The `git reset --soft HEAD` command is used to reset the current branch to a specified commit while keeping your changes in the staging area, allowing you to amend them as necessary.
git reset --soft HEAD^
Understanding Git
What is Git?
Git is a powerful version control system that allows developers to track changes in their code over time. By managing a repository of code, Git helps teams collaborate effectively and maintain an organized record of modifications. Originally developed by Linus Torvalds in 2005 for Linux kernel development, Git has since become the industry standard for version control.
The Role of Commits in Git
In Git, a commit serves as a snapshot of the project at a particular point in time. Each commit records changes made to the code, providing a detailed history that allows developers to understand when and why changes were made. Every commit has a unique identifier, making it easy to reference specific points in time, which is crucial for debugging and collaboration.
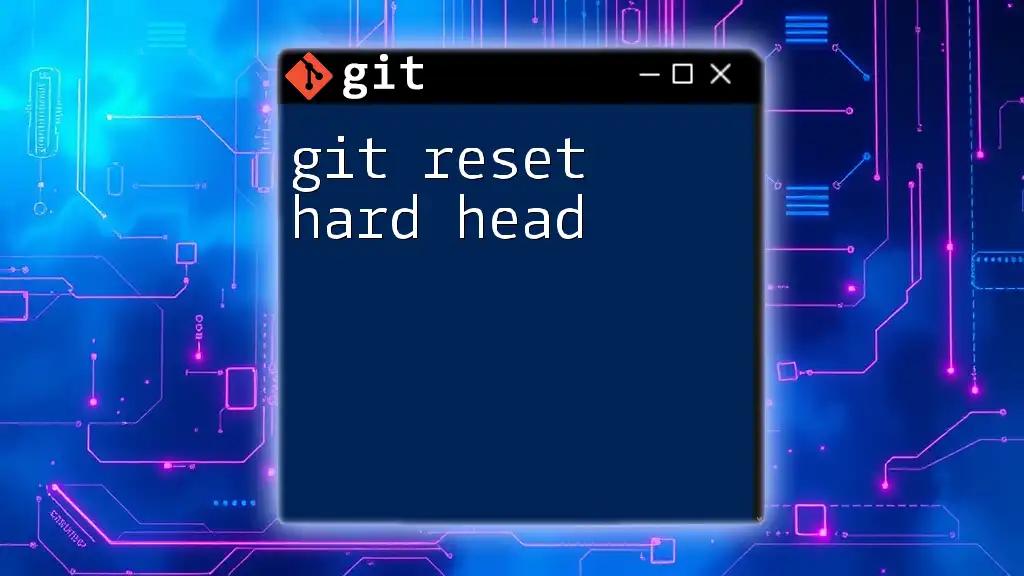
The Git Reset Command
What is git reset?
The `git reset` command is a versatile tool used to undo changes in a Git repository. Depending on the options used with it, `git reset` can modify the HEAD pointer, the staging area (index), or your working directory. The three main modes of `git reset` are:
- Soft: Moves the HEAD pointer but keeps changes in the staging area.
- Mixed: Moves the HEAD and unstages changes (the default mode).
- Hard: Moves the HEAD and resets the working directory to match the commit state, removing all changes.
Understanding HEAD
In Git, HEAD refers to the current commit that your working directory is based on. By pointing to this commit, HEAD serves as a reference for where you are in your project's timeline. This is crucial, as many operations—including resets—manipulate or move this pointer as part of their functionality.
