To revert to a specific commit in Git, use the command `git revert <commit-hash>`, which creates a new commit that undoes the changes made in the specified commit.
git revert <commit-hash>
What is Git Revert?
Understanding the Revert Command
The `git revert` command is a powerful tool within Git that allows you to create a new commit that undoes the changes made by a specific commit. Rather than completely removing the commit from the history (as with `git reset`), `git revert` offers a way to keep a clear history while negating the impact of a previous change.
When to Use Git Revert
There are several situations where you might find yourself needing to revert changes in your project:
-
Mistaken Commits: If you accidentally committed changes that should not be in your branch, `git revert` allows you to negate those changes without altering the full history.
-
Experimentation Gone Wrong: Sometimes, when testing out new features or fixes, you might introduce bugs. Using `git revert` can help you back out those changes safely.
The primary benefit of using `git revert` is that it maintains the integrity of your project’s history while allowing you to correct mistakes. Unlike `git reset`, which modifies history, `git revert` keeps the record of what has happened in your project repository intact.
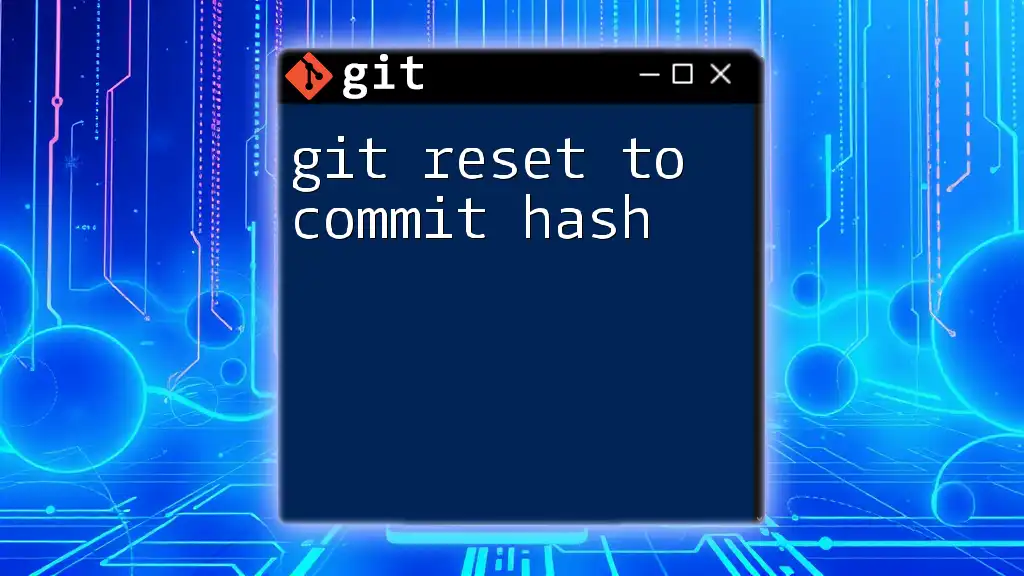
How to Find a Commit Hash
Using Git Log
To revert to a specific commit, you'll first need its commit hash. The easiest way to find this is by using the `git log` command. This command displays the commit history, including the commit hashes, messages, and dates.
For a more concise view, you can run:
git log --oneline
This command shows a simplified version of the history, making it easier to identify the specific commit hash you wish to revert.
Identifying the Correct Commit
When examining your commit history, pay close attention to commit messages and dates. A clear and descriptive commit message will be incredibly helpful in recognizing which commit you need to revert.
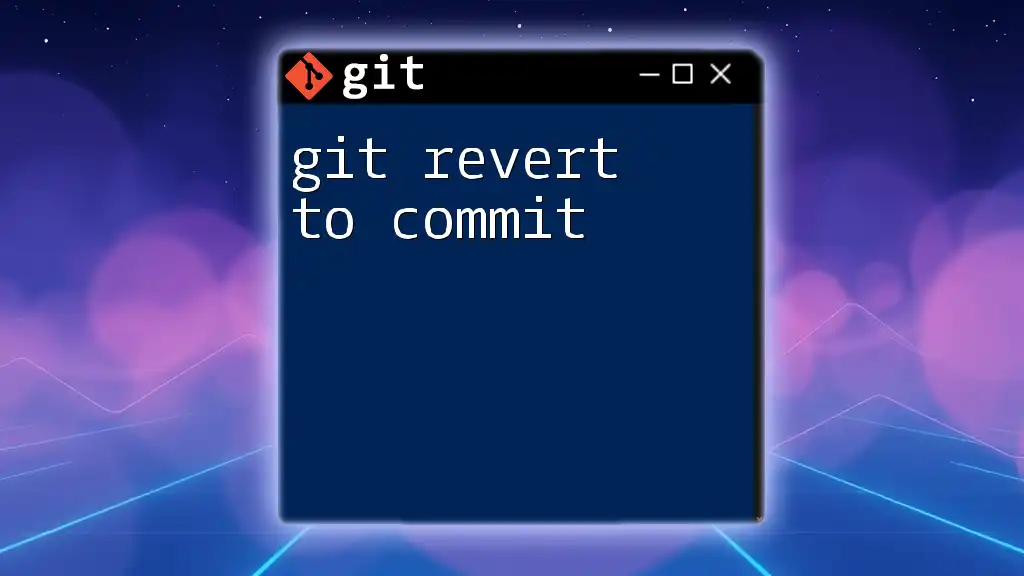
Step-by-Step Guide to Reverting a Commit
The Basics of the Git Revert Command
The syntax for the `git revert` command is straightforward:
git revert <commit-hash>
You simply replace `<commit-hash>` with the hash of the commit you want to negate.
