A Git reference is a pointer to a commit, tag, or branch in a Git repository, allowing you to track and manage your project's history more effectively.
git checkout <branch-name>
Understanding Git Basics
What is Version Control?
Version control is a system that records changes to a file or set of files over time, allowing users to recall specific versions later. It is essential for software development because it enables collaboration among multiple developers, tracks changes, and helps revert to previous states if necessary. Using version control, teams can work on separate features without interfering with each other's progress.
Getting Started with Git
To start using Git, first, you need to install it on your machine:
Installing Git:
- For Windows, you can download and run the installer from the official Git website.
- On macOS, you can install it using Homebrew with the command:
brew install git
- For Linux users, use your package manager to install Git:
sudo apt-get install git # For Debian-based systems
sudo yum install git # For Red Hat-based systems
After installation, check if it was successful:
git --version
Basic Configuration: Before diving deeper, a few configurations are necessary. Set your global username and email so Git can associate your commits with your identity:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
You can check your configuration with:
git config --list
Understand that these configurations are saved in the `.gitconfig` file located in your home directory.
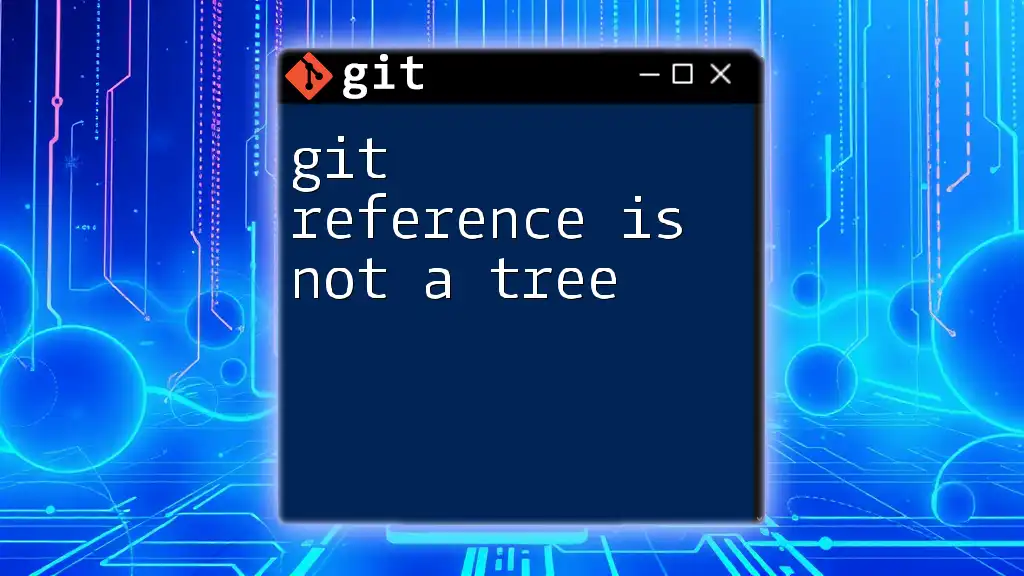
Core Git Concepts
Git Repositories
At the heart of Git is the repository (repo), a directory that contains all your project's files and version history. There are two types of repositories in Git:
- Local Repository: Located on your machine.
- Remote Repository: A version of your project hosted on a platform like GitHub or GitLab.
Creating a New Repository: You can create a new local repository by navigating to your project’s directory and running:
git init my-repo
This command creates a new folder named `my-repo` and initializes a new Git repository in it.
Commits
In Git, a commit is like a snapshot of your project at a particular time. It allows you to save your changes and provides a history of modifications.
Making Your First Commit: To make a commit, you first need to stage your changes:
git add .
This command stages all your changes. After staging, commit these changes with a descriptive message:
git commit -m "Initial commit"
By doing this, you create a point in your project’s history that you can revert to if necessary.
Viewing Commit History: To see the history of your commits, use:
git log
This command lists all commits with details such as the commit hash, author, date, and the commit message.
