To remove untracked files (i.e., files that are not added to the Git index) from your working directory, use the following command:
git clean -f
What Are Unadded Files?
Unadded files refer to those files that exist in your working directory but have not been staged for a commit in Git. Specifically, these files can fall into two main categories:
New Files
These are files you have created in your project but have not yet told Git to track. Because they are not part of the Git repository, they won't be included in any commits until you explicitly stage them.
Modified Files
These are files you have altered in some way after having been added to Git’s tracking system, but you haven’t staged those changes for the next commit.
Importance of Managing Unadded Files
It's crucial to manage unadded files for two major reasons:
- Project Cleanliness: Excess unadded files can clutter your working environment, making it difficult to track important changes. A clean working directory contributes to a streamlined workflow.
- Risk of Accidental Commits: Committing unadded files inadvertently can lead to including unwanted data in your project history. This makes it harder to maintain a clean project environment.
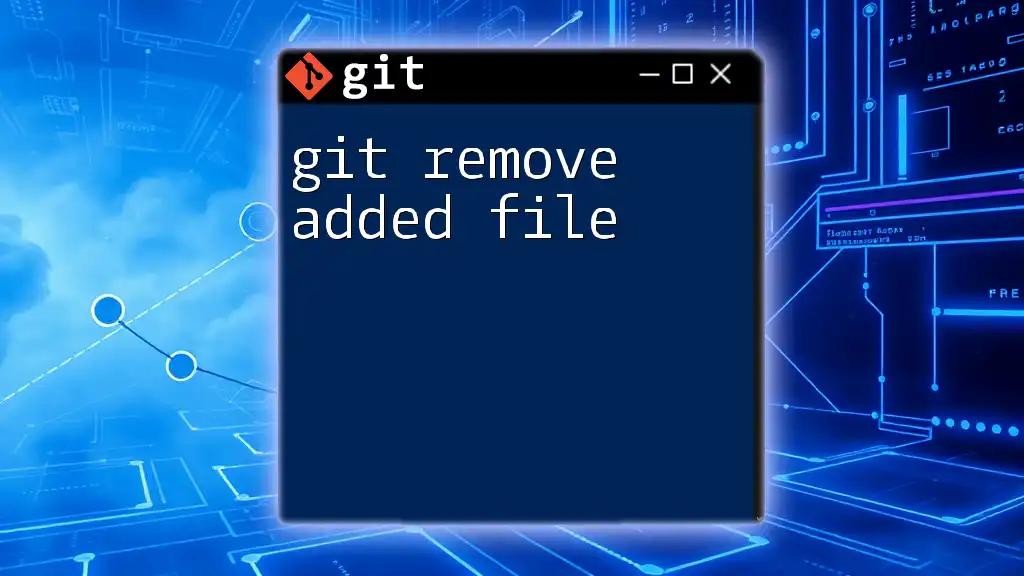
How to Identify Unadded Files
The best way to identify unadded files is by using the `git status` command, which provides detailed information about the current state of your working directory.
To check for unadded files, you can run the following command:
git status
The output of this command will list files in different states, including:
- Untracked files: These are the new files you want to see removed.
- Changes not staged for commit: Any modified files that need to be managed.
Understanding this output is essential to successfully perform clean-up actions on your project.
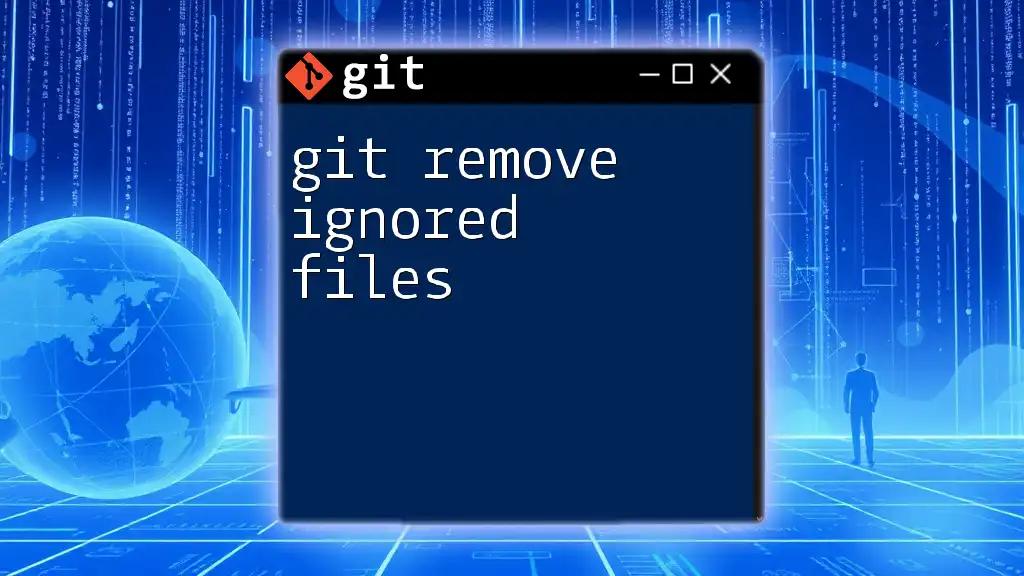
Methods to Remove Unadded Files
Removing unadded files can be done through a few methods, mainly focusing on soft removal and hard removal.
Soft Removal
If you want to temporarily set aside your changes without actually committing them, you can use the `git stash` command. This method allows you to keep your workspace tidy while preserving your changes for later use.
You can stash changes as follows:
git stash push -m "Temporary save"
This command allows you to manage changes without permanently losing them, creating a clean slate in your working directory.
Hard Removal
If you’re looking to clean up your project extensively, the `git clean` command is your best bet. This command deletes files that are not tracked by Git.
