To delete tracked files in Git, use the `git rm` command followed by the filename, which removes the file from the staging area and working directory.
git rm filename.txt
Understanding Git's File Tracking System
What are Tracked Files?
Tracked files are files that have been added to the staging area in Git and are being monitored for changes by the version control system. Unlike untracked files, which Git does not manage, tracked files undergo the process of staging and committing, allowing developers to revert, merge, or modify them efficiently. Understanding the concept of tracked files is crucial, as it helps maintain a clean and organized repository.
How Git Tracks Files
Git maintains a three-part structure consisting of the working directory, staging area, and repository. When you modify files in your working directory, they remain untracked until you tell Git to start monitoring them. This is done using commands such as `git add`, which stages the files, preparing them for a commit. Once staged, files become tracked, meaning Git keeps a history of all changes, enabling collaboration and version control across projects.
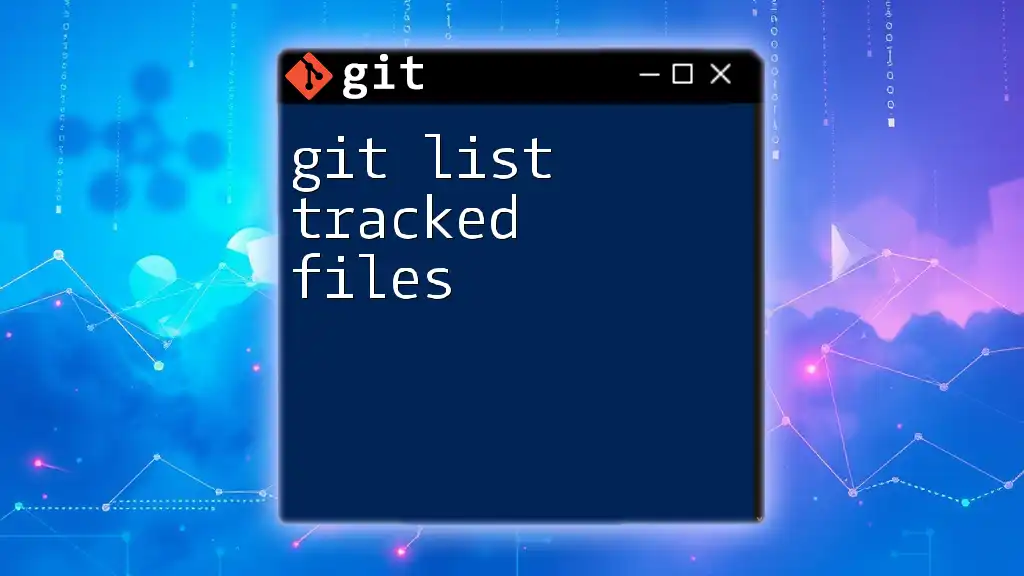
Reasons to Delete Tracked Files
Cleaning Up Your Repository
Over time, repositories can become cluttered with unnecessary files. Keeping your project organized not only enhances performance but also improves collaborative efforts with others. By regularly deleting files that are no longer needed, you contribute to a cleaner and more manageable repository.
Mistakenly Added Files
Sometimes, files may be added to the Git framework accidentally. This could include temporary files, compiled binaries, or other artifacts that should not be versioned. Removing these files is essential to maintaining a clean project structure and ensuring that only relevant files are committed to your repository.
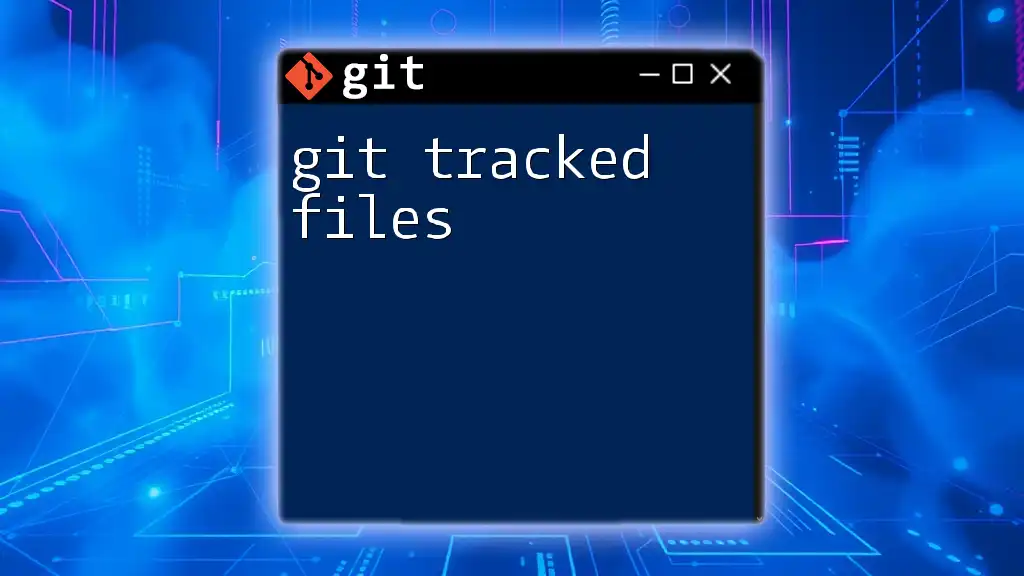
Methods for Deleting Tracked Files
Using the `git rm` Command
The primary command for deleting tracked files in Git is `git rm`. This command immediately removes the specified file from both the working directory and the staging area.
