The `git reset HEAD -1` command undoes the most recent commit, moving changes back to the staging area without deleting any content, allowing for easy modifications before a new commit.
git reset HEAD -1
Understanding Git Reset
What is `git reset`?
`git reset` is a powerful command in Git that allows you to change where the HEAD points in your repository. It can affect the staging area and the working directory, depending on how you use the command. Essentially, it helps you manipulate the state of your commits by altering the pointer to a particular commit.
Key Concepts
- HEAD: This is a pointer that usually points to the latest commit you are working on in your current branch.
- Index: Known as the staging area, this is where changes are prepared before being committed.
- Working Directory: This reflects the files in your project folder as they currently exist, which may differ from the index and HEAD.
Types of `git reset`
Soft Reset
A soft reset moves the HEAD to a specified commit but leaves the index and working directory unchanged. This is useful when you want to undo a commit but keep your changes staged for the next commit.
Mixed Reset
The default type when you use `git reset` is a mixed reset. It moves the HEAD pointer and resets the index but leaves the working directory intact. It’s great for unstaging changes while keeping your work.
Hard Reset
A hard reset is a more drastic option. It resets the HEAD, index, and working directory to the specified commit, discarding all local changes. This can be dangerous, as it permanently deletes your changes.
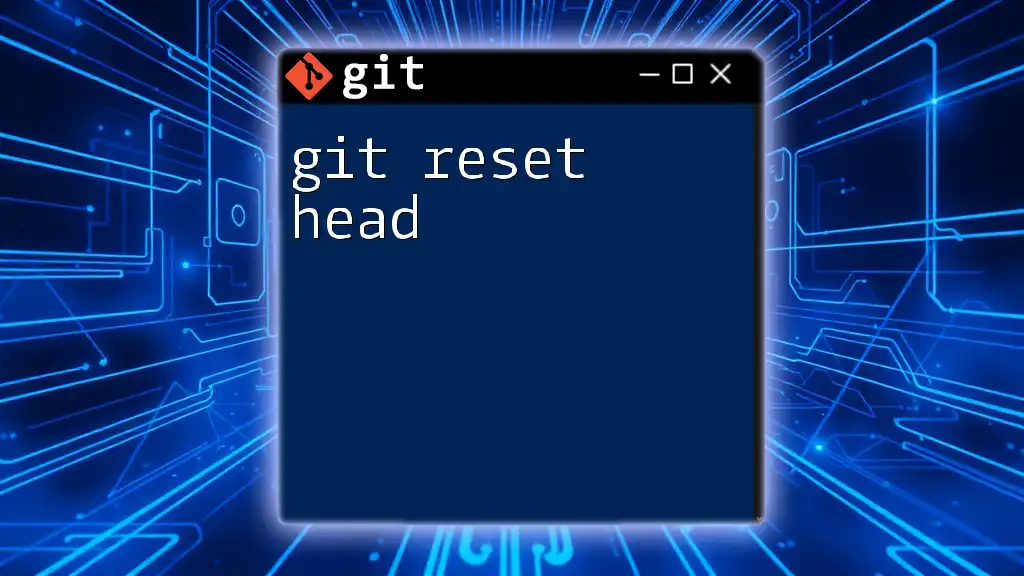
Delving into `git reset HEAD -1`
What Does `HEAD -1` Mean?
When using the `git reset` command, `HEAD -1` refers to the most recent commit (HEAD) and moves the pointer back by one commit. This designation offers a shorthand way to specify the commit to which you want to revert.
The Command Syntax
The command you will use is straightforward:
git reset HEAD -1
This command instructs Git to move your HEAD pointer back one commit, while keeping your current changes in the working directory.
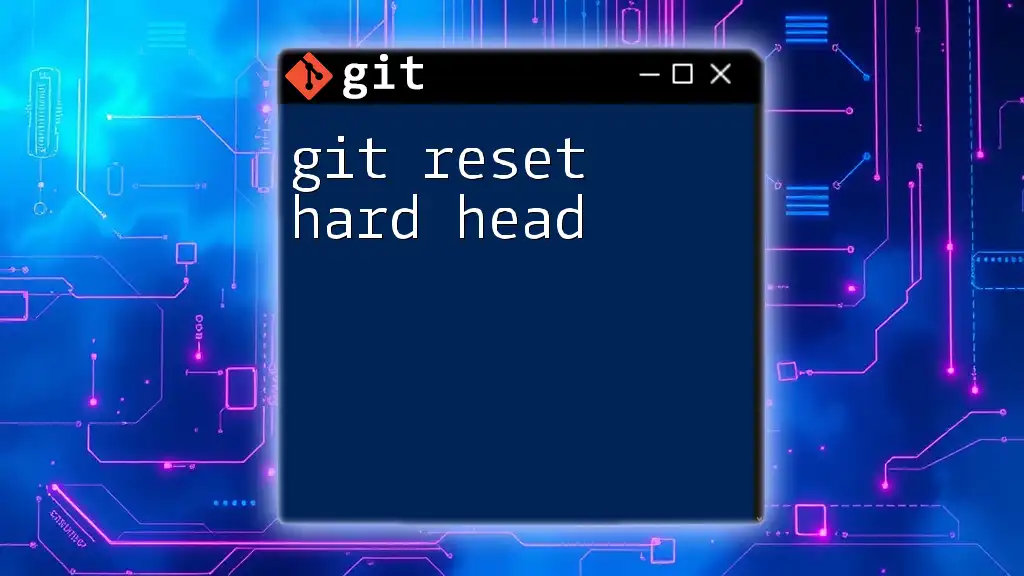
Use Cases for `git reset HEAD -1`
Reverting Local Changes
Scenario: Undoing the Last Commit
If you’ve just committed changes and realized that the commit should not be there (perhaps because of a typo in your commit message or an accidental inclusion of a file), you can use `git reset HEAD -1` to revert that commit without losing your modifications.
