To undo the effects of a specific merge commit in Git, you can use the `git revert` command with the `-m` option to specify the mainline parent you wish to keep.
git revert -m 1 <merge_commit_sha>
Understanding Merge Commits
What is a Merge Commit?
A merge commit is a special type of commit that occurs when two branches are combined into one. This commit has two (or more) parent commits, which allows Git to track the history of both branches that contributed to it. Merge commits are essential in collaborative projects where multiple developers work on various features simultaneously. They help preserve the history of changes while enabling seamless integration.
When Would You Need to Revert a Merge Commit?
While merging is a critical feature of Git, sometimes a merge can introduce issues. Here are common scenarios where you might need to revert a merge commit:
- Introducing Bugs or Unstable Code: If the merged feature contains critical bugs that disrupt functionality, you would want to revert the merge to maintain stability.
- Merging Unintended Branches: Accidental merges can occur, especially in long-running projects with many branches, necessitating a swift rollback.
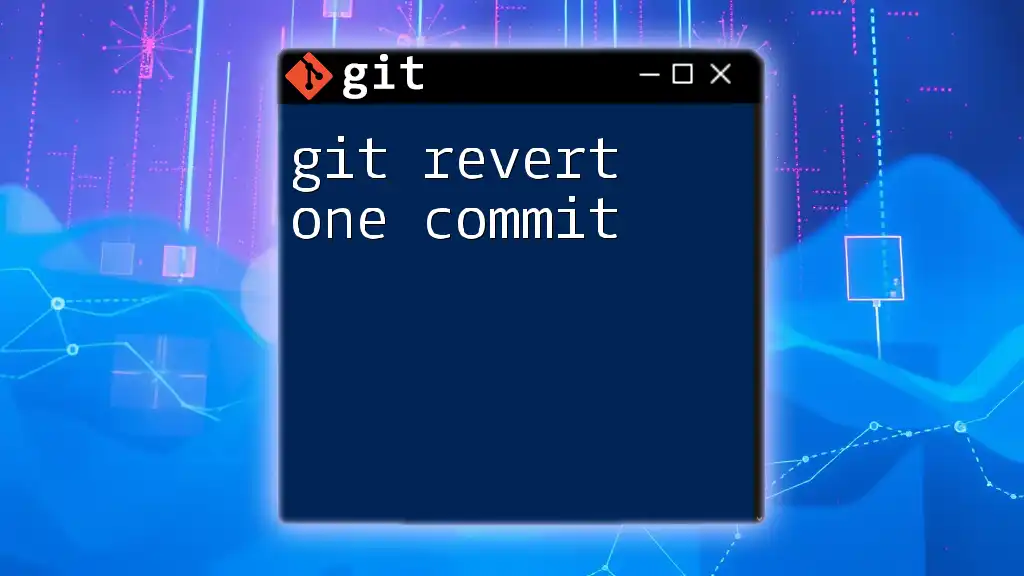
The Mechanics of Reverting a Merge Commit
What Does "Revert" Mean in Git?
In Git, to revert a commit means to create a new commit that undoes the changes made by a specific previous commit. This operation is non-destructive and does not alter existing commit history, making it particularly valuable in a collaborative environment. Instead of erasing the merge, reverting cleans up the effects of the merge without losing any historical data.
How Reverting Differs from Resetting
It’s crucial to understand that reverting is different from resetting. While reverting generates a new commit to negate previous changes, resetting modifies the commit history to a specific state. Using reset can be dangerous since it removes commits permanently, which may lead to loss of work. It's best to use revert to maintain a transparent history of changes.
