Git tracked files are files that Git is monitoring for changes, allowing you to keep track of modifications, additions, or deletions.
Here's how to see which files are being tracked by Git:
git ls-files
Understanding Git Tracked Files
Definition of Tracked Files
In Git, tracked files are those files that have been added to the version control system at least once. The distinction between tracked and untracked files is critical in understanding how Git manages changes to files within your project. Tracked files are monitored by Git, meaning that any modifications to them can be staged and committed to the repository.
On the other hand, untracked files are new files that Git has not been told to monitor yet. These files exist in your working directory but haven't been included in the Git index. Knowing the difference between these two states helps maintain an organized repository.
File States in Git
Files in a Git repository can exist in several states:
-
Tracked: Files that have been seen by Git at least once. They are under version control.
-
Untracked: New files that have been created but not added to Git.
-
Modified: Tracked files that have had changes made since the last commit.
-
Staged: Modified files that you have marked, indicating they are ready to be committed.
To check the current state of your files, you can use the following command:
git status
This command provides a snapshot of your working directory and shows which files are tracked, untracked, modified, or staged.
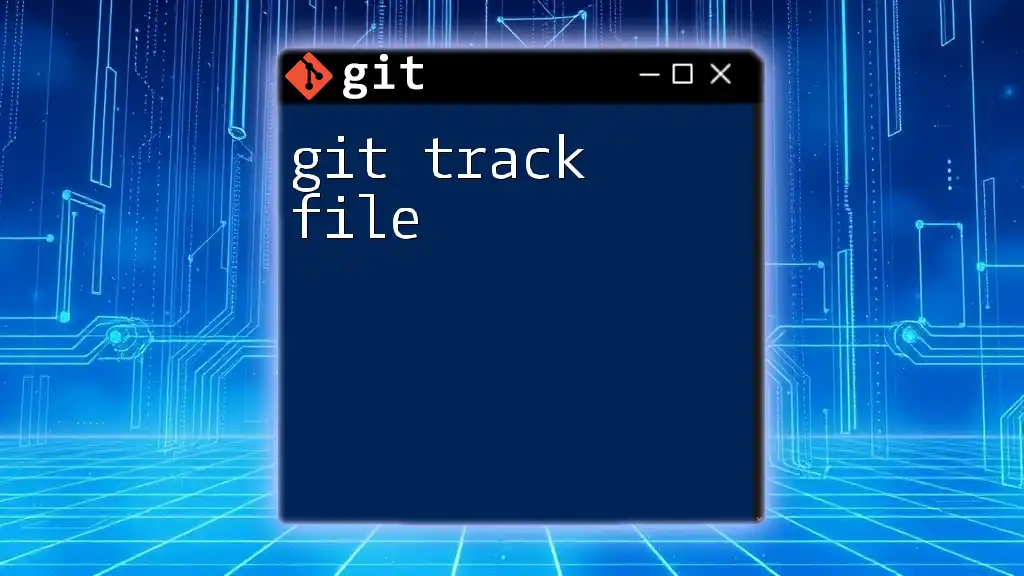
Tracking Files in Git
How to Track New Files
To start tracking a new file in your Git repository, you’ll use the `git add` command. This tells Git to begin tracking the specified file. For example:
git add <filename>
By executing this command, you update the index to reflect that this new file should be monitored by Git. Once a file has been added, it becomes tracked, and any future changes to it can be staged and committed.
Tracking Multiple Files
When you have several new files that you want to track, you can add them all at once using `git add`. For instance, to track all new files in the current directory, execute:
git add .
This will stage all changes, including new files, modifications, and deletions in that directory, simplifying the tracking process.
Tracking File Changes
Modifying a Tracked File
When you modify a tracked file, it is essential to understand how that change affects its status. After you edit a file that is already tracked, the next step is to check the status of your files:
git status
You’ll see the tracked file listed as modified. This means that Git recognizes changes have been made since the last commit.
