The `git reset` command allows you to reset your current branch to a specific commit, and you can use it with the `--hard` option to discard all local changes and align your branch with the remote repository.
Here's how you can reset your branch to match the remote branch:
git fetch origin
git reset --hard origin/main
What is `git reset`?
The `git reset` command is a powerful tool in Git that allows you to undo your local changes by resetting your current HEAD to a specified state. It has three main modes, each serving a unique purpose:
- Soft: Resets the HEAD to a previous commit but keeps changes in the staging area, allowing you to re-commit changes quickly.
- Mixed: Resets the HEAD and the index but keeps working directory changes. This is the default mode and is useful for unstaging files.
- Hard: Resets everything (HEAD, index, and working directory) to a specified state, effectively losing all unsaved changes.
Understanding `git reset` is crucial for managing your project history and collaborating effectively in team environments.
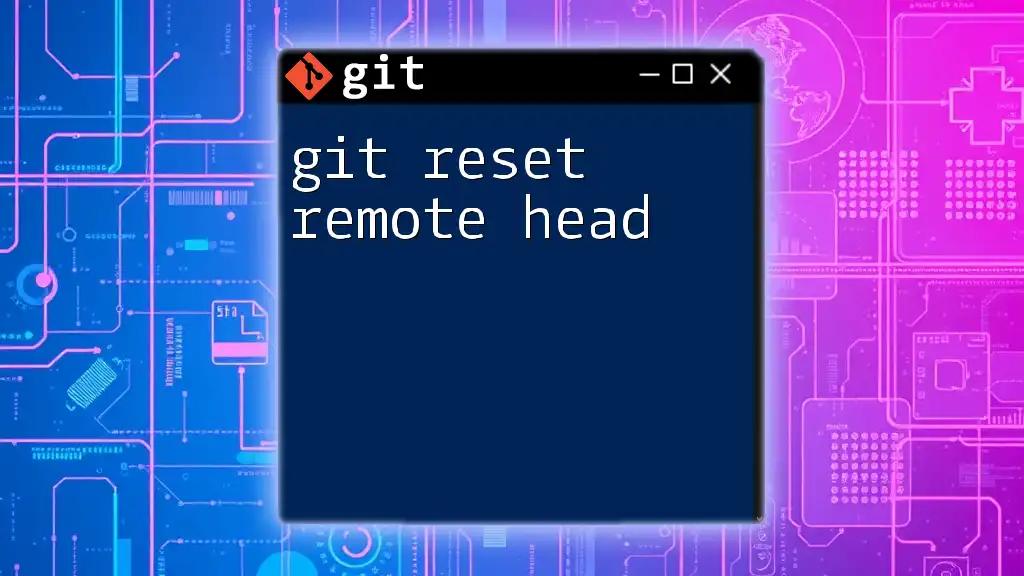
Understanding Remote Repositories
In Git, a remote repository is a version of your project that is hosted on the internet or a network. Common scenarios for using remote repositories include collaboration with team members or deploying code to production servers.
Different commands interact with remote repositories, and knowing how to use them is essential:
- `git fetch`: Retrieves updates from a remote repo without merging them.
- `git pull`: Retrieves and merges updates in one command.
- `git push`: Uploads local changes to the remote repository.