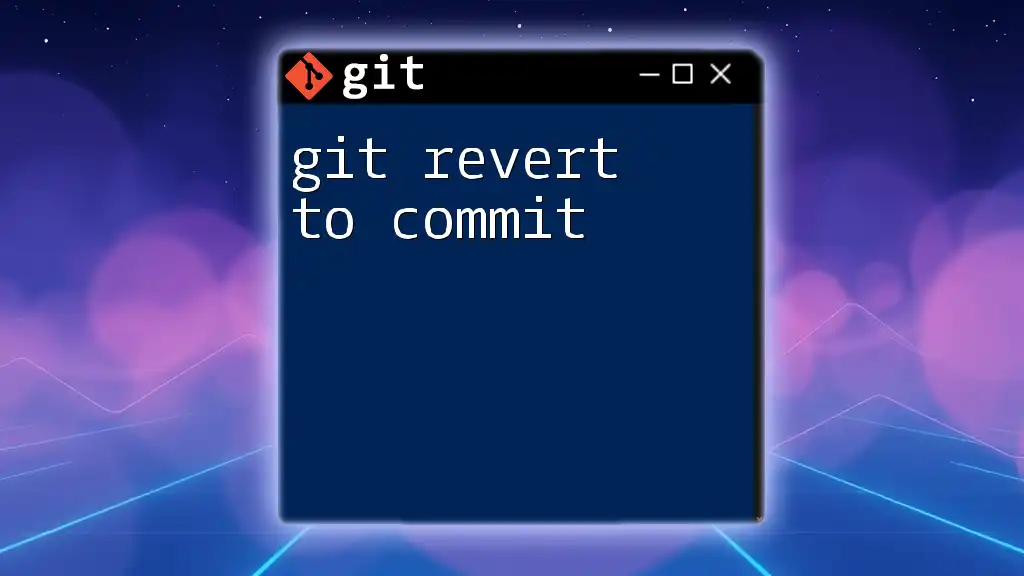
How to Revert a Merge Commit
Step-by-Step Guide to Reverting
Identifying the Merge Commit The first step in the revert process is identifying the hash of the merge commit you want to revert. You can do this by examining your commit history using the following command:
git log --oneline
This command displays a list of commits. Look for the merge commit, which will typically have two or more parent hashes.
Using the Git Revert Command Once you have identified the merge commit hash, you can use the revert command. The syntax is designed specifically for merge commits, and you must specify which parent to keep. The general command is as follows:
git revert -m 1 <merge-commit-hash>
In this command:
- The `-m` option is crucial. It tells Git which parent should be treated as the mainline. In most cases, you typically specify `1` for the first parent.
- `<merge-commit-hash>` should be replaced with the actual hash of the merge commit you identified earlier.
Handling Conflicts During the Revert
Sometimes, reverting a merge commit may lead to conflicts, especially if changes in the mainline branch interfere with the changes introduced by the merge. Here’s how to handle it:
-
Check for Conflict: After running the revert command, use `git status` to check for conflicted files.
git status
-
Resolve Conflicts: Open the conflicted files in your code editor and resolve the conflicts manually. Git also provides a merge tool to assist you.
git mergetool
-
Stage Resolved Files: Once you've resolved the conflicts, add the files back to staging.
git add <resolved-file>
-
Commit Resolutions: Finally, create a commit that includes your changes, commenting that you resolved merge conflicts.
git commit -m "Resolved merge conflicts after reverting merge commit"
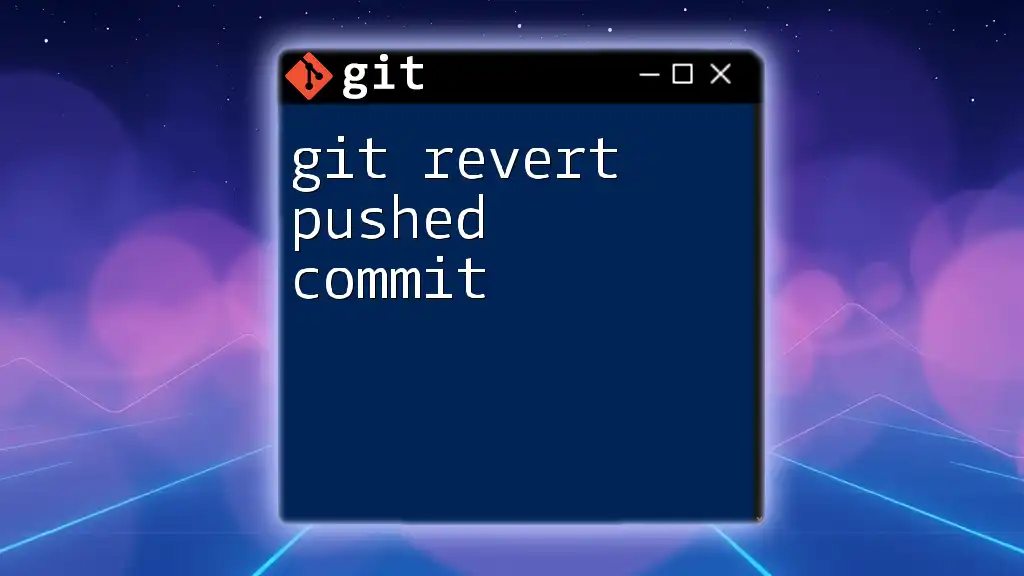
Best Practices for Reverting Merge Commits
Always Test Before Committing
Before committing the revert, it’s a good practice to test the changes locally. This ensures that the reverted code behaves as expected and doesn’t introduce new issues. Consider using a separate branch to experiment freely.
