The `git rebase HEAD` command is used to move or combine a series of commits to a new base commit, effectively updating the base of your current branch with the latest changes from another branch or the latest commit on the same branch.
git rebase HEAD
Understanding Git Rebase
What is Git Rebase?
Rebasing is a process in Git that allows you to integrate changes from one branch into another. Essentially, it involves moving or combining a sequence of commits to a new base commit. This is fundamentally different from merging, which integrates the histories of two branches together, creating a new commit for the merge.
Why Use Git Rebase?
Using rebase can introduce several advantages, making it a preferred choice for many developers:
- Cleaner Project History: When you rebase, the project history appears linear, as if all changes from a branch were applied sequentially without branches. This is beneficial for understanding the history of a project at a glance.
- Easier Navigation: With a linear history, tools for analyzing your project's history, such as `git log`, become simpler and more intuitive.
Rebase is particularly useful when working on feature branches, as it can help maintain a clean commit history before integrating finalized changes into the main branch.
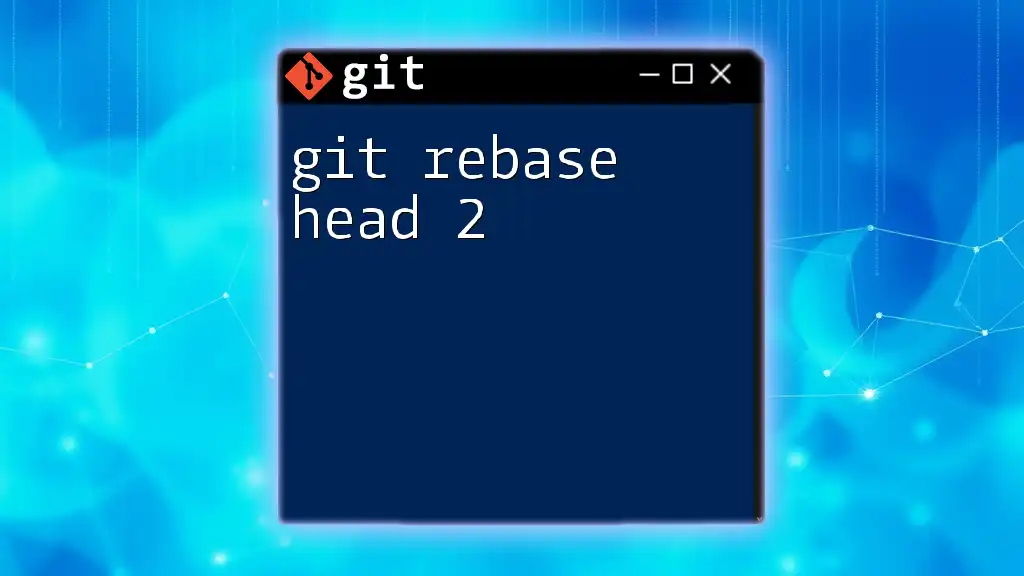
The Concept of HEAD
What is HEAD in Git?
In Git, HEAD refers to the pointer that indicates the current branch you are working on. It is essentially the alias of the most recent commit in your current working branch.
Understanding Detached HEAD State
Sometimes, you may end up in a detached HEAD state, which occurs when you check out a commit that is not at the tip of a branch. In this state, you can make changes, but they won't belong to any branch unless you create a new one. Being in a detached HEAD state can be risky if not handled properly, as it might lead to lost commits if they aren't saved correctly.
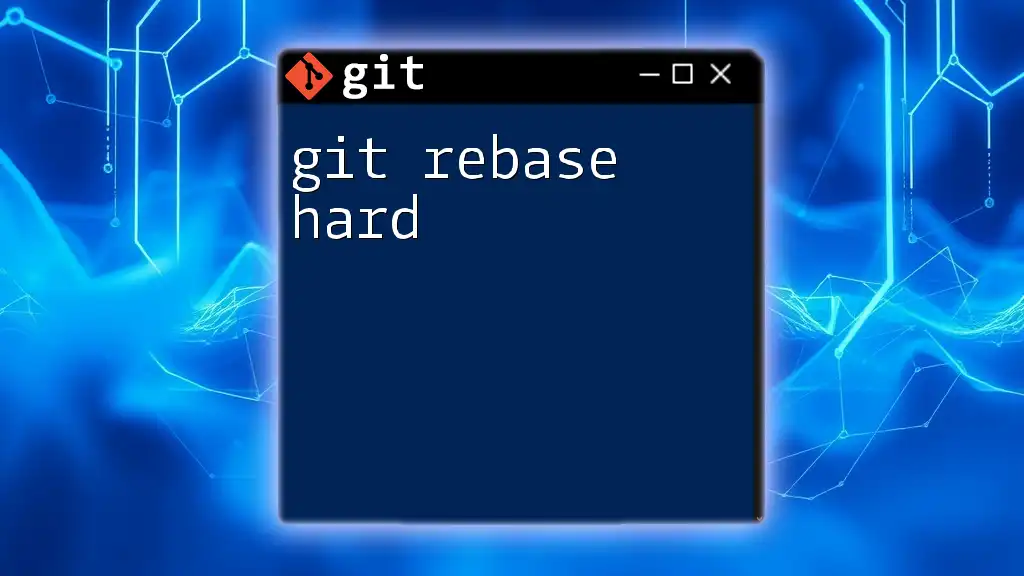
The `git rebase HEAD` Command
What Does the Command Do?
