Git releases are tagged points in the project’s history that represent a specific version, enabling easier tracking of changes and deployment.
Here's an example of how to create a release tag in Git:
git tag -a v1.0 -m "Release version 1.0"
git push origin v1.0
Understanding Git Releases
Git releases are crucial milestones in the development lifecycle, providing a clear way to manage different versions of your software. A release signifies a set of changes that are stable enough to be shared with users, marking a point of progress in your project. These releases integrate the features developed, bug fixes, and any modifications that improve user experience or functionality.
The importance of git releases cannot be underestimated—they help in tracking project progress, simplify the deployment of new features, and serve as a communication tool for stakeholders to understand the latest updates in the project.
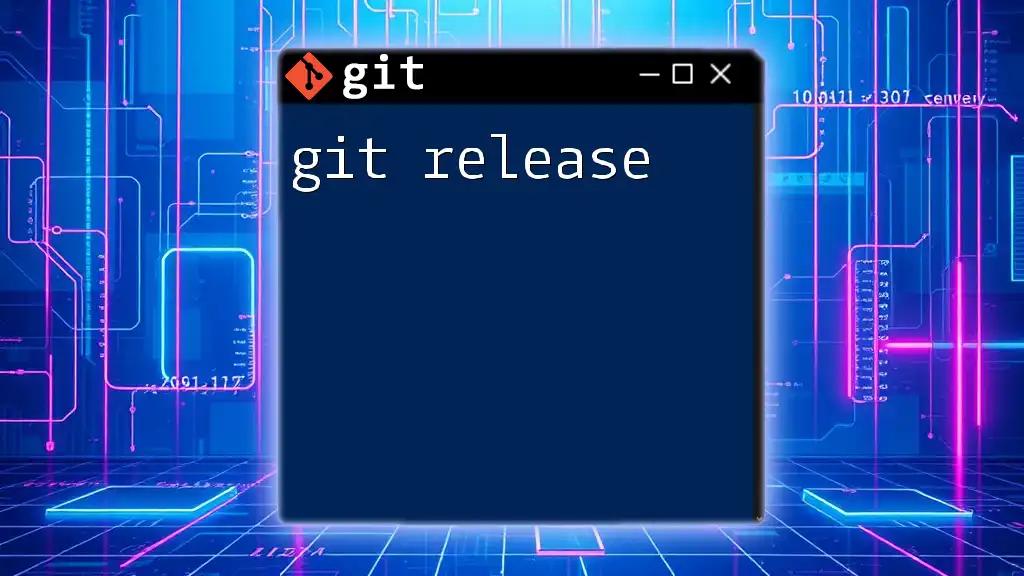
Understanding Versioning
Semantic Versioning
Semantic versioning plays a crucial role in git releases. It follows a simple format: MAJOR.MINOR.PATCH. Each component represents a different aspect of the version:
- MAJOR: Increments when there are incompatible API changes.
- MINOR: Increments when adding functionality in a backward-compatible manner.
- PATCH: Increments for backward-compatible bug fixes.
For example, a version number change from `1.0.0` to `2.0.0` indicates a major change or breaking change, while changing from `1.1.0` to `1.1.1` implies minor bug fixes.
Creating a Versioning Strategy
Establishing a clear versioning strategy is essential for effective git releases. Decide how your team will handle version increments based on feature releases and bug fixes. This clarity helps streamline communication and avoids confusion regarding project status.
