To untrack a file in Git while keeping it in your working directory, you can use the `git rm --cached` command followed by the filename. Here's a code snippet to illustrate:
git rm --cached filename.txt
Understanding Git Tracking
What is Tracking in Git?
In the context of Git, tracking refers to the process by which Git monitors changes made to files in a repository. When you add a file to a Git repository, it becomes tracked, meaning Git keeps a record of changes made to that file over time. This tracking allows you to utilize Git's version control functionalities, such as committing changes, viewing history, and reverting to previous states.
Why Would You Want to Untrack a File?
Untracking a file may seem counterintuitive when you first think about it, but there are several scenarios where this action is essential:
-
File Size Considerations: Large files can bloat your repository. When you untrack these files, you prevent them from taking up additional space in your Git history.
-
Sensitive Information Management: Occasionally, you may inadvertently add sensitive files (like API keys or passwords) to your repository. Untracking these files is crucial to ensure that they don’t get pushed to remote repositories.
-
Project Management and Clutter Reduction: Keeping tracked files to a minimum helps in maintaining a cleaner project environment. Removing unneeded files from tracking allows you to focus on what is essential.
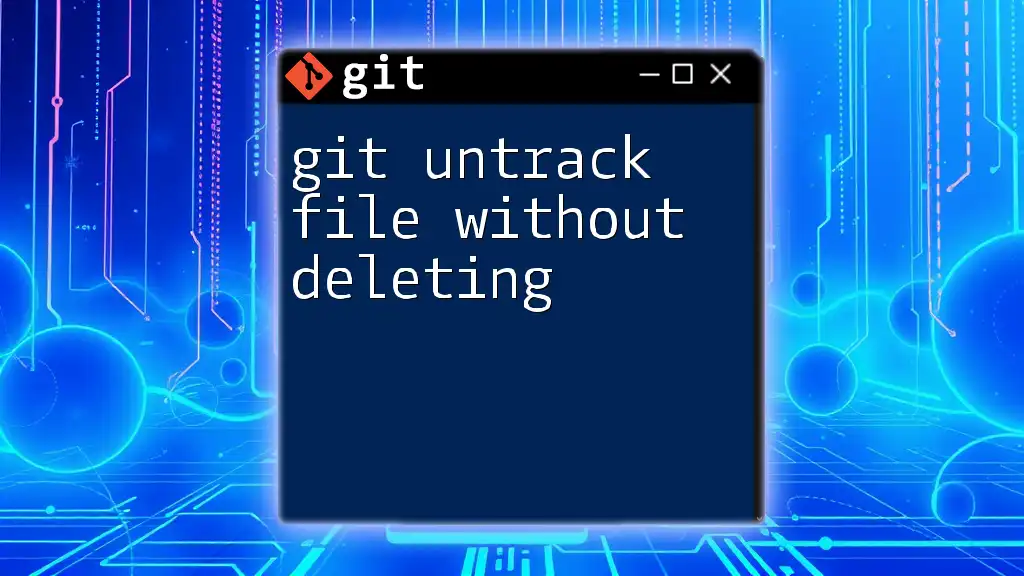
The Basics of Untracking Files in Git
Using `.gitignore` to Untrack Files
A common approach to untracking files in Git is the use of a `.gitignore` file. This file tells Git which files or directories to ignore in the repository. Here’s how it works:
-
To use `.gitignore`, create this file in the root of your repository if it doesn’t already exist.
-
Adding Files to `.gitignore`: Simply list the files or patterns you want to ignore. For example, if you want to ignore a file named `config.yml`, you would add it like this:
config.yml
After adding files to `.gitignore`, remember that it will not untrack files that have already been committed. Therefore, further steps are necessary if you want to untrack a file that has already been tracked.
Untracking a File with `git rm --cached`
The command `git rm --cached` is a powerful tool for untracking files from your Git repository. This command removes a file from Git’s index but leaves the file itself intact in your working directory, allowing you to keep your local copy.
