A "git file" typically refers to any file that is tracked by Git in a repository, and you can manage these files using various Git commands, such as adding, committing, or checking their status.
Here's a simple command to check the status of tracked files in your Git repository:
git status
Understanding Git Files
What is a Git File?
A git file refers to any file that resides in a Git repository, which is a collection of files that Git tracks. Understanding the significance of files in Git is crucial, as files can exist in different states: tracked, untracked, and ignored.
- Tracked files are those that Git is currently monitoring. These can be further divided into staged (ready to be committed) and committed.
- Untracked files are files that Git has not been told to track. They are essentially new files in your repository that have not yet been added.
- Ignored files are files you explicitly tell Git to ignore, usually through a `.gitignore` file. This is often used for files that are generated during development that do not need to be tracked, like logs or temporary files.
The Git Directory Structure
Understanding the internal workings of a Git repo involves familiarizing yourself with the `.git` directory. This hidden directory contains all versioning information for the project.
- Objects: This directory stores all content in the repository in the form of blobs (files) and trees (directories).
- Refs: This area keeps track of pointers to commits, such as branches and tags, essential for navigating the history.
- Config: The configuration file for the repository, containing settings and preferences.
One of the most important files for managing which files Git should ignore is the `.gitignore` file. This file is crucial for keeping your repository clean and avoiding unnecessary clutter.
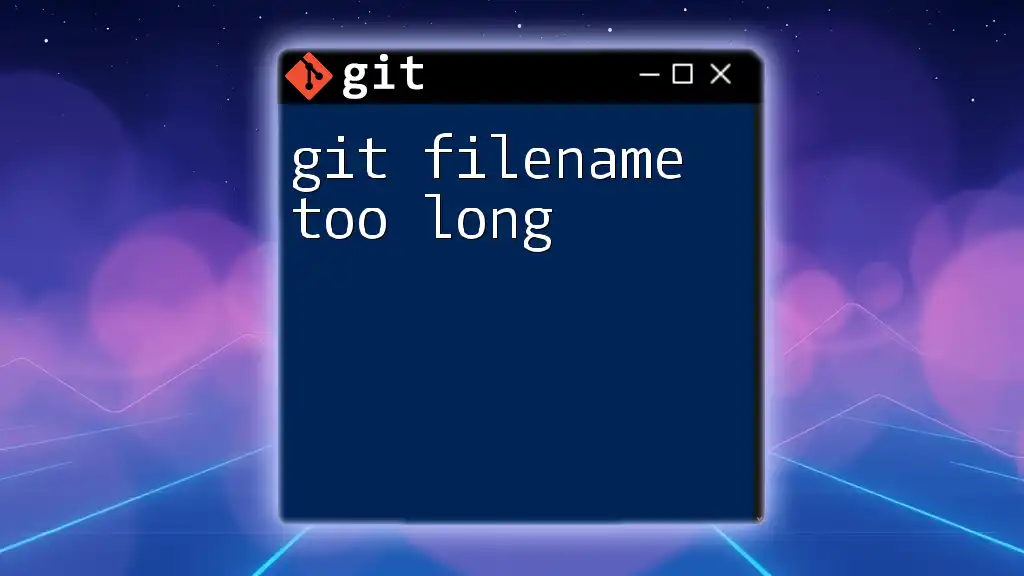
Managing Files in Git
Tracking Files with Git
To start tracking files, you need to add them to the staging area. The command for this is:
git add <file>
For example, if you want to track a file named `myfile.txt`, you would use the following:
git add myfile.txt
This command stages the file, making it ready for your next commit.
Committing Files
Once you've staged your files, the next step is to commit them. A commit represents a snapshot of your project at a particular point in time. The command to commit files is:
git commit -m "commit message"
A good practice is to include a meaningful message that describes what changes you made. For instance, a suitable commit might look like this:
git commit -m "Add initial project files"
This message helps others (and future you) understand the history of changes within the repository.
