The `git reset remote HEAD` command is used to reset your local repository's HEAD to match the latest commit on the remote branch, effectively discarding your local changes and aligning with the remote state.
git fetch origin
git reset --hard origin/main
Understanding Git Reset
What is `git reset`?
The `git reset` command is a powerful tool in Git utilized to move the current HEAD to a specified state. This command helps you unstage changes or revert your repository to an earlier commit, allowing you to manage your project history more effectively. Git reset primarily operates in three different modes:
- Soft: Moves the HEAD pointer to a specified commit, but keeps all changes in the staging area. This is useful when you want to amend commits.
- Mixed (default): Moves the HEAD pointer and unstages the changes. The changes remain in the working directory, letting you edit them further.
- Hard: Resets the HEAD pointer and discards all changes in the staging area and working directory. This should be used with caution, as it permanently deletes your work.
How does `git reset` work?
When using `git reset`, it's crucial to understand how it alters the staging area and the working directory. A reset only affects the commits and the staging area unless it is accompanied by the `--hard` option, which also affects the working directory.
It's important to note that `git reset` operates locally, meaning the changes you make do not automatically affect the remote branch. Understanding how it interacts with the local repository is essential for effective usage.
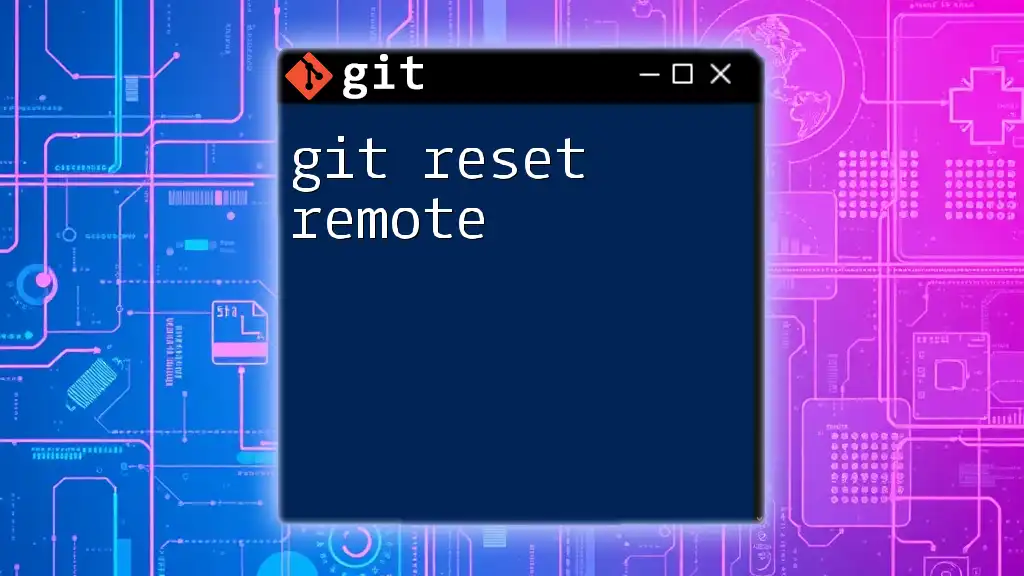
The Concept of Remote Head
What is a Remote Head?
In Git terminology, remote head refers to the tip of a branch in a remote repository. Understanding this concept is vital for collaborative development. The term `HEAD` points to the current commit your working directory is based on. When working with teams, the remote head acts as a reference point, helping you align your work with others.
How to Check the Current Remote Head
To examine the current state of the remote head, use the following command:
git ls-remote <remote-name>
This command retrieves references from a remote repository and displays the remote heads, enabling you to ensure you are working with the latest state of the project.