Staging and Committing Changes
After modifying your tracked file, the next step is to stage these changes. The staging area serves as a buffer between your working directory and the repository. To stage your changes, use:
git add <filename>
Once staged, you can commit these changes to the repository with a meaningful commit message, which is crucial for maintaining a clear project history:
git commit -m "Add feature X, improve functionality for Y"
Proper commit messages help you and your team understand what changes have been made and why.
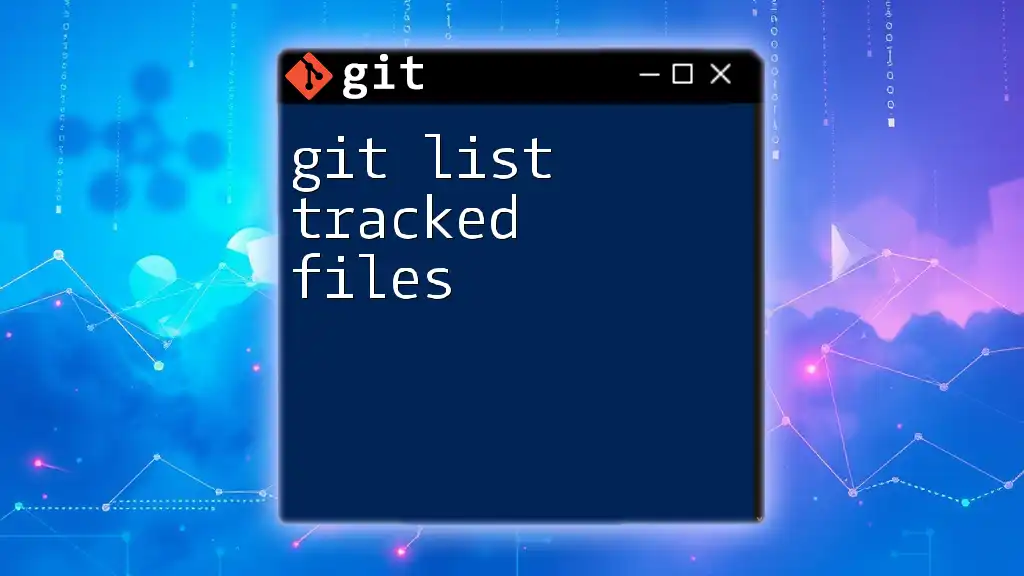
Viewing Tracked Files
Listing Tracked Files
You may want to see all the files that Git is currently tracking in your repository. The command below lists all tracked files:
git ls-files
This command returns a list of file paths in the index, allowing you to easily view what is being tracked.
Understanding Output
The output of `git ls-files` provides crucial insights into your project. Each file listed is one that has been added to version control, ensuring you're aware of which files Git is monitoring.
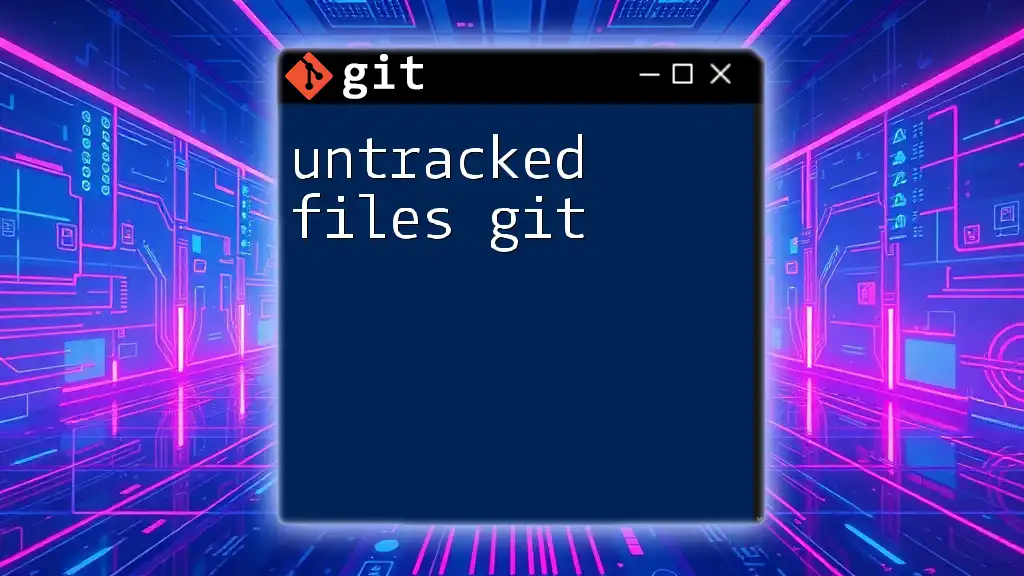
Modifying Tracked Files
Untracking a File
If you need to stop tracking a file, Git allows you to untrack it. You’ll use the `git rm --cached` command to remove the file from tracking but leave it in your working directory:
git rm --cached <filename>
Keep in mind that untracking a file means it will no longer be monitored by Git, so changes to it will not be captured unless you add it back again.