Example: A Step-by-Step Guide
- Check your commit history to confirm your last commit:
git log
- Use the reset command:
git reset HEAD -1
- Your files will now be unstaged, and you can make necessary corrections before recommitting.
Cleaning Up Changes Staging Area
Scenario: Unstaging Changes
If you mistakenly staged a file, or perhaps changed your mind about including it in the upcoming commit, you can unstage it with the command:
git reset HEAD -1 <file_name>
This operation brings the specified file back to the working directory, allowing you to continue modifying it without committing changes prematurely.
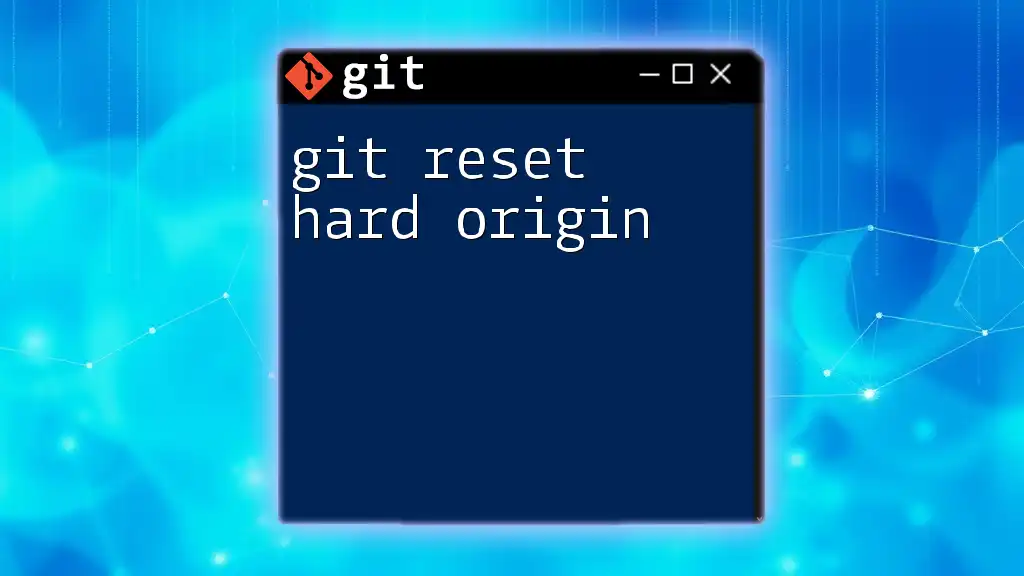
Effects of `git reset HEAD -1`
Changes to the Working Directory
Executing the command `git reset HEAD -1` modifies the staging area but preserves your changes in the working directory. This means that any modifications to files from the last commit are left untouched, and you will have the opportunity to either fix issues or adjust them as needed.
Common Pitfalls
- Forgetting What You’ve Reset: It's crucial to remember that this command doesn’t erase your changes; it just unstages them. Misunderstanding this can lead to confusion.
- Accumulating Uncommitted Changes: Frequent usage without planning can result in cluttering your working directory with too many uncommitted changes, making it hard to keep track of your project’s state.
