To undo a pushed commit in Git, you can use the `git revert` command followed by the commit hash to create a new commit that reverses the changes made by the specified commit.
git revert <commit-hash>
Understanding Git Revert
What is Git Revert?
The `git revert` command is a vital tool in the Git version control system that allows you to undo changes that have already been committed to your repository. Instead of erasing the history or rolling back to a previous state, `git revert` creates a new commit that effectively undoes the changes made by a specified commit. This is especially useful in collaborative environments where maintaining a clear history of changes is essential.
When to Use Git Revert
Utilizing `git revert` is appropriate in various scenarios, particularly when you need to:
- Undo a commit that has already been shared with other team members.
- Preserve the integrity of your project history.
- Avoid the complications that can arise with commands like `git reset`, which may lose commit history.
The primary benefit of `git revert` is its ability to create a new commit that offsets previous changes while maintaining a complete history, making it an ideal option for collaborative work.
How Git Revert Works
When you execute `git revert`, Git generates a new commit that applies the inverse of the changes made in the identified commit. This mechanism ensures that your project history remains intact and traceable.
To illustrate, if you have a commit that introduced a new feature and you find it to be buggy, running `git revert <commit-hash>` allows you to undo that specific change without removing it from the commit history. Instead of erasing this feature permanently, you are effectively applying a corrective change on top of it.
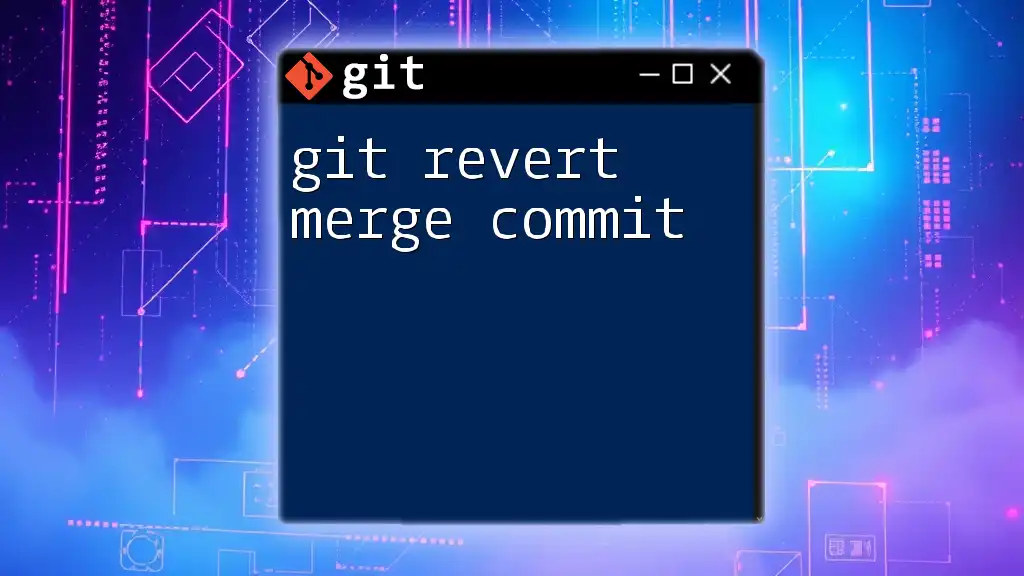
Steps to Revert a Pushed Commit
Step-by-Step Guide to Reverting
Identifying the Commit to Revert To begin, you need to find the commit hash of the change you want to revert. You can do this using the `git log` command, which will display a history of commits, including their hashes and messages:
git log
In the log output, look for the commit that introduced the unwanted changes. The hash will appear at the beginning of each commit line.
Executing the Git Revert Command With the commit hash in hand, use the following syntax for the `git revert` command:
git revert <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit you identified earlier. This action will create a new commit that undoes the changes made by the specified commit.
Committing the Changes After executing the revert command, it typically generates a commit message automatically, describing the revert. Ensure you push this revert commit to the remote repository to share your changes with your team:
git push origin <branch-name>
Replace `<branch-name>` with the branch you are working on (e.g., `main` or `develop`).
Example Scenario
Consider a situation where a developer has mistakenly pushed a feature that breaks the application. To remedy this, the developer would:
- Identify the buggy commit using `git log`.
- Execute the revert command with the committed hash:
git revert abc1234 # Replace with the actual commit hash
git push origin main
This straightforward process allows the developer to recover from the mistake while maintaining a clear project history.