When to Use this Command: Use this when you want to remove tracked files but don’t want to delete them from your local storage. For example, if you mistakenly added a large log file to your repository and wish to untrack it:
git rm --cached large-log-file.log
After running this command, it's essential to commit the changes to complete the untracking process:
git commit -m "Untrack large-log-file.log"
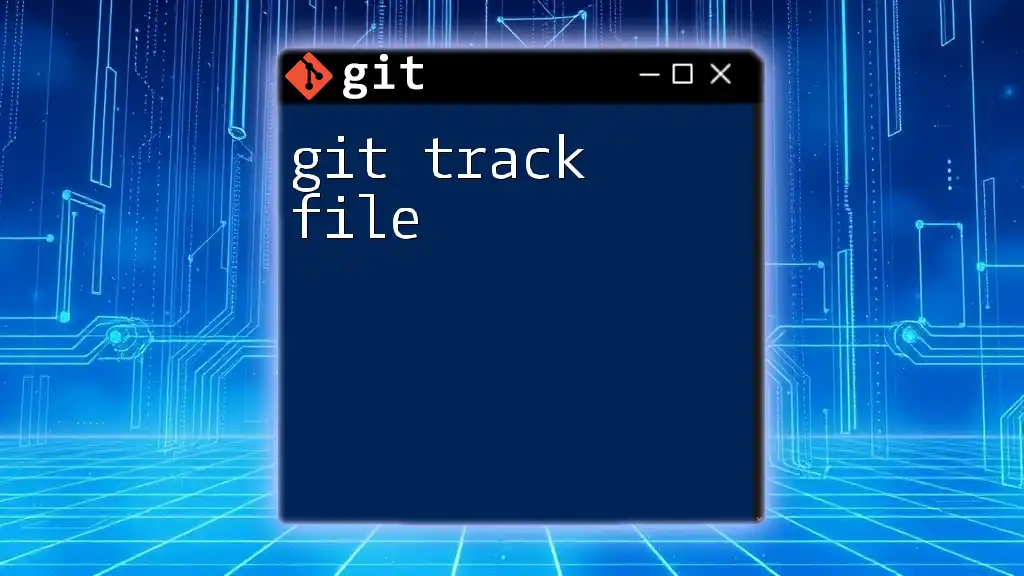
Practical Examples of Untracking Files
Scenario 1: Untracking a Configuration File
Consider a scenario where you've added a `secrets.json` file containing sensitive configuration details to your repository. Here’s how to untrack it securely:
- Add `secrets.json` to your `.gitignore`:
echo "secrets.json" >> .gitignore
- Untrack the file with:
git rm --cached secrets.json
- Commit the changes:
git commit -m "Untrack secrets.json and update .gitignore"
Scenario 2: Untracking Compiled Binary Files
Another typical situation involves untracking compiled binary files that do not belong in the repository. If you've accidentally added a binary file such as `app.exe`, you can swiftly untrack it:
- Add `app.exe` to the `.gitignore`:
echo "app.exe" >> .gitignore
- Untrack with:
git rm --cached app.exe
- Commit the action:
git commit -m "Untrack app.exe and update .gitignore"
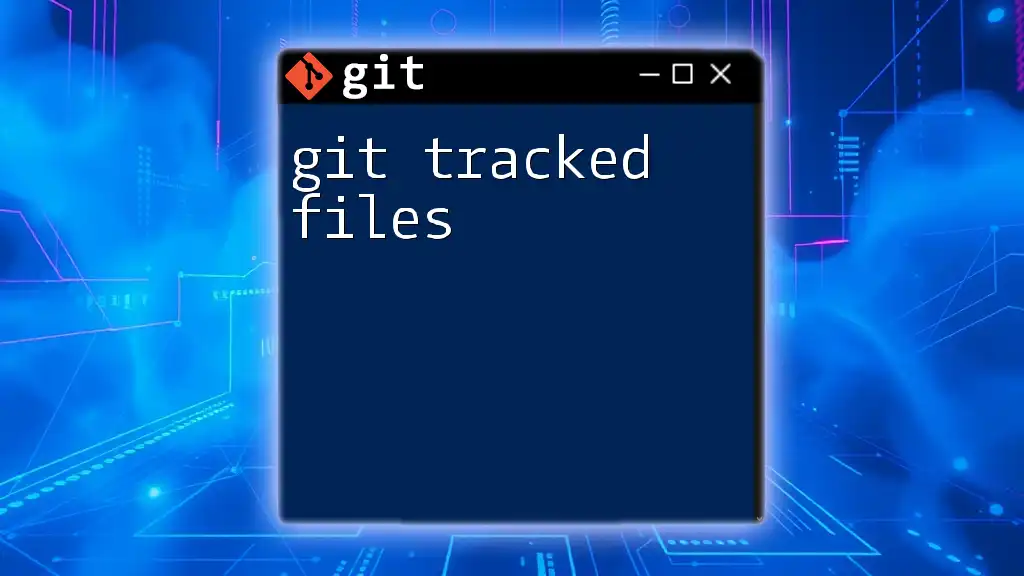
Best Practices for Untracking Files
Setting Up a Proper `.gitignore` File
Creating an effective `.gitignore` file is crucial for managing which files should not be tracked by Git. Here are some recommended practices:
-
Keep It Updated: Regularly review and update the entries in your `.gitignore` file as your project evolves.