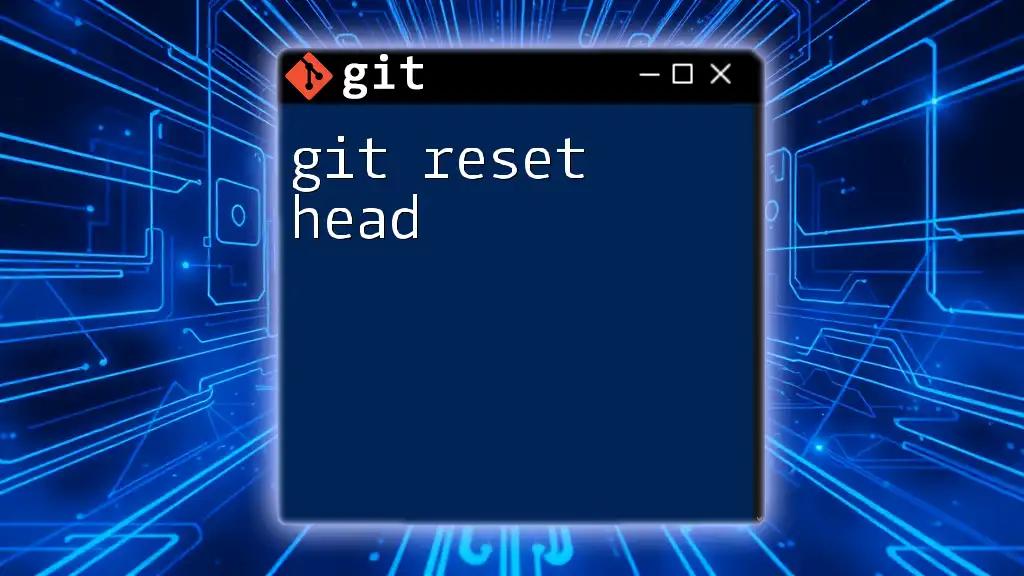
The Functionality of git reset soft HEAD
What does git reset soft HEAD do?
The command `git reset --soft HEAD` shifts the current HEAD pointer to a specified commit (or to the previous commit if none is specified) while preserving changes in the staging area. Unlike other reset modes that might erase or discard changes, the soft reset is a non-destructive way to "undo" commits but keep the modifications ready for a new commit.
Why Use git reset soft HEAD?
Using `git reset soft HEAD` is particularly useful in several scenarios:
- Undoing the last commit without losing changes: If you accidentally committed something too early or with mistakes, a soft reset allows you to rework the content while keeping it staged.
- Preparing changes for a new commit: You may want to refine or group changes into a single commit for clarity in your project history. A soft reset lets you do this seamlessly by keeping changes staged for easy re-commit.
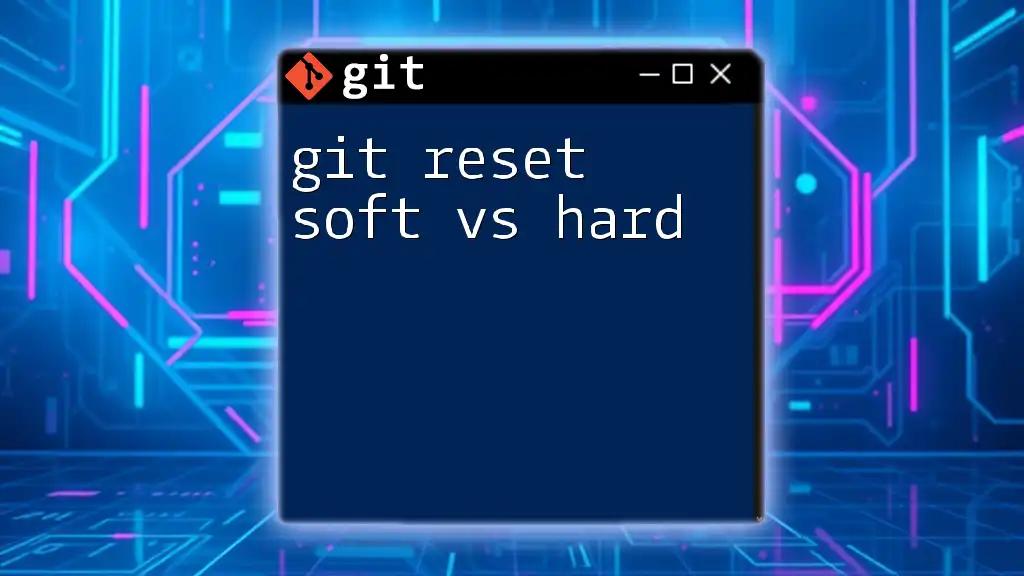
Practical Examples
Example 1: Undoing the Last Commit
Imagine you’ve just realized that the last commit should be revised. You want to keep your changes but remove the last commit from history. Here’s how to do it:
git reset --soft HEAD^
By executing this command, you move the HEAD to the previous commit. Your last commit will be undone, but the changes involved are still present in the staging area. You can now amend them as needed, making it a great option for minor adjustments without starting from scratch.