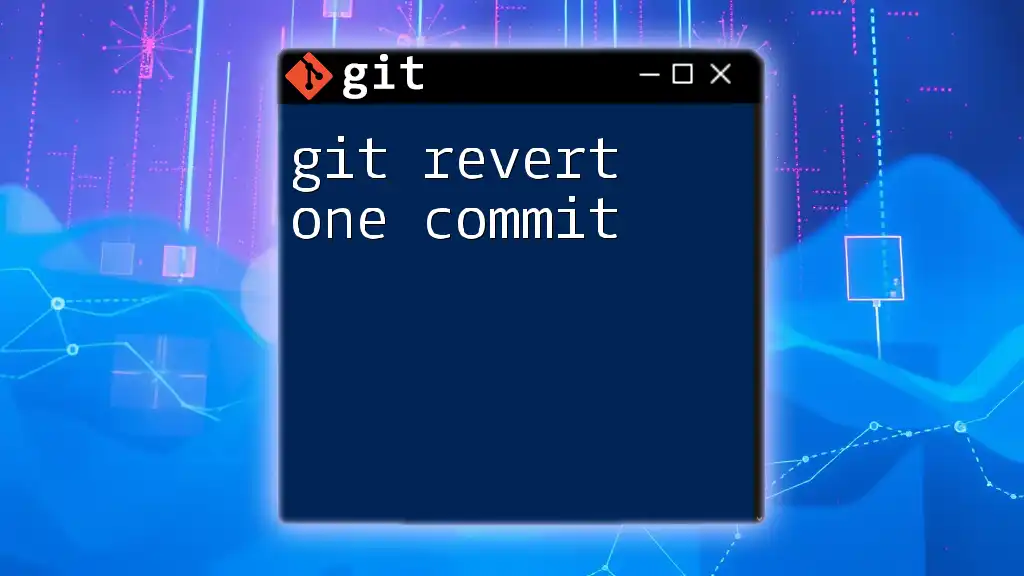
Common Issues and Troubleshooting
Potential Errors During Revert
Reverting a commit is generally a smooth process, but conflicts can arise. This occurs when changes in the revert conflict with other modifications made after the commit you are reverting. If you encounter a conflict, the following steps can help you resolve it:
- Run `git status` to identify which files have conflicts.
- Edit the conflicting files manually to resolve the issues.
- Stage the resolved files to mark them as fixed and continue with the revert:
git add <file-name>
git revert --continue
Best Practices After Reverting
After successfully reverting a commit, communication is crucial. Inform your team about the revert so everyone is on the same page regarding changes. Additionally, always double-check that you are operating on the correct branch before executing any revert command to avoid confusion.
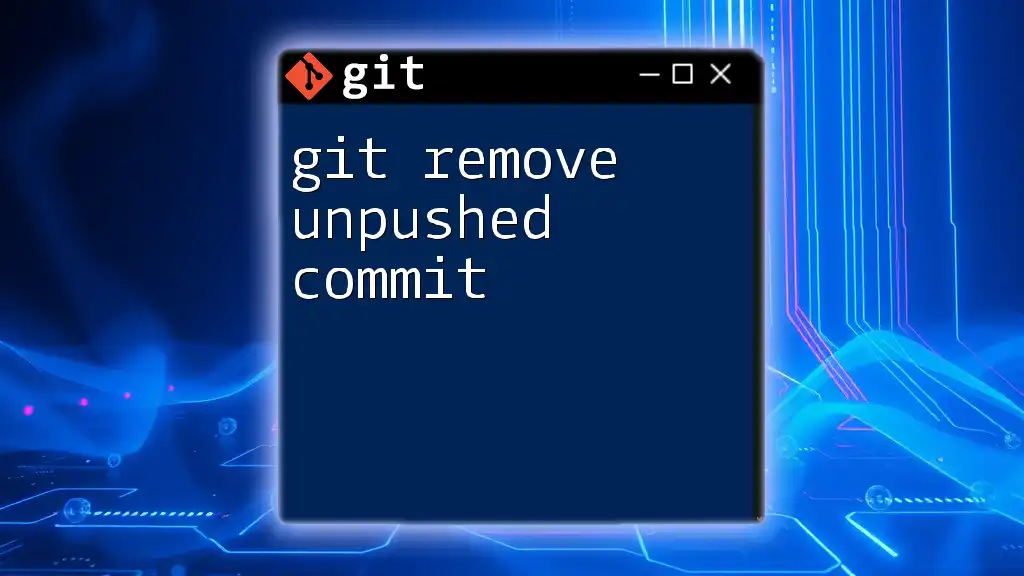
Alternatives to Git Revert
Using Git Reset vs. Git Revert
While `git revert` is designed to create a new commit to undo changes, `git reset` is a command that can alter your commit history by removing commits entirely from the branch. Understanding when to use each command is essential for effective version control management.
Pros and Cons of Each Command
- `git revert`:
- Pros: Non-destructive, maintains project history.
- Cons: Creates additional commit, may clutter history if overused.
