To list untracked files in a Git repository, use the command `git ls-files` with the `--others` and `--exclude-standard` options to display only those files that are not being tracked by Git.
git ls-files --others --exclude-standard
Understanding Untracked Files
What are Untracked Files?
Untracked files in Git refer to files that have been created in your working directory but are not being tracked by Git. This means that these files have not been staged for commit and will not be included in version history unless explicitly added.
It is crucial to understand the distinction between tracked and untracked files. Tracked files are those that are monitored by Git, meaning any changes made to them can be staged and committed to the repository. In contrast, untracked files are essentially ignored by Git until they are added to the staging area.
Why You Need to List Untracked Files
Regularly listing untracked files plays a vital role in maintaining a clean working directory. Untracked files can clutter your development environment, making it difficult to focus on the files that matter most. Identifying these files is essential for several reasons:
- Adding Necessary Files: By reviewing untracked files, developers can determine which files need to be added to version control, thus ensuring that no important changes are left overlooked.
- Avoiding Accidental Commits: Being aware of untracked files helps prevent accidental commits of temporary or sensitive files that should not be included in the repository.
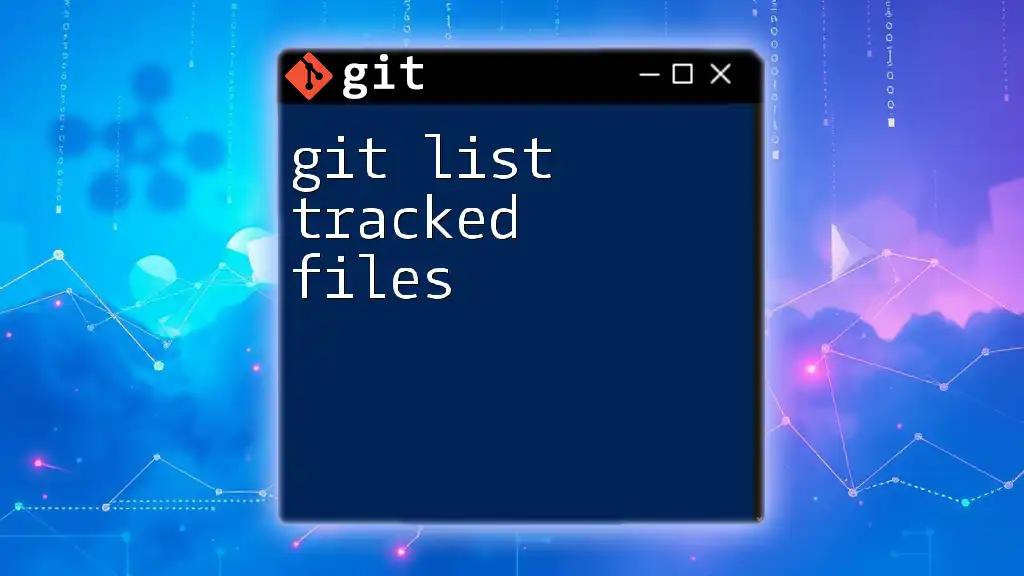
How to List Untracked Files in Git
Using `git status`
The most straightforward way to see untracked files is by using the `git status` command. This command provides a summary of the current state of your working directory and staging area.
When you run the command:
git status
You will get an output that may look something like this:
On branch main
Your branch is up to date with 'origin/main'.
Untracked files:
(use "git add <file>..." to include in what will be committed)
file1.txt
directory/file2.txt
In this output, files prefixed with "Untracked files:" indicate those that are not currently tracked by Git. Identifying these files allows you to decide whether to add them to tracking or to leave them untracked.
Using `git ls-files` Command
Another effective way to list untracked files is by using the `git ls-files` command, which provides greater specificity in its output. To list only untracked files, use the following:
git ls-files --others --exclude-standard
Here's a breakdown of the options used in this command:
- `--others`: This option displays untracked files.
- `--exclude-standard`: This option excludes files specified in the `.gitignore` file from the output.