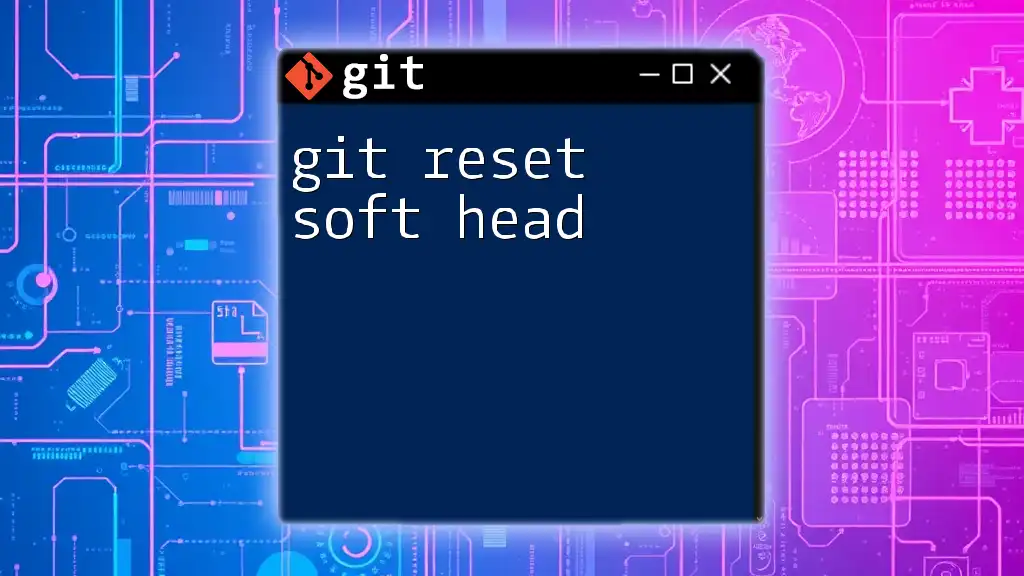
When to Use `git reset remote head`
Common Scenarios for Resetting Remote Head
Resetting the remote head is often necessary in scenarios such as:
- Removing mistakes post-push: If you've accidentally pushed wrong changes, resetting allows you to remove them from the remote history, ensuring a clean project state.
- Reworking features: If a feature requires major changes, resetting helps you realign your working state without the unnecessary clutter of previous commits.
Risks of Resetting Remote Head
While resetting the remote head can be beneficial, there are potential pitfalls. If not coordinated with your team, resetting may lead to conflicts or data loss. It’s essential to communicate and ensure that everyone is aware of the changes being made.
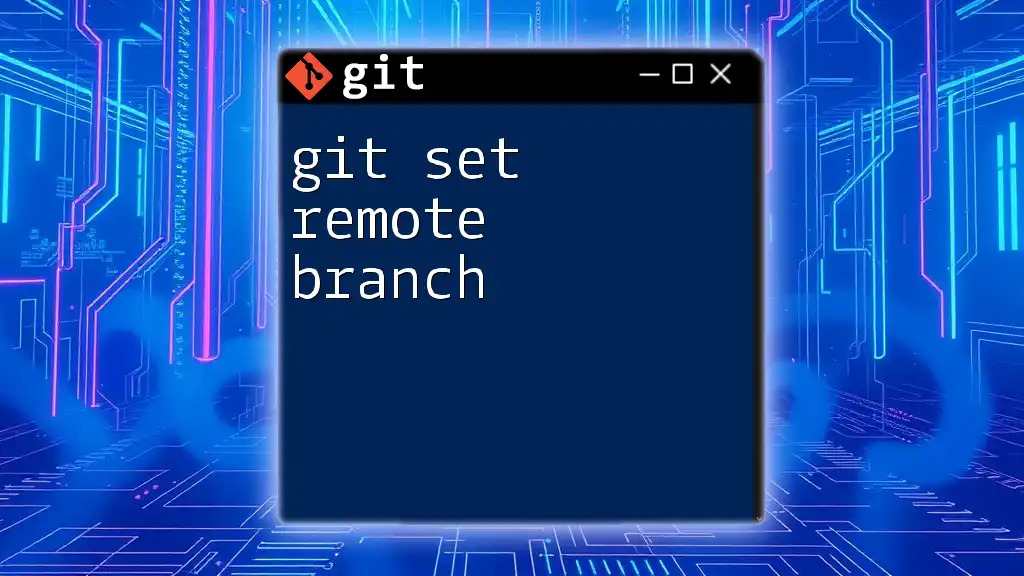
The Process of Resetting Remote Head
Step-by-Step Guide to Resetting Remote Head
To reset the remote head effectively, follow these steps:
- Determine the commit you want to reset to. You can find this with:
git log
- Reset your local branch to this commit. Here’s how to execute a mixed reset to remove changes from the staging area:
git reset origin/main
- If you want to make a hard reset, which clears both staging and working directories:
git reset --hard origin/main
- Push the changes to the remote repository. This is crucial to update the remote head. If you performed a hard reset, you will need to force the push:
git push origin HEAD --force
Confirming the Reset
After performing the reset, it's important to verify that everything functioned as expected. Use the following commands:
git status
git log
These commands will confirm your current branch state and verify that the HEAD now points correctly to your desired commit.
