branches with Remote Repositories
Branches play a vital role in managing feature development and fixes in collaborative environments. To create a new branch, switch to it, and push it to the origin, you can use:
git checkout -b new-feature
git push origin new-feature
The first command creates and switches to the `new-feature` branch, while the second command pushes that branch to the remote repository.
Pull Requests and Merging
When collaborating, a common practice is to open a pull request to notify team members about new changes and request a review. This process involves merging feature branches into the main branch of the remote repository, thus promoting code review and quality assurance.
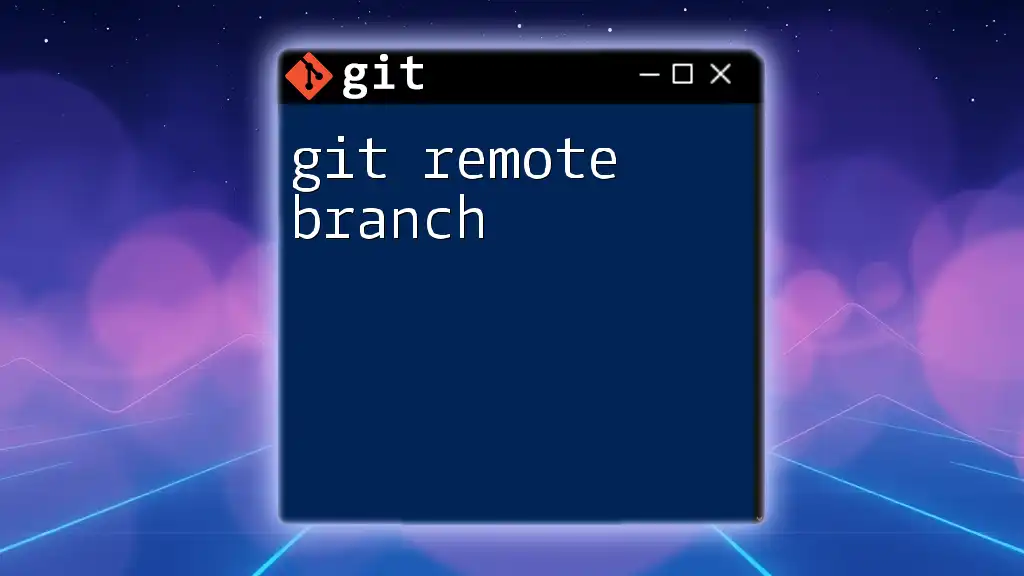
Troubleshooting Common Issues
Remote Connection Errors
Sometimes, you may encounter issues when your local Git tries to connect to a remote repository. Common errors include:
- Authentication errors can stem from incorrect credentials or SSH key issues.
- Network errors may arise from internet connectivity issues or firewall restrictions.
In such cases, double-check your repository URL and network settings.
Conflict Resolution
Conflicts can happen after you perform a `git pull` when multiple modifications to the same lines of code exist between your local and remote branches. To handle conflicts, follow these steps:
- Run `git status` to identify conflicted files.
- Open the conflicted files using your text editor, and look for the conflict markers (<<<<<<<, =======, >>>>>>>).
- Resolve the conflicts manually by editing the files.
- Once resolved, stage the changes with:
git add <file-name>
- Complete the merge by completing the commit:
git commit
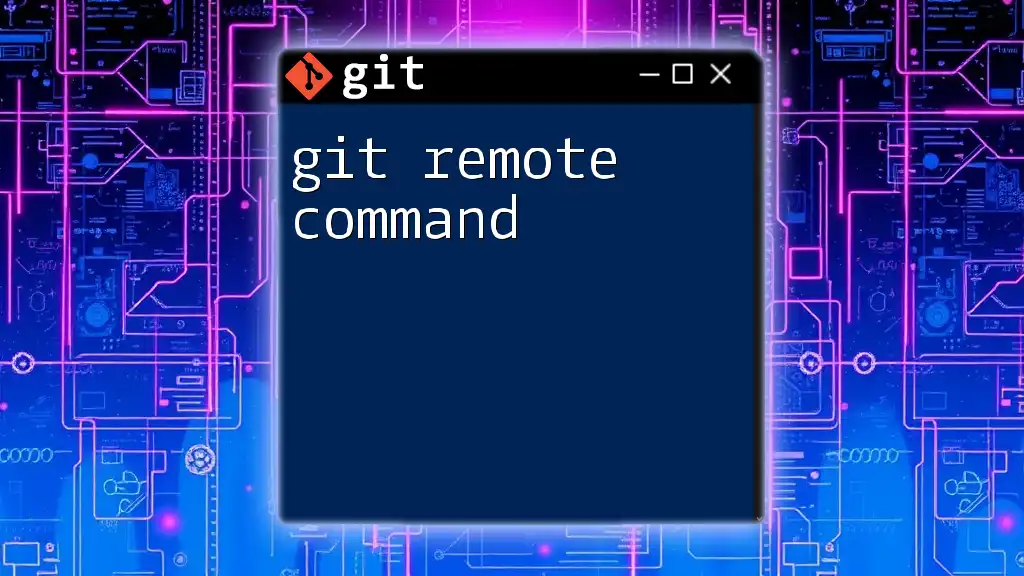
Best Practices for Using Git Remotes
Regularly Updating Remote References
It's essential to keep your local repository updated with remote changes. Regularly using `git fetch` is a good practice, as it allows you to stay informed about updates without immediately merging them into your branches.