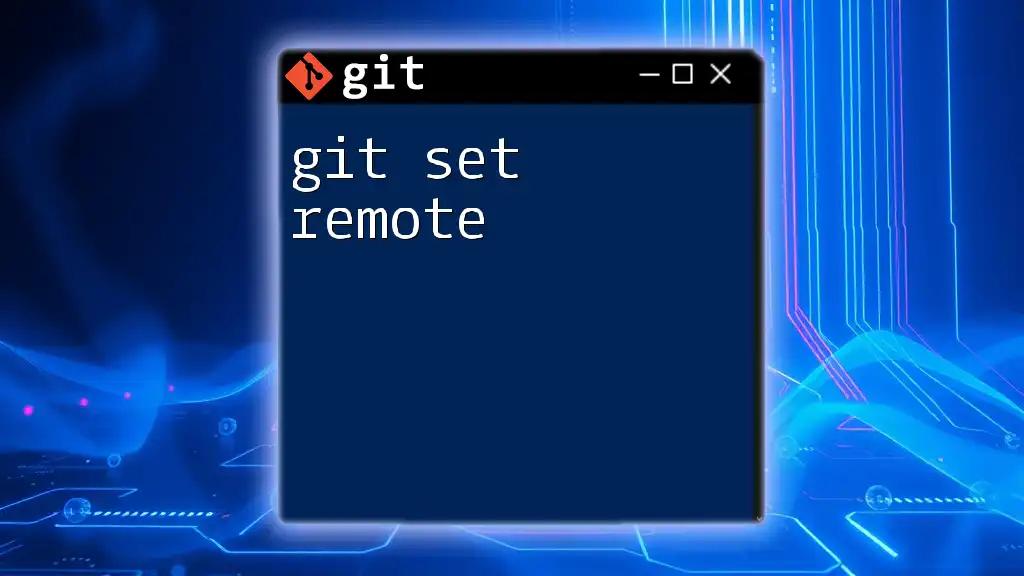
The Role of `git reset` with Remote Repositories
Resetting a remote repository can sometimes be necessary, especially after introducing problematic changes or commits. There are several scenarios where resetting a remote using `git reset` becomes relevant:
- You may want to remove erroneous commits that accidentally made their way to the remote repository.
- Alternatively, you may need to revert to an earlier state after realizing that recent changes have negatively impacted your project. Understanding the implications of using `git reset` on remote branches is crucial, as it can significantly affect collaborators who rely on the same branch history.
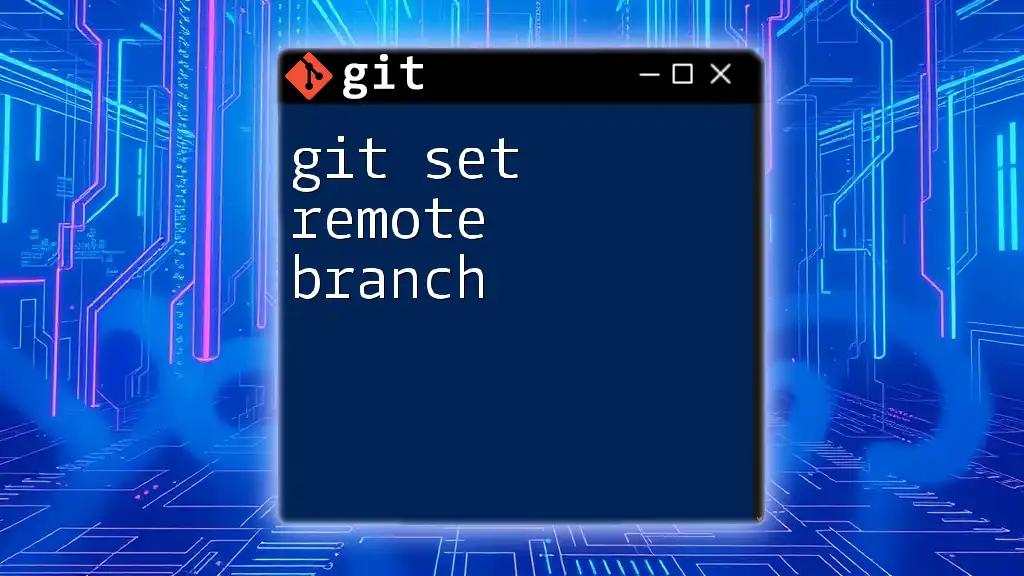
How to Perform a `git reset remote`
Before executing a `git reset remote`, make sure you're prepared.
Prerequisites
Ensure you have Git installed and properly set up with your repository cloned. It's a good practice to communicate with your team before performing a reset. Confirm that any important changes have been committed and that everyone is aware of the action to avoid confusion.
Common Scenarios
Resetting to a Specific Commit
Sometimes you may need to revert to a stable commit before a problematic one was introduced. For example:
git reset --hard <commit_hash>
git push origin <branch_name> --force
In this command, the first line resets the local repository to the specified commit, while the second line forces the update to the remote, effectively removing any commits made after the specified commit.
