In Git, you can create an empty commit to serve as a marker or for documentation purposes by using the following command:
git commit --allow-empty -m "Your commit message here"
Understanding Git Commits
What is a Git Commit?
A Git commit serves as a snapshot of your project at a specific point in time. Each commit represents a change or a set of changes that have been recorded in your repository. This enables developers to keep a history of their work and facilitates collaboration in a team environment. Commits are essential in version control as they allow you to revert to previous versions, track changes over time, and effectively manage contributions from multiple team members.
How Git Records Changes
Git manages changes by creating a series of snapshots rather than keeping an ongoing record of changes. This means that all the file changes made in a commit get saved together with a unique identifier. The commit object includes metadata such as the author, timestamp, and message, which provides context around the changes made.
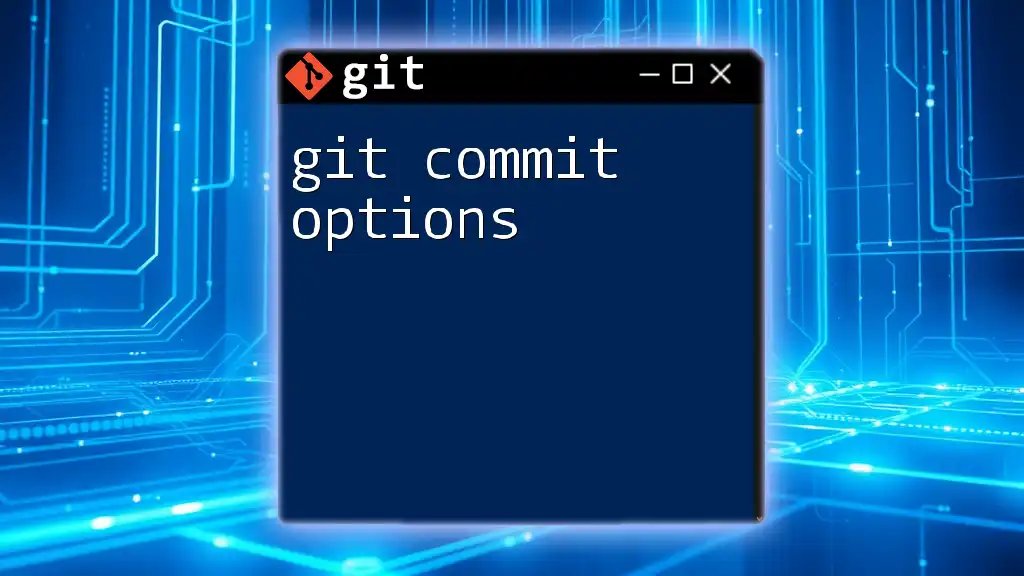
The Concept of an Empty Commit
What Constitutes an Empty Commit?
An empty commit in Git is a commit that contains no changes. This means no modifications were made to any files in the repository; however, you still create a commit entry in the project's history. This may seem counterintuitive, but there are specific instances where this can be particularly useful.
Use Cases for Empty Commits
-
Creating a Marker: Empty commits can serve as markers, indicating a specific milestone or point in development. This can provide a clear reference within the commit history that signifies, for example, the start of a new feature or the completion of a significant phase in a project.
-
Triggering CI/CD Processes: Continuous Integration/Continuous Deployment (CI/CD) pipelines often rely on commits to trigger builds. An empty commit can thus serve as a trigger point without changing any code. This is advantageous if you want to refresh your build system or trigger tests without altering your codebase.
-
Communicating Changes: Sometimes, team members may want to document a status update or decision without making any code changes. An empty commit allows you to add a message for future reference without affecting the content of the repository.
