To undo changes in your Git repository, you can use the `git reset` command, which allows you to move the current branch's HEAD to a specified state, discarding changes in the staging area or working directory as needed.
git reset --hard HEAD~1
Understanding `git reset`
What is `git reset`?
`git reset` is a powerful command used in version control to reset the state of your Git repository. By using this command, you can move the HEAD pointer to a specific commit, thereby altering the staging area and, in some cases, your working directory. Knowing how to wield this command effectively is essential for any Git user, as it allows for greater control over your project's history.
It's crucial to distinguish `git reset` from `git checkout` and `git revert`. While `git checkout` allows you to switch branches or restore files, `git revert` creates a new commit that undoes changes made by a previous commit without altering the commit history. In contrast, `git reset` changes the pointer of the HEAD and can affect the staging area and working directory.
Types of `git reset`
There are three primary types of reset you can perform, each serving a different purpose:
Soft Reset
A soft reset changes HEAD to a specified commit, but it keeps the current index (staging area) unchanged. This means that all changes made after the commit you reset to remain in the staging area as if they were never committed.
When to use soft reset:
When you realize that you want to modify or amend your last commit without losing your changes.
Example:
git reset --soft HEAD~1
This command resets the HEAD to the previous commit but retains all files in the staging area, allowing for a modification of the commit message or content easily.
Mixed Reset
The mixed reset is the default behavior of `git reset`. It resets the HEAD to a specific commit and updates the index to match that commit while leaving the working directory unchanged. This is useful when you want to unstage files but not discard any changes in your working directory.
When to use mixed reset:
When you want to unstage files that were mistakenly added.
Example:
git reset HEAD~1
This command moves HEAD back one commit and moves the changes from the index to your working directory. Your files will still reflect the modifications, but they will need to be staged again for a new commit.
Hard Reset
The hard reset goes a step further by resetting both the index and working directory to match the specified commit. This means any changes made after the commit will be lost.
When to use hard reset:
You need to discard all changes after a certain commit completely.
Example:
git reset --hard HEAD~1
This command reverts the repository back to the previous commit, discarding all changes made since. Please use this command cautiously, as it can lead to irreversible data loss if you haven't backed up your work.
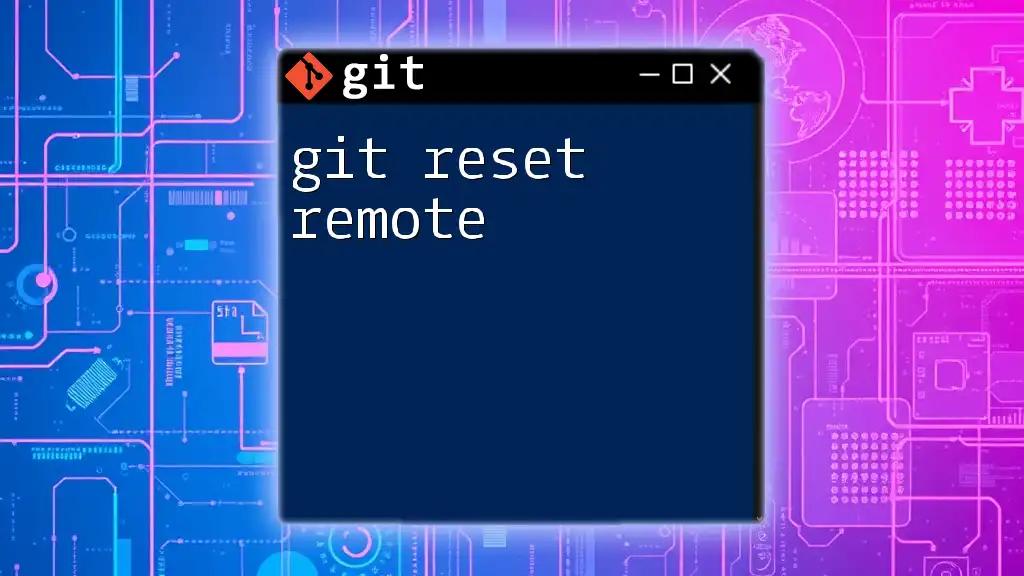
Why You Might Want to Undo Changes
Common Scenarios for Using `git reset`
Several common scenarios prompt the use of `git reset`:
-
Accidental commits: If you accidentally commit sensitive information such as credentials or configuration files, a reset can help you remove that commit from your history.