Undoing Multiple Commits
If the issue requires you to undo several recent commits, you can do so in one swift command. For example, to remove the last three commits:
git reset --hard HEAD~3
git push origin <branch_name> --force
This command resets the HEAD to three commits back and forces the update to the remote branch.
Using Tags with `git reset`
Tags are valuable for marking specific points in Git history (e.g., releases).
Tagging a Commit
Before resetting, consider tagging the commit for reference:
git tag -a <tag_name> -m "Tagging the commit for future reference"
git push origin <tag_name>
This way, you'll always have a marker for the reset point, providing a reference for your team or future work.
Resetting to a Tag
If you need to revert to a specific version tagged as a stable release:
git reset --hard <tag_name>
git push origin <branch_name> --force
Similar to previous examples, this command effectively resets your branch to the snapshot captured by the tag.
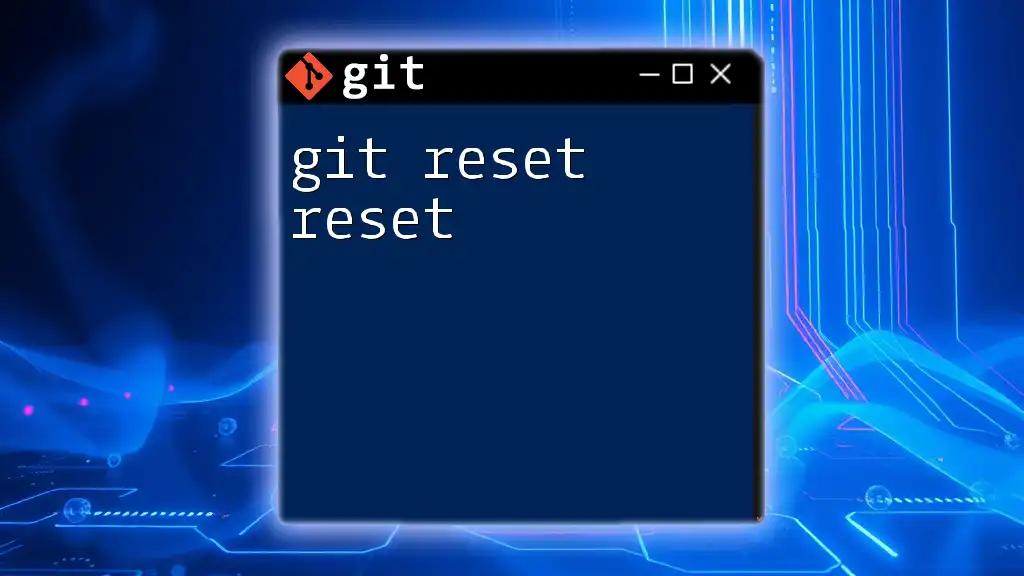
Consequences of Using `git reset remote`
Losing Data
Data loss is a recurring theme when using `git reset`, especially in hard mode. When you reset, you're effectively discarding changes that haven't been committed. It’s imperative to have backups and to communicate with your team before proceeding with a reset to avoid unexpected consequences.
Collaboration Impact
Resetting a remote branch can significantly impact your collaborators' workflow. They'll find that their branch history has changed, which could affect their local work. It’s best practice to communicate clearly about any resets and potentially adopt mechanisms to prevent disruptions.