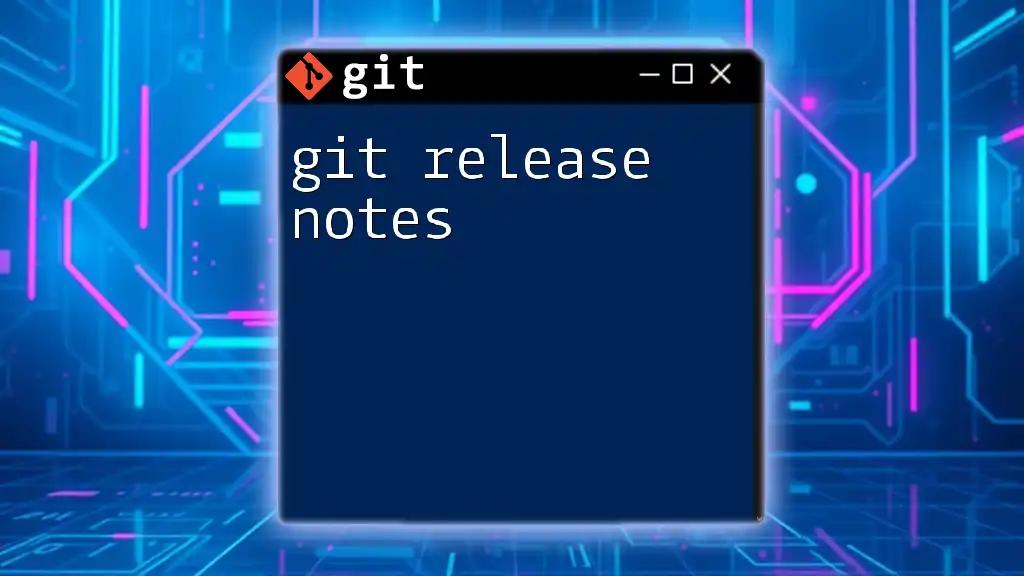
Creating Releases in Git
Using Git Tags
Tags in Git are pointers to specific commits, commonly used to mark release points. They are vital when creating git releases, providing easy access to significant versions of your project.
Creating a Tag
Creating a tag is straightforward. For example, to create your first release tag, you can run the following command:
git tag -a v1.0 -m "Initial release"
In this command:
- `-a` specifies that you are annotating the tag.
- `v1.0` is the tag name.
- `-m` allows you to add a message that describes the tag.
Listing Tags
To view the tags you've created, use the following command:
git tag
This will list all tags, giving you an overview of your project's release history.
Pushing Tags to Remote
Once you've created a tag, you need to push it to a remote repository. This is done using:
git push origin v1.0
This command ensures that other team members and stakeholders can access the tag and its associated release.
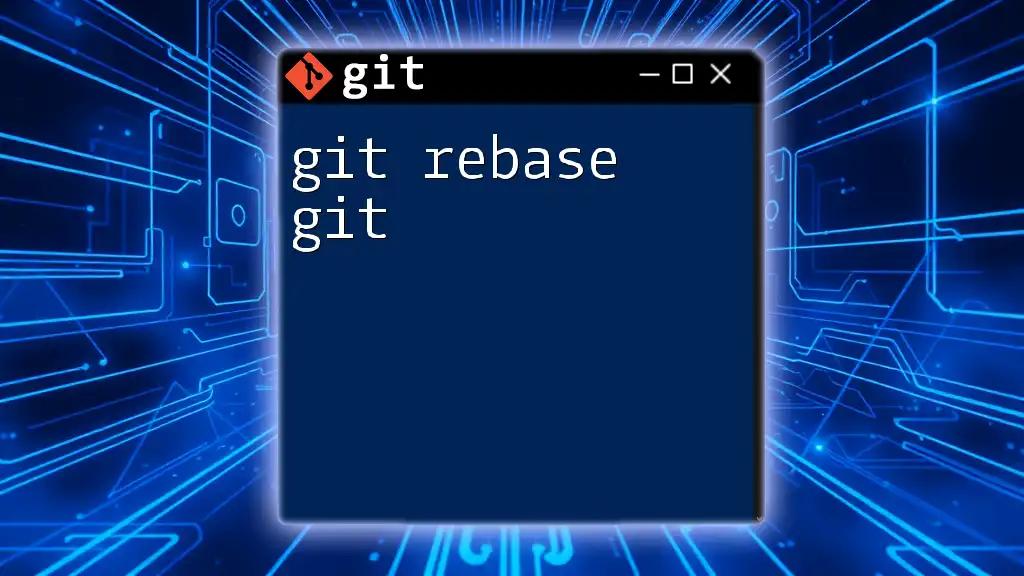
Release Management Practices
Best Practices for Managing Releases
Efficient release management is essential for the success of your project. Here are some best practices for managing git releases effectively:
- Documentation: Keep an up-to-date `CHANGELOG.md` file to document the changes made in each release. This helps users understand what's new, improved, or fixed.