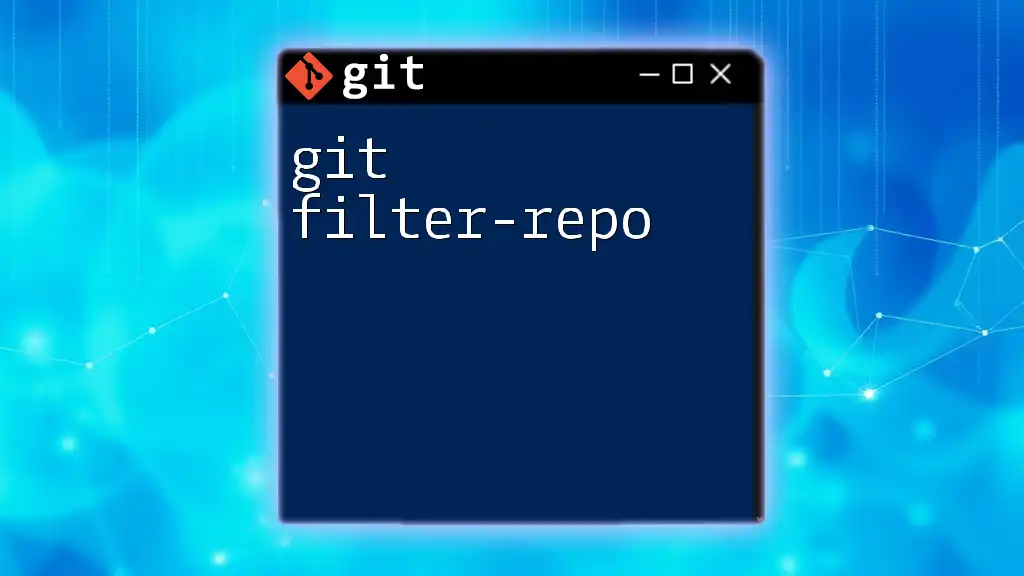
View and Manage Changes in Files
Checking the Status of Files
To see the current status of files in your Git repository, utilize:
git status
This command provides an overview of tracked and untracked files, showing you what's staged, what's changed, and what's not being tracked. This is an essential command for understanding the state of your project before you commit.
Viewing File Changes
If you want to see what modifications have been made to your files before they are staged or committed, you can use:
git diff
This command displays the differences between your working directory and the index (staging area). For a specific file:
git diff myfile.txt
This will show precisely what has changed in `myfile.txt`.
Viewing Committed Changes
To view the history of commits made in the repository, use:
git log
This will display a list of commits along with their messages and identifiers. If you want to delve deeper into a specific commit's changes, use:
git show <commit_id>
This command provides detailed information about what changes were made in that specific commit, including changed files and the differences.
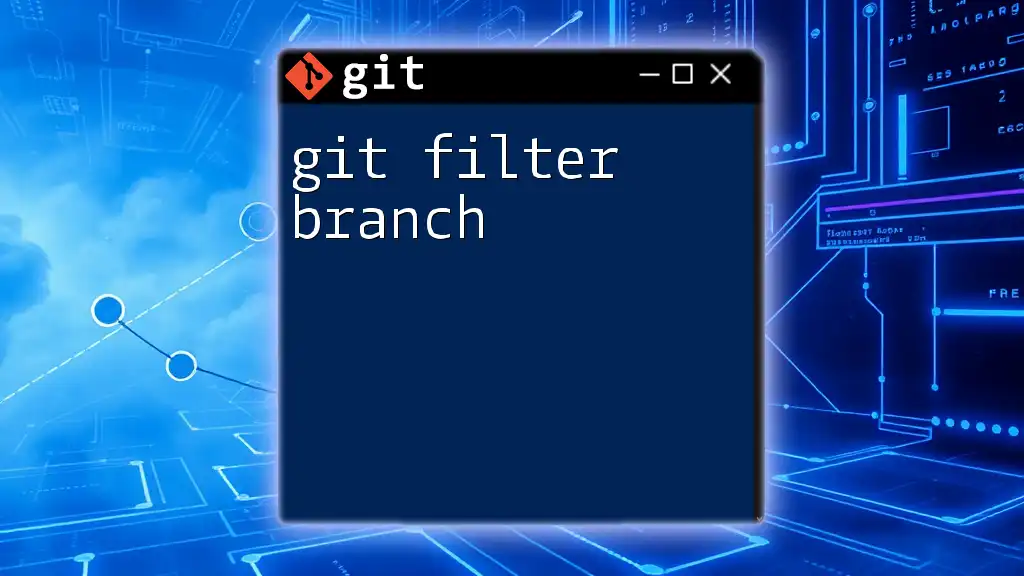
File Operations in Git
Renaming and Moving Files
Git makes it straightforward to rename or move files. Use the following command:
git mv <old-fname> <new-fname>
For example, if you want to rename `myfile.txt` to `newfile.txt`, execute:
git mv myfile.txt newfile.txt
This will track the rename as a change in Git.
Deleting Files
If you want to remove a file from the repository, you can use:
git rm <file>
For example, to delete a file called `obsolete-file.txt`, you would run:
git rm obsolete-file.txt
This command not only deletes the file from your working directory but also stages that deletion for the next commit.