When you execute the `git rebase HEAD` command, you are essentially telling Git to reapply your current commits (your local changes) on top of another base commit. This specific command operates on the current branch and commits, incorporating the commits made on the base where HEAD points to.
Use Cases for `git rebase HEAD`
The `git rebase HEAD` command can be useful in several scenarios:
-
Cleaning Up Local Branch Commits: You might have made several incremental changes on a feature branch. Using this command allows you to combine these commits into a cleaner, single commit before merging back into the main branch.
-
Linearizing a Series of Commits: If you have made multiple changes across several tasks but want the history to reflect that work as happening sequentially, an interactive rebase might be the answer.
Common Scenarios
Example 1: Rebasing to Incorporate Changes from the Base
Imagine you're working on a feature branch and want to apply your latest commits on top of the base branch. Here’s how you can do it:
git checkout feature-branch
# Making changes and committing
git commit -m "Feature commit"
git rebase HEAD
This command will apply your changes on the very latest commit in your base branch, ensuring that your feature branch is up to date with the main work.
Example 2: Fixing Mistakes in Commit History
Sometimes, you may need to amend previous commits. This can be done using an interactive rebase. Here’s how to rewrite the last three commits:
# Start interactive rebase
git rebase -i HEAD~3
This command opens an editor allowing you to modify commit messages, squash commits together, or even reorder them. This helps ensure that your commit history is as clean and coherent as possible.
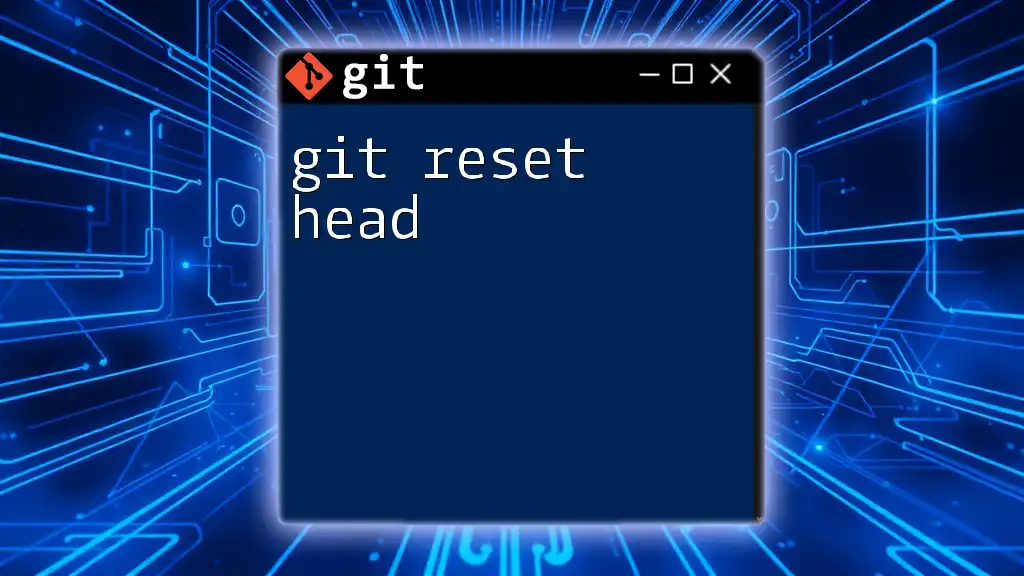
Steps to Perform `git rebase HEAD`
Pre-Rebase Preparations
Before rebasing, it's crucial to prepare your current branch thoroughly. This includes reviewing your current changes and making sure you've either committed or stashed uncommitted changes. This minimizes the risk of conflicts and ensures a smoother rebase process.
Executing the Rebase
Executing the `git rebase HEAD` command involves a few straightforward actions. After preparing your branch, simply run the command:
git rebase HEAD
This initiates the rebase process, moving your changes on top of the newest commit.
Handling Conflicts
What are Merge Conflicts?
During a rebase, if two branches have made changes to the same line or file, conflicts can arise. In such cases, Git will pause the rebase and prompt you to resolve these conflicts before continuing.
