To remove an unpushed commit in Git, you can use the command `git reset` followed by the appropriate option to either keep changes in your working directory or discard them.
git reset HEAD~1
Understanding Git Commits
What is a Git Commit?
A commit in Git is a snapshot of your project at a particular point in time. Essentially, it captures the state of your files, allowing you to track changes, revert to previous versions, and maintain a history of your project. Each commit includes metadata such as the author's name, date, and a message describing the changes. This historical record is crucial for collaboration and understanding the evolution of a project.
What Does “Unpushed” Mean?
Unpushed commits are commits made to your local repository that have not yet been sent to the remote repository (e.g., GitHub, GitLab). Understanding this distinction is important because it influences how and why you might want to remove these commits. When you run the `git push` command, all your unpushed commits are shared with the remote repository, making them accessible to other collaborators. Before pushing, you have the flexibility to manage local changes without affecting the shared history.
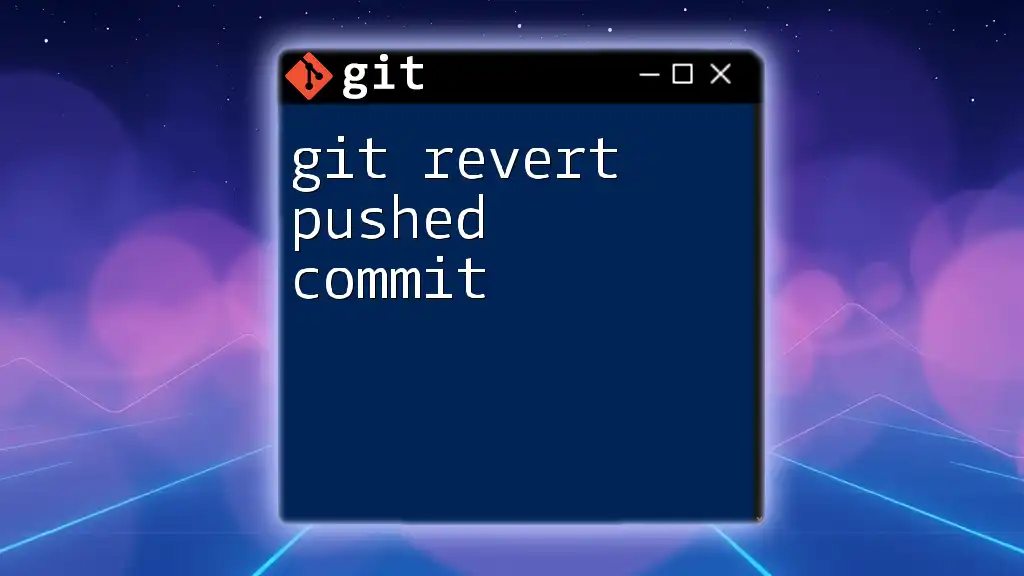
Reasons for Removing Unpushed Commits
Mistakes in Recent Commits
Many developers encounter situations where they need to remove unpushed commits due to mistakes made during the commit process. This could include:
- Accidental changes: Files that should not have been modified or included in the commit.
- Incorrect commit messages: Messages that fail to accurately describe the changes or are just poorly written.
- Unintended automation: Committing files that were automatically generated instead of manually edited.
Cleaning Up Commit History
A clean and readable commit history is vital for future maintainability and collaboration. When working in teams, retaining a clear narrative of changes allows others to understand decisions quickly. By removing unnecessary, confusing, or erroneous unpushed commits, you enhance the overall quality and utility of your project's commit log.
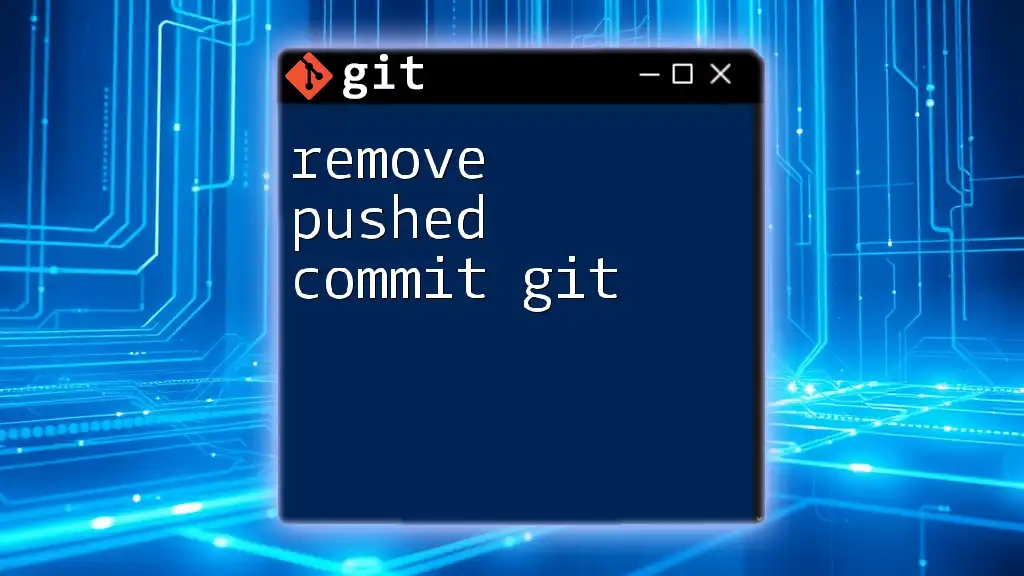
Methods to Remove Unpushed Commits
Using `git reset`
One of the most effective ways to remove unpushed commits is by using the `git reset` command. This command allows you to change the commit history based on your preferences. It's essential to understand the three types of reset:
- Soft Reset: Moves the HEAD pointer to a specified commit but leaves your changes staged.