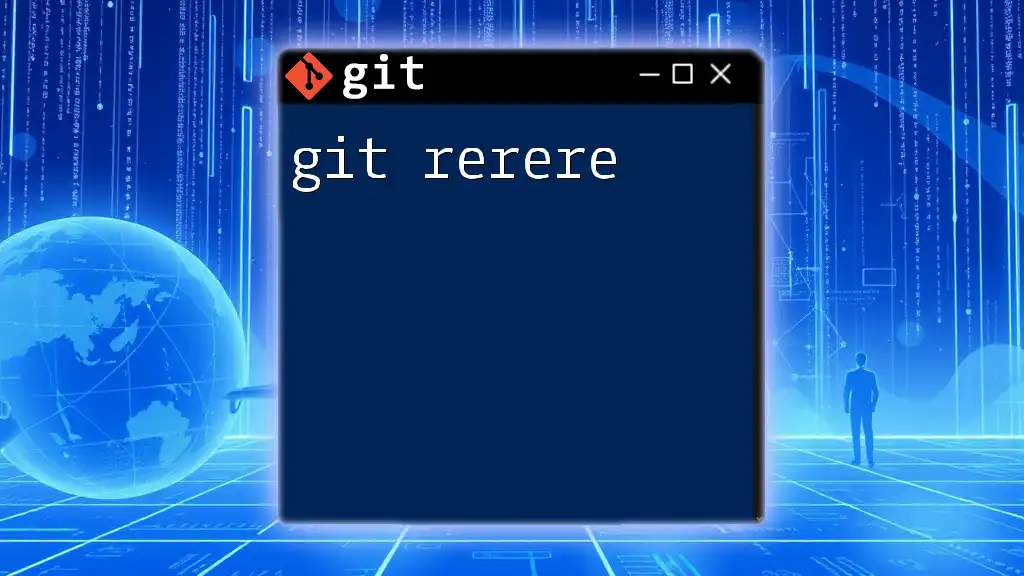
Essential Git Commands
Working with Files
Staging Changes: Understanding the difference between staging and committing is crucial. You stage changes with:
git add filename.txt
or for all changes:
git add .
After staging, you then commit the staged changes to your repository.
Undoing Changes: If you made a mistake and wish to discard changes, you can revert a file to its last committed state using:
git checkout -- filename.txt
This action restores the file to how it was in the last commit, discarding any uncommitted changes.
Deleting Files: To remove files from both the staging area and working directory, use:
git rm filename.txt
This command not only untracks the file but also deletes it from your local folder.
Branching and Merging
What is a Branch? A branch in Git is used to develop features or fix bugs independently without affecting the main project. By default, your repository contains a branch named `main` or `master`.
Creating and Switching Branches: To create a new branch, use:
git branch new-branch
Switch to the new branch with:
git checkout new-branch
This command allows you to work in isolation on your changes.
Merging Branches: Once your work on a branch is complete, you can merge it back into the main branch:
git checkout main
git merge new-branch
If there are conflicting changes, Git will prompt you to resolve them before completing the merge.
Remote Repositories
Working with Remotes: Adding a remote repository allows you to store your code in a centralized location. You can add a remote with:
git remote add origin https://github.com/user/repo.git
This command links your local repository to a remote one specified by the URL.
Pushing Changes: After making local commits, you can share these changes by pushing to the remote repository:
git push origin main
This command uploads your local commits to the specified remote branch.
Pulling Changes: When collaborating, you might need to incorporate changes made by others. To fetch and merge changes from the remote repository, use:
git pull origin main
This action updates your local repository with the latest version from the remote.