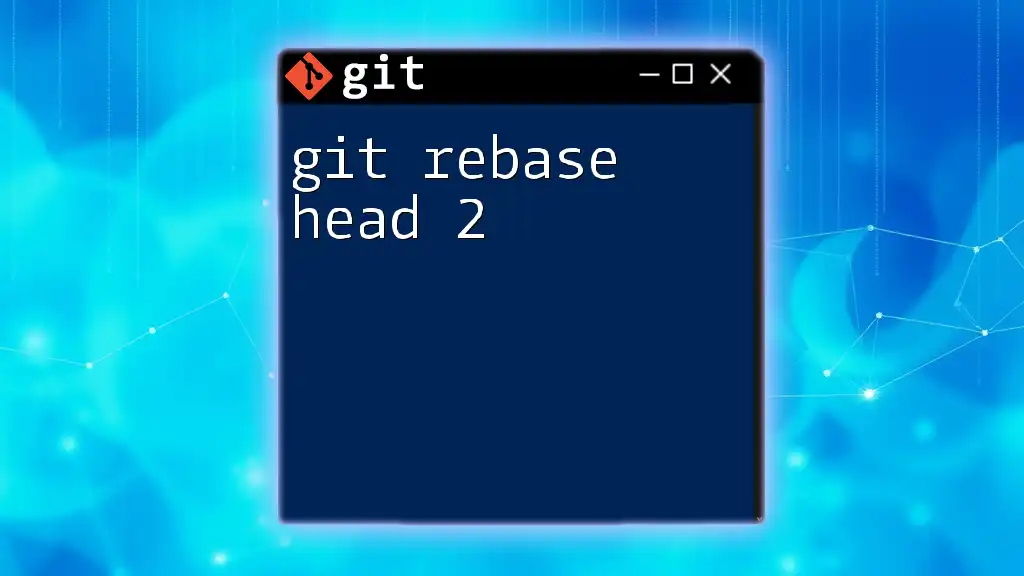
Best Practices
How to Safely Use `git reset`
Before using `git reset`, particularly if you’re not sure how it will affect your project, consider creating a backup. One effective way is to create a temporary branch:
git branch temp-backup
This allows you to preserve your current state before making any resets.
Documenting Changes
Always use clear commit messages when making your commits. This helps not only you but also others who may look through the history of changes you’ve made. Precise commit messages make tracking changes more manageable, especially when using commands like `git reset HEAD -1`.
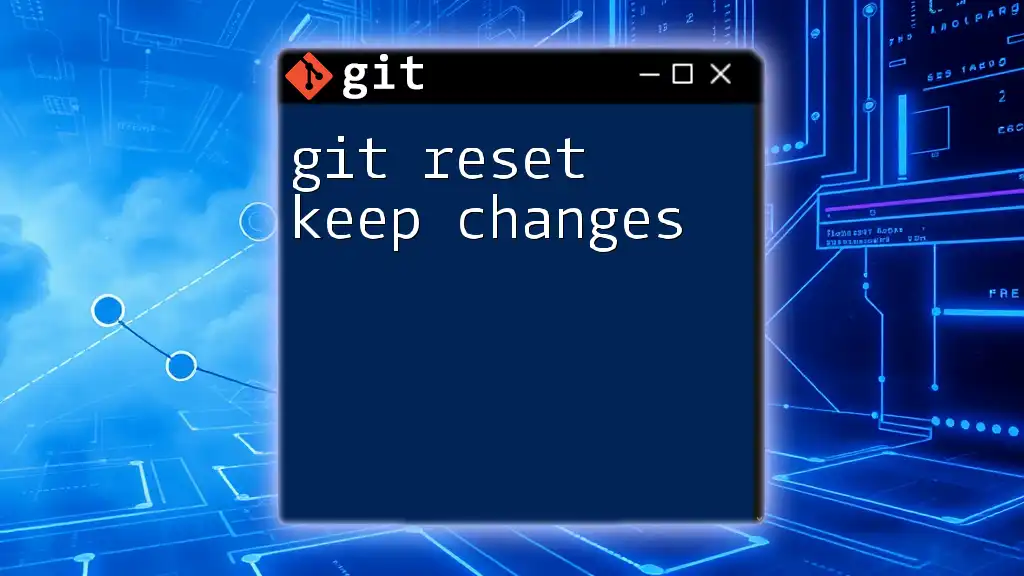
Conclusion
In summary, understanding how to use `git reset HEAD -1` effectively is essential for any developer looking to manage their commit history and maintain control over their working directory. This command can be a lifesaver when properly understood and utilized.
Call to Action
Don’t hesitate to practice `git reset HEAD -1` in a safe environment; play around with it to grasp how it functions. Experimenting with a test repository can help solidify your understanding of this important Git command.
Frequently Asked Questions
What is the difference between `git reset HEAD -1` and `git reset HEAD~1`?
While both commands achieve a similar goal, `HEAD -1` is a shorthand for the last commit, whereas `HEAD~1` is the first parent of the current commit. They are subtly different in context but often lead to the same result.
Can I recover from a bad reset?
If you've made a mistake using `git reset`, you can generally recover your commits if they are still referenced. You may use the `git reflog` command to view your references to recently deleted commits and restore them.
How do I combine `git reset` with other Git commands?
`git reset` can be used in conjunction with commands like `git stash` or `git checkout` to help manage more complex workflows, particularly when dealing with larger projects.