A git commit is a command used to save changes to the local repository, capturing a snapshot of your project’s current state along with a descriptive message. Here's an example command to make a commit:
git commit -m "Your commit message describing the changes"
What is a Git Commit?
A git commit is a fundamental operation in Git, a distributed version control system. It represents a snapshot of your project's files at a specific point in time. Each commit saves changes made to files in your working directory and records a message that describes those changes. This allows you to track the history of your project and collaborate effectively with others.
The role of commits is vital in Git's architecture. They form the timeline of a project, allowing developers to navigate through previous versions of their code. Well-structured commit messages can provide valuable context, making it easier for team members to understand what changes were made and why.
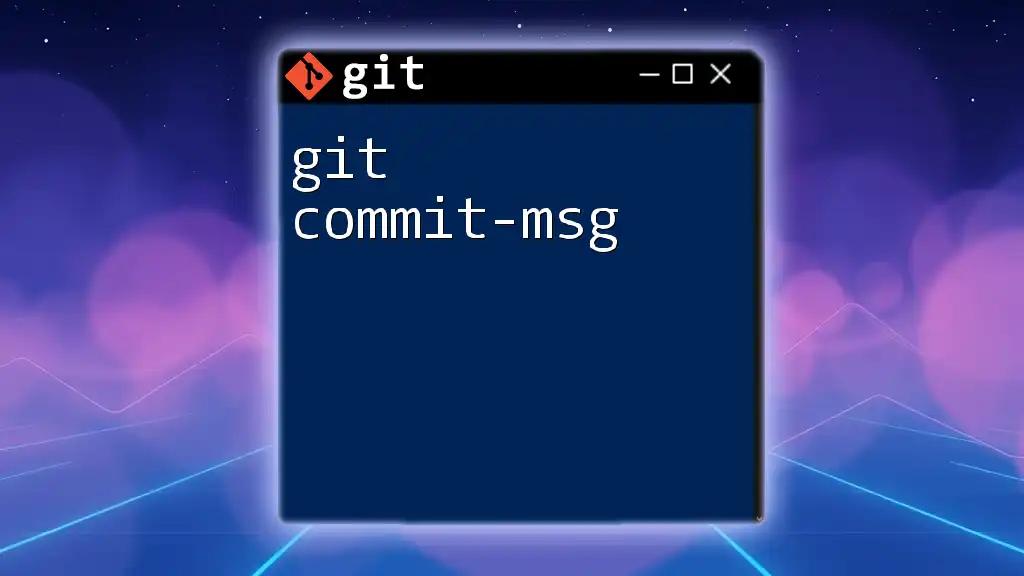
Understanding the Commit Process
The Three States of Git
In Git, there are three essential states that files can reside in during the commit process:
- Working Directory: This is where you make changes to your files. Any modifications are saved in this area.
- Staging Area: Once you’ve made changes, you prepare them for a commit by "staging" the files. This area acts as a middle ground between your working directory and the repository.
- Repository: The finalized commits are stored here. This is where your project's history resides.
How Committing Works
The process of committing involves several steps. Here’s a concise overview:
-
Make Changes: Modify your files in the working directory.
-
Stage Changes: Add these changes to the staging area with the command:
git add <file-name>
You can stage all changes by using:
git add .
-
Commit Changes: Once staged, you can commit the changes using:
git commit -m "Your descriptive commit message here"
This straightforward process allows you to track different versions of your project over time.
