A Git pipeline is a series of automated processes that allow developers to continuously integrate and deploy code, ensuring that changes are tested and released efficiently.
Here’s a basic example of a Git pipeline using a GitHub Actions workflow:
name: CI Pipeline
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
What is a Git Pipeline?
A Git pipeline is a metaphorical representation of the stages and steps that code goes through from development to production. It encompasses various processes such as testing, building, and deploying your application. Understanding the Git pipeline is integral to ensuring a smooth, automated workflow, as it allows teams to deliver code changes quickly and reliably. Developers often utilize pipelines in relationship with Continuous Integration (CI) and Continuous Delivery/Deployment (CD), which are foundational principles of modern DevOps practices.
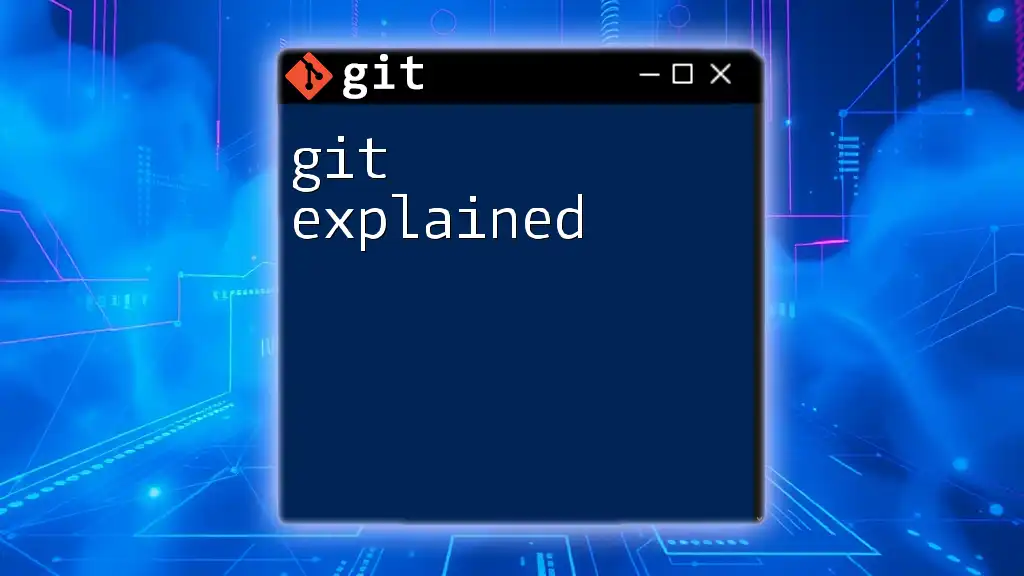
Stages of a Git Pipeline
Development Stage
The development stage is where the code is written, modified, and managed through version control. A well-defined workflow typically incorporates branching strategies, which allow multiple features or fixes to be worked on simultaneously without clashing.
Main Branch: This branch represents your production-ready code. It reflects the most stable version of your application.
Feature Branches: Developers create these branches to work on new features or bug fixes. After completing changes, they will later merge these branches back into the main branch for further testing.
To create a new feature branch, you can utilize the following command:
git checkout -b feature/my-new-feature
This command makes it easy to segment work and manage multiple ongoing developments without disrupting the main codebase.
Testing Stage
Once code has been developed, it must be tested to ensure its quality and functionality. Automated testing within a pipeline is crucial as it allows developers to quickly identify issues without manual intervention.
There are several types of tests:
- Unit Tests: Check individual components for expected behavior.
- Integration Tests: Verify that different modules or services interact correctly.
- End-to-End Tests: Validate the complete workflow of the application.
For example, using a GitHub Actions workflow, you can set up automated testing after each push to the repository:
name: CI
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
In this setup, every time new code is pushed, the tests will automatically execute, providing immediate feedback on the code's integrity.
Deployment Stage
Following successful testing, the next phase is deployment. Deployment can take the form of Continuous Delivery (CD), where code changes are automatically pushed to staging environments, or Continuous Deployment, where code is immediately deployed to production if tests pass.
