The `git ls-files` command is used to show the files that are currently tracked in the Git repository, along with their status in terms of staging and other attributes.
git ls-files
What is `git ls-files`?
`git ls-files` is a powerful command used in Git that allows users to view the files that are being tracked in their repository. Understanding this command is essential for efficiently managing your project files, as it helps you to quickly assess the contents of your staging area and working directory. By listing the files tracked by Git, you gain insight into what changes have been made, what files are staged for commit, and which files are not.
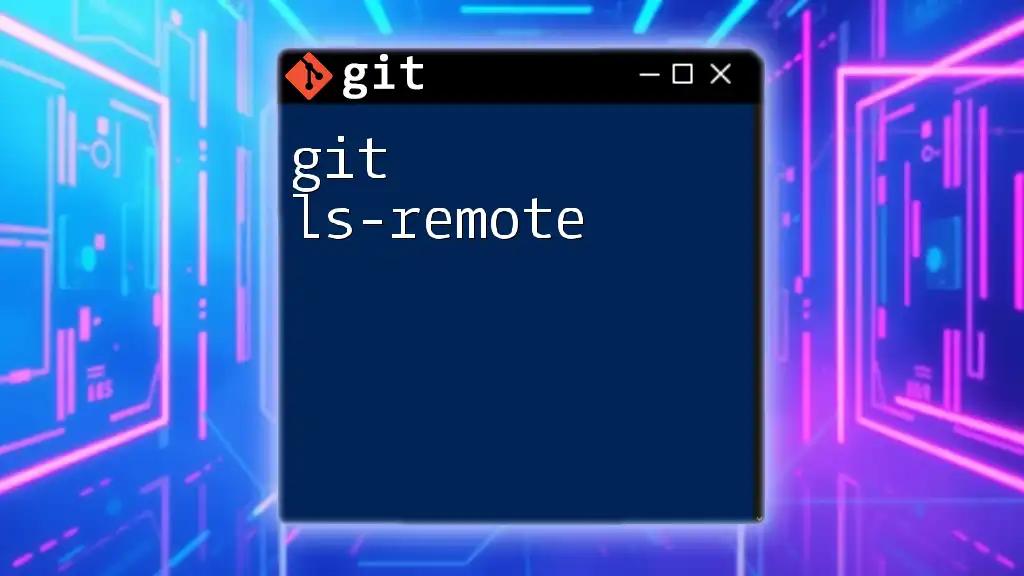
Basic Usage of `git ls-files`
Syntax of `git ls-files`
The basic syntax of the `git ls-files` command is simple and straightforward, facilitating ease of use:
git ls-files [options] [pathspec...]
- options: Flags that modify the command’s behavior.
- pathspec: Optional specifications for filtering files based on paths.
Displaying Tracked Files
To display all files tracked by Git in your current repository, you can enter the following command:
git ls-files
This command will provide a clean list of files currently under version control. Tracked files refer to those files that have been added to the staging area with `git add` and are being monitored by Git for any changes.
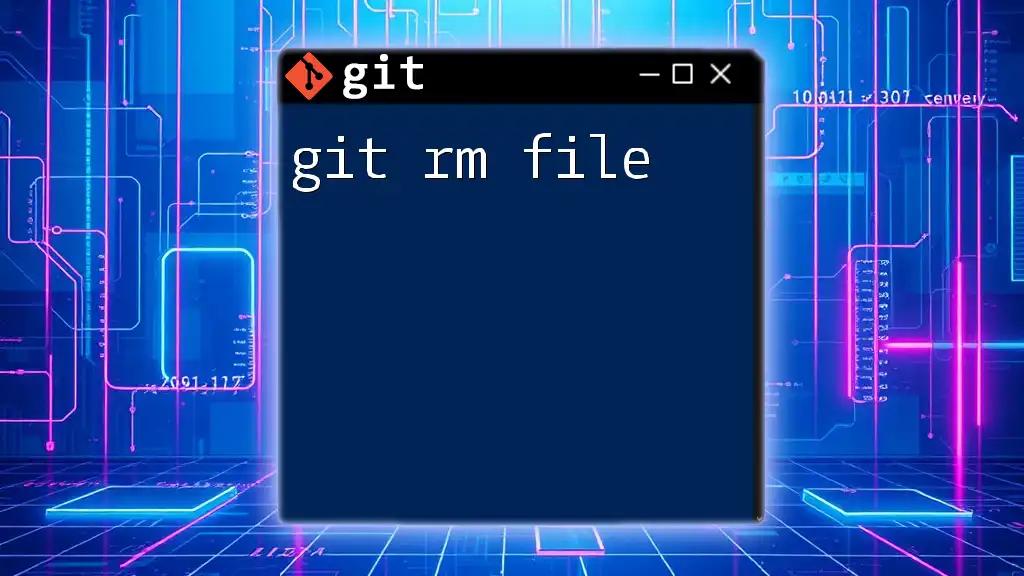
Options and Flags with `git ls-files`
Commonly Used Options
Using options expands the functionality of `git ls-files`, allowing for a more tailored output:
-
`-o`: Show other (untracked) files To view untracked files in your repository, use:
git ls-files -o
This will list files that are not under version control, giving you a quick overview of what might need to be added.