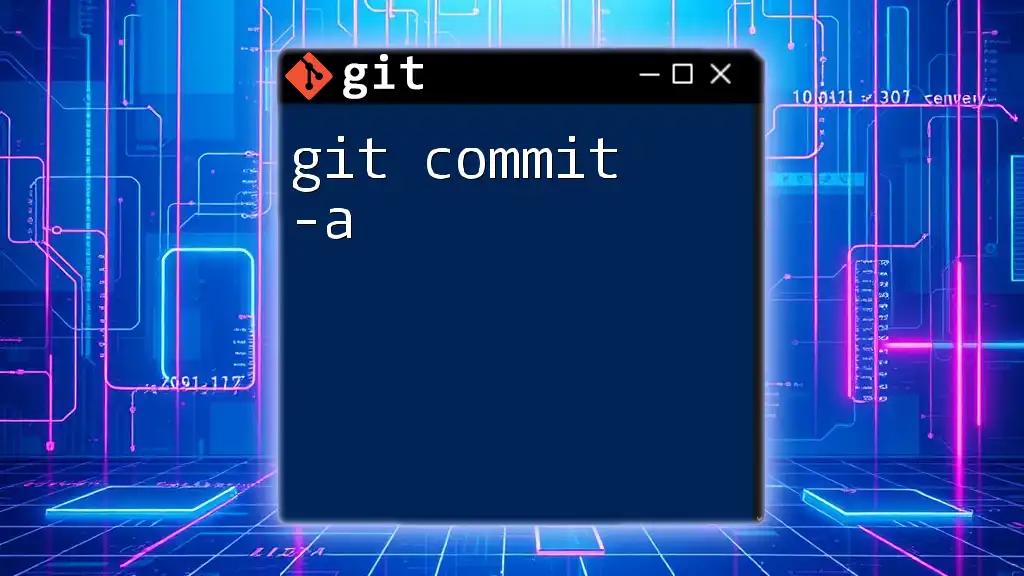
Crafting Meaningful Commit Messages
Best Practices for Commit Messages
Crafting clear and concise commit messages is crucial for maintaining a coherent project history. Here are some key practices to follow:
- Start with a summary that concisely describes the changes.
- Use the imperative mood (e.g., "Fix bug" instead of "Fixed bug").
- Limit the summary to around 50 characters for easy readability.
- In the description, explain the why behind the changes if necessary.
Examples of Good vs. Bad Commit Messages
Understanding the distinction between effective and ineffective commit messages is important:
-
Good Message: "Fix bug in payment processing logic"
This message is clear about what was fixed. -
Bad Message: "Fixed stuff"
This is too vague, offering no insight.
Using specific messages enhances collaboration and minimizes confusion when other developers review the project history.
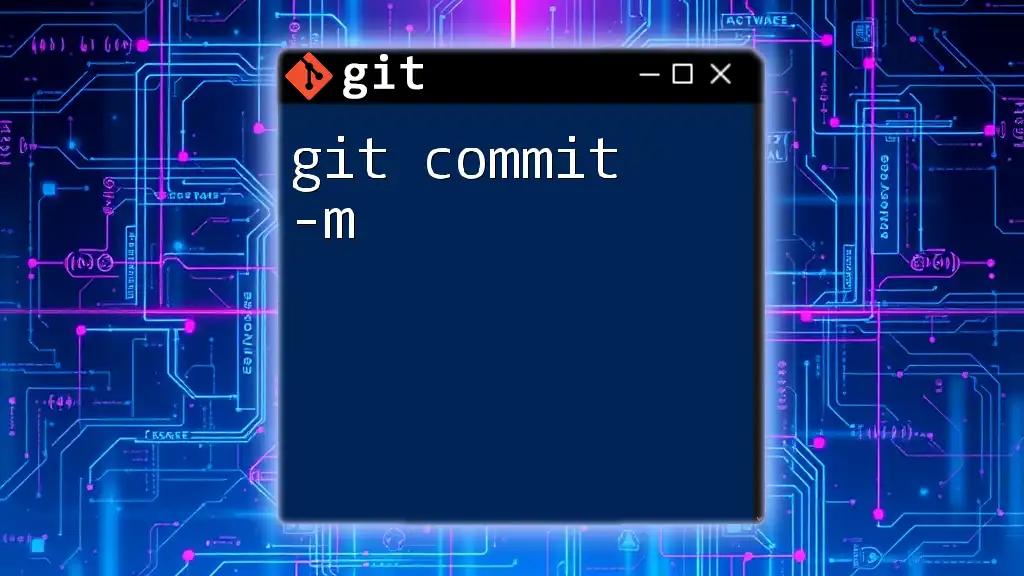
The Anatomy of a Git Commit
Understanding Commit Metadata
Every commit contains metadata, forming a commit object in Git. The notable components include:
- Author Information: Identifies who made the commit.