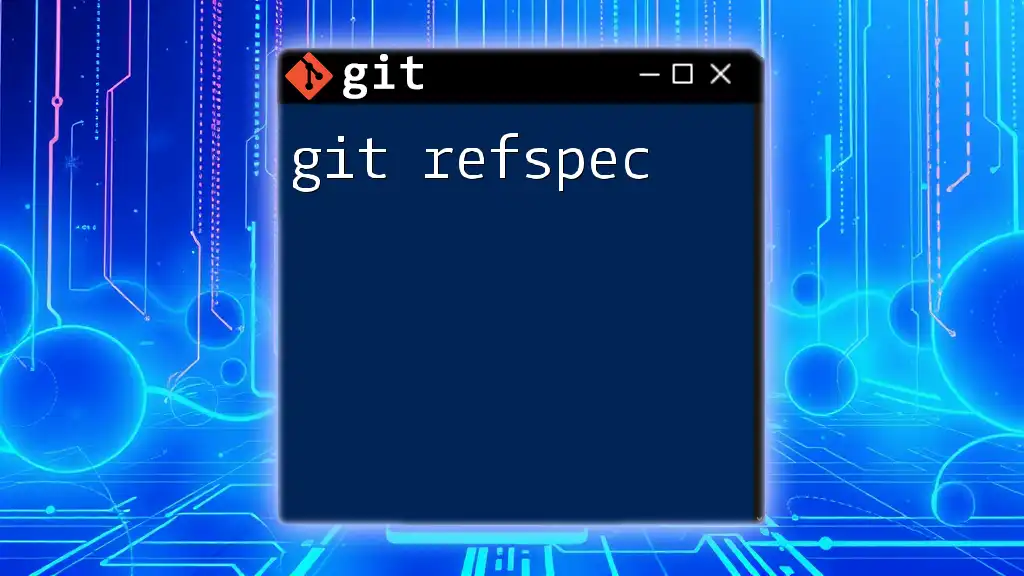
Advanced Git Commands
Cherry-Picking
What is Cherry-Picking? Cherry-picking allows you to apply commits from one branch to another selectively, which can be useful for applying specific features or fixes without merging entire branches. To cherry-pick a commit, use:
git cherry-pick <commit-hash>
This command takes the specified commit from another branch and applies it to your current branch.
Rebasing
Understanding Rebasing vs. Merging: Rebasing brings your changes to the base of another branch, which allows for a cleaner project history compared to merging. You can rebase your current branch onto the main one with:
git rebase main
Always ensure your work is committed before using rebase, as unresolved conflicts may arise.
Stashing Changes
What is Stashing? If you need to switch branches but don't want to commit unfinished work, stashing allows you to save changes temporarily. To stash your changes, run:
git stash
You can apply the stashed changes later using:
git stash apply
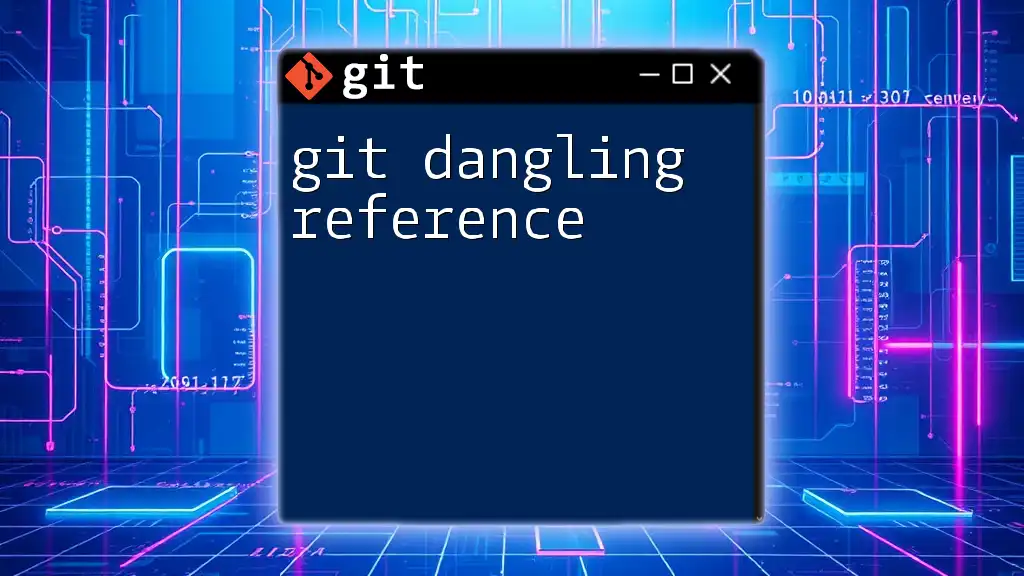
Common Git Workflows
Feature Branch Workflow
The feature branch workflow is a popular approach that encourages developers to create a new branch for each feature or bug fix. This keeps the main branch clean and stable. After developing and testing on the feature branch, it can be merged back into the main branch following a code review process.
