A git commit hash is a unique identifier for each commit in a Git repository, represented as a 40-character hexadecimal string, which can be used to reference specific changes in your project history.
Here’s an example of how to view the commit hash of the latest commit:
git log -1
Understanding Git Commit Hash
What is a Git Commit Hash?
A git commit hash is a unique identifier that git generates for each commit in a repository. This hash is crucial for maintaining the integrity of your data and serves as a reference point in the history of your project. Each commit is a snapshot of your project at a certain stage, and the commit hash allows you to pinpoint exactly where and when changes were made.
Structure of a Git Commit Hash
The git commit hash is typically a 40-character string represented in hexadecimal format, derived from the SHA-1 cryptographic hashing algorithm. For example, a typical commit hash may look like this: `e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd`. Each hash is calculated based on the contents of the commit, including the files changed, timestamps, and the author's information.
This structure means that even a tiny change in the commit—or the slightest variation in its details—results in a radically different hash. This uniqueness is fundamental for version control, as it ensures that each commit can be individually referenced.
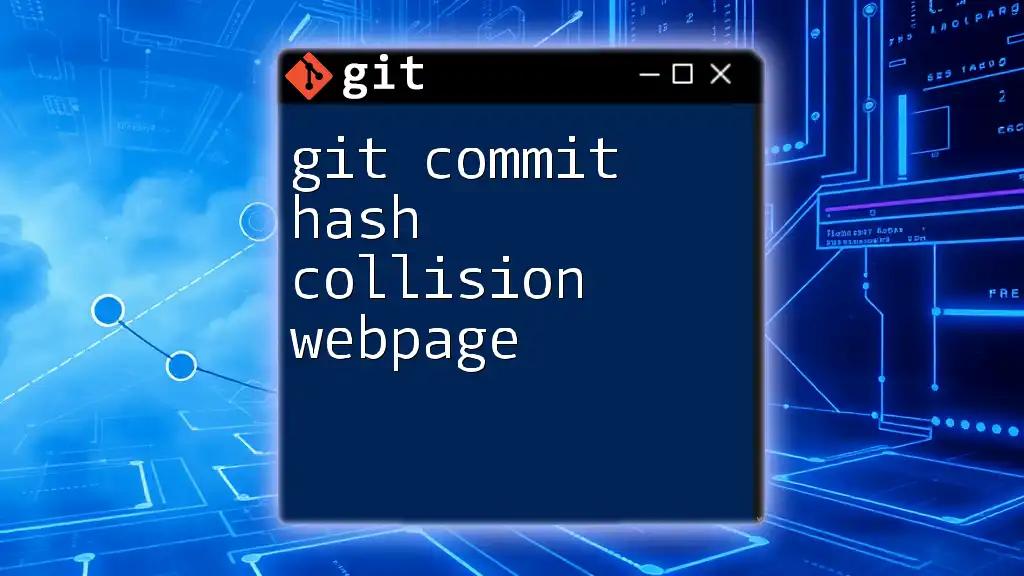
The Importance of Git Commit Hash
Unique Identification of Commits
Every commit hash serves as a unique identifier that distinguishes one commit from another. It allows developers to track changes with precision, navigating through a project's history to see how it has evolved over time. This is particularly vital when you are working collaboratively with others, as it provides a point of reference that can prevent confusion.
Role in Collaborative Development
In a team setting, the git commit hash facilitates coordination among developers. When working on shared codebases, knowing the exact commit hash allows team members to discuss specific changes, further enhancing communication. For example, if a developer encounters a bug, referring to a commit by its hash can help others understand what was altered before the issue arose, simplifying collaboration and problem-solving.