-
Incorrect commit messages: If you realize that the message of your last commit is misleading or erroneous, a soft reset allows you to correct it easily.
-
Merging the wrong branch: Mistakenly merging an undesirable branch can be undone through a reset to bring your project back to a stable state.
-
File modifications: After realizing that certain files should not be part of the commit, a simple reset can help you unstage them.
Alternative Methods to Undo Changes
While `git reset` serves as a robust method to undo commits, it's not the only option available.
Overview of `git revert`:
`git revert` creates a new commit that undoes the changes made in previous commits while preserving the original commit history. This method is particularly useful when collaborating with others to maintain a clear record of all actions taken in the repository.
Example of `git revert`:
git revert <commit_hash>
Using this command will generate a new commit that reverses the changes from the specified commit, ensuring a cleaner and more collaborative approach to undoing mistakes.
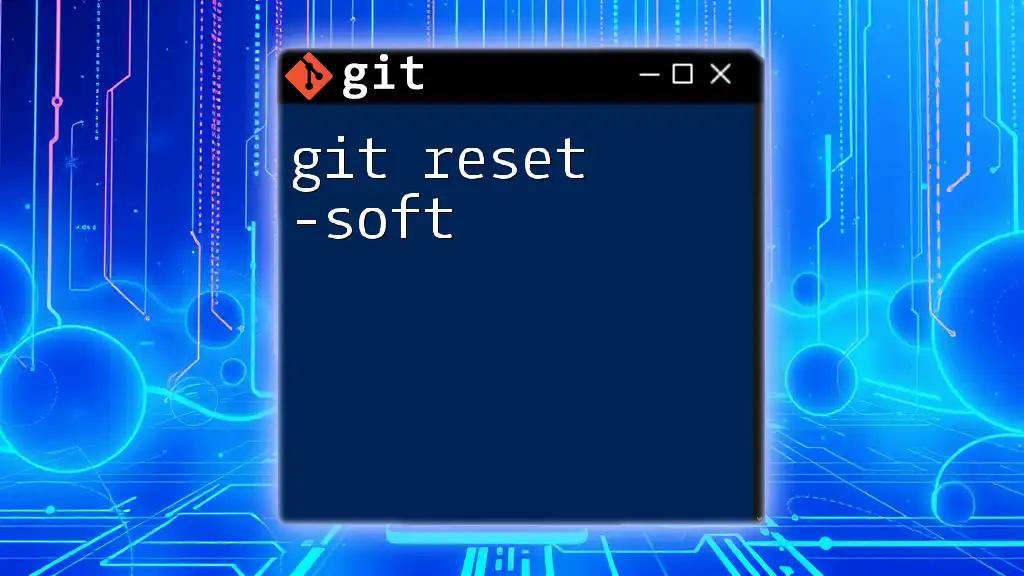
How to Safely Undo Commits with `git reset`
Best Practices Before Resetting
Before executing a reset, it's essential to adopt best practices to safeguard your work:
-
Importance of backups: Always create a new branch to store your changes before resetting. This precaution ensures you can return to your prior work if needed.
-
Utilize `git stash`: Stashing allows you to save your current progress temporarily without committing it. Use this command:
git stash
This is particularly useful when you are unsure about your next steps.
- Confirm the current branch and status: Always check your current state before making significant changes:
git status
This command helps build confidence in understanding where you are in your project.
Step-by-Step Guide to Using `git reset`
- Identifying the commit to reset: Use `git log` to find the commit you want to revert to:
git log --oneline
This command will list recent commits abbreviated for easy identification.
-
Execute the appropriate reset command: Depending on the situation, choose whether to perform a soft, mixed, or hard reset.
-
Verify the results: After executing the chosen reset command, always check your repository's status:
git status
You can also use:
git log --oneline
These commands will help confirm that your HEAD is at the desired commit and that your staging area reflects your intentions.