To use `git rm`, the syntax is quite straightforward:
git rm <file-name>
Example: Deleting a Single Tracked File
Suppose you have a file named `unwanted-file.txt` that you want to delete. The command you would use is:
git rm unwanted-file.txt
Upon execution, you will receive a confirmation that the file has been removed. It’s essential to note that this action cannot be easily undone unless you commit your changes.
Example: Deleting Multiple Tracked Files
You can efficiently delete multiple files in a single command. For instance, if you wish to remove `file1.txt`, `file2.txt`, and `file3.txt`, you could use:
git rm file1.txt file2.txt file3.txt
This command expedites the cleanup process, particularly when handling numerous files at once.
Removing Files from Staging Without Deleting
If you wish to untrack a file but keep it in your working directory, you can do this by using the `--cached` option with `git rm`. This command removes files from the staging area without deleting them from your local disk.
The syntax for this operation is:
git rm --cached <file-name>
Example: Untracking a File without Deleting
Imagine you have a file named `config.yml` that you don't want to track in Git anymore. To untrack it while retaining it on your filesystem, you would run:
git rm --cached config.yml
This is particularly useful for configuration files or sensitive data that should not be shared in a repository.
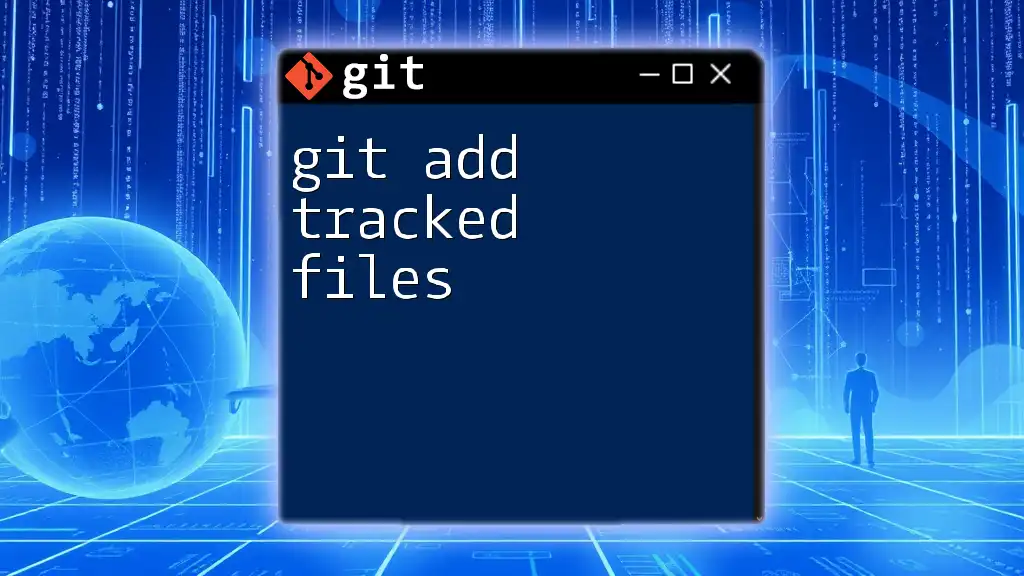
Using Git to Ignore Files
Importance of Git Ignore
In many projects, certain files and directories do not need to be tracked by Git. This may include log files, compiled code, and personal IDE settings. In such cases, utilizing a `.gitignore` file helps streamline the tracking process by preventing specific files or patterns from being added to the repository.
Creating a `.gitignore` File
Creating and maintaining a `.gitignore` file is essential for managing the files that Git should ignore. To set up a `.gitignore`, simply create a new file named `.gitignore` in the root directory of your Git repository.
For example, to ignore all `.log` files and a specific configuration file, your `.gitignore` should look like this:
# Ignore all .log files
*.log
# Ignore specific files
secret-config.yml
By specifying patterns in this file, you can prevent unwanted files from being tracked in your repository, making future deletions unnecessary.
