The command `git reset --hard origin` is used to reset your current branch to match the state of the remote branch named "origin," discarding any local changes and commits.
git reset --hard origin/your-branch-name
The Basics of Git Reset
What is `git reset`?
The `git reset` command is a powerful tool used to reset your current HEAD to a specified state. It is essential for managing your commits and the state of your working directory. Git reset comes in three primary modes:
-
Soft Reset: Moves the HEAD pointer to a previous commit while leaving your working directory unchanged. This means your changes remain staged in the index.
-
Mixed Reset: This is the default mode if no options are specified. It also moves the HEAD pointer but resets the index, leaving your working directory intact. Unsaved changes remain in your files but are unstaged.
-
Hard Reset: This mode resets the HEAD pointer, index, and working directory to a specified state, discarding all changes. It is the most destructive option, as uncommitted changes are permanently lost.
Understanding the Origins
In Git, "origin" refers to the default remote repository where your code is typically hosted. It is a common convention to name the main remote repository as "origin." Understanding the role of this remote repository is crucial because it serves as the reference point for your team's work.
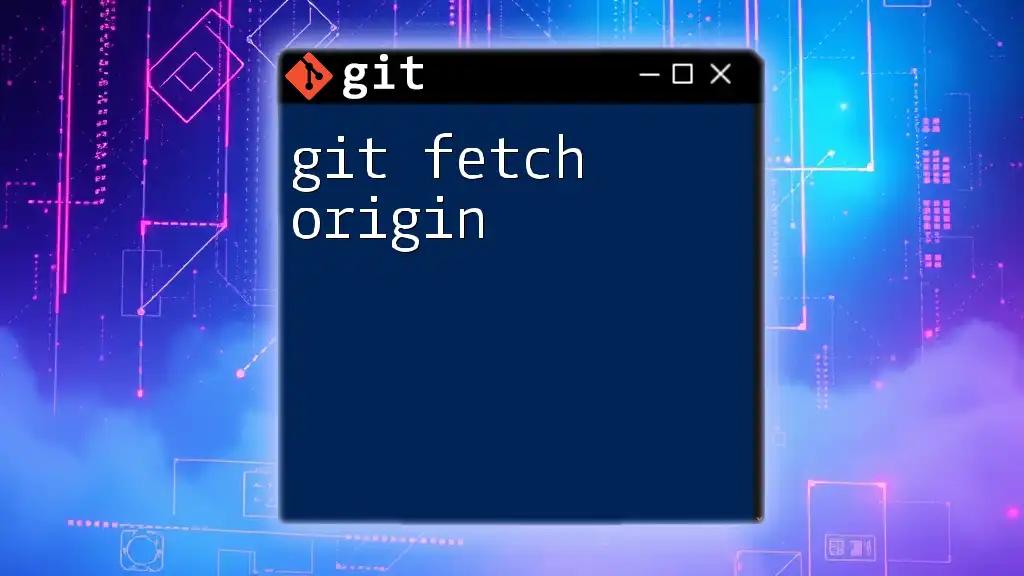
The Command: `git reset --hard origin`
What Does `git reset --hard origin` Do?
The command `git reset --hard origin` is used to forcefully reset your local branch to match the state of a branch in the remote repository, effectively discarding any local changes.
This command is particularly useful when you want to abandon uncommitted changes and revert your local branch to the last committed state from the remote branch.
When to Use `git reset --hard origin`
Utilizing `git reset --hard origin` can be beneficial in specific scenarios, such as:
- After making experimental changes that you wish to discard entirely.
- When you want to ensure your local branch aligns perfectly with the remote, particularly after pulling changes and encountering conflicts.
- Previously committed changes that you no longer want to keep can be removed with this command.
It's important to note the risks involved. Any uncommitted changes will be lost irrevocably, so it is crucial to be certain that you won't need the discarded changes.