-
`-d`: Show deleted files To see any deleted files that were previously tracked:
git ls-files -d
The output here will indicate which files have been removed from the working directory while still being tracked by Git.
-
`-i`: Show ignored files To list files that are ignored by Git (as defined in your `.gitignore` file):
git ls-files -i --exclude-standard
This option provides insight into what files Git is designed to overlook.
Advanced Options
-
`-z`: Handle output with null delimiters Using this option may be beneficial when processing the output programmatically:
git ls-files -z
-
`-s`: Show staged files If you're interested in viewing what files are staged for your next commit, you can use:
git ls-files -s
This displays the cached files along with their status, streamlining your staging review process.
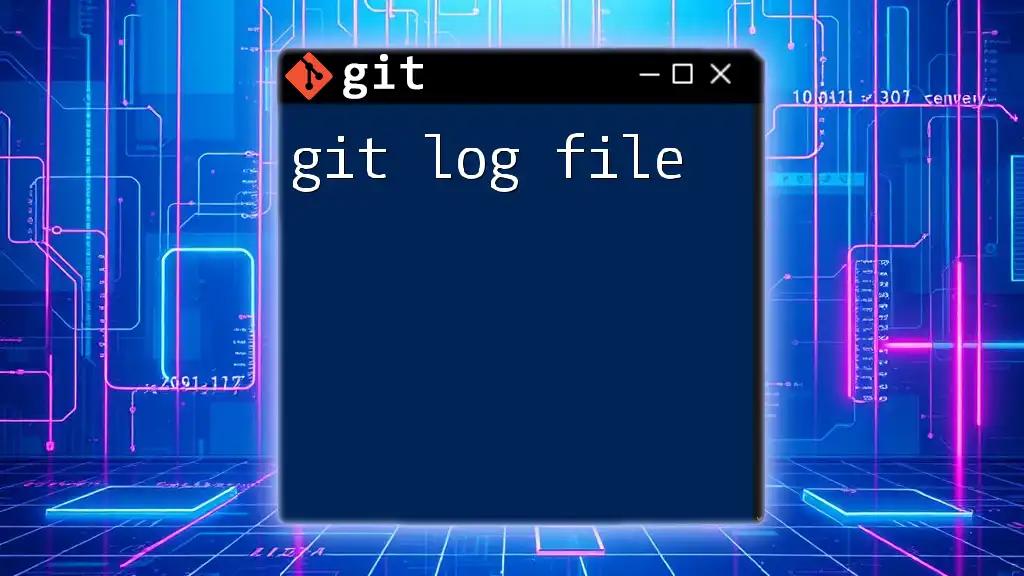
Filtering Output with Pathspec
Pathspecs refine your output by allowing you to specify particular files or types of files. For instance, if you only want to see text files, you can run:
git ls-files "*.txt"
This command filters the results, providing a focused output. Using pathspec can dramatically enhance the usability of `git ls-files`, particularly in larger projects with numerous files.