Documenting the Reason for Reverting
Transparency is vital in collaborative projects. When reverting a merge commit, make sure to write a clear and informative commit message that explains why the revert was necessary. This practice helps future collaborators understand the rationale behind changes and contributes to maintaining a clean, informative project history.
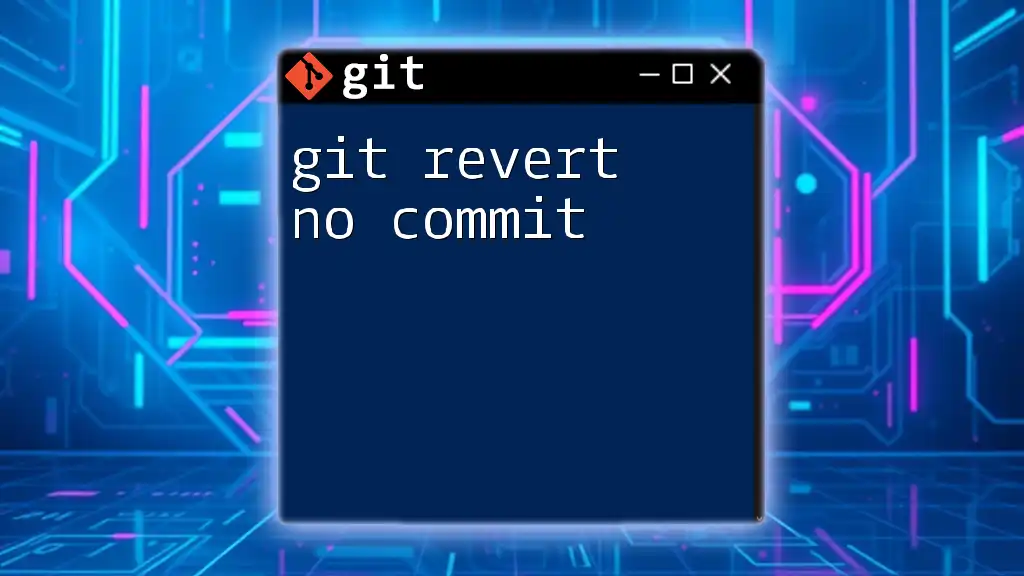
Real-World Scenarios and Examples
Example 1: Reverting a Bad Merge
Imagine you merged a feature branch that unexpectedly broke functionality in your main application. By following the steps to revert the merge, you use:
git log --oneline
You find the hash `abc1234` for the problematic merge. You then run:
git revert -m 1 abc1234
After resolving any conflicts, your project is back to a stable state without losing historical integrity.
Example 2: Collaborative Project Challenge
In a scenario where a teammate mistakenly merges their branch into yours, causing unexpected behavior, it’s essential to calmly address the situation. By reverting the merge, you:
- Identify the merge commit.
- Perform the revert.
- Communicate with your team about why and how this decision was made.
This not only resolves the issue but reinforces team collaboration through good communication practices.