The output will show you a list of untracked files clearly, making it easy to manage them as needed.
Alternative Methods to List Untracked Files
Using `git add -n`
Another useful command is `git add -n`, which can be employed to preview what will be added if you run `git add`. This is particularly useful for listing untracked files:
git add -n .
Running this command will show you which files would be staged for commit without actually staging them. This provides a clear insight into untracked files, making it an excellent option for verification.
Using `.gitignore` to Exclude Files
Utilizing a `.gitignore` file is a common practice to specify files and directories that Git should ignore. By reviewing your `.gitignore` settings, you can understand which files are being deliberately excluded from tracking, thus clarifying the untracked files list.
Mistakes in your `.gitignore` can lead to confusion regarding why certain files remain untracked. For example, a simple `.gitignore` might look like this:
*.log
node_modules/
*.tmp
This tells Git to ignore all `.log` files, the `node_modules` directory, and temporary `.tmp` files. Understanding how this affects file tracking helps maintain clarity in your project.
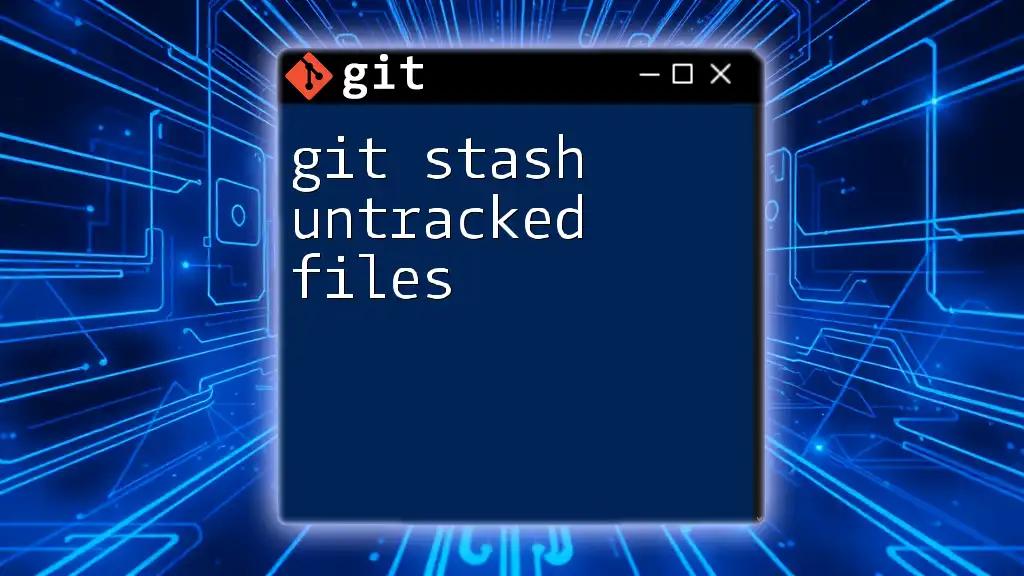
Practical Use Cases
In a New Project
When starting a new Git repository, you’ll likely encounter a number of untracked files. It’s essential to ensure that your initial commit includes all necessary files. After reviewing your project structure, you can use `git status` to check for any untracked files, helping you establish a solid foundation.
After confirming which files should be tracked, add them using:
git add <file-name>
This proactive approach sets the stage for effective version control from the start.
In an Ongoing Project
In an ongoing project, regularly checking for untracked files enables developers to keep their workspace organized. This helps eliminate missed files that could be critical to the project’s success or lead to issues down the line.
Routine usage of the command `git status` can foster good practices, allowing you to remain aware of evolving project needs.
Case Study: A Real-World Example
Imagine a scenario where a developer, while working on a feature, inadvertently created temporary files that were never meant to be pushed to the repository. By regularly listing untracked files, they identified unnecessary files before making a commit, thereby avoiding potential pitfalls. By temporarily using `.gitignore` to exclude certain paths, they maintained control over their project’s repository and preserved its integrity.