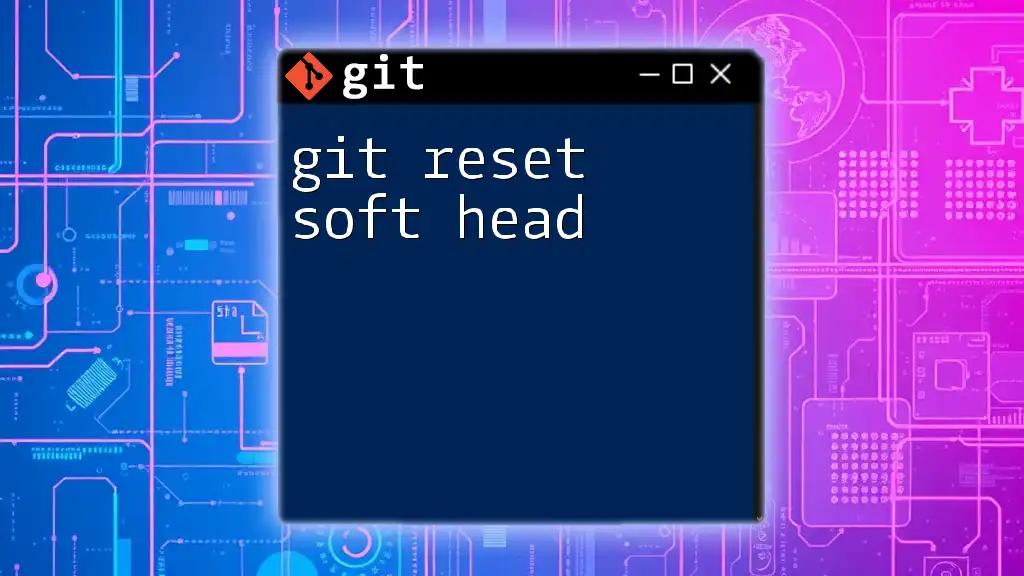
Common Mistakes to Avoid
Unintended Data Loss
One of the largest risks of using `git reset` is the potential for data loss. Understanding the different types of resets is critical; choosing a hard reset without prior consideration can lead to a complete loss of changes.
Forgetting Staged Changes
When using mixed or soft resets, users often forget that this action may leave staged changes that are not yet committed. Always double-check your index and working directory after the reset to ensure no unintended changes remain.
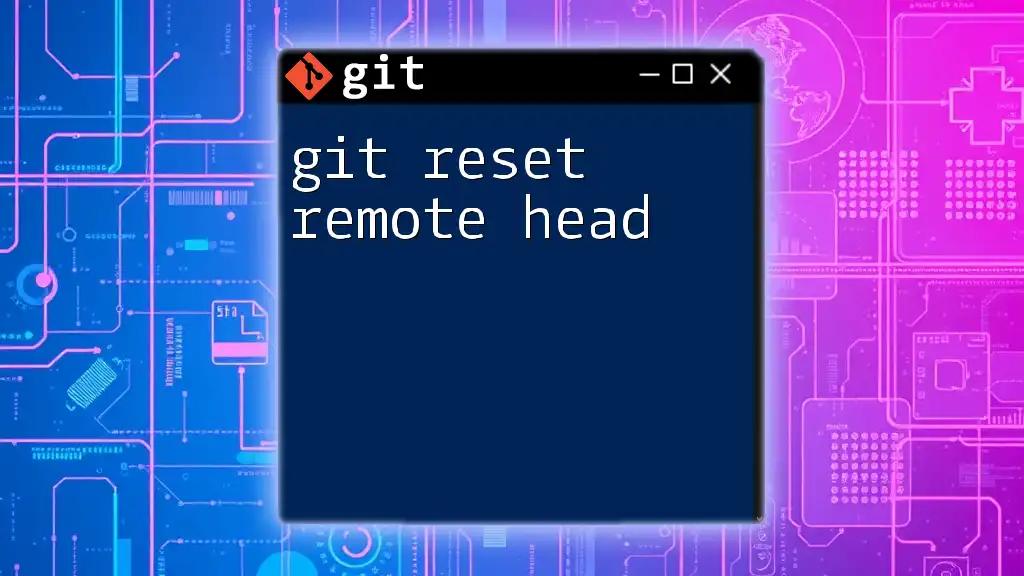
Recovering Lost Commits
How to Use `git reflog`
A powerful feature for recovering lost commits is `git reflog`. This command records changes made to the branches and can help you find "lost" commits after a hard reset.
Example usage:
git reflog
By running this command, you can see a log of your HEAD history. If you find a commit you want to restore, you can reset HEAD back to that commit using:
git reset --hard <commit_hash>
This action will restore the state of your repository to that point in time.
Conclusion
The command `git reset undo` is an essential tool for managing your version control history effectively. Understanding how to use the different types of resets allows you to tailor your version control to your needs, whether that be amending a commit message or recovering from mistakes.