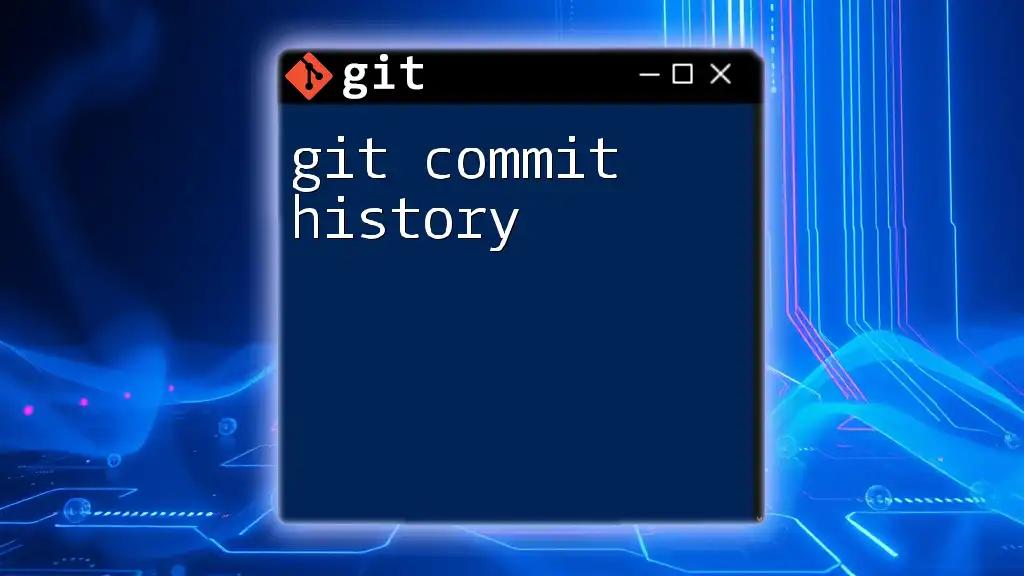
Working with Git Commit Hash
Viewing Commit Hashes
To view the commit hashes in your repository, you can use the command:
git log
The output will display a list of commits along with their hashes, authors, dates, and commit messages. Each entry starts with the commit hash. For instance:
commit e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd
Author: Jane Doe <jane.doe@example.com>
Date: Tue Oct 3 14:12:31 2023 -0400
Fixed a bug in the authentication module
In this output, `e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd` is the commit hash. Understanding this output is essential for navigating your project’s history.
Using Commit Hashes
Checking Out a Specific Commit
When you need to access the code as it was in a specific commit, you can check it out using the commit hash:
git checkout <commit-hash>
For example, if you want to view the state of the project at the commit `e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd`, you would use:
git checkout e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd
Note that checking out an old commit places you in a detached HEAD state, meaning that you're no longer on a branch. Any changes made here won't be associated with any branch unless you create a new one.
Comparing Commits Using Hash
You can also utilize commit hashes to compare the differences between two different commits. This can be accomplished with:
git diff <commit-hash-1> <commit-hash-2>
This command will show you the differences between the two specified commits, allowing for critical analysis of what has changed during specific phases of development.
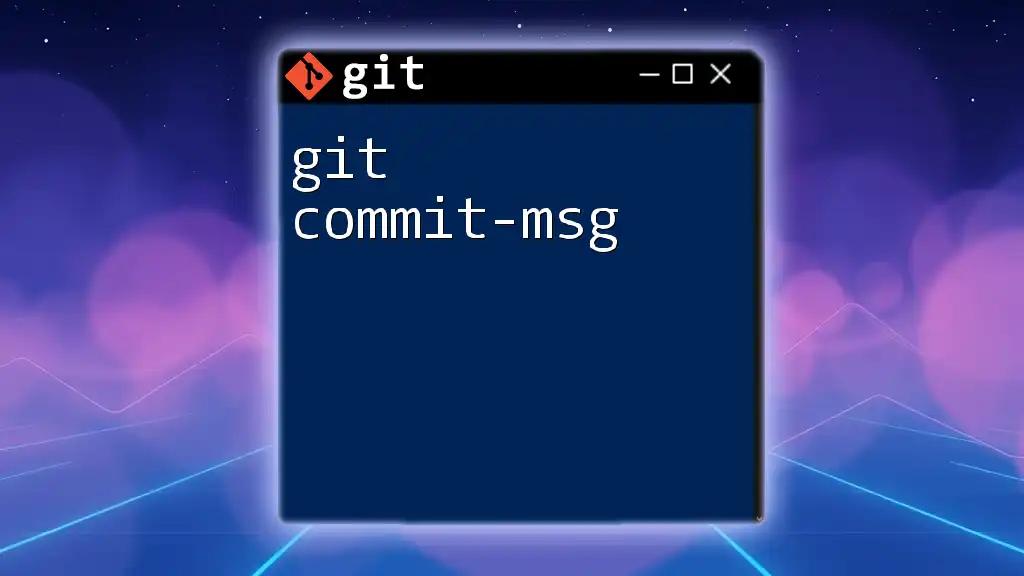
Finding Commit Hashes
Using Git Log for Commit History
The `git log` command not only allows you to view the hashes but also provides a comprehensive history of all commits in reverse chronological order. Each log entry will show the commit hash, the author, the date, and the commit message.