- Mixed Reset: Moves the HEAD pointer and unstages changes—this is the default behavior of `git reset` without any options.
- Hard Reset: Moves the HEAD pointer and discards all changes in your working directory.
You can execute these commands as follows:
git reset --soft HEAD~1 # Moves the HEAD pointer but keeps changes staged
git reset --mixed HEAD~1 # Moves the HEAD pointer and un-stages changes
git reset --hard HEAD~1 # Moves the HEAD pointer and discards changes
When deciding which option to use, consider whether you want to keep your local changes (`--soft` and `--mixed`) or completely discard them (`--hard`).
Using `git revert`
Alternatively, you can use the `git revert` command if you'd prefer to create a new commit that undoes the changes made by a previous commit. This is particularly useful if you want to maintain a record of your actions without rewriting history.
For example:
git revert HEAD # Creates a new commit that undoes the last commit
This method is ideal for collaborative environments where tracking all changes is crucial, as it preserves the project's history while effectively nullifying the undesired commit.
Editing the Last Commit with `git commit --amend`
If you find that your last commit contains errors, instead of removing it entirely, you can modify it using `git commit --amend`. This command allows you to adjust the commit message or include additional changes.
Example usage:
git commit --amend -m "New commit message" # Amend the commit message
This approach is particularly beneficial when you realize that minor adjustments or a clearer message would greatly improve your commit's usefulness to others.
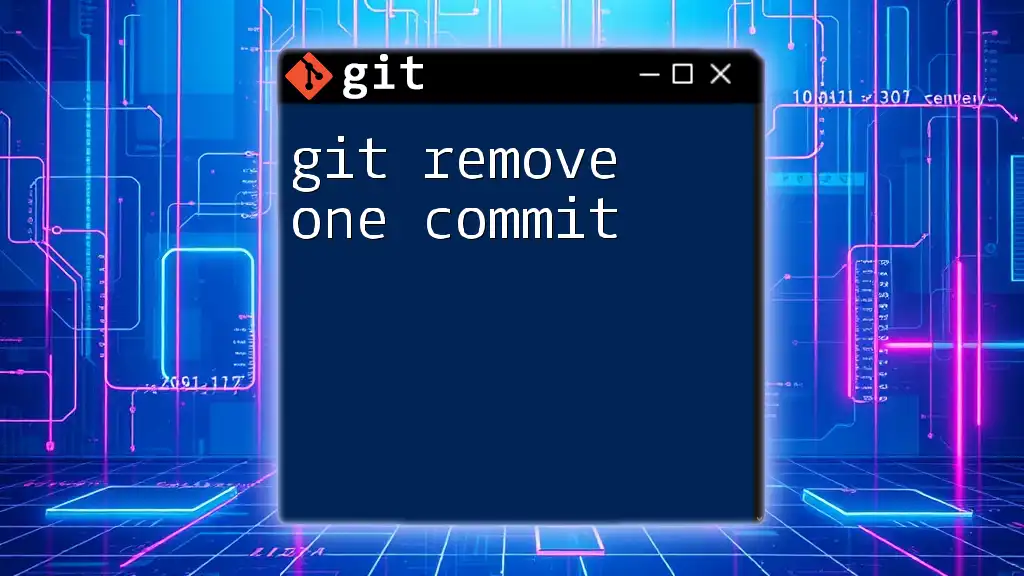
Best Practices for Managing Commits
Commit Often but Thoughtfully
Frequent commits are advantageous because they ensure you have a comprehensive history of your project. However, it's essential to be thoughtful. Each commit should encapsulate a logical chunk of work with a meaningful message, providing clarity on your intent.
Use Branches for New Features or Fixes
Utilizing branches to work on new features or fixes is one of Git's strengths. This allows you to isolate changes and minimizes the potential for unpushed commits to become problematic. Once your feature is stable, you can merge it back into the main branch, ensuring that the commit history remains organized.
Regularly Review Commit History
Make it a habit to periodically review your commit history using commands like `git log` and `git reflog`. These commands offer insights into your project progression, letting you see not only the commits you've made but also their effects. This routine can help identify unnecessary or incorrect commits before they become an issue.