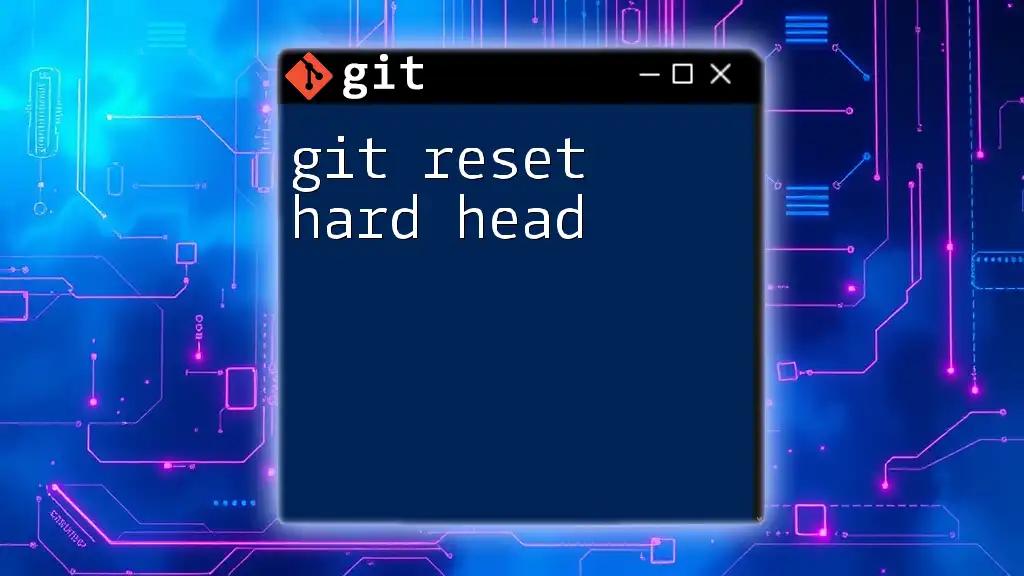
Detailed Breakdown of the Command
Syntax of the Command
The command follows this general syntax:
git reset --hard origin [branch_name]
Each component serves a unique function:
- `git reset`: The command itself, which will change the state of your current HEAD.
- `--hard`: Specifies the type of reset, indicating that both the index and working directory will also be reset.
- `origin`: Refers to the remote repository.
- `[branch_name]`: The specific branch on the remote that you want to reset to.
Example of Using `git reset --hard origin`
Consider a situation where you're working on a project and have made several local changes that you no longer wish to keep. You also realize that your team has pushed a new set of changes to the `main` branch in the remote repository.
To discard your local changes and align your branch with the remote `main`, you can execute:
git fetch origin
git reset --hard origin/main
In this case, `git fetch origin` ensures that you have the latest updates from the remote repository. Afterward, `git reset --hard origin/main` will set your local branch to be exactly like the `main` branch on the origin, losing all your prior modifications.
Understanding Impact on Your Local Repository
When you execute `git reset --hard origin`, the following occurs:
- Working Directory: Your working directory is reverted to match the state of the specified branch from the remote, removing any changes you made.
- Index: The index, which staged changes, is also cleared, meaning you’ll need to re-add any files that you want to keep or push again.
This resets the state of your project to a clean slate matching the remote branch, which is key for maintaining order and preventing conflicts when collaborating with team members.
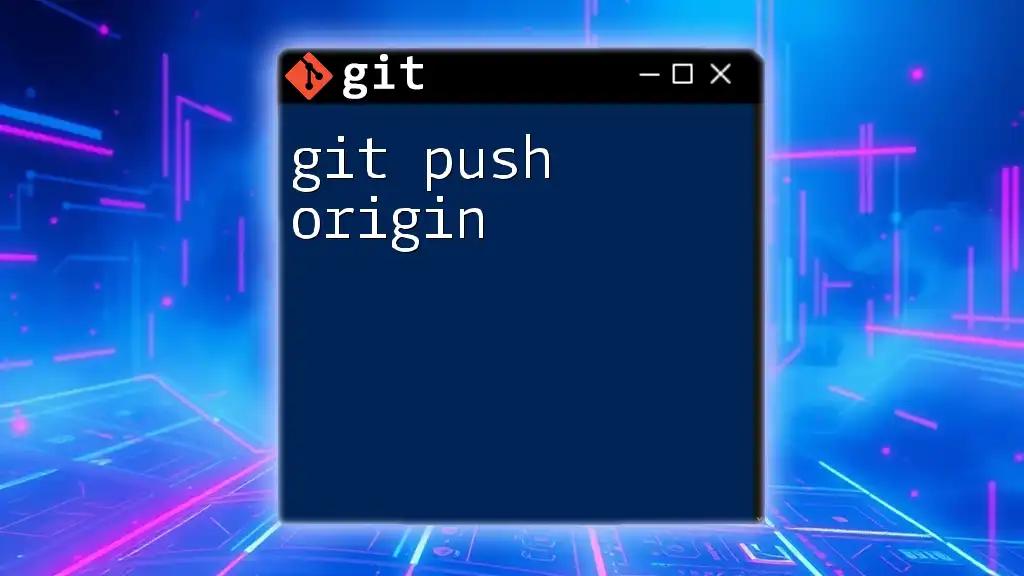
Common Mistakes and How to Avoid Them
Misunderstanding the Hard Reset
One common pitfall is misunderstanding what a hard reset means. Expecting the command to only discard uncommitted changes while retaining the committed changes can lead to frustration. Ensure you fully grasp what "hard" entails before executing this command.