You can navigate through this history using various options, such as:
- `git log --oneline` for a more condensed view.
- `git log --author="Author Name"` to filter commits by a specific author.
- `git log --since="date"` or `git log --until="date"` to focus on commits within a particular timeframe.
Searching for a Specific Commit Hash
Finding a commit hash associated with a particular message can be easily achieved with the `--grep` option:
git log --grep="specific commit message"
This will return all commits whose messages match the query, making it easier to locate the exact commit you're interested in.
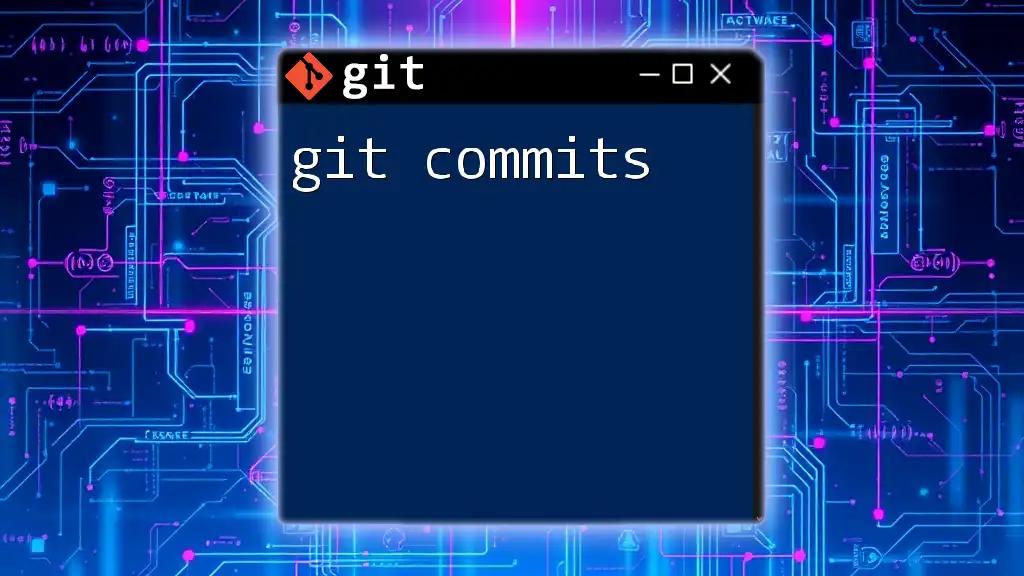
Best Practices for Using Git Commit Hashes
Document Your Commit Messages
Clear and concise commit messages are vital for understanding the context of each commit. You should aim to include information about what was changed and why. An example of a well-structured commit message might be:
Fix: Resolve race condition in authentication logic
Such a message clearly states what the change addresses, making it easier for collaborators to understand the history of the project.
Using Tags with Commit Hashes
Tags are useful for marking important commits, like releases or milestones, allowing for easy reference in the future. You can tag a commit using:
git tag -a <tagname> <commit-hash> -m "Tag message"
For instance:
git tag -a v1.0 e98b1a6cfd4f59d212d1a6aabf8e2c70027328fd -m "Release version 1.0"
This approach not only helps in versioning but also improves clarity when navigating through your commit history.
Avoiding Common Pitfalls
To prevent confusion while navigating commit history, always ensure you are aware of your current branch and the state of the repository. Utilize commands such as `git status` frequently to keep track of changes. When working with complex projects, it's also beneficial to keep recent commit hashes easily accessible to skip past them when working with older history.