- Timestamp: Records when the commit was made.
- Commit Message: Provides a description of the changes made.
- Changeset: Details the actual changes to files.
Viewing Commit Details
You can explore the specifics of a commit using the `git show` command:
git show <commit_hash>
This command presents the commit’s content, allowing you to examine the files affected and the messages associated with the changes.
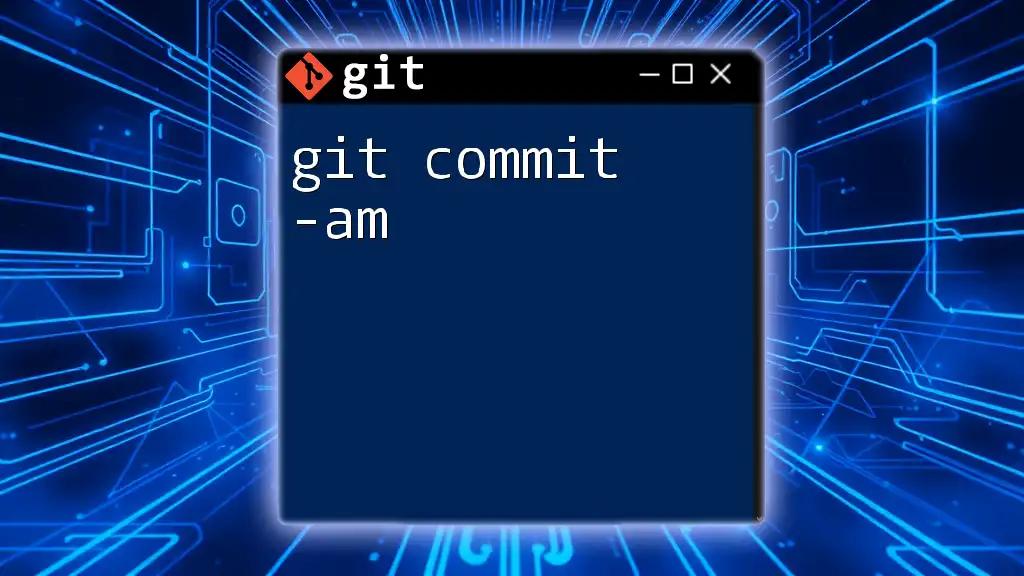
Making Your First Commit
Initializing a Git Repository
To get started with Git, you first need to initialize a new repository. You can do this with the command:
git init
This command creates a new folder structure where your project will reside, including the necessary git configurations.
How to Stage Changes
Once you have made changes to your files, you will need to add them to the staging area. You can do this using:
git add <file>
For all files at once, use:
git add .
This command ensures all modified files are ready for the commit.
Executing Your First Commit
After staging, you can finalize your changes with your first commit:
git commit -m "Initial commit"
This creates a snapshot of your project’s current state, allowing you to return to it later.
