The `git revert -m` command is used to revert a merge commit by specifying the parent number you want to keep, effectively creating a new commit that undoes the changes introduced by the merge while preserving the history.
git revert -m 1 <merge_commit_sha>
Understanding `git revert`
What is `git revert`?
`git revert` is a powerful Git command used to create a new commit that undoes the changes made by a previous commit. Unlike `git reset`, which alters the commit history by removing commits from the history, `git revert` ensures that the history remains intact. This preservation of history is essential for collaborative environments where multiple developers are working on the same repository.
When to Use `git revert`
The `git revert` command is particularly useful when you need to undo changes from a commit that has already been shared with others. Use cases include:
- When a feature introduces bugs or instability and you need to revert it without deleting history.
- To backtrack on an unwanted change while keeping the original context intact.
Maintaining history provides clarity and transparency for all collaborators about changes made over time.
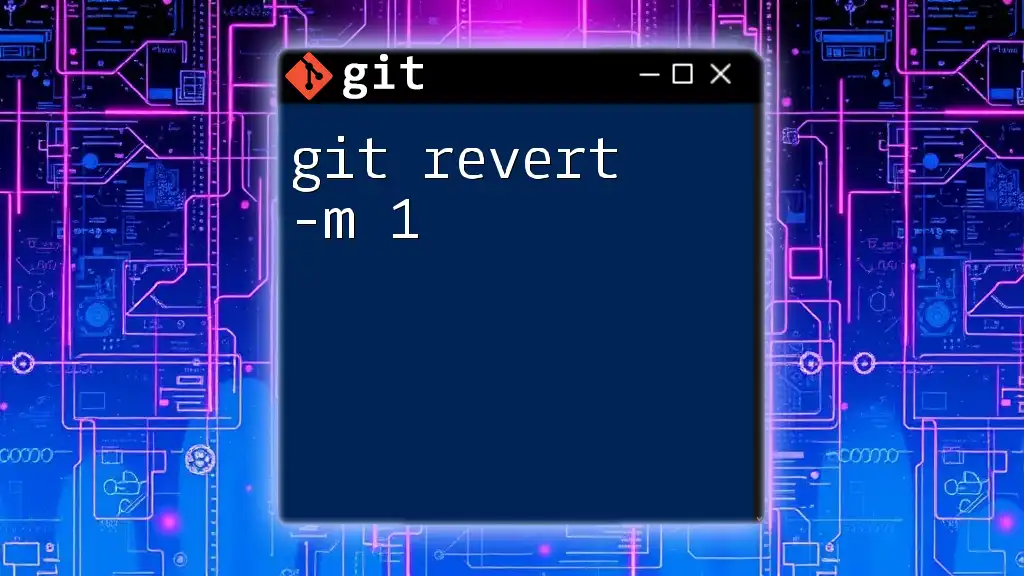
Introduction to Merge Commits
What is a Merge Commit?
A merge commit is created when two branches are combined, capturing the history of both branches. It typically has two parent commits: one from each branch involved in the merge. It allows Git to retain the complete history of all changes made.
Why Revert a Merge Commit?
There are times when a merge introduces code or changes that aren’t compatible with the ongoing development. In such cases, reverting a merge commit is necessary to roll back the integrated changes selectively while preserving the history of what was merged.
However, reverting a merge commit can be complex because it involves determining which parent’s changes you want to keep or revert.
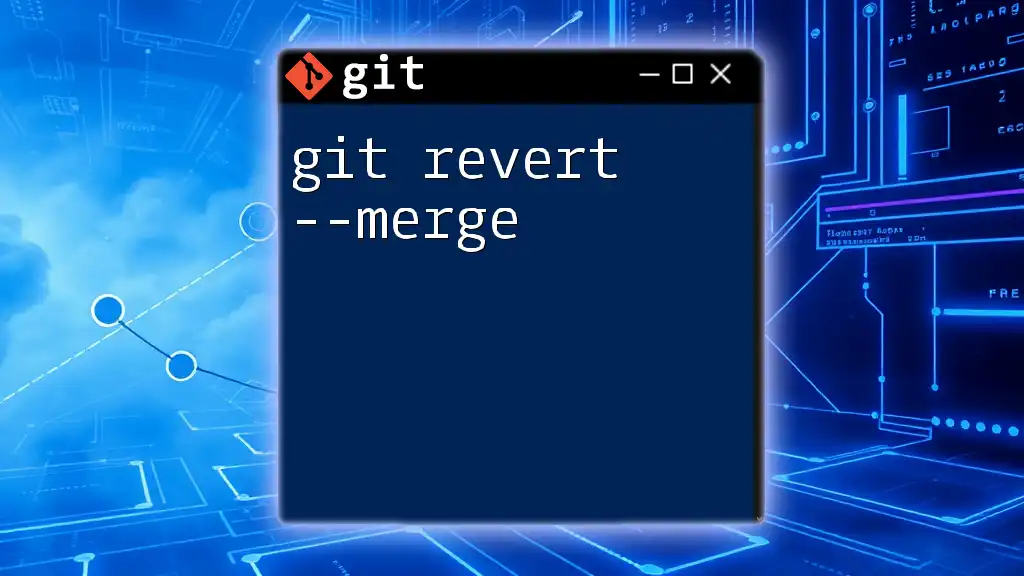
The `-m` Option Explained
What Does the `-m` Flag Do?