Avoiding Data Loss
Before executing `git reset --hard origin`, always consider:
- Backing Up Changes: If you're unsure about losing changes, create a new branch to store your current state:
git checkout -b backup-branch
- Stashing Changes: To temporarily save your work without committing, stash your changes with:
git stash
This way, you're protected from losing important modifications in case the hard reset doesn't go as planned.
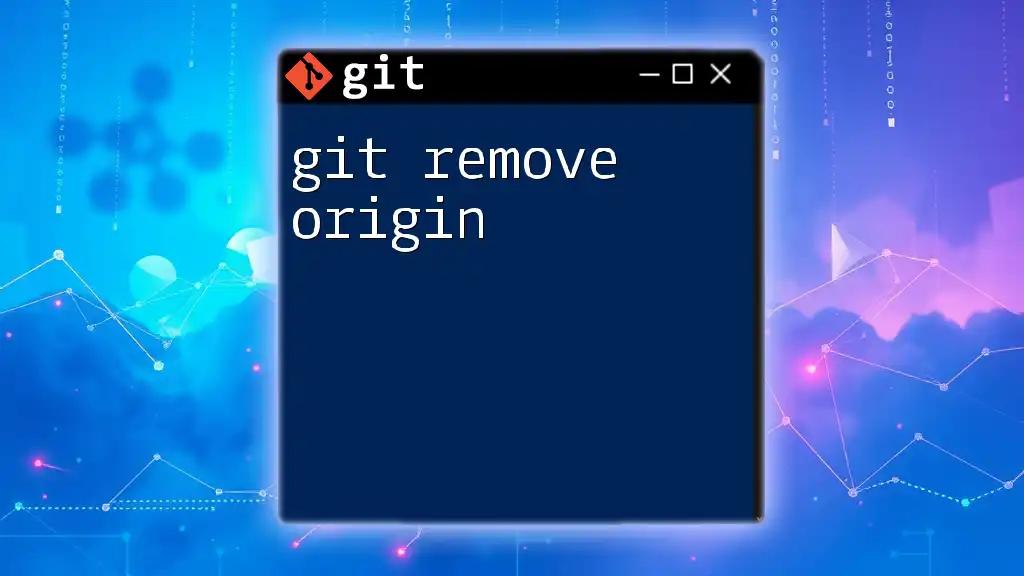
Alternatives to `git reset --hard origin`
Using `git checkout`
If your goal is simply to view or change to a specific commit or branch without affecting your current work, you can consider using `git checkout`. This method allows you to explore the repository’s history without the destructive effects of a hard reset:
git checkout origin/main
Using `git revert`
If you prefer to keep the history intact and avoid losing changes permanently, `git revert` can be a suitable alternative. This command creates a new commit that undoes changes from a specified commit. This is particularly useful for teams collaborating on shared branches:
git revert HEAD
This way, changes can be reversed without permanently removing the history, allowing for safer collaboration.