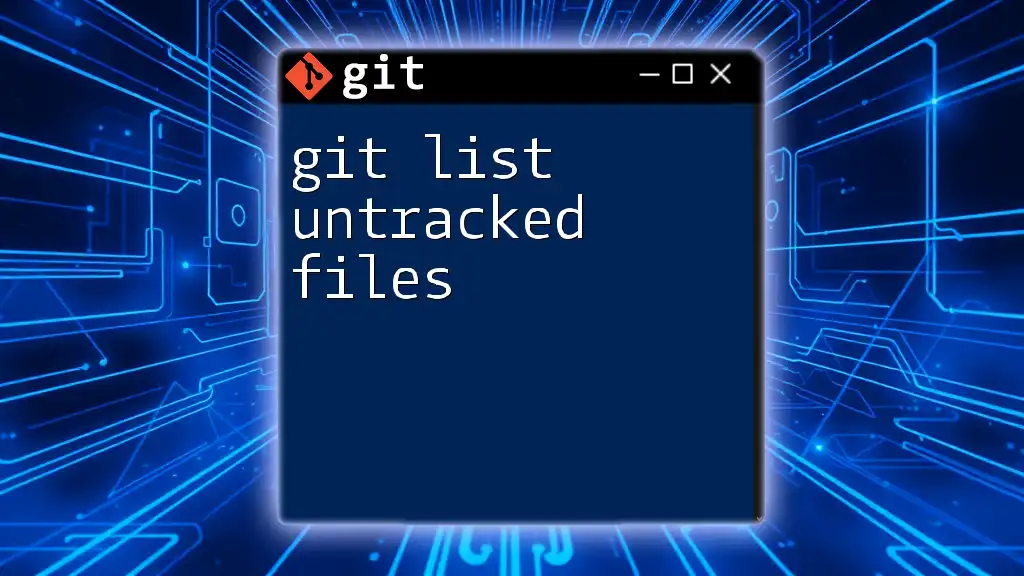
Best Practices for Deleting Tracked Files
Always Double Check Before Deleting
Before executing any deletion commands, it’s vital to review which files you are about to remove. A simple mistake can lead to the permanent loss of important files. Using the command `git status` prior to deletion can help you verify that you are only removing what’s necessary.
Committing Changes After Deletion
Once you’ve deleted the tracked files, don’t forget to commit your changes. This action ensures that your repository reflects the updated state without the unwanted files. When writing your commit message, clarity is key. A good message might look like:
git commit -m "Remove unnecessary tracked files"
Committing after deletions helps to maintain an up-to-date and clean commit history.
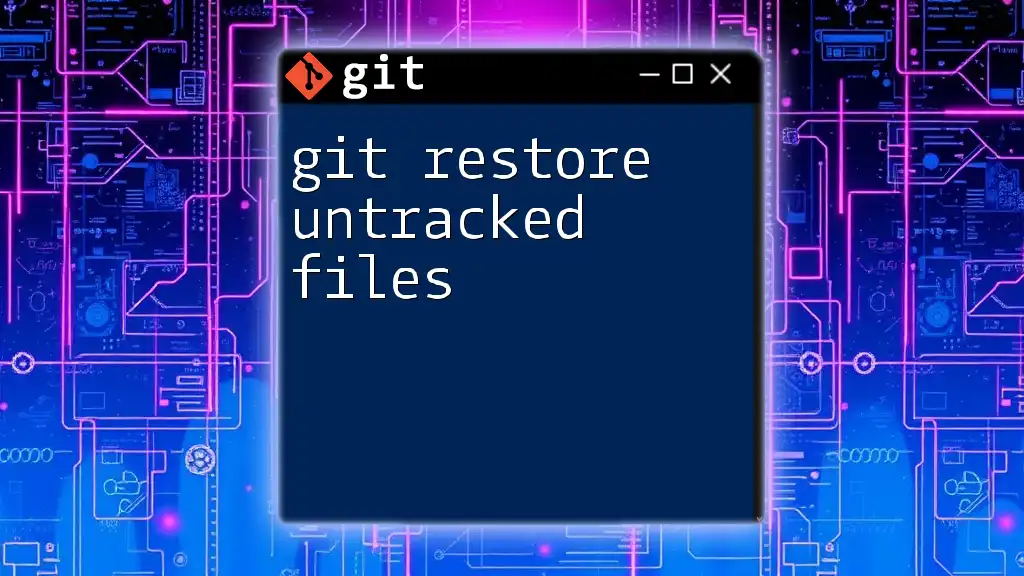
Conclusion
In summary, knowing how to git delete tracked files efficiently is an essential skill for anyone using Git. By understanding tracked files, the methods for deletion, and best practices, you can maintain a clean and organized repository, simplifying the version control process. Regular practice with these commands will enhance your Git proficiency and contribute to more efficient project management.