The `-m` option in the `git revert` command specifies which parent commit you want to keep. When reverting a merge commit, Git needs to know which preceding commit you want to revert back to. The two commit options in a merge commit are usually referred to as parent `1` and parent `2`.
Understanding Parent Commits
In Git terminology, the first parent commit (`-m 1`) refers to the branch that was merged into, while the second parent commit (`-m 2`) refers to the branch that was merged. Understanding which parent to select is critical to successfully reverting a merge.
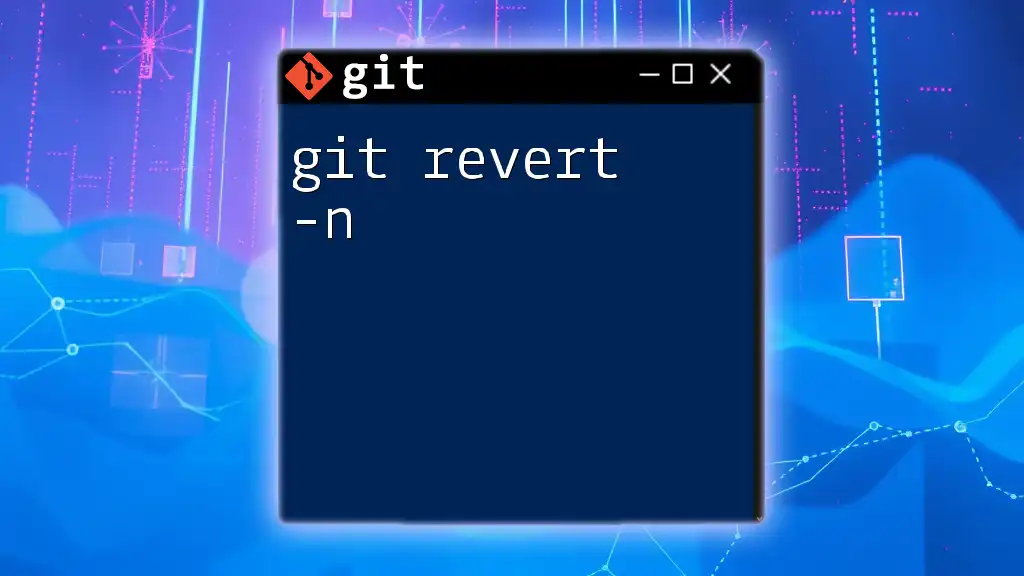
Practical Examples of Using `git revert -m`
Example Scenario
Imagine a scenario where your team merged a feature branch into the main branch, and it introduced breaking changes that need to be undone. The merge commit appears in the Git log and can be reverted.
Step-by-Step Revert Process
First, you’ll need to find the hash of the merge commit you want to revert. You can do this using:
git log --oneline
Once you have the merge commit hash, you can run the following command to revert it, assuming you want to keep changes from parent `1`:
git revert -m 1 <merge-commit-hash>
By executing this command, Git will create a new commit that reverts the effects of the specified merge commit. This command effectively retains the changes from the specified parent (in this case, parent `1`) while reversing the changes from the other parent.
Analyzing the Result
After running the revert command, it’s essential to confirm the integrity of your changes. You can check the status of your working directory with:
git status
This will show you the current state post-revert operation, allowing you to inspect the modifications.