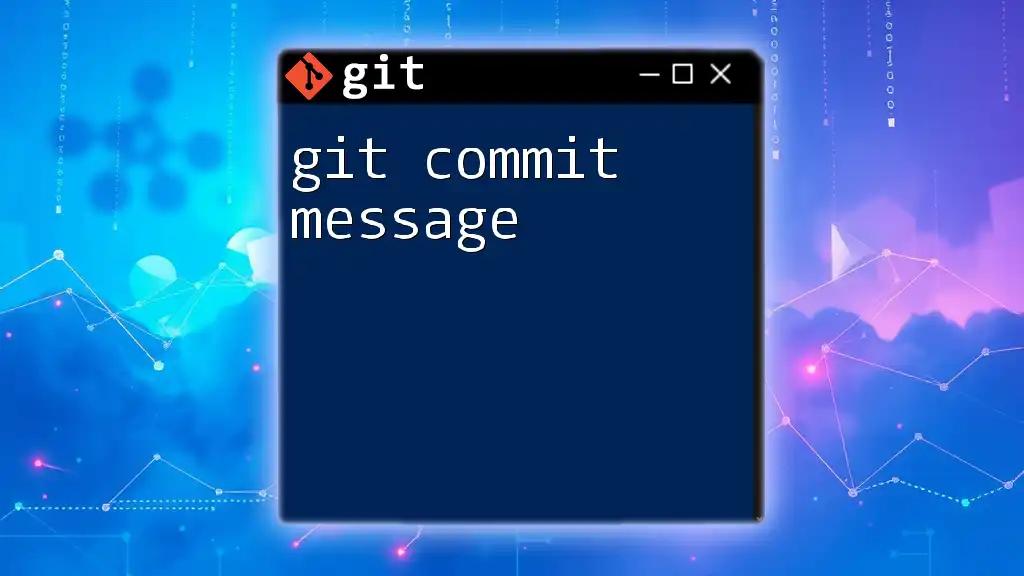
Amending Commits
Understanding the Need for Amending
There may be times when you realize a recent commit needs modification, such as correcting a message or adding a forgotten file. In such cases, amending a commit can be a useful option.
How to Amend a Commit
You can amend the most recent commit with the command:
git commit --amend
This command allows you to edit the existing commit message or add additional changes. Be cautious, as amending can alter the project's history, especially if the commit has already been shared with others.
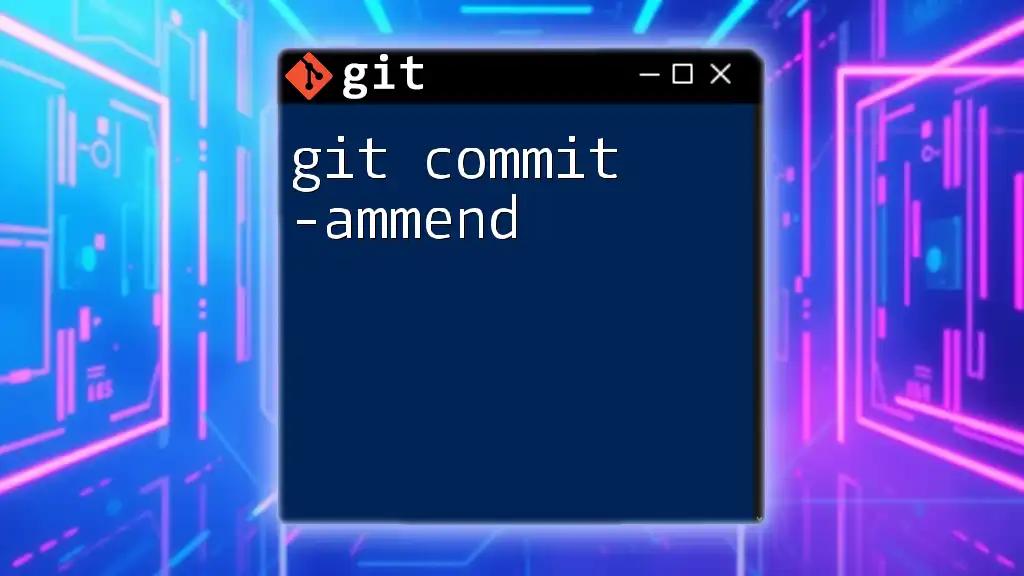
Reverting and Undoing Commits
The `git revert` Command
If you need to undo changes made by a commit, the `git revert` command is a safe option. This command creates a new commit that reverses the changes made by the specified commit, keeping your project history intact:
git revert <commit_hash>
This is particularly useful for undoing changes without losing the context of your project’s history.