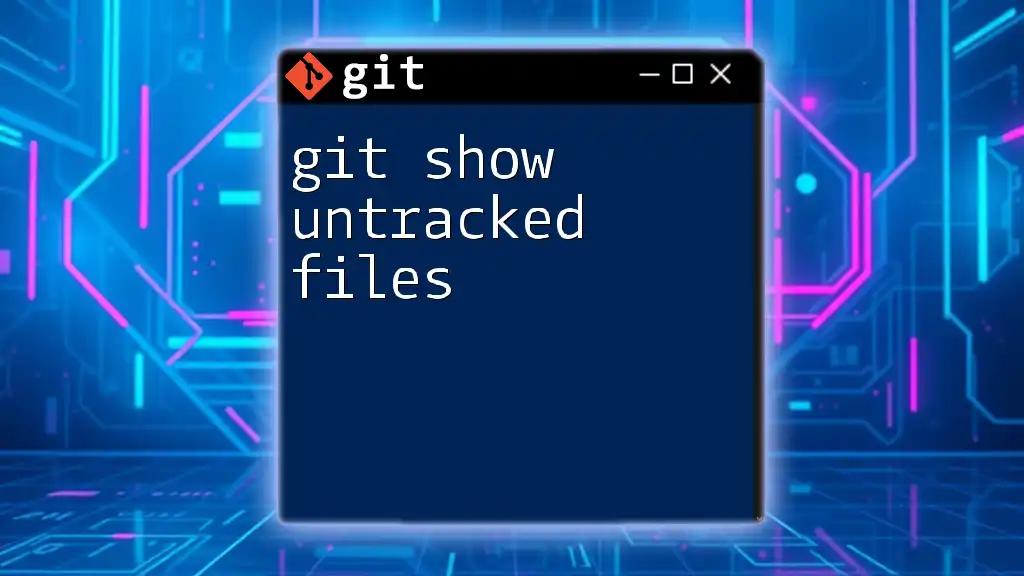
Troubleshooting Common Issues
Files Not Showing as Untracked
If you notice that certain files are not appearing as expected in your untracked files list, several factors could be at play:
- Files Are Already Tracked: If the files were previously added and committed, they will not appear as untracked.
- Inclusion in `.gitignore`: Verify the contents of your `.gitignore`. Included files will be ignored by Git.
Accidental Inclusion of Untracked Files in Commits
To avoid the unintentional inclusion of untracked files in your commits, familiarize yourself with using `git add` cautiously. For additional safety, consider utilizing `git stash` to temporarily save changes, allowing you to commit other parts of your work while keeping certain files safely separated.
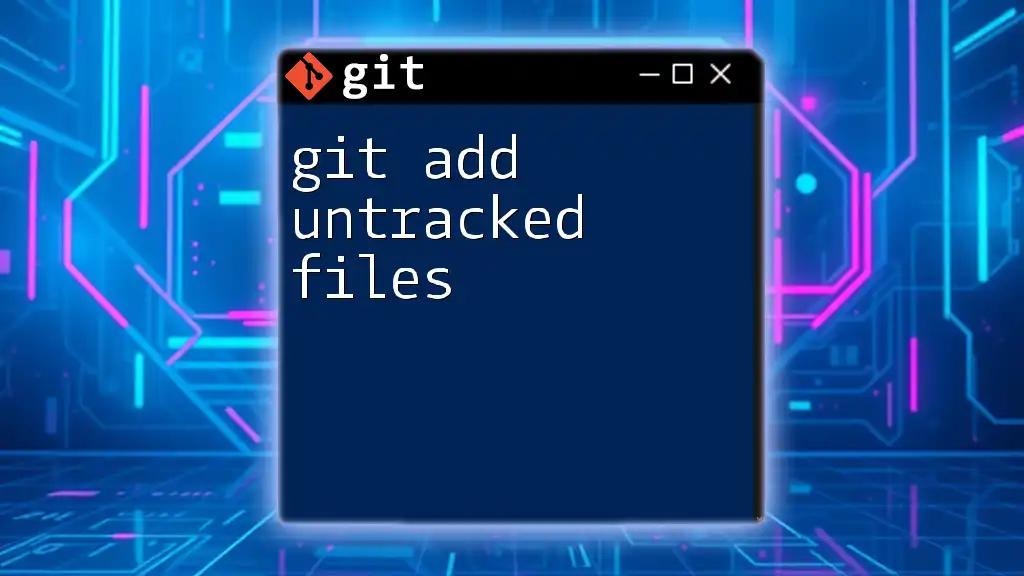
Conclusion
Managing untracked files is an essential part of working with Git. By understanding how to effectively list untracked files and recognizing their importance, developers can maintain a clean and organized working environment. Regular practice of the commands discussed will empower you to better manage your projects and prevent unwanted complications. Embrace these practices, and you’ll find that effective storage of your project files will lead to a smoother development process.