Remember to practice these commands in a safe environment, familiarize yourself with the risks, and employ best practices for a successful Git experience. Whether you are a beginner or an experienced developer, mastering `git reset` is invaluable for maintaining control over your projects.
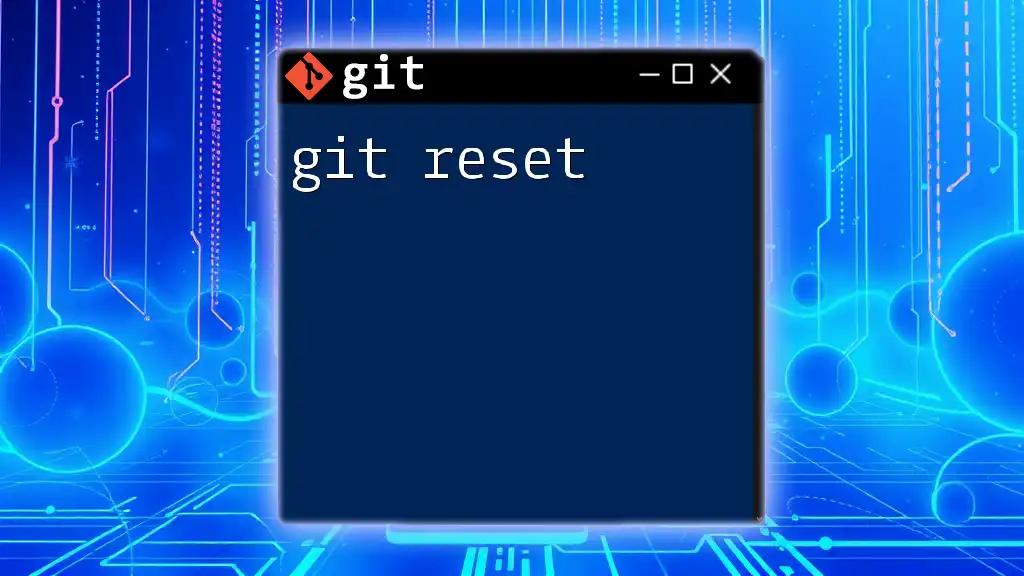
Additional Resources
For more information, refer to the official Git documentation for a thorough understanding of version control techniques. Consider exploring tutorials that delve into more advanced topics and providing guides to tools that can complement your Git workflow.
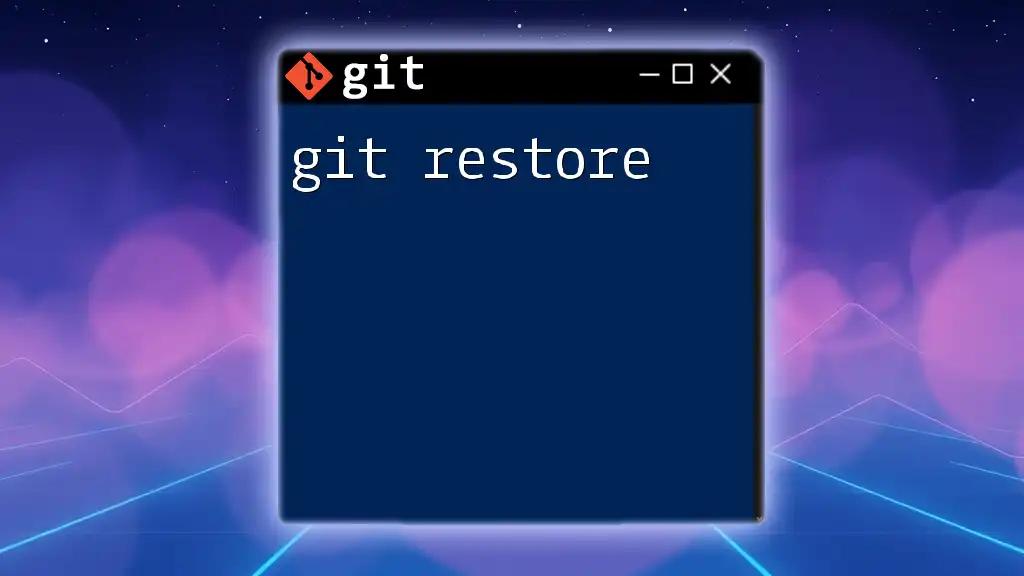
Call to Action
Follow us for more tips and tricks on leveraging Git commands effectively. We’d love to hear about your experiences using `git reset`—feel free to share your thoughts in the comments or across social media!