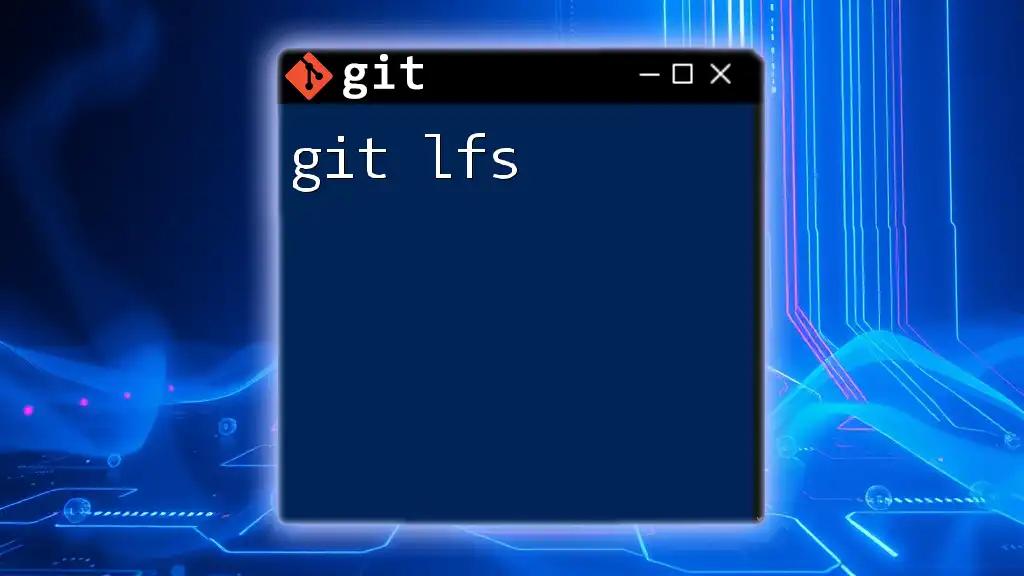
Understanding Output Formats
Output of `git ls-files`
When you execute `git ls-files`, the standard output consists of the paths of tracked files, one per line. If there are no tracked files, you can expect no output at all. This minimalistic design makes it easy to understand what's being tracked at a glance.
Customizing Output
You can further customize the output depending on your requirements by combining various options. For instance, to see a list of both tracked and untracked files, you might use:
git ls-files -o --exclude-standard
This customization allows you to tailor the output to your specific context, enhancing your project management capabilities.

Use Cases for `git ls-files` in Projects
Cleaning Up Untracked Files
In collaborative projects, untracked files can accumulate over time. Use `git ls-files` to identify and clean up these files by executing:
git ls-files --others --exclude-standard
This will show all untracked files, which you might want to add or remove based on your project’s structure.
Validating Staging Operations
By using `git ls-files` to verify what has been staged, you can ensure that the correct files are included in your commit. A simple command to check staged files is:
git ls-files --cached
This validation step can be crucial before finalizing your commits.
Assessing Project Status
`git ls-files` can serve as an efficient tool to get a quick overview of your project’s files. By running it in conjunction with `git status`, you can achieve a comprehensive assessment of your repository's state.
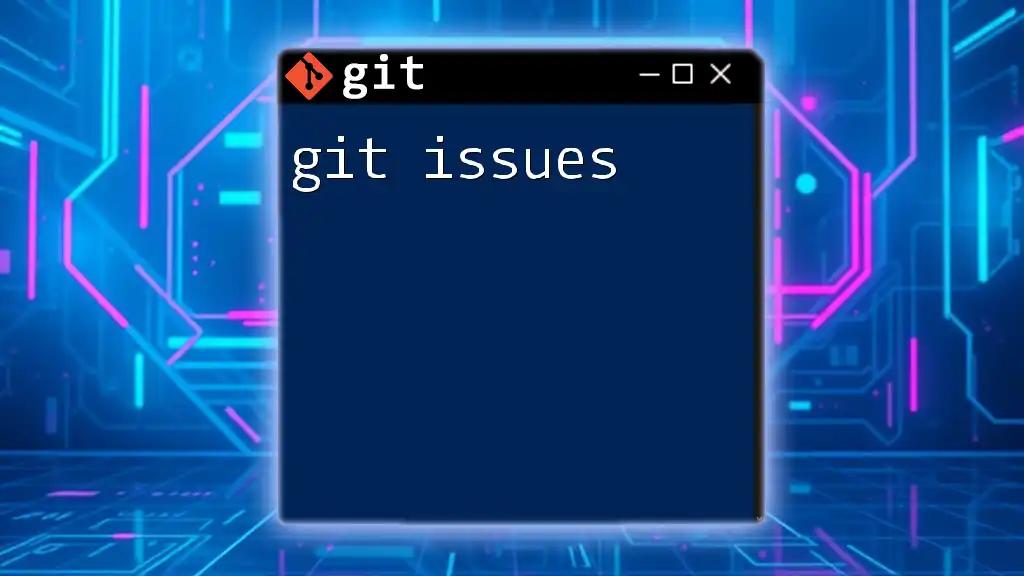
Best Practices for Using `git ls-files`
Regularly Check Tracked vs. Untracked Files
Maintaining a clean workspace is essential for productivity. Regular checks with `git ls-files` can help you stay on top of which files are being tracked versus those that are untracked, ensuring you don’t miss any important changes.
Leveraging Scripting for Automation
Consider creating scripts that utilize `git ls-files` for automating routine checks. For example, a simple shell script can be set up to notify you if untracked files exist:
#!/bin/bash
untracked=$(git ls-files --others --exclude-standard)
if [ -n "$untracked" ]; then
echo "Untracked files:"
echo "$untracked"
else
echo "No untracked files found."
fi
This proactive approach can streamline your workflow and keep you organized.