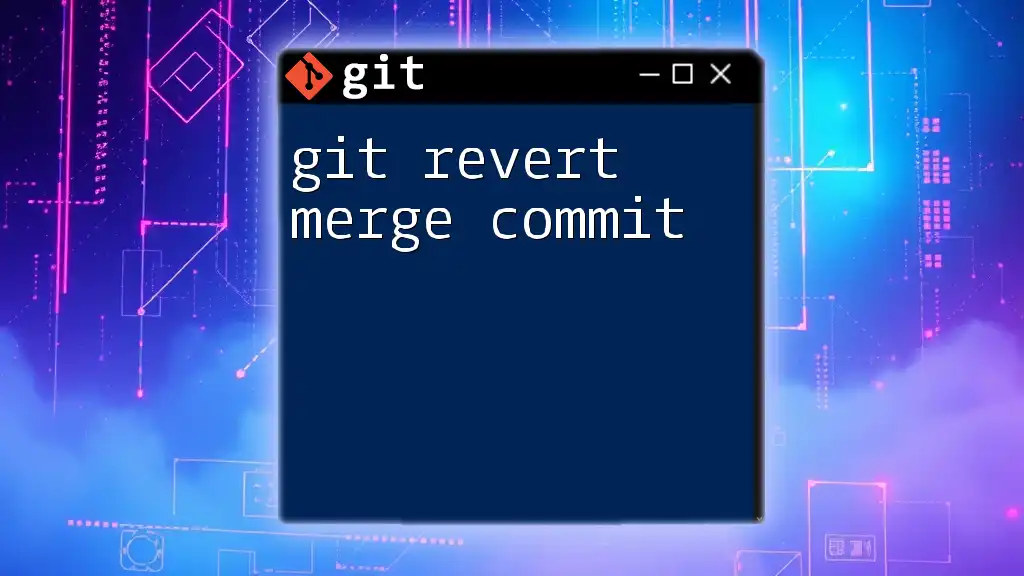
Handling Complications
Conflicts During Reversion
Conflicts are a possibility during a `git revert -m` operation, especially when the changes in the merge commit overlap with subsequent commits. If conflicts arise, Git will notify you, and you’ll need to manually resolve them before finalizing the revert.
Reverting a Revert
If you realize that reverting the merge commit was a mistake, you can reverse the revert. Using `git log`, find the revert commit hash and run:
git revert <revert-commit-hash>
Doing this will apply the changes from the reverted commit back into your working branch, effectively undoing the revert action.
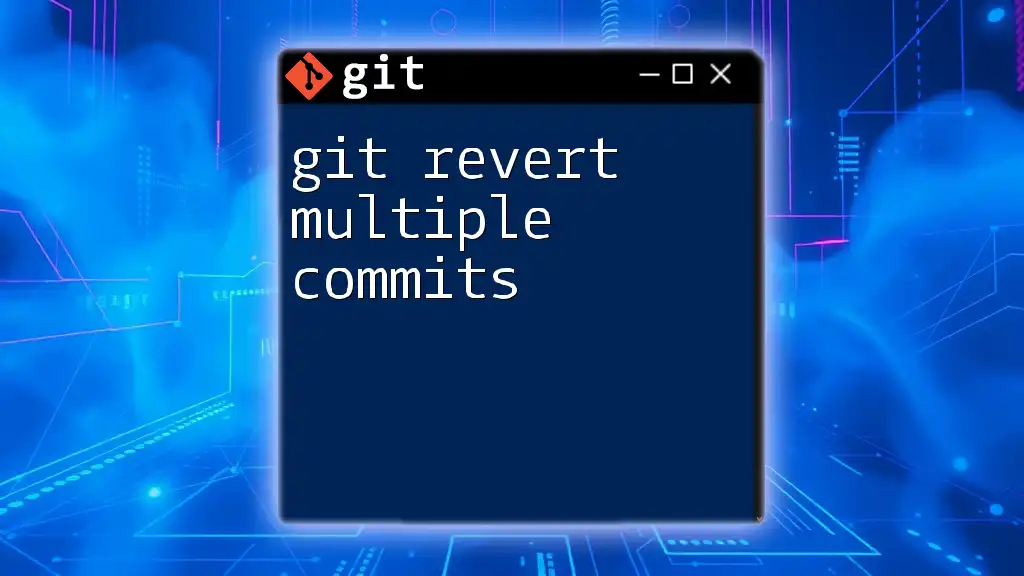
Best Practices for Using `git revert -m`
Always Test Before Reverting
Before executing a revert, it’s a good idea to ensure that no crucial features are negatively impacted. Running tests, examining the code, and discussing the changes with your team are effective strategies for avoiding unnecessary complications.
Keeping Commit Messages Clear
When creating a revert commit, ensure your commit messages are clear and informative. A well-documented commit message conveys why the revert was necessary, which is vital for team collaboration and future reference. For instance, documenting the reason for the revert in the commit message can greatly assist your teammates in understanding the decision.
Documentation and Communication
It’s essential to communicate with your team when performing a `git revert`, especially one on a merge commit. Clear communication can prevent confusion, ensure everyone is aligned, and facilitate collaborative efforts in managing the repository.