- `git reset`:
- Pros: Can streamline history by removing unwanted commits.
- Cons: Potentially destructive, requires careful handling, especially in shared environments.
When to Use Each Command
Use `git revert` in collaborative settings where the integrity of the history is vital. Reserve `git reset` for personal or local branches, where you have the freedom to modify commit history without impacting other developers.
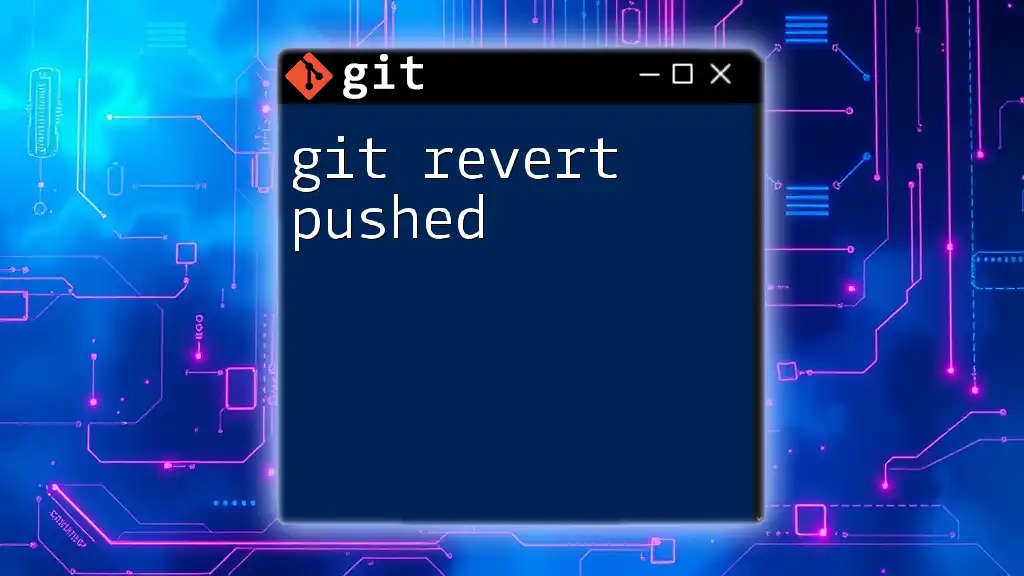
Conclusion
In this guide, we explored how to effectively revert a pushed commit using Git. From understanding the fundamentals of the `git revert` command to executing it in real scenarios, this skill is indispensable for any developer.
Practice this in a safe environment to build confidence. Being proficient with `git revert` not only enhances your Git capabilities but also promotes effective collaboration within your team.
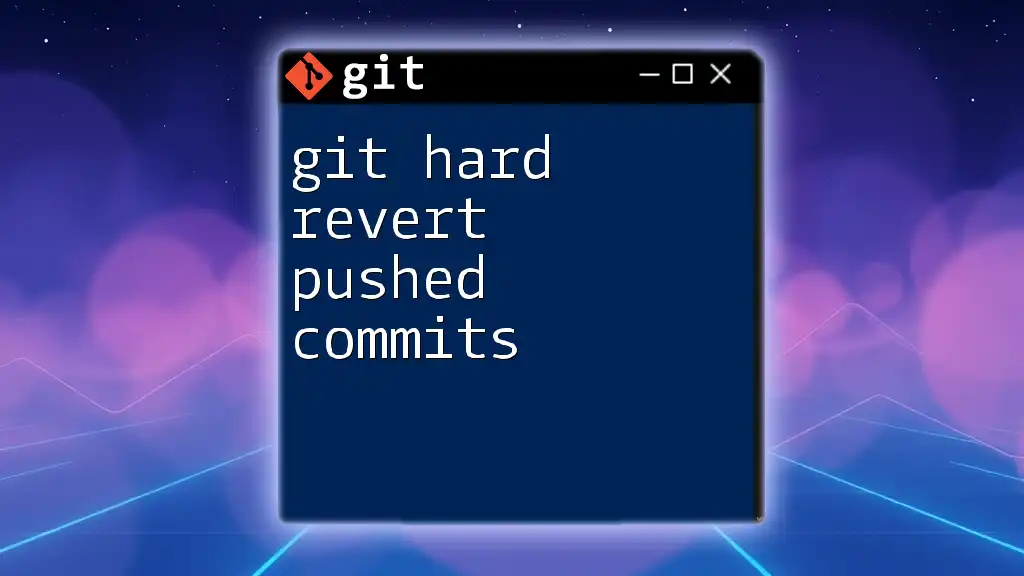
Additional Resources
For additional learning materials, explore reputable online articles, instructional videos, and the official Git documentation. Consider joining Git-focused communities to gain insights from fellow developers and continue expanding your knowledge in version control processes.