Having an efficient deployment process saves time and reduces the risk of human error. A sample command to push code to a cloud platform like Heroku could be as simple as:
git push heroku main
This command deploys the code from your main branch directly to production, making it live for users.
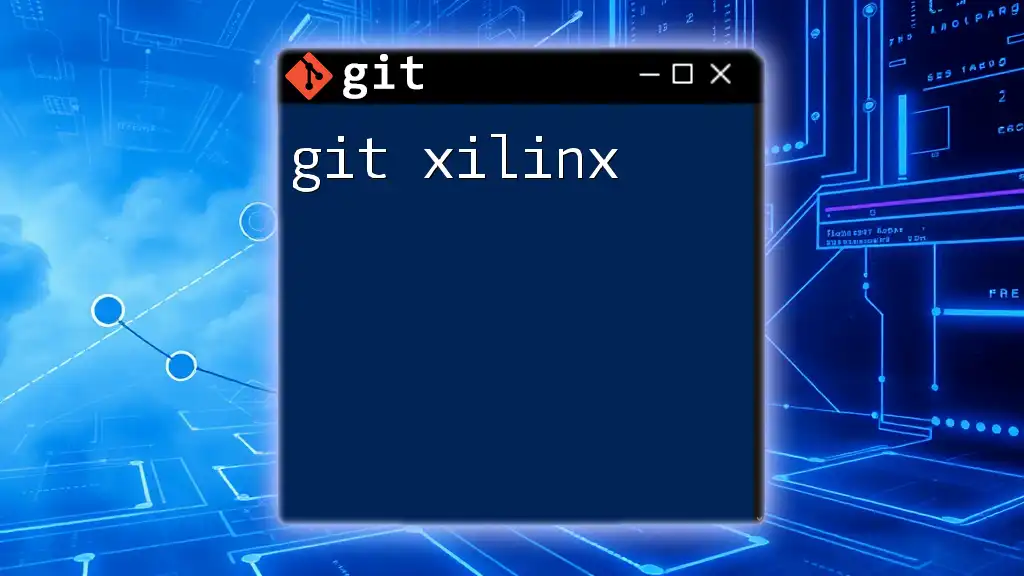
Tools and Technologies for Git Pipelines
CI/CD Tools Overview
Several essential tools can help set up and manage Git pipelines effectively. Here are some of the most popular:
- Jenkins: Highly extensible and open-source automation server used for CI/CD.
- GitHub Actions: Offers integrated CI/CD workflows directly in GitHub repositories.
- GitLab CI/CD: Provides seamless integration for GitLab repositories with embedded CI/CD features.
- CircleCI: Cloud-based CI/CD service that automates the testing and deployment processes.
Each of these tools comes with unique features tailored to streamline the development workflow, allowing developers to choose the best fit for their needs.
Integrating Git with CI/CD Tools
Integrating Git with CI/CD tools is essential for creating efficient workflows. For instance, using GitHub Actions, you can establish a basic configuration file (`.github/workflows/main.yml`) that automates testing, building, and deployment:
name: Node.js CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Dependencies
run: npm install
- name: Run Build
run: npm run build
In this workflow, every push or pull request triggers a series of automated steps that validate and build the application, ensuring that only quality code makes it into production.