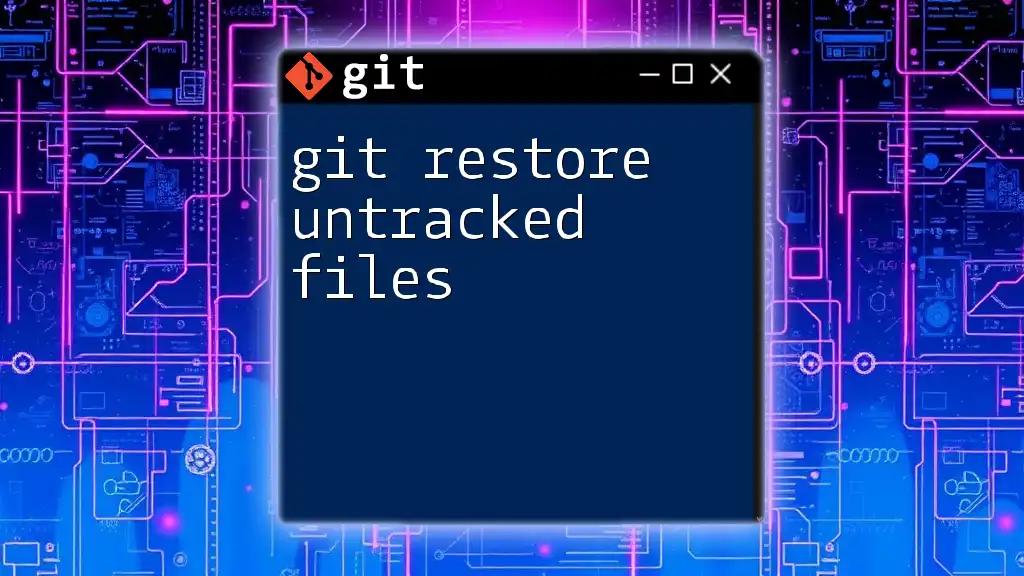
Additional Resources
For further learning, check out the official Git documentation, where you'll find more in-depth discussions about commands, configurations, and best practices. You may also explore tools that enhance the usability of Git in various development environments, helping you streamline your experience.
Appendices
Common Git Commands Cheat Sheet
A quick reference to essential Git commands includes:
- `git init`: Initialize a new Git repository
- `git add`: Stage changes for commit
- `git commit`: Commit staged changes
- `git pull`: Update your local repository from the remote
- `git push`: Upload changes to a remote repository
- `git status`: Display the current state of the working directory
FAQ Section
- What do I do if I accidentally committed the wrong untracked file? You can use `git reset HEAD~1` to undo the last commit, staging the changes back for review.
- Can I view untracked files in a specific directory? Yes, you can navigate to a specific directory and run the commands like `git status` or `git ls-files` from there to filter the results.
By mastering these concepts and commands, you'll become adept at managing untracked files, thus enhancing your overall productivity and collaboration within your development team.