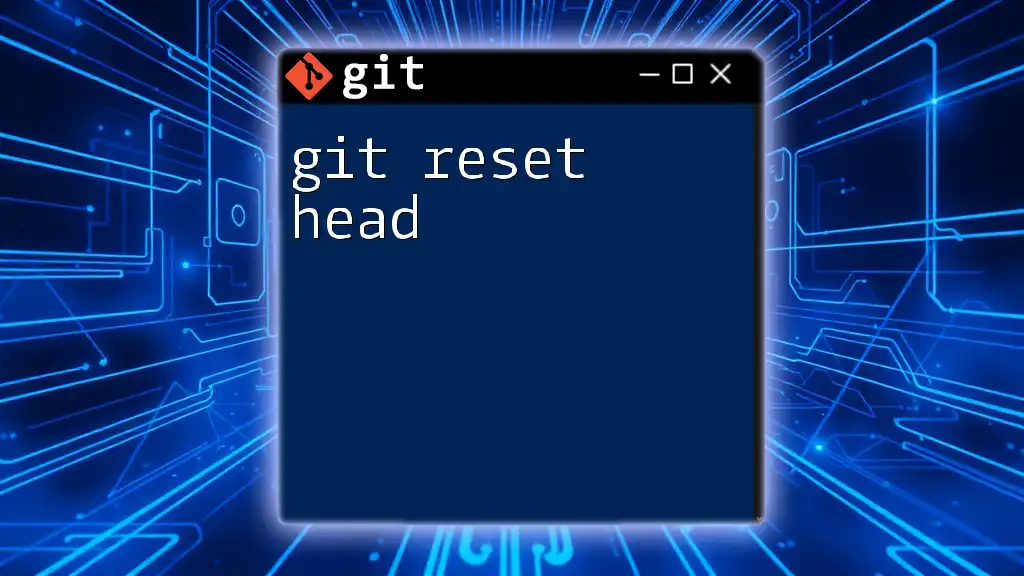
Alternative Approaches
Reverting Commits Instead of Resetting
Sometimes, it is better to use the `git revert` command to undo changes without altering the commit history. This method allows you to generate new commits that effectively cancel the effects of previous ones. For instance, if you want to revert a specific commit, you can use:
git revert <commit-hash>
This approach is particularly useful in collaborative environments where history integrity must be maintained.
Force Pushing After Reset
After completing a local reset, you may require a forced push to sync your changes with the remote repository. This is vital to ensure the remote head reflects your local state. Remember to communicate any changes to your team since a forced push can overwrite others’ work.
Collaboration Considerations
Before resetting the remote head, always consult with your team. Conflict resolution and coordination are critical in team projects. Establish a clear understanding of each developer's work to avoid potential complications.
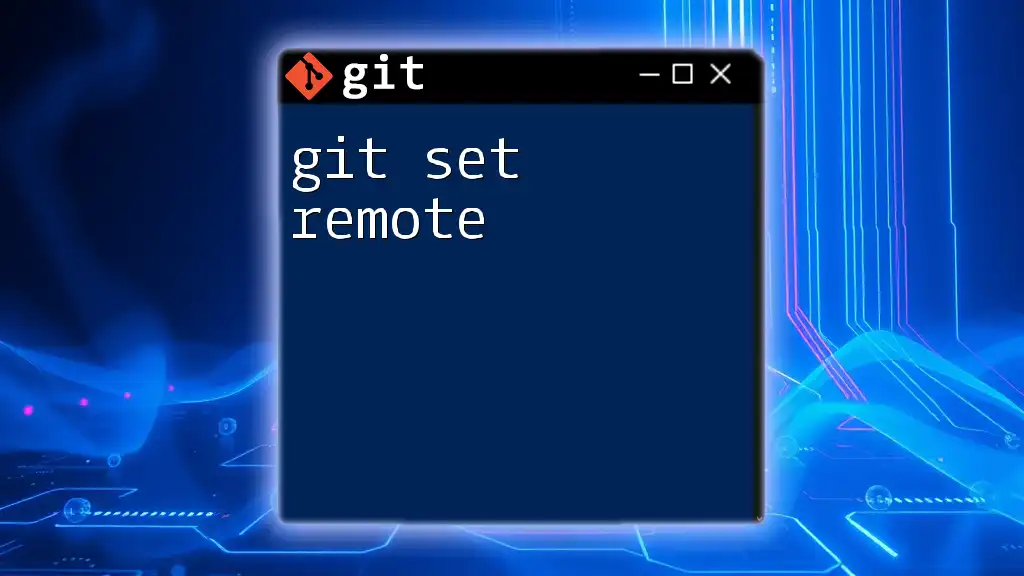
Troubleshooting Common Issues
What to Do When You Encounter Errors
While working with `git reset remote head`, you may face certain issues, such as conflicts when pushing changes after a reset. Here are common errors and tips to resolve them:
- Rejection due to non-fast-forward updates: If you encounter a message indicating your push was rejected, it may mean your remote branch has diverged. This requires a proper pull or rebase before pushing your changes.
- Uncommitted changes preventing a reset: Always check for uncommitted changes in your working directory. Stage or stash these changes before performing a reset.