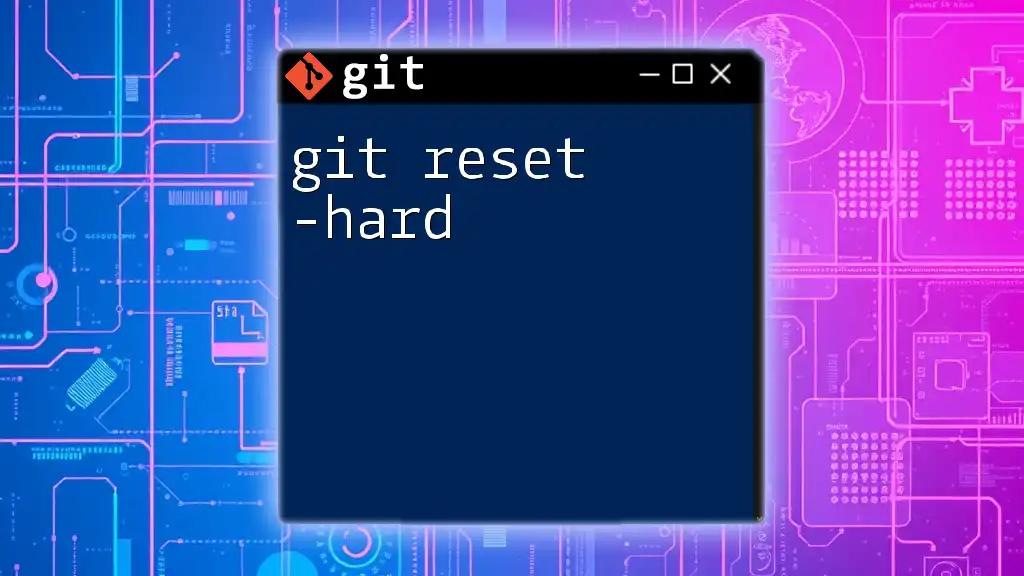
Conclusion
Understanding `git reset --hard origin` is crucial for navigating version control effectively. This command is an essential tool for maintaining synchronization with remote repositories while ensuring all local changes can be completely discarded when necessary.
Always remember the implications of this command and consider safer alternatives when applicable. By mastering this command and handling it with caution, you can leverage Git's capabilities in your development process, ultimately contributing to a more efficient and collaborative workflow.
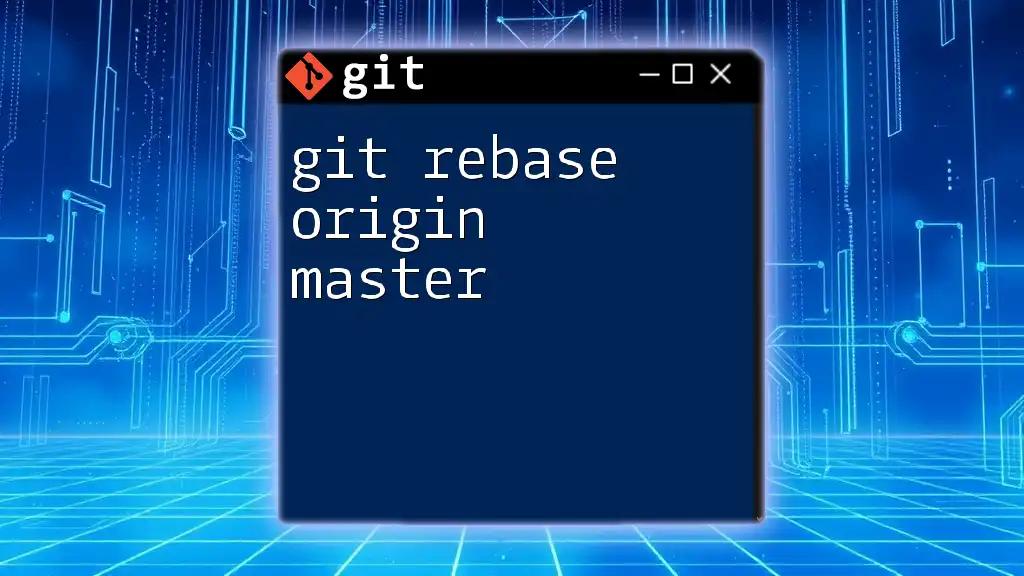
Additional Resources
For further exploration of Git commands, consider diving into the official Git documentation or seeking out tutorials that cover advanced Git techniques. Engaging with community forums can also provide valuable insights and troubleshooting support.