Introduction to Git Clean
The `git clean` command removes untracked files from your working directory. Be careful when using it, as it will permanently delete these files.
You can execute a basic clean with:
git clean -f
This command will remove all untracked files in the current directory, which means they will be permanently deleted.
Explanation of Flags
- The `-f` option is crucial; it stands for force, which you must include to avoid accidentally deleting files.
- If you want to remove untracked directories as well, you can add the `-d` flag:
git clean -fd
This command ensures not just the files but also any untracked directories are removed, allowing for thorough cleaning.
Remove Specific Unadded Files
In some instances, you may not want to remove all unadded files. The `-e` flag allows you to exclude certain patterns when using `git clean`.
For example, if you want to remove everything but `.log` files, run:
git clean -f -e "*.log"
This command ensures that the specified file types remain untouched while all other untracked files are removed.
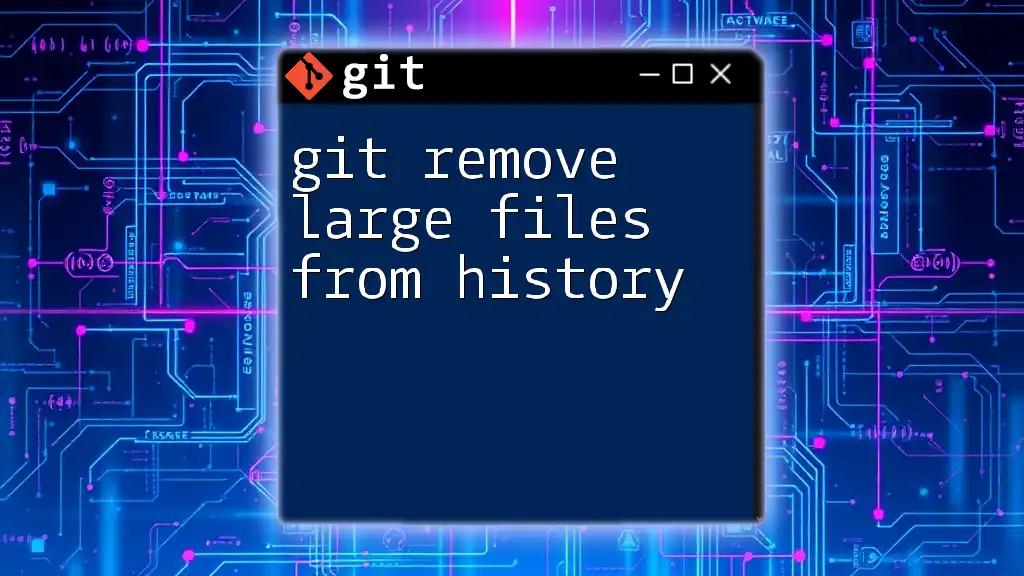
Best Practices for Managing Unadded Files
Regular Maintenance
Implement routine checks on your Git repository. Developing a habit of running `git status` before each commit can help catch unadded files early. This practice helps ensure that only the desired changes are committed to your project history.
Utilizing .gitignore
Another best practice is to use a `.gitignore` file effectively. This file instructs Git on which files or directories it should ignore.
Creating a `.gitignore` file is straightforward. Simply create a new file named `.gitignore` in your project’s root directory. You can specify patterns for files you want Git to ignore, such as:
*.log
temp/
Utilizing a `.gitignore` file greatly reduces the chances of unadded files cluttering your working directory or accidentally getting committed.
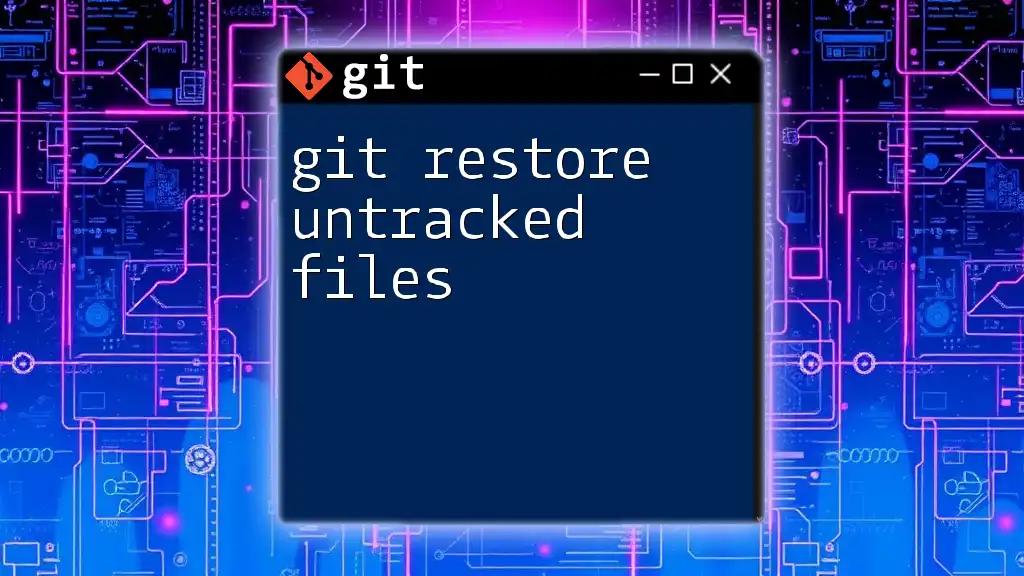
Conclusion
By understanding how to remove unadded files in Git, you can maintain a cleaner, more organized project environment. Regularly checking for and managing these unadded files will improve not only your workflow but also the integrity of your project history.
Make it a practice to implement these methods and best practices as part of your Git routine, and always stay updated on more advanced Git functionalities to further improve your coding experience.