Example: Reverting a Specific Commit
Let’s walk through a practical example. Assume you want to revert a commit with the hash `abc1234`. The command you would use is:
git revert abc1234
Executing this command will create a new commit that effectively reverses the changes introduced in commit `abc1234`. Git will automatically open your default text editor to allow you to edit the commit message. You can leave it as is or customize it to reflect the action taken.
Handling Merge Commits
What are Merge Commits?
Merge commits can complicate the revert process because they involve combining histories from multiple branches. When you attempt to revert a merge commit, you risk losing changes that were made in a different branch.
Reverting Merge Commits
To revert a merge commit, use the `-m` option followed by the parent branch you want to keep. The syntax is as follows:
git revert -m 1 <merge-commit-hash>
In this command, `1` indicates that you want to keep the first parent of the merge commit. For instance, if your merge commit hash is `def5678`, the complete command would be:
git revert -m 1 def5678
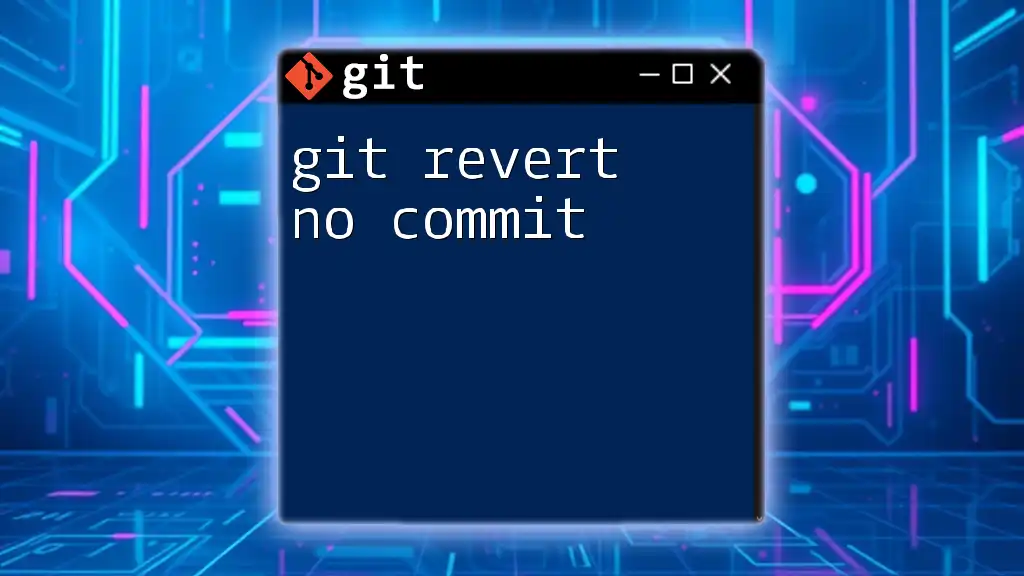
Handling Conflicts After Reverting
Understanding Conflicts
Conflicts can emerge during a revert operation, especially if the changes being reverted intersect with changes made after the original commit. Conflicts must be resolved before the revert can be completed.
Resolving Conflicts
Follow these steps to resolve conflicts:
-
Identify Conflict Areas: When a conflict occurs, Git will mark the conflicting files in the output. You can check the status with:
git status
-
Manually Edit Files: Open the conflicted files in your editor. Git will insert conflict markers to indicate what needs to be resolved.