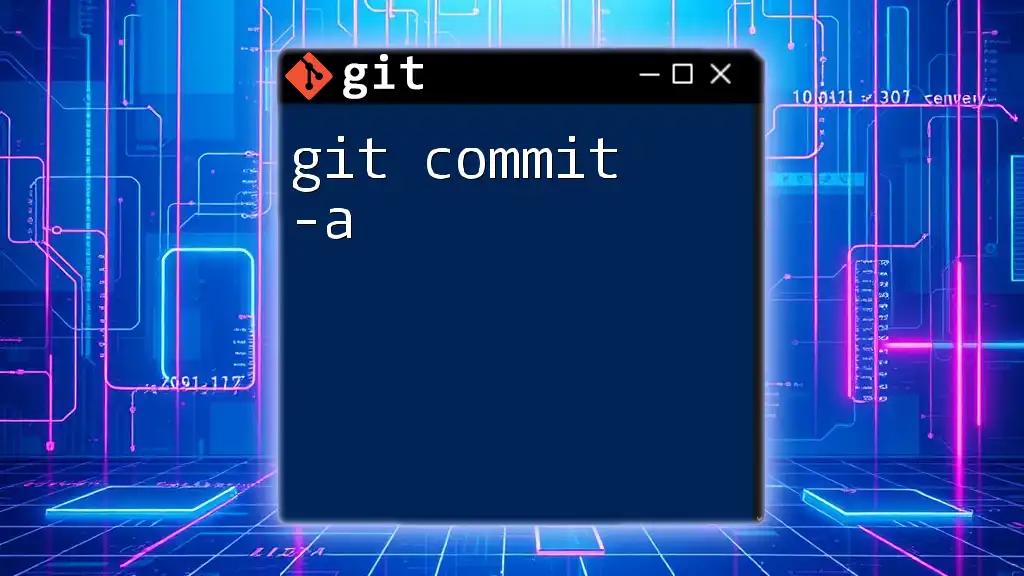
Conclusion
A clear understanding of git commit hashes is essential for effective version control and collaborative software development. These unique identifiers not only enable precise tracking of changes but also provide the foundation for clear communication among development teams. As you continue to explore Git, build good habits around documenting commits, utilizing tags, and referencing commits will significantly enhance your workflow.
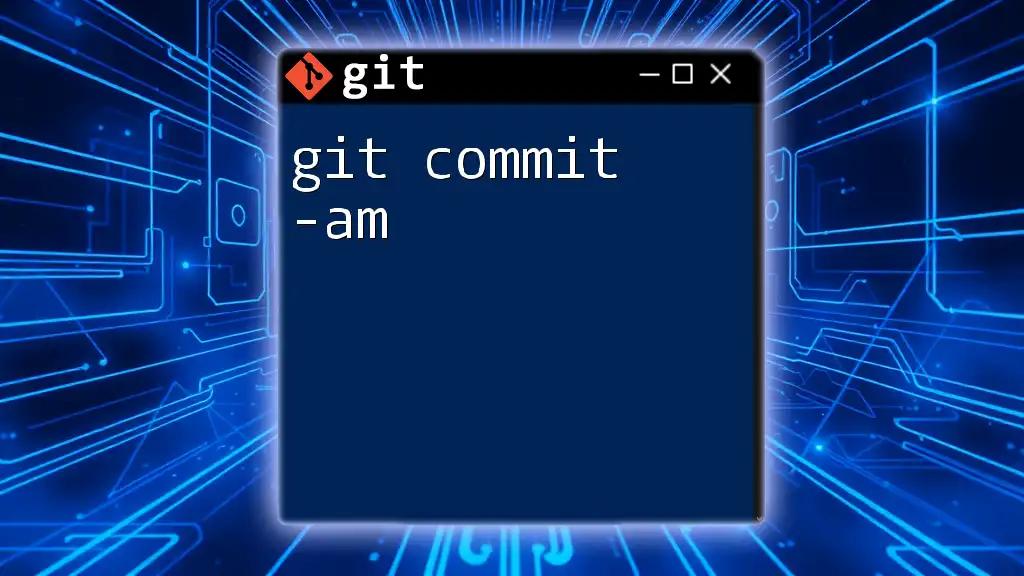
Additional Resources and References
For further learning and mastery of git commands, refer to the official Git documentation, as well as recommended books and online courses that deep dive into version control practices.