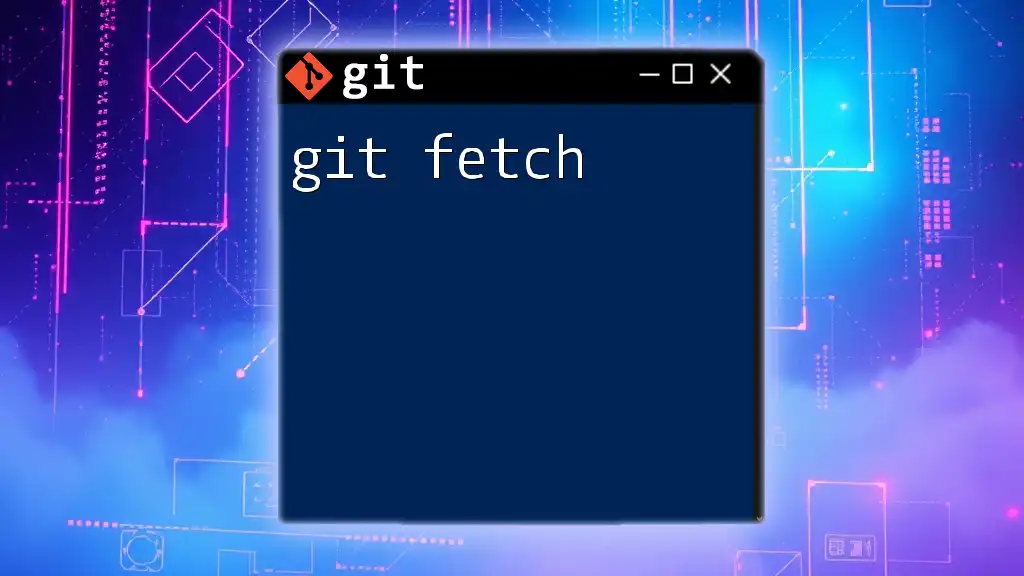
Ignoring Files
Creating a .gitignore File
To keep certain files from being tracked in your Git repository, you can create a `.gitignore` file. This file tells Git which files or directories to ignore. For instance, if you want to ignore all log files, your `.gitignore` file might look like this:
# Ignore all log files
*.log
Common Patterns for .gitignore
The `.gitignore` file allows various patterns to exclude files. You can use wildcards and specific paths to fine-tune what gets ignored. Examples include:
- To ignore a specific file:
secret.txt
- To ignore an entire directory:
logs/
By using `.gitignore`, you ensure that unnecessary files do not clutter your repository.
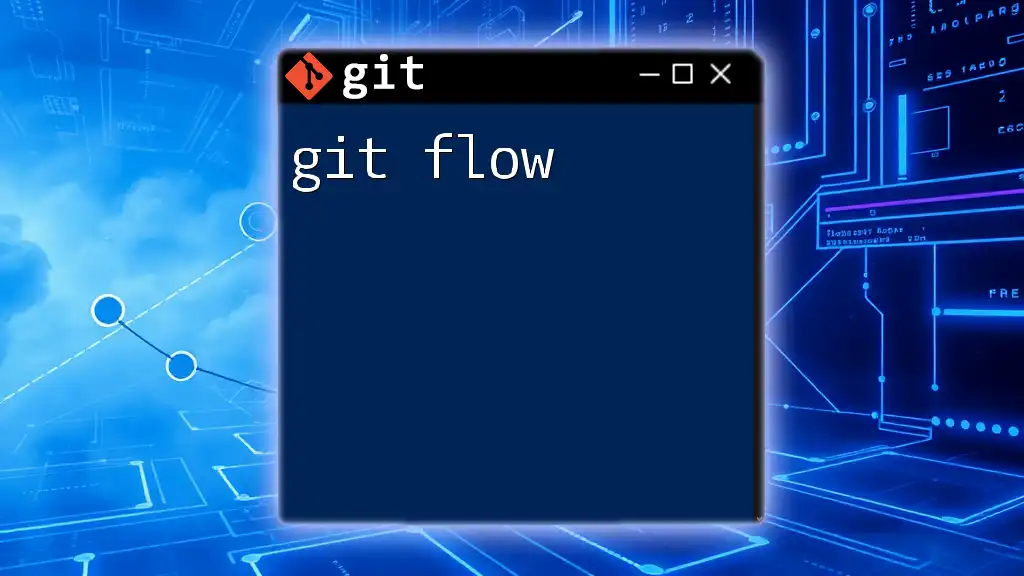
Advanced File Management
Stashing Changes
Sometimes you may want to save your work in progress temporarily without committing. In such cases, you can use:
git stash
This command safely stores your changes, allowing you to work on something else. You can later retrieve your work with:
git stash apply
Reverting File Changes
If you need to discard changes made to a file since the last commit, you can revert those changes with the following command:
git checkout -- <file>
This command resets the specified file to its last committed state, effectively discarding any changes made since then.