- Branch Structure: Use a clear branching strategy like having separate branches for `master`, `develop`, and `feature` to help manage code changes effectively and ensure releases are tested.
Automating Releases
Continuous Integration and Continuous Deployment (CI/CD) practices enhance the release process. By automating the workflow, you can integrate changes regularly and deploy them smoothly.
For instance, using GitHub Actions, you can automate the release process by creating a `.github/workflows/release.yml` file with a simple configuration to build and deploy your project upon tagging.
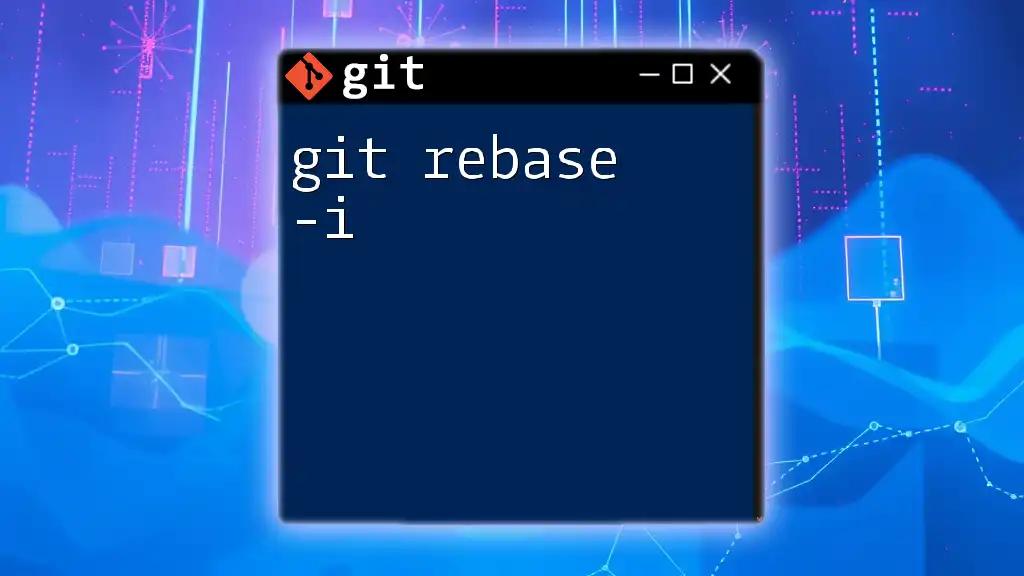
Creating Release Notes
What to Include in Release Notes
Effective release notes are essential for maintaining transparency and making sure users are informed about new changes. Important components to include in your release notes are:
- Summary of Changes: A brief overview of what the release includes.
- Known Issues: Highlight any bugs or problems not yet resolved.
- Upgrade Instructions: Provide guidelines on how to migrate to the new version.
Example Release Note Structure
A sample structure for your release notes might look like this:
<InternalLink slug="git-rebase-branch" title="Mastering Git Rebase Branch: A Quick Guide to Success" featuredImg="/images/posts/g/git-rebase-branch.webp" />
## [1.0.0] - 2023-10-01
### Added
- New user authentication feature.
### Changed
- Updated user interface for better accessibility.
### Fixed
- Resolved an issue causing application crashes.
This structured format makes it easy for users to quickly grasp the relevant changes and how they can affect their usage of the software.