Gitflow Workflow
The Gitflow workflow formalizes the branching model with specific roles for branches (e.g., `develop`, `release`, `hotfix`). In Gitflow, you maintain a strict branching structure, facilitating easier feature additions and bug fixes while ensuring the stability of the main project.
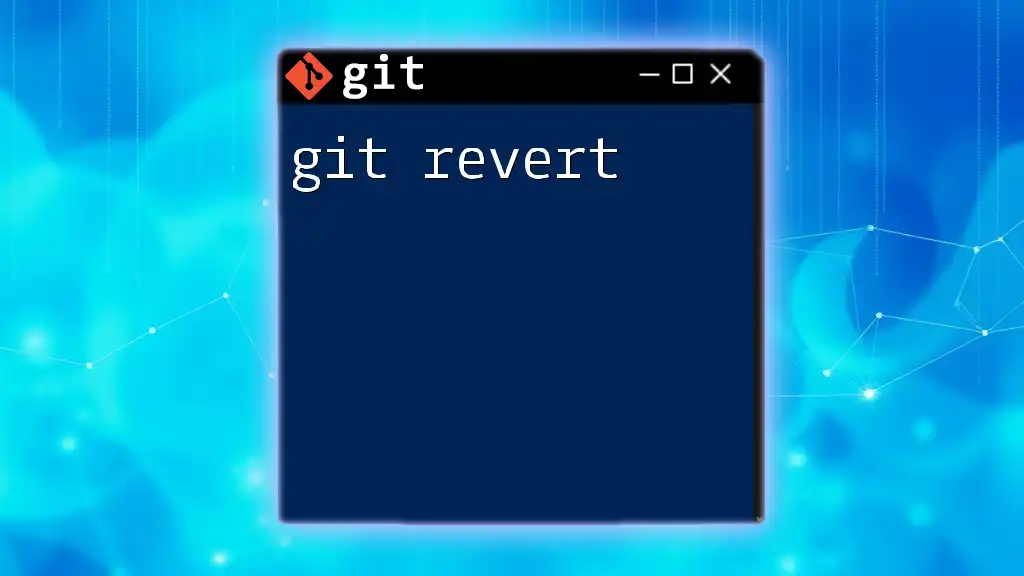
Troubleshooting Common Issues
Resolving Merge Conflicts
When multiple branches modify the same part of a file, Git cannot automatically merge these changes, resulting in a merge conflict. To resolve this:
- Open the conflicted file(s).
- Identify the conflict markers (`<<<<<`, `=====`, `>>>>>`).
- Decide which changes to keep and delete the markers accordingly.
- Stage the resolved files.
- Complete the merge.
Incorrect Commits
If you make a commit but realize it was a mistake, you can amend the last commit with:
git commit --amend
This command allows you to modify the message or contents of the previous commit. However, be cautious when amending commits that have already been pushed to a remote repository, as this can lead to complications for others relying on the original commit.