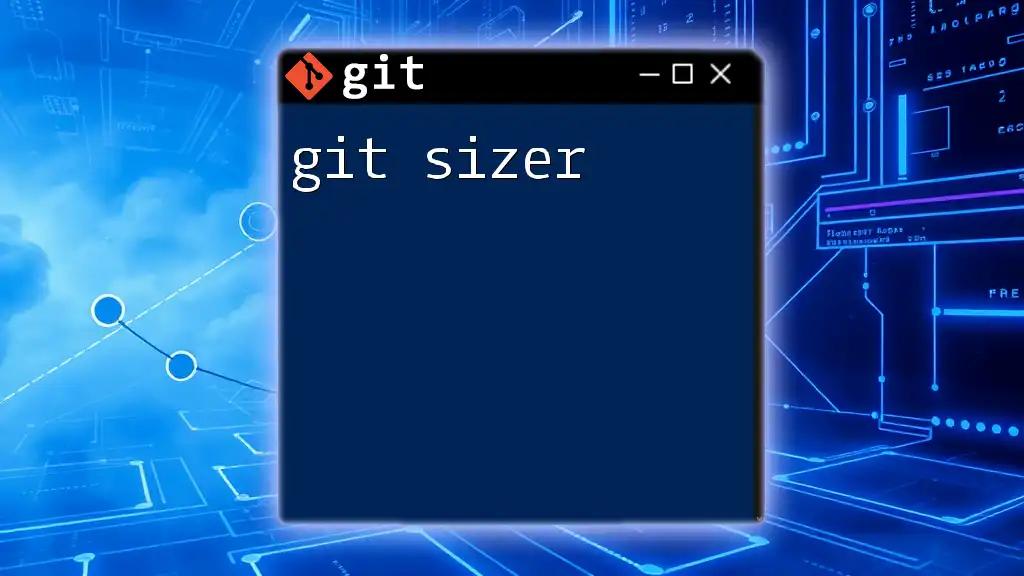
Conclusion
The `git ls-files` command is a vital component of effective Git usage, providing clarity and control over files within your repository. From monitoring tracked files to identifying untracked ones, it enhances your ability to manage your project efficiently. Regular use of this command will not only improve your productivity but also equip you with a deeper understanding of your Git workflow. Embrace the power of `git ls-files`, and watch your version control skills soar.
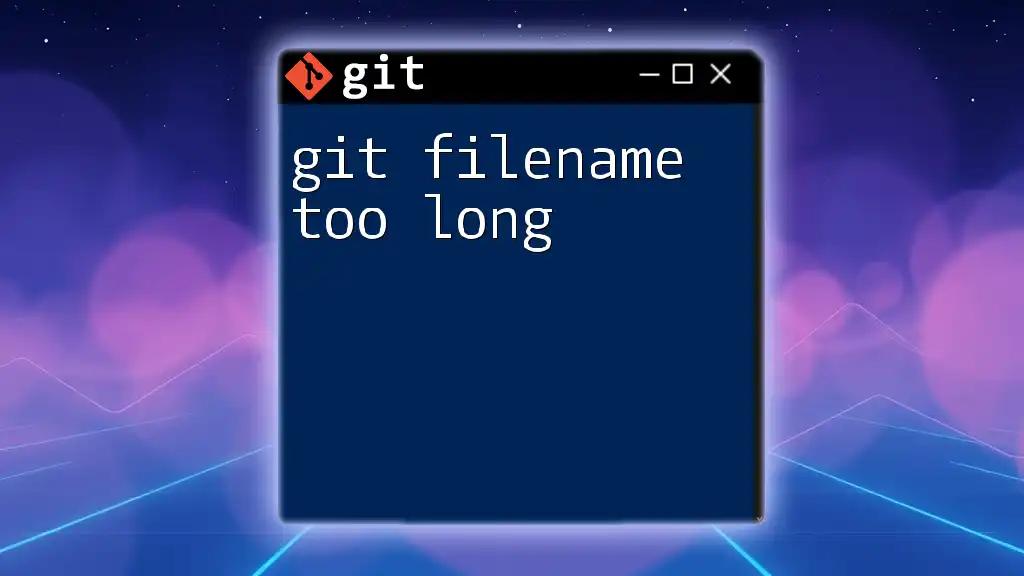
Additional Resources
To further enhance your Git skills, consider exploring literature, online courses, and interactive tutorials tailored to the intricacies of Git commands. These resources will support your journey to becoming a proficient Git user.