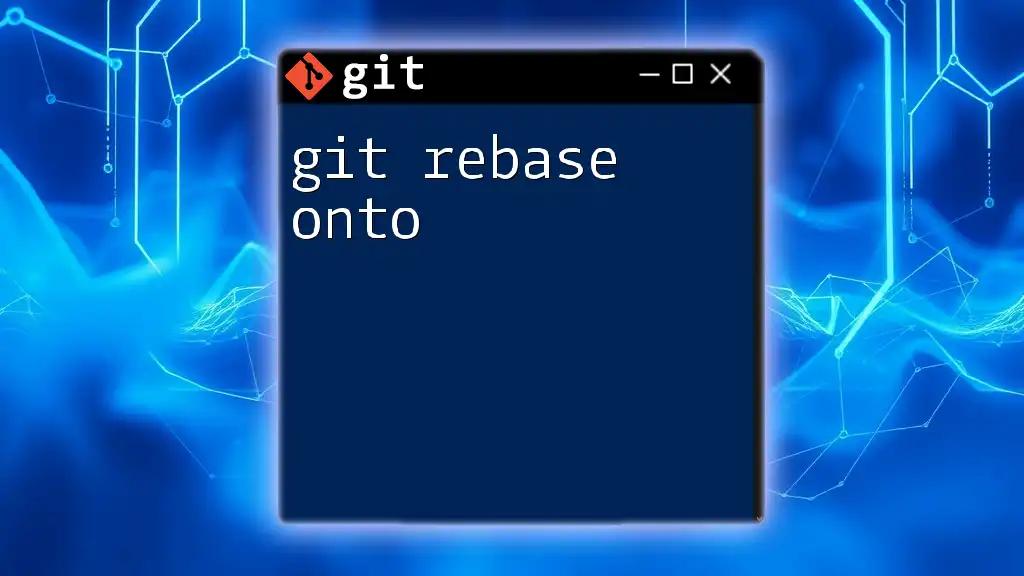
Handling Pre-Releases
Understanding Pre-Releases
Pre-releases are critical for testing new features before a full release. They can help gather user feedback and identify issues. A pre-release might include suffixes like `-beta` or `-alpha` in its version number.
Tagging Pre-Releases
You can tag a pre-release version with a command like:
git tag -a v1.0.0-beta -m "Beta release for testing"
This signifies that the version is still under testing and not fully stable. Keep your users informed about the status and expectations of pre-releases.
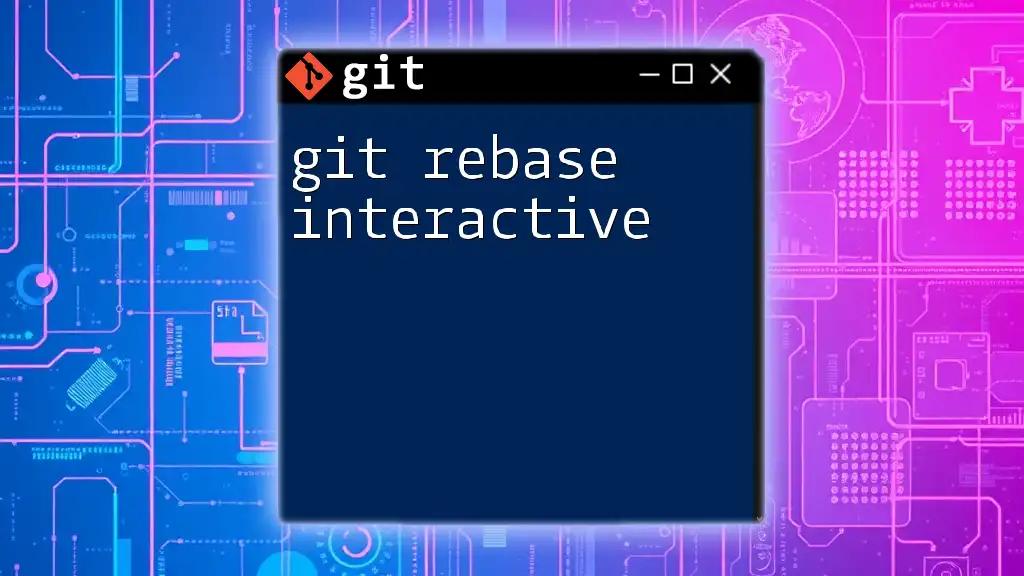
Case Studies of Releases in Popular Projects
Example 1: Git
The Git project itself utilizes a well-defined release cycle, using tags to manage its releases effectively. Each version is documented thoroughly, showing the principles of semantic versioning and the importance of communication through release notes.