How to Recover from a Mistaken Reset
If you accidentally reset your repository and want to recover lost changes, Git’s reflog can come to the rescue. You can view your previous HEAD states with:
git reflog
Using reflog, you can find the commit you want to revert back to and reset or checkout accordingly. This allows for a recovery of your previous state without losing valuable work.
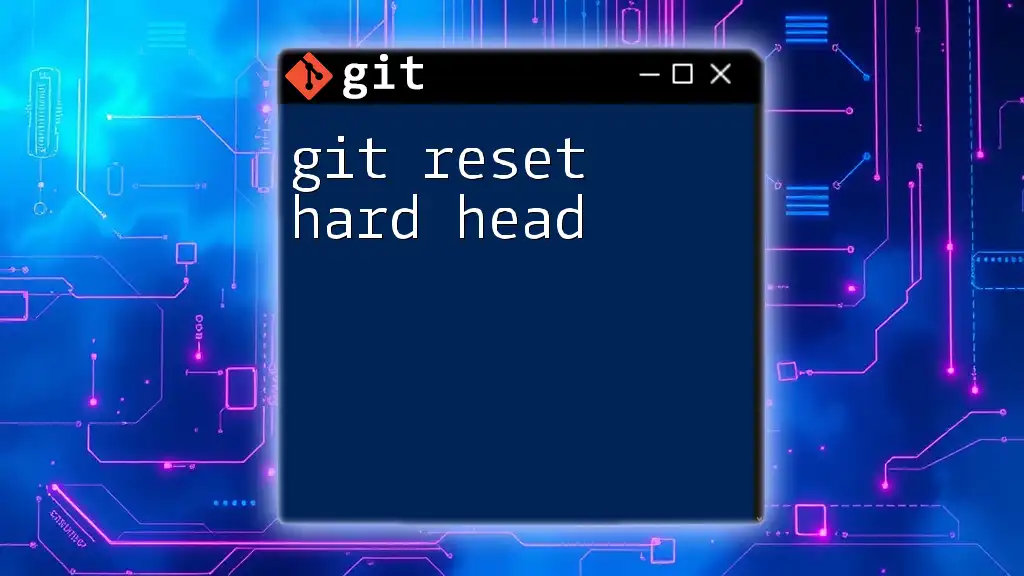
Conclusion
Understanding how to effectively use `git reset remote head` is essential for maintaining order in your Git workflow. By recognizing its utility, nuances, and potential pitfalls, you can navigate project histories confidently and collaborate effectively with your team. Practicing these commands in a safe environment will help solidify your Git skills and prepare you for real-world application. As you continue learning, consider exploring more detailed Git functions and commands to fully leverage this powerful version control tool.
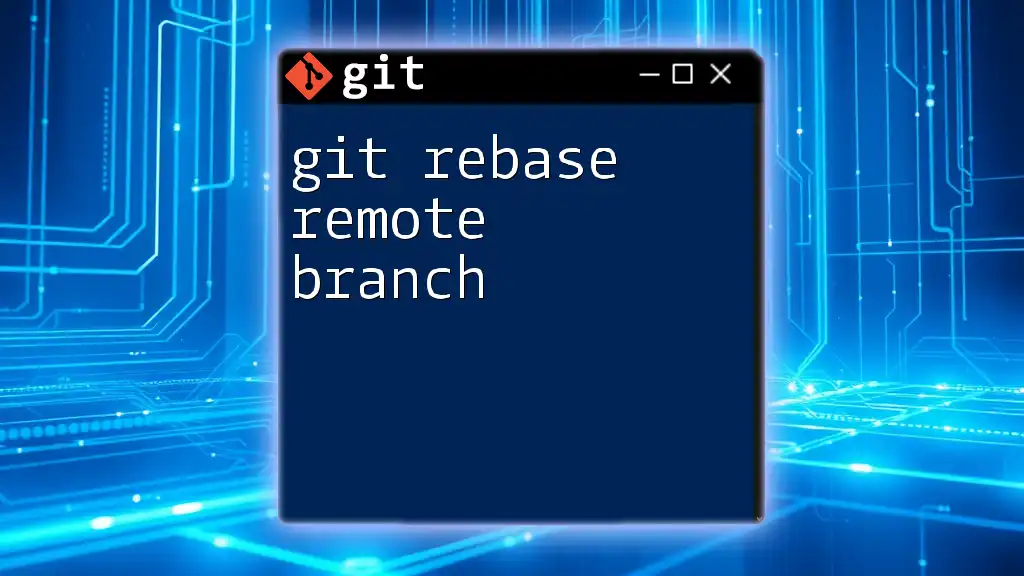
Additional Resources
For further exploration, check out the official Git documentation and consider engaging with online courses that provide in-depth tutorials on Git commands and workflows.