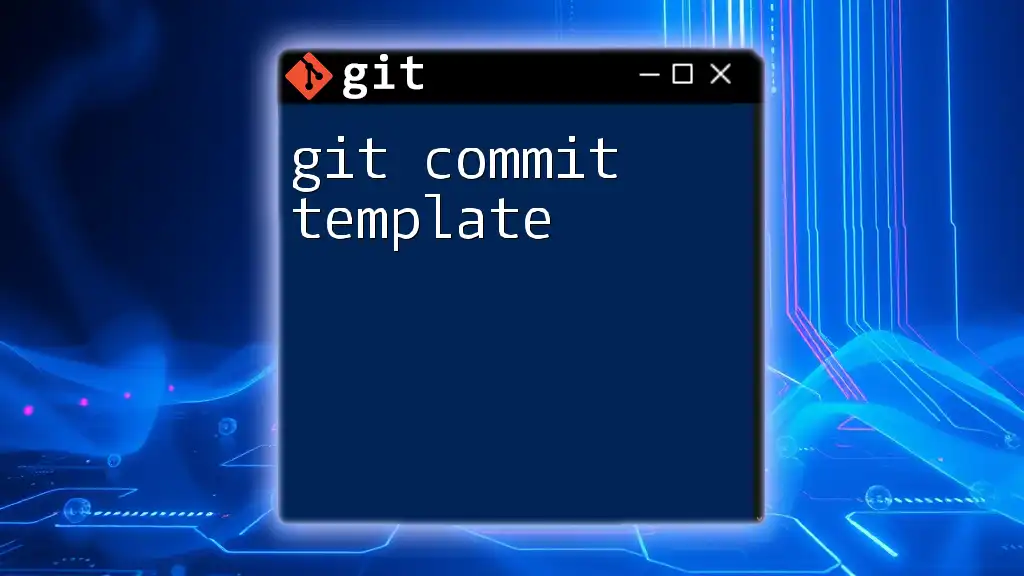
How to Create an Empty Commit in Git
Using the Command Line
Creating an empty commit is straightforward. You can do this using the following command:
git commit --allow-empty -m "Your commit message here"
In this command, `--allow-empty` is the key flag that permits you to create a commit even when there are no changes staged.
Practical Examples
Example 1: Creating a Marker Commit
Suppose you've reached a significant milestone and want to document this in the commit history. You can simply run:
git commit --allow-empty -m "Marker: Starting new development phase"
This will create an empty commit tagged with a meaningful message to signify this starting point.
Example 2: Triggering a CI/CD Pipeline
If you need to trigger your CI/CD pipeline without any code changes, use:
git commit --allow-empty -m "Trigger CI/CD build without code changes"
This commits an empty change and allows your CI/CD system to start the testing and deployment process.
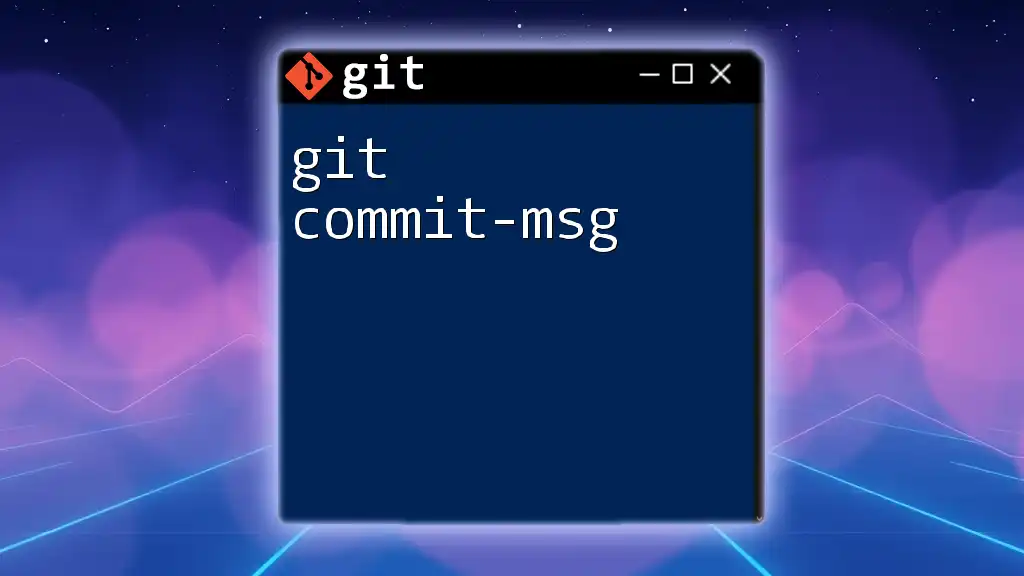
Advanced Usage of Empty Commits
Combining Empty Commits with Other Git Features
Using empty commits with branches
You can create an empty commit on a specific branch, which can help manage your development flow. For example, if you're on a new feature branch:
git checkout feature/my-new-feature
git commit --allow-empty -m "Initial setup for new feature development"
Amending an empty commit
If you decide you need to change the message of an empty commit you created earlier, you can amend it using:
git commit --amend --allow-empty -m "Updated marker commit message"
Integrating Empty Commits in Workflows
Best Practices
While empty commits can be useful, they should be used judiciously. Ensure that any empty commit serves a clear purpose – be it marking a milestone, triggering a deployment, or documenting a project decision. Overusing empty commits may clutter your commit history and make it challenging for team members to navigate.