Resolving Conflicts During Rebase
To resolve conflicts, you'd generally follow these steps:
- Check the status to identify conflicting files.
git status # Check for conflicts
- Edit the files to resolve conflicts as necessary.
- Once conflicts are resolved, stage the changes.
git add <file>
- Finally, continue the rebase process using:
git rebase --continue
Git will then apply the rest of the changes after the conflict resolution.
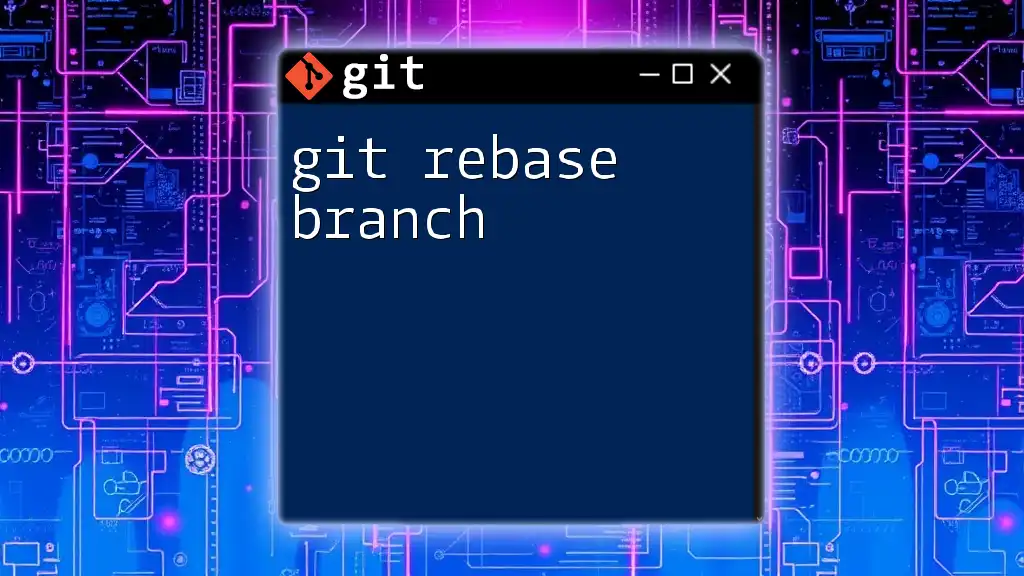
Reverting a Rebase
Undoing a Rebase with `git reflog`
If you encounter issues after a rebase, you can utilize `git reflog` to reference previous commits in your repository. This command maintains a log of where your HEAD pointers have pointed previously, enabling easy recovery.
Rolling Back Changes
If a rebase isn't what you wanted, you can safely roll back using:
git rebase --abort
This command will restore your branch to its state before the rebase began.
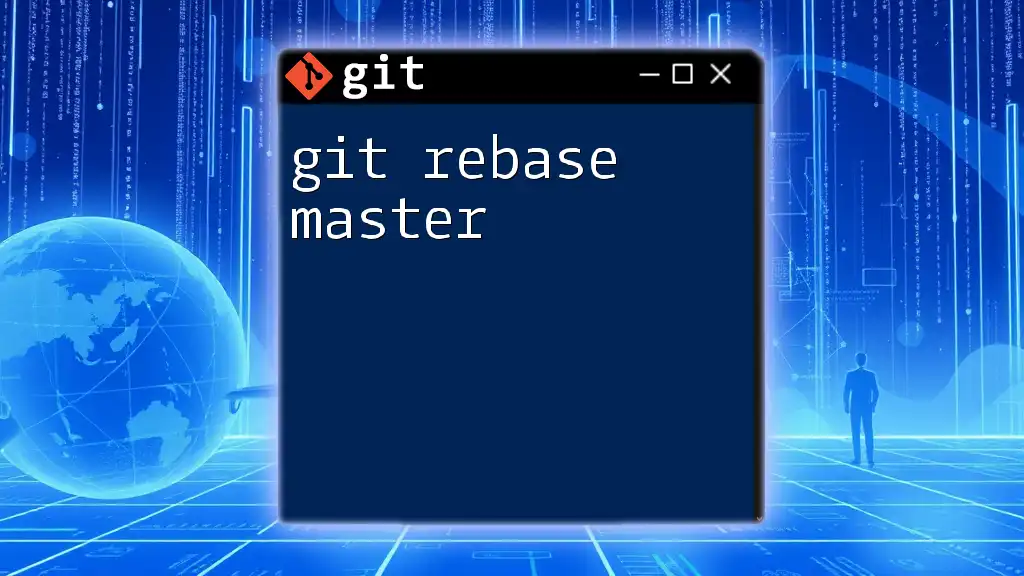
Best Practices for Using `git rebase HEAD`
When using the `git rebase HEAD` command, consider the following best practices:
- Use rebase before merging to clean up your commit history.