-
Use Patterns and Wildcards: This allows you to ignore multiple files that match criteria, such as:
*.log # Ignore all .log files
tmp/ # Ignore all files in the tmp directory
Regular Auditing of Tracked Files
Maintaining a clean project requires regular audits of tracked files. You can use the following Git commands to analyze which files are currently tracked:
git ls-files
This command will output a list of all tracked files, making it easy to identify which files you may want to untrack.
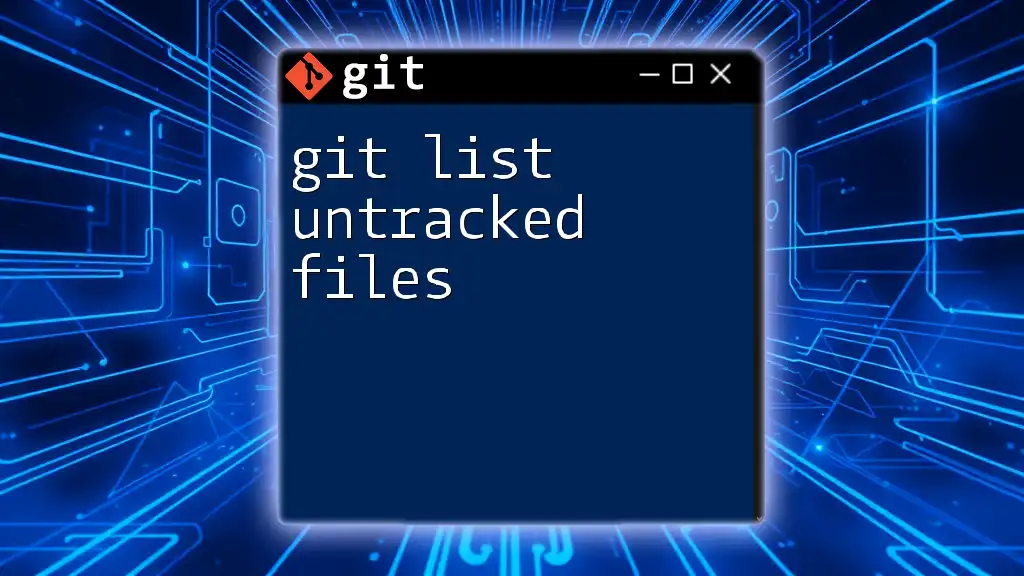
Common Mistakes When Untracking Files
Forgetting to Commit Changes
One common error users make is forgetting to commit their changes after untracking files. Without committing, the untracked status will not be saved. Always remember:
git commit -m "Commit message reflecting changes"
Misusing `.gitignore` for Already Tracked Files
A widespread misconception is that adding a file to `.gitignore` untracks it automatically. In reality, `.gitignore` only affects untracked files. For tracked files, you must use `git rm --cached`.
Failing to Communicate Changes with Team Members
When working on a project with multiple collaborators, it’s essential to communicate any changes you make to `.gitignore` and untracked files. Failing to do so can result in confusion and unwanted behaviors when others try to track files you’ve intentionally left out.