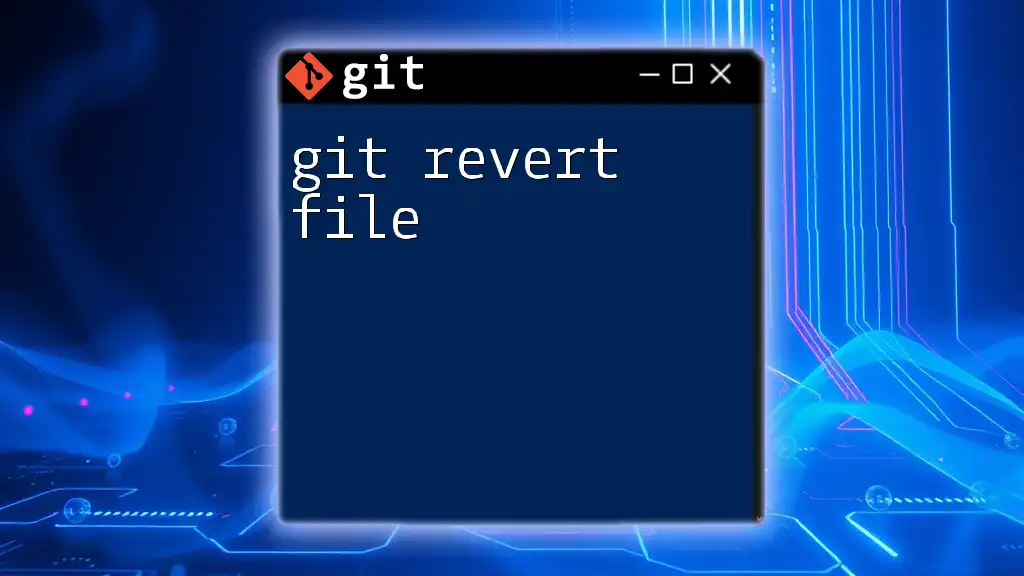
Conclusion
In summary, `git revert -m` is a crucial command for handling adjustments in a Git repository, especially in the context of merge commits. This guide provides insights into its functionality and best practices for effectively using this command. As a next step in your Git mastery, practice applying the concepts outlined here.
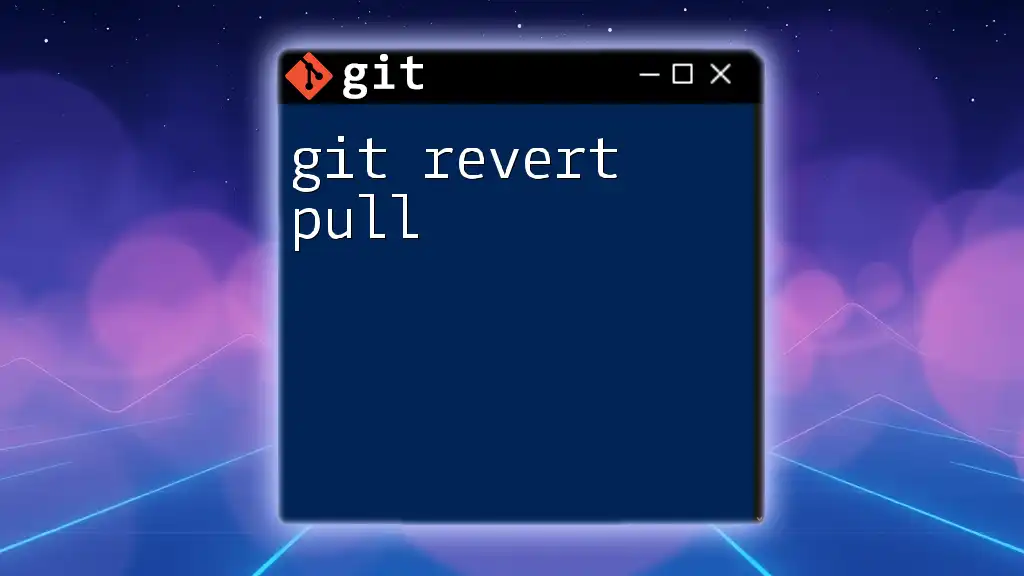
Frequently Asked Questions (FAQ)
What happens if I forget the `-m` option?
Forgetting the `-m` option when reverting a merge commit will result in an error, as Git requires you to specify which parent you want to keep.
Can `git revert` be used on any commit?
Yes, `git revert` can be used on any commit, including merge commits. However, it’s important to specify the correct parent using the `-m` option when reverting merges.
How does `git revert` differ from `git cherry-pick`?
While both commands help apply changes, `git revert` creates a new commit that undoes a previous commit, whereas `git cherry-pick` applies changes from a specific commit to your current branch.
Is it possible to revert without specifying a parent in a merge commit?
No, when reverting a merge commit, specifying a parent is mandatory since Git needs to know which changes to keep and which to discard.