Ignoring Files
Sometimes, you may want to prevent certain files from being tracked altogether. This is where a `.gitignore` file comes into play. This file lists patterns for files and folders that should be ignored by Git. For example, your `.gitignore` might look like this:
*.log
node_modules/
Using `.gitignore` is essential for excluding temporary files, build artifacts, or sensitive data that should not be versioned.
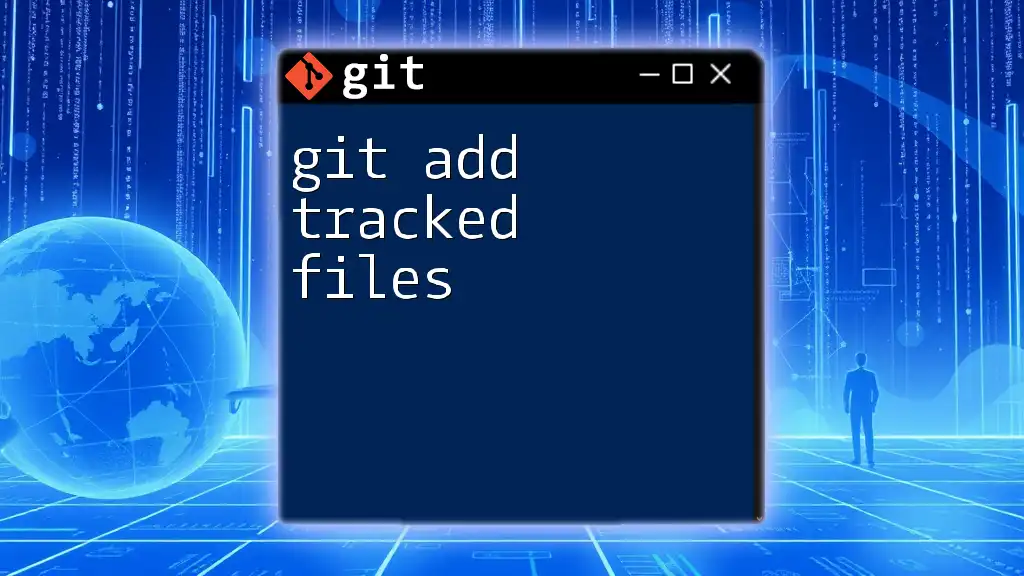
Best Practices for Managing Tracked Files
Keeping Track of Changes
To effectively manage tracked files, it's crucial to regularly check and review the status of your repository using:
git status
Additionally, using `git diff` allows you to see exactly what changes have been made before staging them. This continuous monitoring fosters better habits and ensures you are aware of the modifications made in your project.
Organizing Files and Repositories
A well-structured project directory significantly enhances collaboration and maintains clarity among team members. Keeping related files organized and adhering to a coherent directory structure is advisable, as this practice simplifies both navigation and understanding of your codebase.