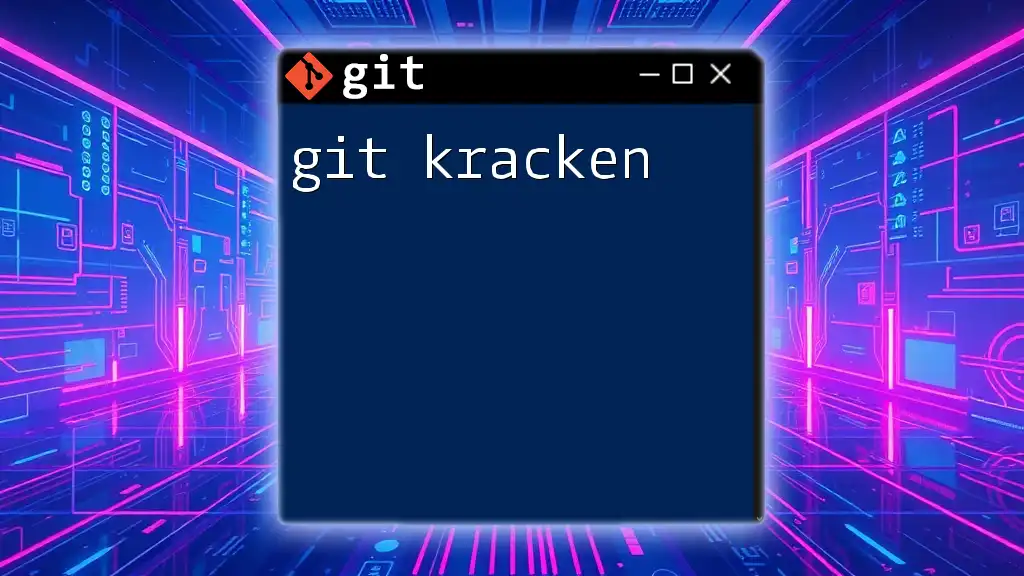
Conclusion
Understanding how to git untrack file is vital for effective version control. By managing your tracked files properly, you can keep your repository clean, secure sensitive information, and improve collaboration with team members. Implement best practices, communicate with your team, and regularly audit your files for optimal results.
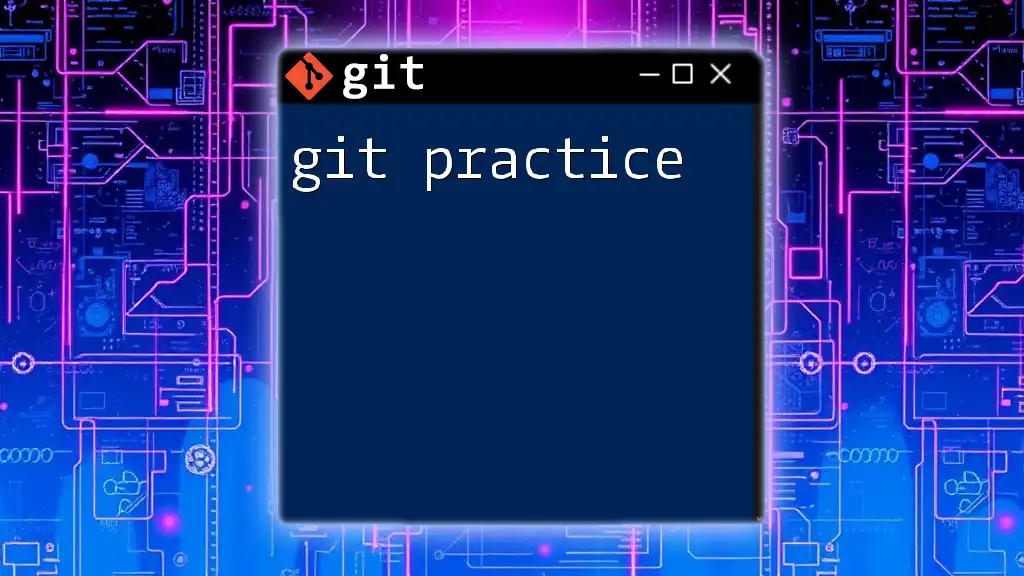
Additional Resources
Recommended Reading
For further knowledge, you can explore the [Git official documentation](https://git-scm.com/doc). Complementing this knowledge with additional articles on Git usage and project management techniques will enhance your understanding.
Join the Conversation
We invite you to share your experiences with untracking files in Git or ask any questions you might have. Engaging with our community can provide valuable insights and tips on mastering Git commands.