- Avoid rebasing public branches that may be used by others since this can lead to confusion and conflicts.
- Always make backup branches before performing complex rebases or when unsure of the outcomes.
- Practice on a local branch to build confidence with rebase and understand the implications thoroughly.
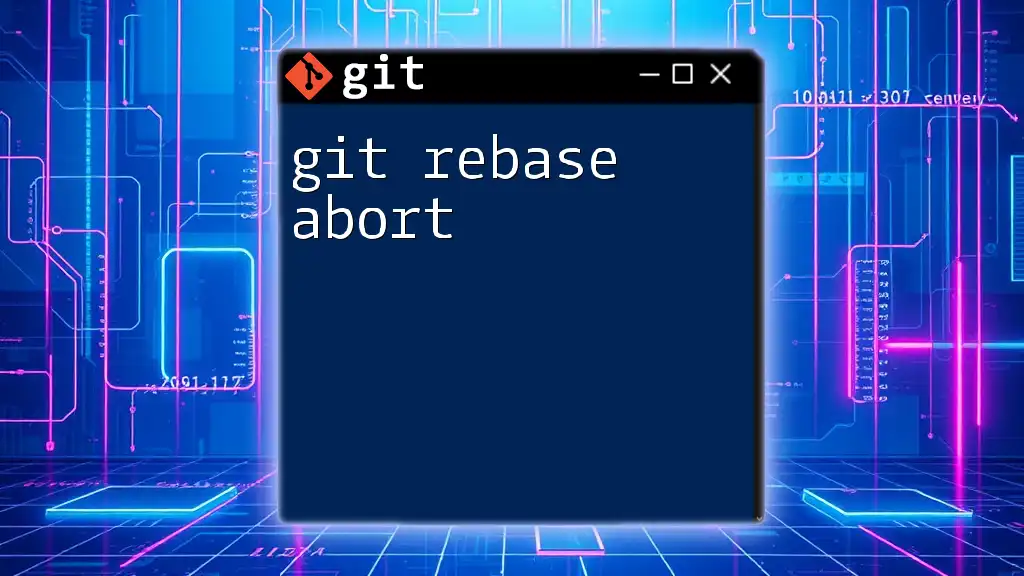
Conclusion
Understanding and mastering the `git rebase HEAD` command is essential for maintaining a tidy and understandable commit history. By leveraging the power of rebasing, you can streamline your workflows and present a cleaner project history. It's always encouraged to practice rebasing in various scenarios to fully grasp its nuances and apply it effectively in your development process.
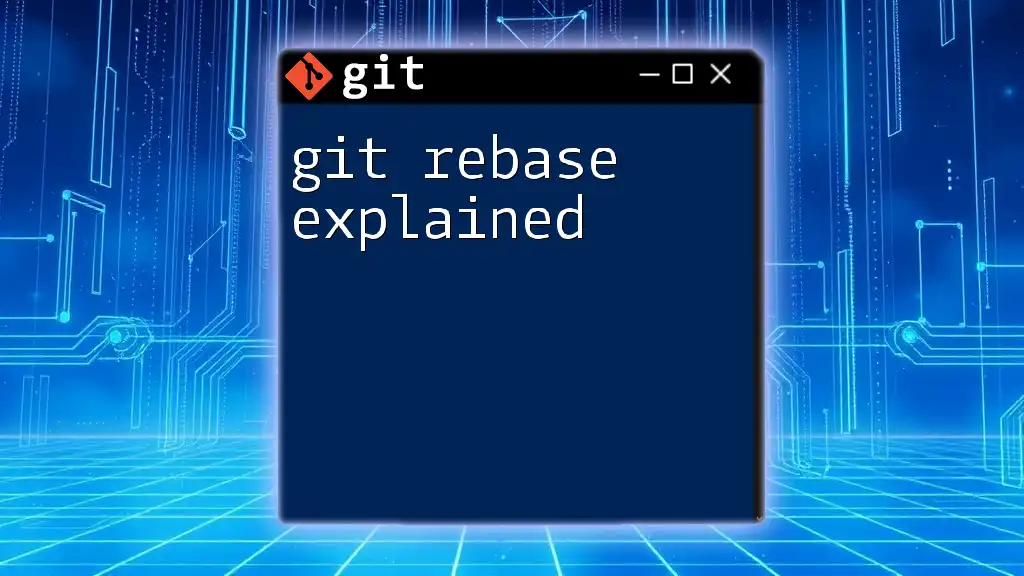
Additional Resources
For further learning, consider exploring the official Git documentation. This resource offers deeper insights into more complex rebasing techniques, as well as a comprehensive understanding of Git and version control systems.