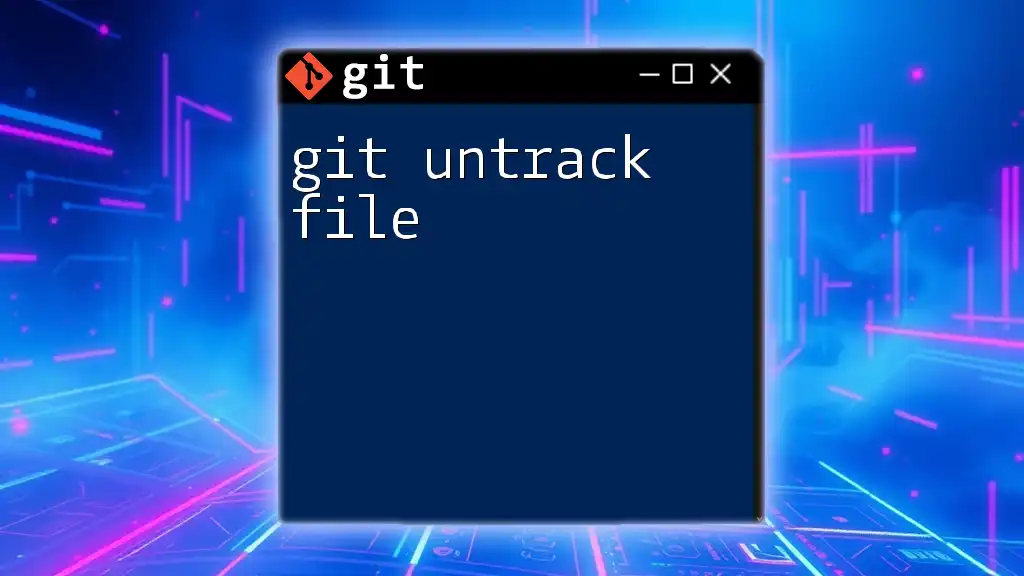
Conclusion
Understanding git tracked files is fundamental for effective version control practices. By mastering how to track, modify, and manage your files in Git, you empower yourself to maintain a clean, organized, and understandable project history. Embrace these concepts, practice them, and you will be well on your way to becoming proficient in using Git for version control in your projects.
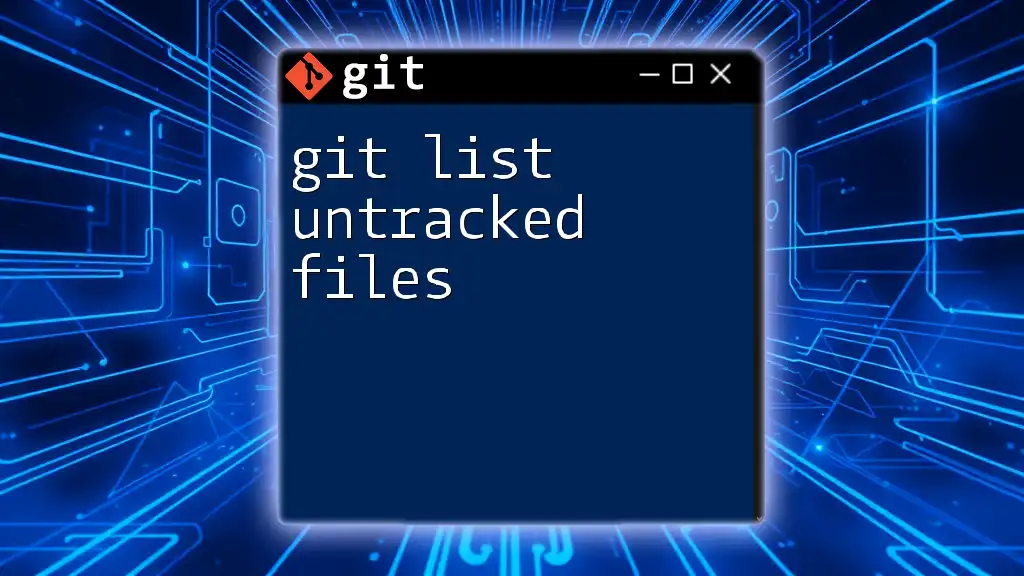
Additional Resources
For those looking to deepen their understanding of Git, consider exploring comprehensive resources and documentation that cover a broader range of commands, techniques, and best practices in version control.