Using `git reset` to Undo Changes
Another approach to undo commits is using the `git reset` command. This comes in three types:
- Soft Reset: Keeps changes in the staging area.
git reset --soft HEAD~1
- Mixed Reset (default): Keeps changes in the working directory but unstages them.
git reset HEAD~1
- Hard Reset: Discards all changes, reverting to the specified commit.
git reset --hard HEAD~1
Choose the appropriate reset option based on your intentions and the implications of losing changes.
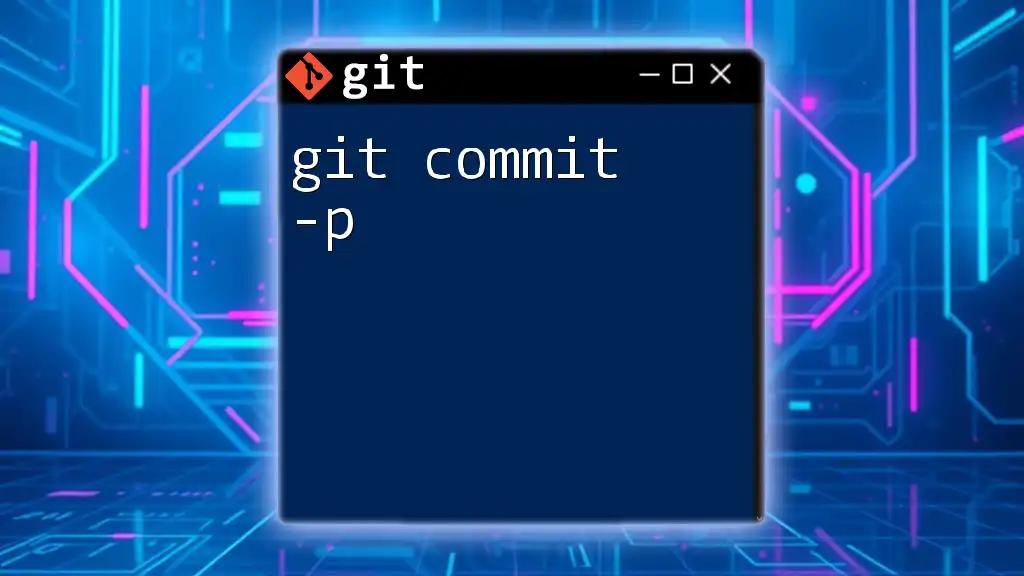
Commit Best Practices
Keeping Your Commit History Clean
Maintaining a clean commit history is essential for effective project management. Aim for atomic commits, which means each commit should represent a single logical change. This practice facilitates easier understanding and debugging for team members.
Using Branches Effectively
Branching is an effective technique for managing your commits. By creating a branch, you can develop features or fix bugs without affecting the main codebase. After development is complete, you can merge the branch back into the main branch. Here’s how you can create a branch:
git branch <branch-name>
And then switch to it with:
git checkout <branch-name>
This allows you to manage separate lines of development seamlessly.