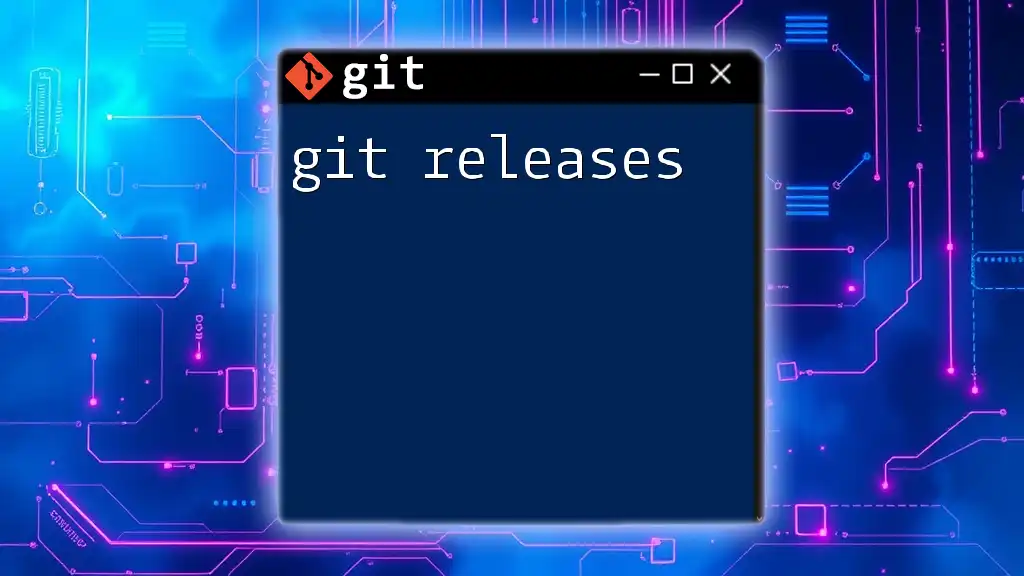
Conclusion
In summary, having a git reference to guide you through Git commands and concepts is invaluable for both beginners and experienced developers. The commands and workflows outlined in this article offer a reliable foundation for using Git effectively. The key to mastering Git is practice; as you become familiar with the commands, you'll gain confidence and proficiency in version control. Embrace Git, and you’ll enhance not only your development skills but also your ability to collaborate on projects efficiently.
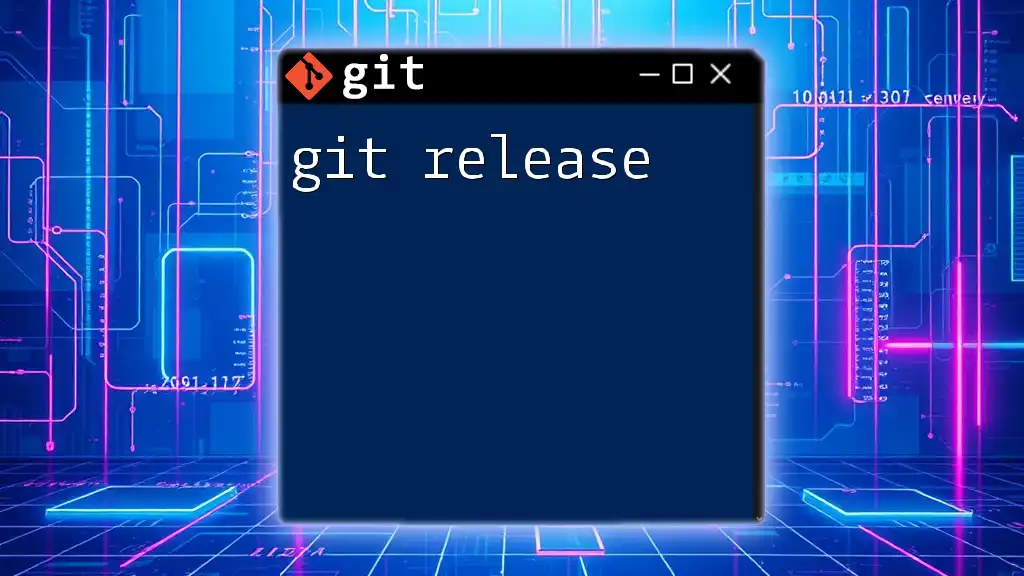
Additional Resources
For further reading and deeper understanding, consider exploring the official [Git documentation](https://git-scm.com/doc) or utilizing various online tutorials and courses. Engaging with community forums can also provide insights and solutions to specific Git challenges you might encounter.