-
Stage the Resolved Changes: After resolving the conflicts, stage the resolved files:
git add <resolved-file>
-
Finalize the Revert: Once all conflicts are resolved and staged, commit the changes with a meaningful message indicating you resolved conflicts after reverting:
git commit -m "Resolved conflicts after reverting commit abc1234"
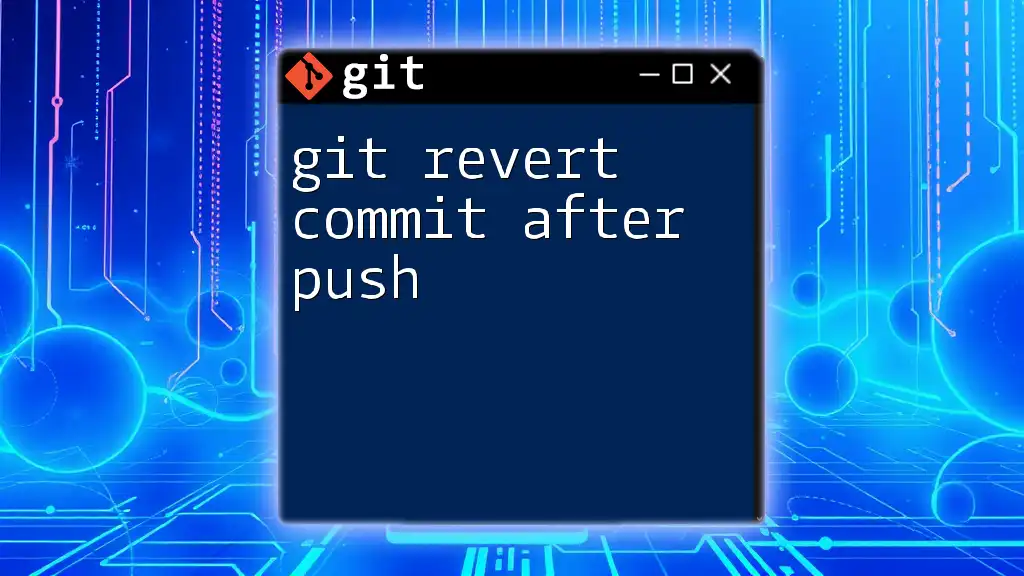
Alternatives to Revert
Git Reset vs. Git Revert
While `git revert` is a safe method for negating changes, there are scenarios where you might choose `git reset` instead. Unlike `git revert`, which preserves commit history, `git reset` removes commits from the history altogether. Use it with caution as it can lead to lost changes.
Example Demonstrating the Impact
If you use `git reset` to reset to a previous commit, you might execute:
git reset --hard <commit-hash>
This command will discard all changes made after `<commit-hash>`. It can be powerful but potentially destructive, so always ensure this is your desired outcome.
Other Useful Commands
Git Checkout
The `git checkout` command allows you to switch branches or restore files to a previous state. However, it doesn't create a new commit negating changes like `git revert` does. For example:
git checkout <branch-name>
This command switches your working directory to the specified branch.
Git Restore
As another alternative, the `git restore` command can be used to discard changes in your working directory or index without creating a new commit. For example, to discard uncommitted changes, you could use:
git restore <file>
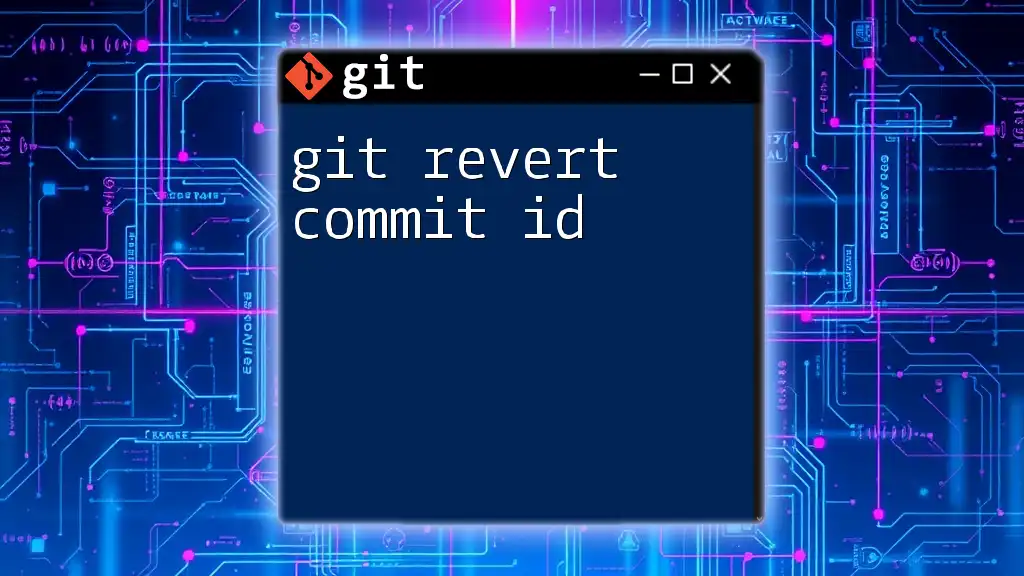
Conclusion
Understanding how to use `git revert to commit hash` is essential for any developer working with Git. By utilizing `git revert`, you can effectively manage your project's history while ensuring that mistakes are easily corrected without losing significant data.
Embrace the power of Git and practice these commands to deepen your understanding and improve your workflow. For further resources, consider exploring additional guides and documentation to enhance your Git expertise.