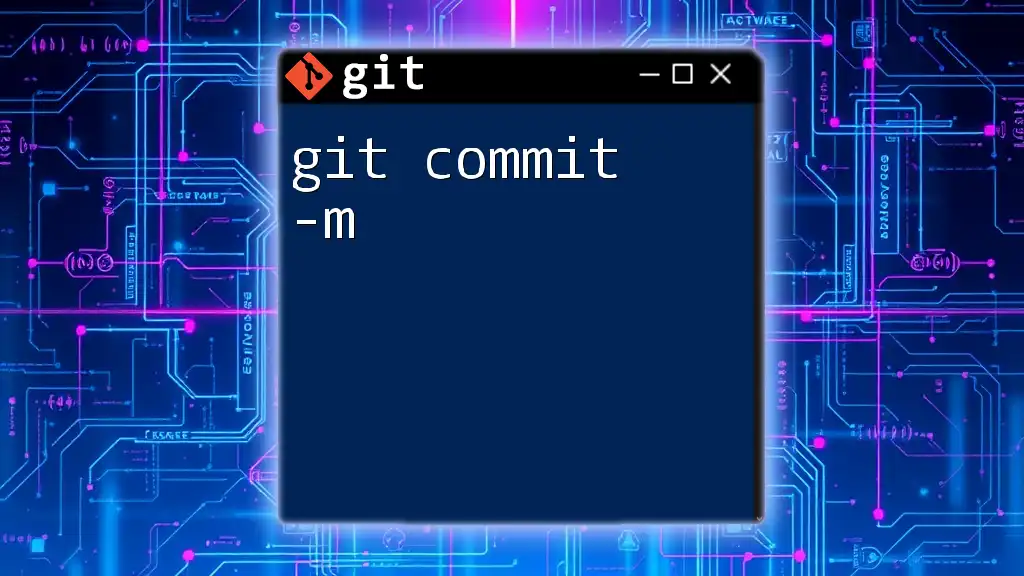
Troubleshooting Common Issues
"Nothing to Commit" Errors
When attempting to create an empty commit, you may encounter a “nothing to commit” error. This could happen if you do not include the `--allow-empty` flag. To prevent this, always remember to specify the flag when you wish to create an empty commit.
When CI/CD Does Not Trigger
If you find your CI/CD pipeline isn't being triggered despite committing an empty change, consider the following:
- Build Configuration: Ensure that your CI/CD setup is configured to recognize empty commits as a valid trigger.
- Permissions: Check that your Git service (like GitHub, GitLab, etc.) allows for empty commits to pass through to the CI/CD system.