Example 2: Open Source Projects
Many open-source projects such as Kubernetes or React follow similar practices by using Git tags and maintaining comprehensive release notes, showcasing the need for clear structure and documentation in software development.
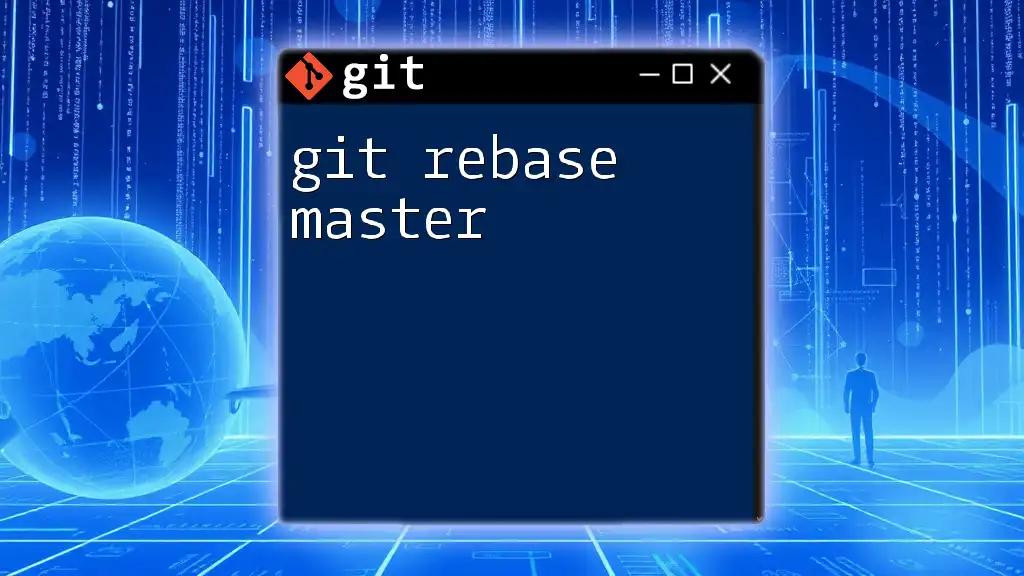
Troubleshooting Common Issues
Deleting a Tag
Sometimes, you may need to delete a tag, perhaps due to errors in the release. You can do this with:
git tag -d v1.0.0
This command removes the local tag; for remote deletion, you would use:
git push origin --delete v1.0.0
Reverting a Release
If a release needs to be reverted, you can easily checkout the previous version using:
git checkout v0.9.0
This command allows you to revert to the previous state while investigating issues in the latest release.
Handling Version Conflicts
When working with multiple developers, version conflicts can arise during updates. It is crucial to communicate changes effectively and resolve any discrepancies promptly. Utilizing branch protection rules and pull requests can help prevent conflicts.