Using SSH vs. HTTPS for Remote URLs
When linking repositories, you have two main protocols to choose from: SSH and HTTPS.
- SSH is generally more secure and allows for easier credential management once set up.
- HTTPS is user-friendly and works well without additional configuration but usually requires credentials for each push.
Many teams opt for SSH for regular collaborations due to its efficiency, especially in larger projects.
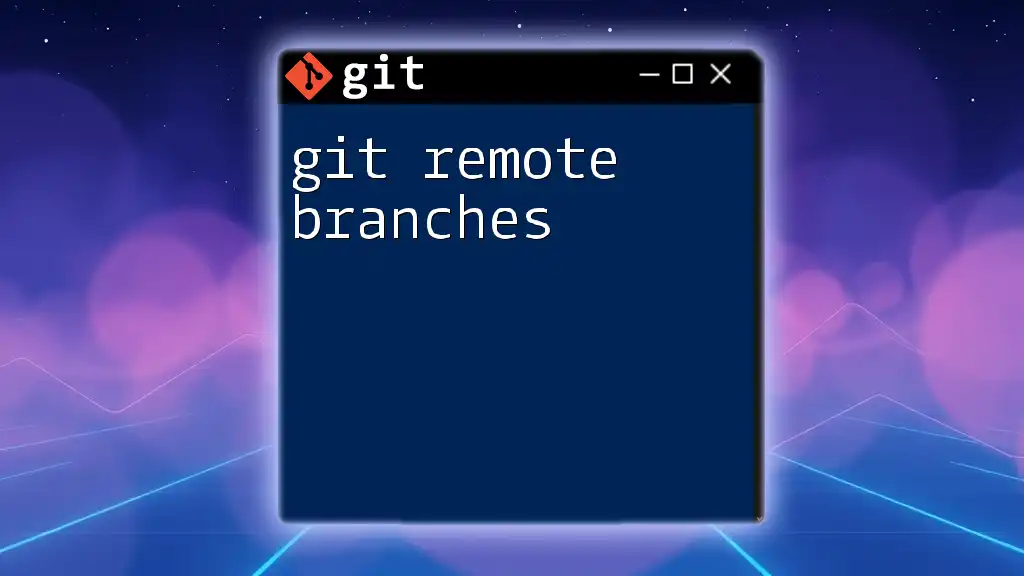
Conclusion
In this guide, we examined the essentials of git remote and origin. Understanding how to set up and manage remotes lays the foundation for successful collaborative software development. Whether you are pushing changes or addressing conflicts, knowing how to navigate remotes is invaluable in today's software development landscape.
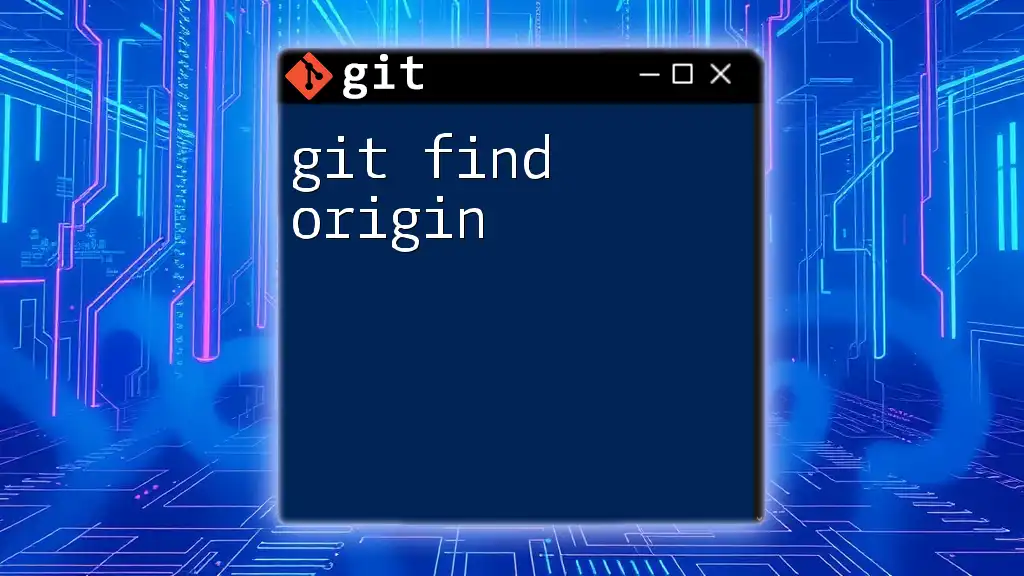
Additional Resources
For further learning, consider exploring the official Git documentation and various online tutorials that dive deeper into Git practices and collaboration techniques. Engaging with the Git community can also enhance your understanding and skills in using Git effectively.