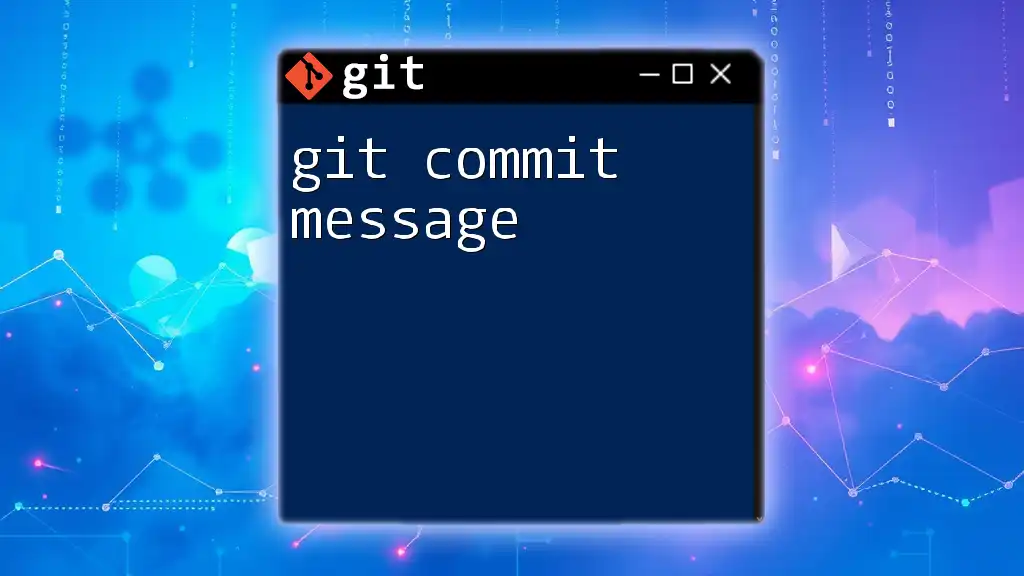
Conclusion
Understanding how to effectively use the `git commit empty` command can significantly enhance your workflow, whether for marking development phases, triggering CI/CD processes, or providing clear project communication. Grasping the nuances of empty commits enables you to leverage your version control system more effectively while maintaining a clean and understandable commit history.
With this knowledge, you can confidently incorporate empty commits into your version control practices. Start experimenting with empty commits in your projects to assess their utility, and see how they can streamline your development process!
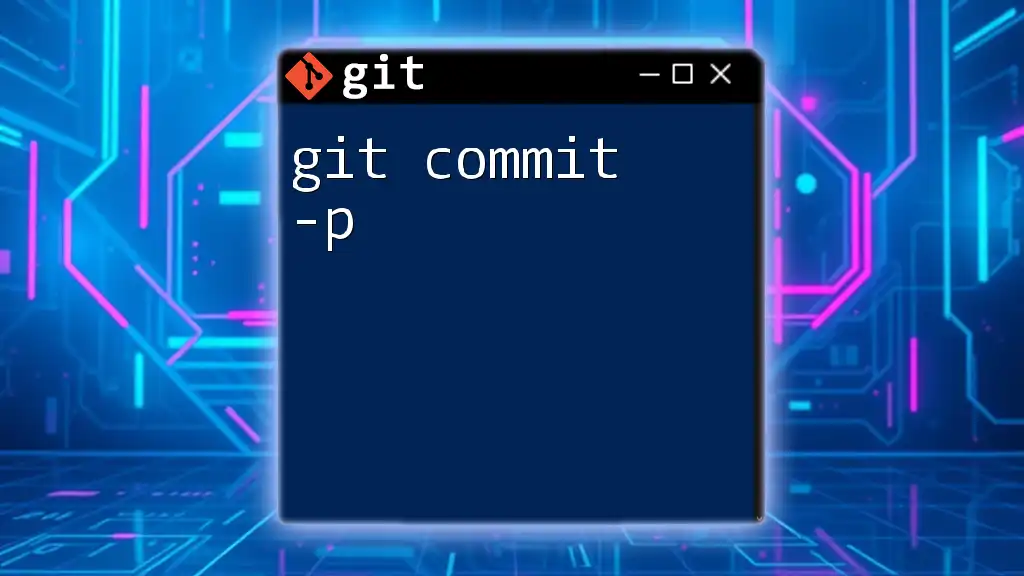
Further Reading and Resources
For those looking to deepen their understanding, consider exploring the official Git documentation on commits, diving into advanced Git techniques, or participating in community forums and discussion groups focused on Git usage.