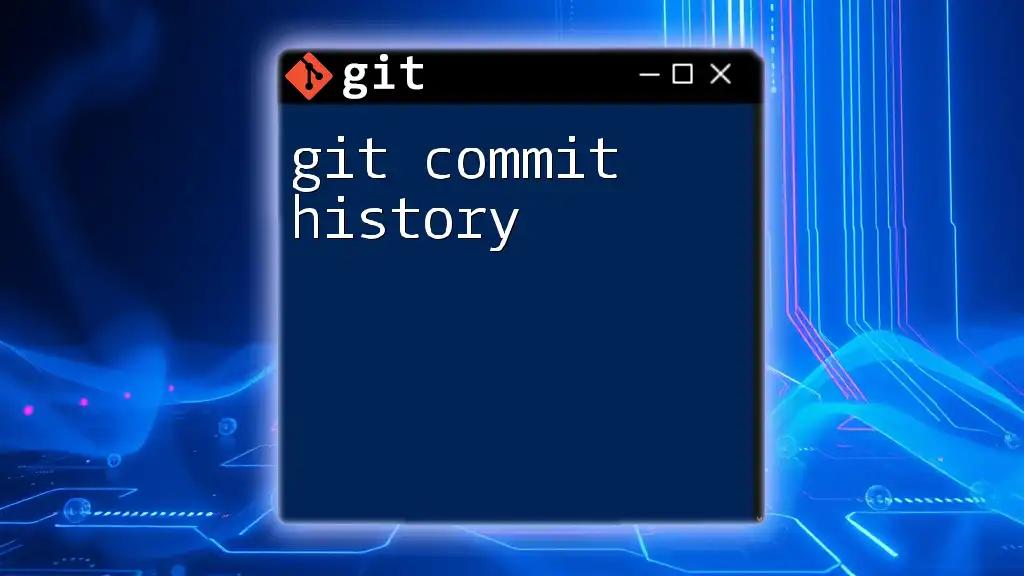
Conclusion
Understanding git commits is foundational for anyone looking to leverage Git effectively. By adhering to best practices for crafting messages, managing your commit history, and utilizing branching strategically, you can enhance both individual and collaborative development processes. Good commit practices not only document your work but also enable effective collaboration across teams, making version control more efficient and streamlined.
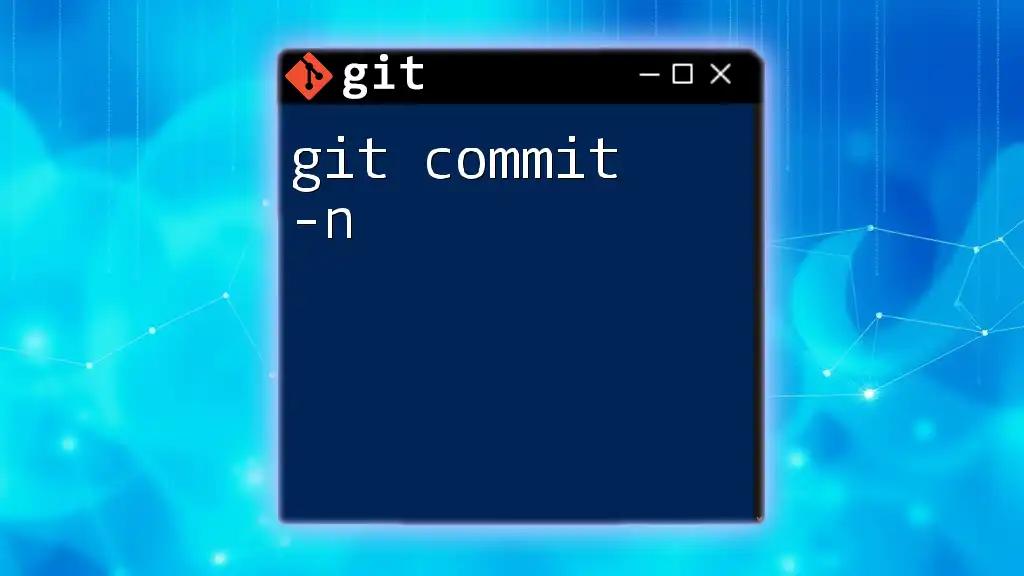
Additional Resources
To further enrich your knowledge about git commits and version control, consider exploring detailed documentation and resources available online, including official Git documentation and various tutorials tailored for developers at all levels. Tools like Git GUI can also simplify your commit management, providing a user-friendly interface for creating and navigating commits.