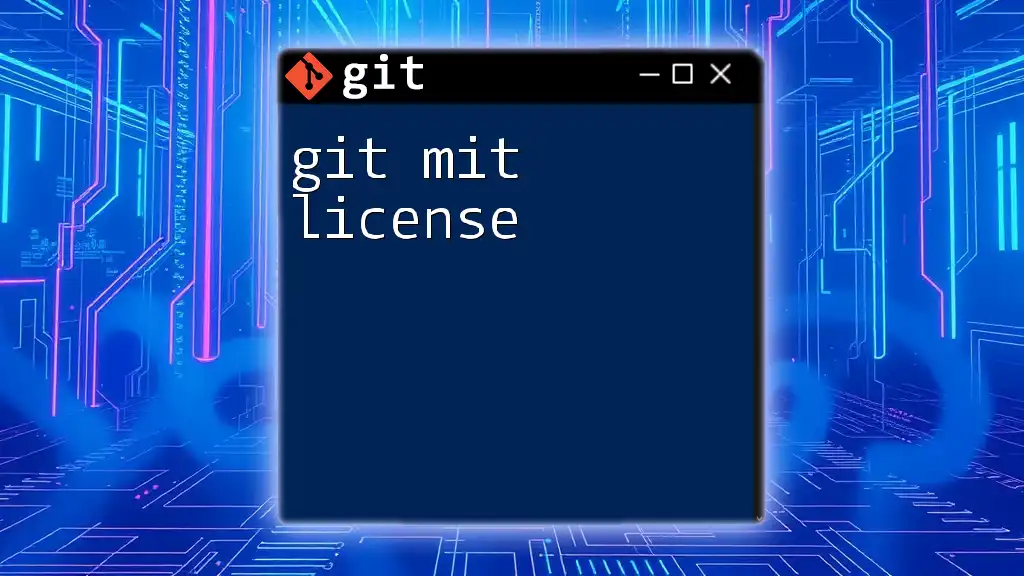
Best Practices for Defining a Git Pipeline
Clear and Consistent Branching Strategy
Having a clear branching strategy is vital for maintaining a coherent development process. Teams should establish a standard that defines how and when branches are created and merged. This practice minimizes conflicts and keeps the codebase organized. Using descriptive naming conventions for branches can significantly improve clarity. For example, using prefixes like `feature/`, `bugfix/`, or `hotfix/` can make the branch's purpose immediately clear.
Automation of Testing and Deployment
Automating testing and deployment is one of the most significant advantages of using a Git pipeline. It reduces manual errors and enhances developer productivity. Writing effective automated test cases is fundamental; tests should cover various scenarios and edge cases, ensuring comprehensive validation of the code.
Monitoring and Alerting
Keeping track of the pipeline's health is necessary for maintaining a robust workflow. Implementing monitoring tools enables teams to detect issues early and maintain pipeline reliability. Moreover, setting up alerts for failures helps teams respond quickly to issues, minimizing downtime. For instance, you might use services like Slack to receive notifications when a pipeline fails:
# Example scenario of alert configuration with pseudo-code
if pipeline.status == "failed":
send_slack_alert("Pipeline failed! Check here: <link>")
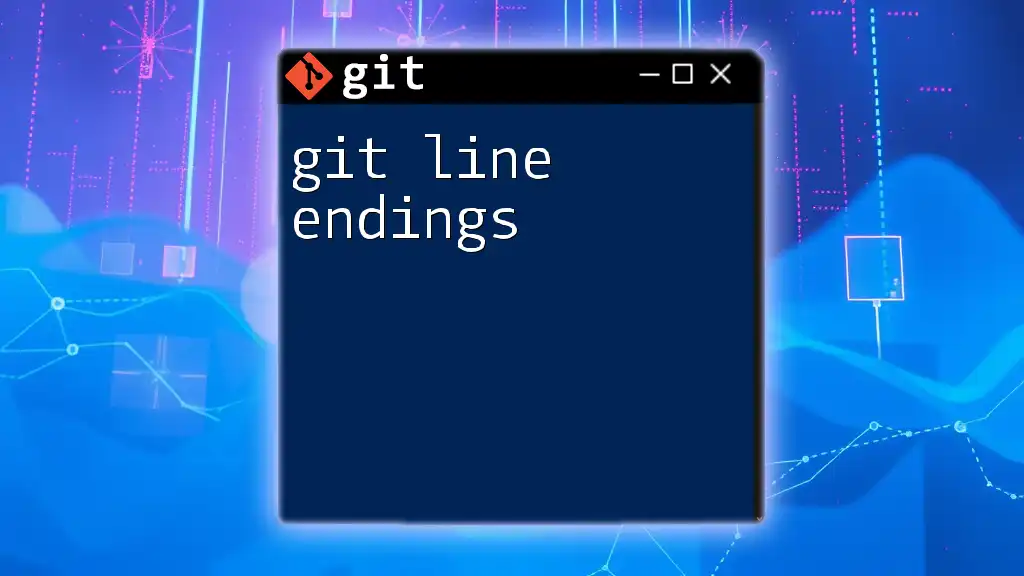
Common Traps and Solutions in Git Pipelines
Not Following Semantic Versioning
One pitfall that teams may encounter is neglecting to follow semantic versioning. Proper versioning helps to communicate changes effectively and ensures compatibility between different software components. Using a systematic approach to versioning – major, minor, and patch – allows development teams to manage releases more intelligently.