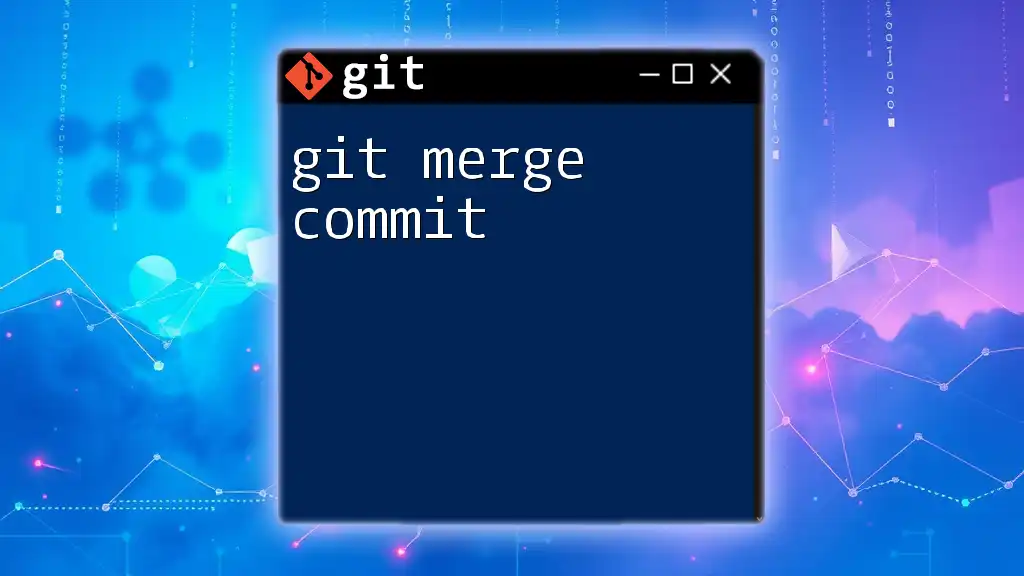
Conclusion
Knowing how to git revert merge commit is a crucial skill for any developer using Git. By understanding the process of reverting merges and implementing best practices, you not only maintain project stability but also preserve the integrity of your code history. As you continue to work on collaborative projects, keeping these principles in mind will serve you and your team well.
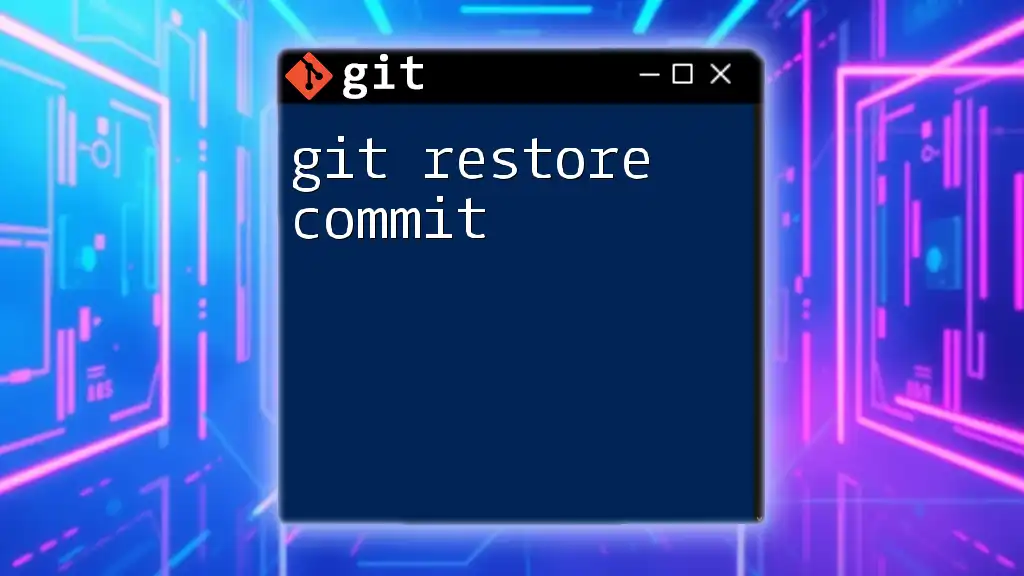
Additional Resources
Recommended Articles and Documentation
For further learning, consult the official Git documentation that dives deeper into `git revert` and merge operations. There are numerous online tutorials available that can enhance your Git skills.
Community and Support
Engage with forums or platforms like Stack Overflow or GitHub Community, where fellow developers can assist you with questions related to Git commands and best practices. Collaborative learning will elevate your understanding of version control systems.