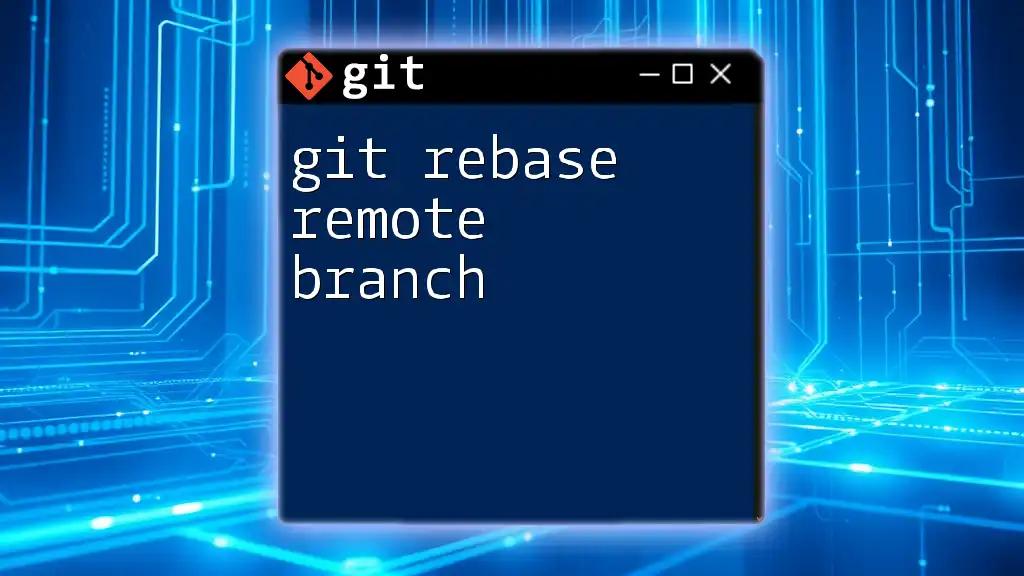
Alternatives to `git reset`
Safer Commands
Instead of using `git reset`, consider safer alternatives like `git revert`. This command undoes changes by creating new commits that negate prior changes, which is less disruptive for collaborators. For example:
git revert <commit_hash>
git push origin <branch_name>
By using `git revert`, you maintain the history of all previous commits while effectively undoing the unwanted changes.
Using Branches
Another strategy is to create separate branches for testing or experimental features. Instead of resetting main branches frequently, create a feature branch and experiment there:
git checkout -b <feature_branch>
This way, your main branch remains stable while you explore new changes.
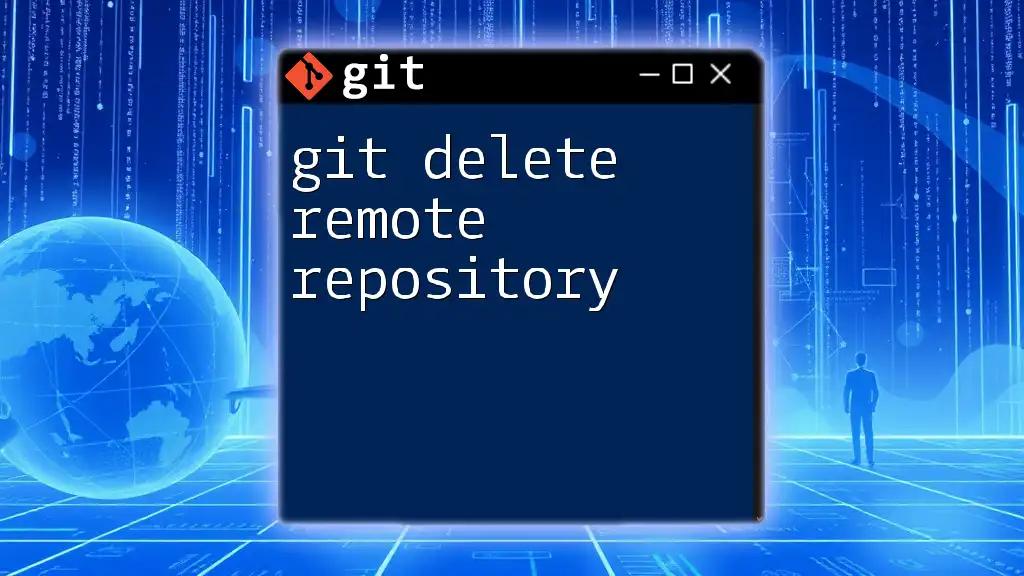
Conclusion
Having a solid grasp of how to execute a `git reset remote` is fundamental for effective version control management. While powerful, the command should be used judiciously and with a thorough understanding of its consequences. Emphasizing communication and proper strategy when collaborating will lead to smoother project workflows and fewer disruptions.
Additional Resources
To deepen your understanding, explore the official Git documentation and leverage tools designed for Git operations. Continuous learning will help solidify your skills and ensure effective collaboration.