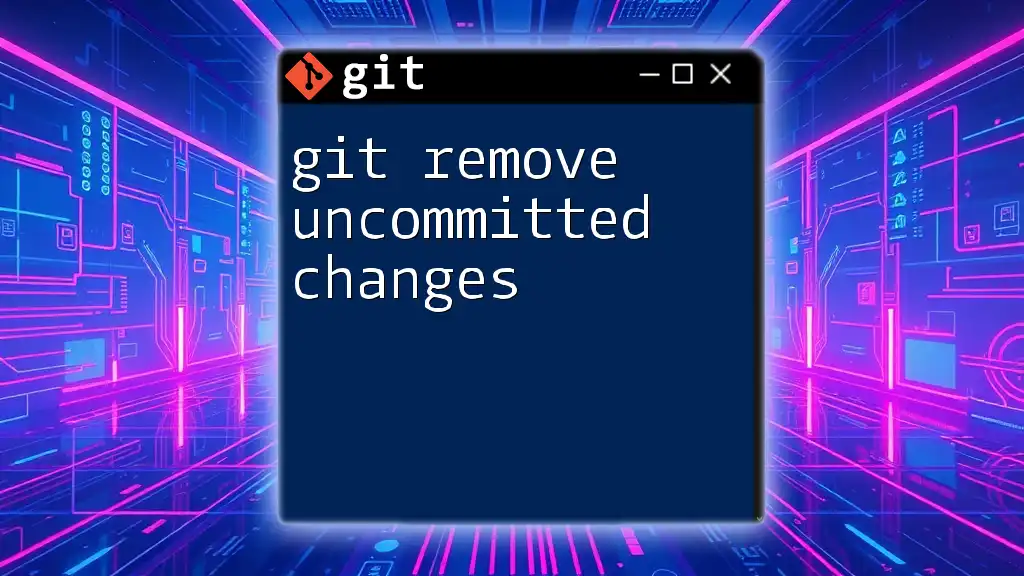
Conclusion
Navigating the process of how to git remove unpushed commit is crucial in maintaining a clean and effective commit history. Whether you're correcting mistakes with `git reset`, creating reversions through `git revert`, or making amendments with `git commit --amend`, mastering these commands greatly enhances your Git proficiency.
Maintaining a coherent commit history fosters collaboration and simplifies future development. Practice these strategies regularly to reinforce your understanding and elevate your version control skills. Ultimately, a well-managed commit history contributes to a more successful project and a more efficient development workflow.
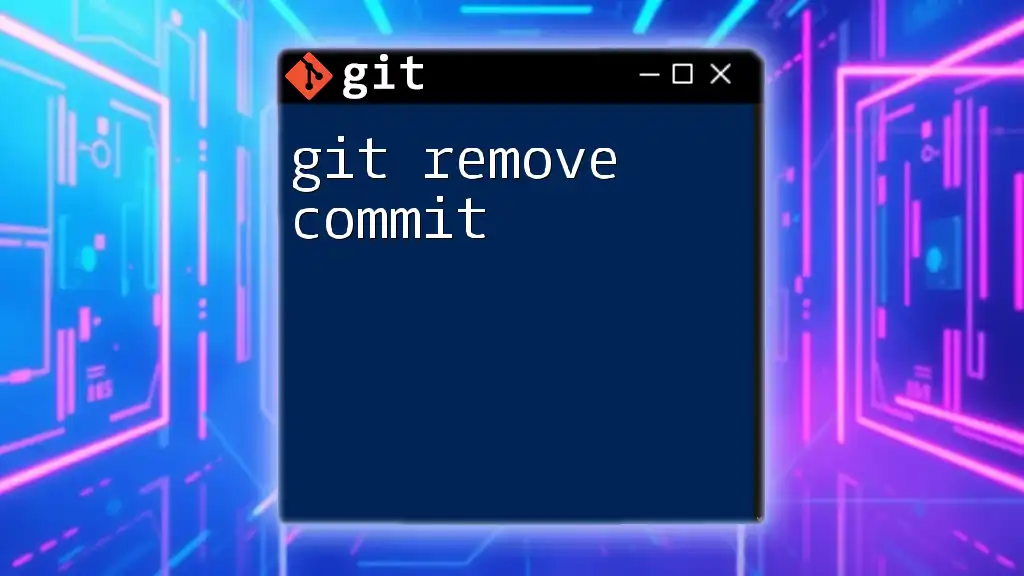
Additional Resources
Useful Commands Cheat Sheet
Familiarize yourself with these essential Git commands to enhance your toolkit:
- `git log`: View the commit history.
- `git reflog`: View a reference log of your actions.
- `git status`: Check the state of your working directory.
Recommended Tools and References
Explore additional resources tailored to improve your Git knowledge, including official documentation, tutorials, and community forums. These resources will provide in-depth insights as you continue your Git journey.