Inefficient Workflow
An inefficient workflow can slow development significantly. It's vital to regularly evaluate your pipeline processes to identify and eliminate bottlenecks. Techniques such as parallel testing and reducing the number of manual steps can drastically improve efficiency.
Ignoring Code Reviews
Code reviews are critical for maintaining code quality and sharing knowledge across teams. Fostering a culture that encourages reviews can help catch issues early and improve overall code quality. Tools that facilitate the review process, such as pull requests on GitHub or GitLab, should be actively used and encouraged among team members.
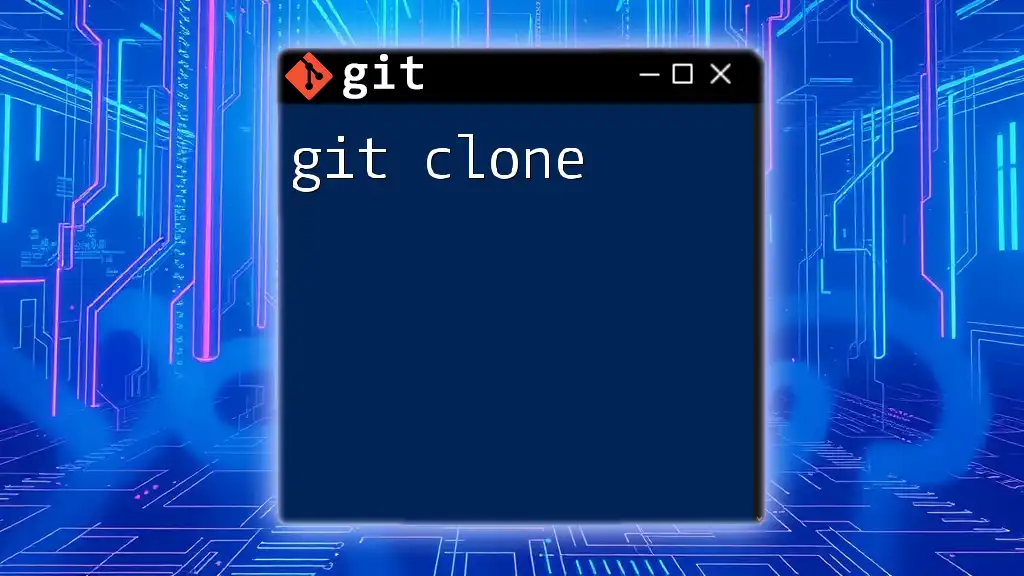
Conclusion
Understanding and implementing a Git pipeline is not just about automating tasks; it's about creating a reliable, efficient workflow that enhances the development process. By utilizing the stages of development, testing, and deployment effectively and integrating the best CI/CD tools, teams can deliver high-quality software rapidly.
As you embark on this journey, consider adopting the practices we discussed. Not only will they enhance your team's productivity, but they will also encourage better collaboration and communication within your projects.