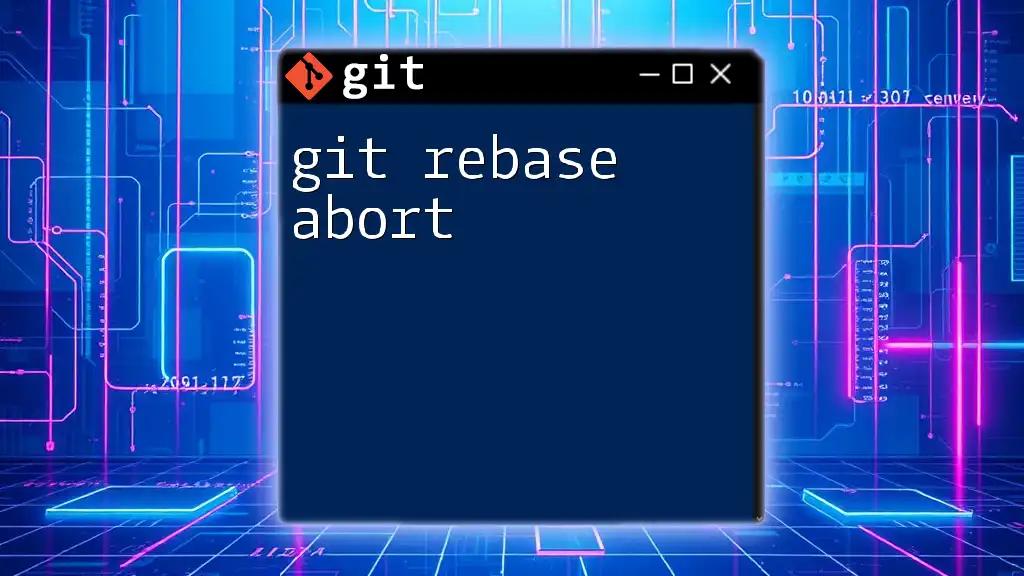
Conclusion
In summary, understanding git releases is vital for successful software development practices. They provide clarity about project progress, simplify the deployment process, and enhance communication within teams. With a solid approach to versioning, tagging releases, and documenting changes, you can make your development workflow much more efficient. Engage with these strategies, and unlock the true potential of your projects with effective git release management.
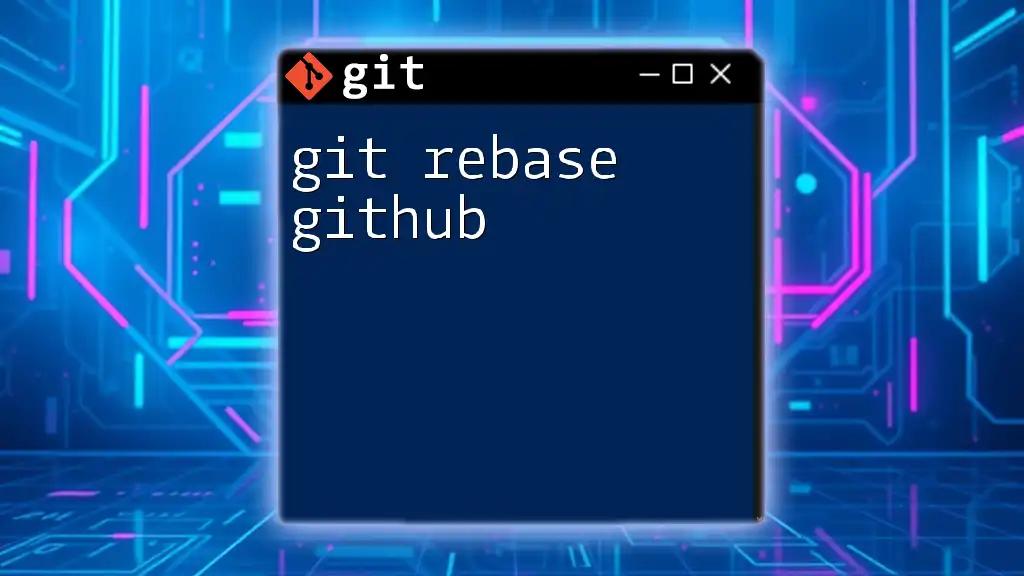
Additional Resources
To dive deeper into mastering Git and releases, consider exploring the following resources:
- Books focused on Git version control.
- Blogs that detail project management with Git.
- Online courses that cover Git practices and automation strategies for releases.