For more significant reverts (i.e., reverting an entire commit), you can use:
git revert <commit_id>
This creates a new commit that undoes the changes made by the specified commit.
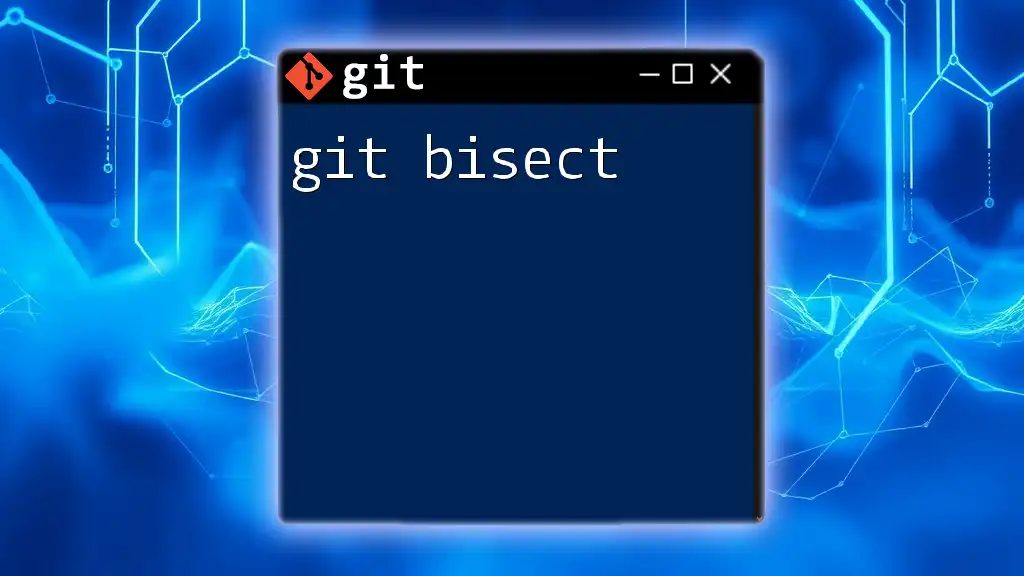
Conclusion
Mastering git file management is vital for effective version control in your projects. Whether you are tracking, viewing, or modifying files, knowing the appropriate Git commands empowers you to manage changes confidently. By practicing the commands discussed above, you will solidify your understanding and improve your workflow. Remember, the more you practice, the more proficient you will become with Git and file management.
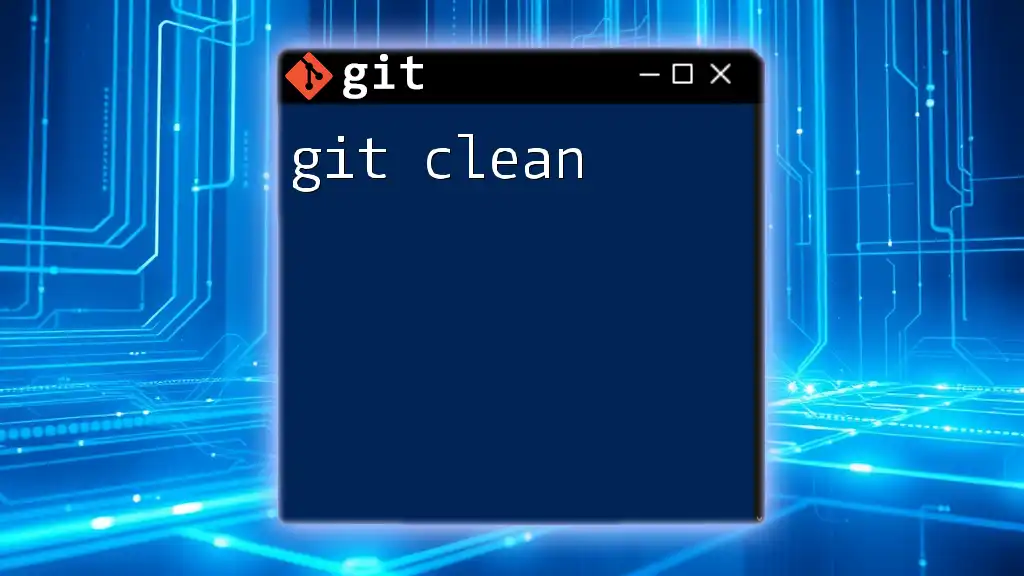
Additional Resources
For those looking to deepen their understanding of Git, there are many resources available online, including [the official Git documentation](https://git-scm.com/doc) and various tutorials that can provide further practice and insights. Don’t hesitate to explore these resources to enhance your Git skills!