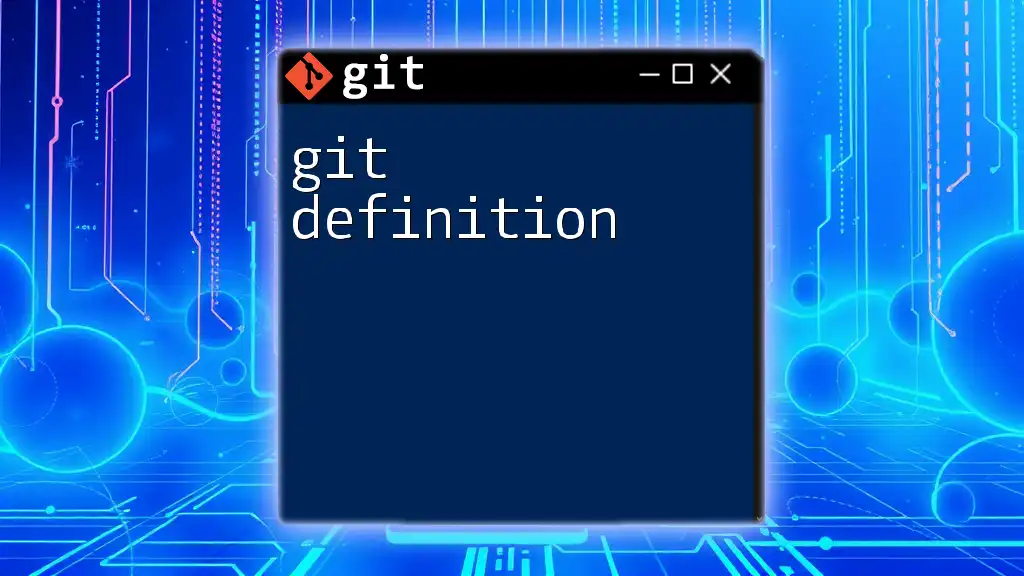
Additional Resources
For further learning, refer to the official [Git documentation](https://git-scm.com/doc) to deepen your understanding, explore recommended books on Git practices, or join community forums where you can interact with other developers.
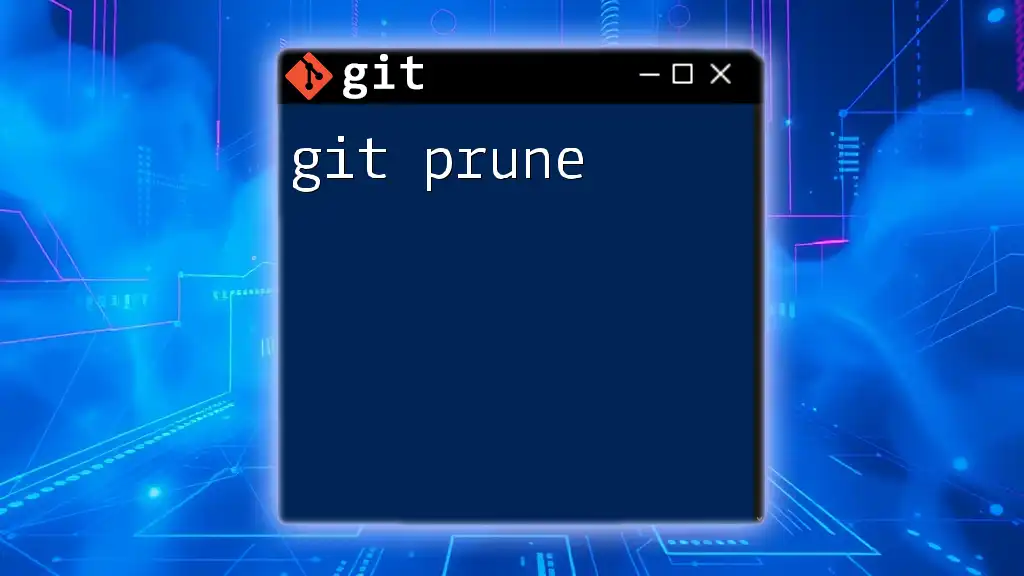
Call to Action
Feel free to join our mailing list or subscribe to our updates for more articles that can help elevate your skills in Git and software development. We encourage you to share your experiences or tips about setting up Git pipelines in your projects!