Example 2: Amending a Commit Message
If you need to correct a commit message, the soft reset can help. Here’s the process:
git reset --soft HEAD^
git commit -m "New commit message"
This snippet first undoes the last commit (keeping changes staged), then allows you to create a new commit with a more appropriate message. Since commit messages are vital for clarity in collaborative environments, this practice is a significant advantage of using soft resets.
Example 3: Combining Changes
Sometimes, you might want to combine several commits into one for a cleaner history. Consider the following command:
git reset --soft HEAD~2
git commit -m "Combined commit message"
This effectively resets your HEAD to two commits back, gathering the changes from both commits back into the staging area. The new commit then consolidates those changes into one unified entry, streamlining your project history.
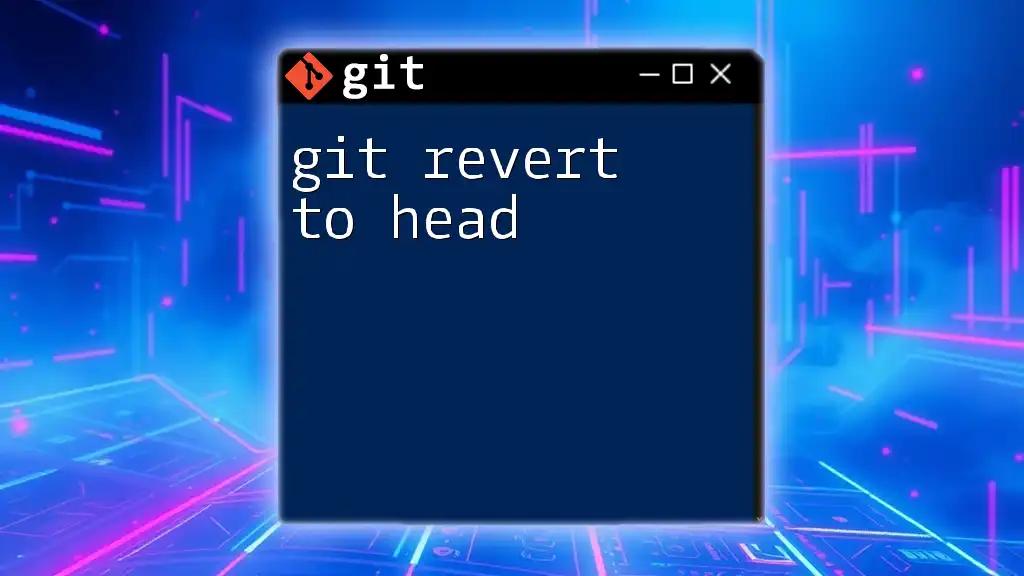
Potential Pitfalls
The Risks of Using git reset soft HEAD
While `git reset soft HEAD` is a powerful tool, it should be used with caution. Misusing this command can lead to confusion in project history. It’s important to fully understand the implications of modifying commits and to ensure that your team is on the same page to avoid conflicts.
How to Recover from Mistakes
If you find yourself in a situation where an unintended reset has occurred, hope is not lost! Git offers a method to recover lost commits using the `git reflog` feature:
git reflog
git checkout <commit_id>
Using `git reflog` allows you to see a log of moves made to HEAD, including those made by resets. By referencing the appropriate commit ID, you can return to a previous state and restore your work.
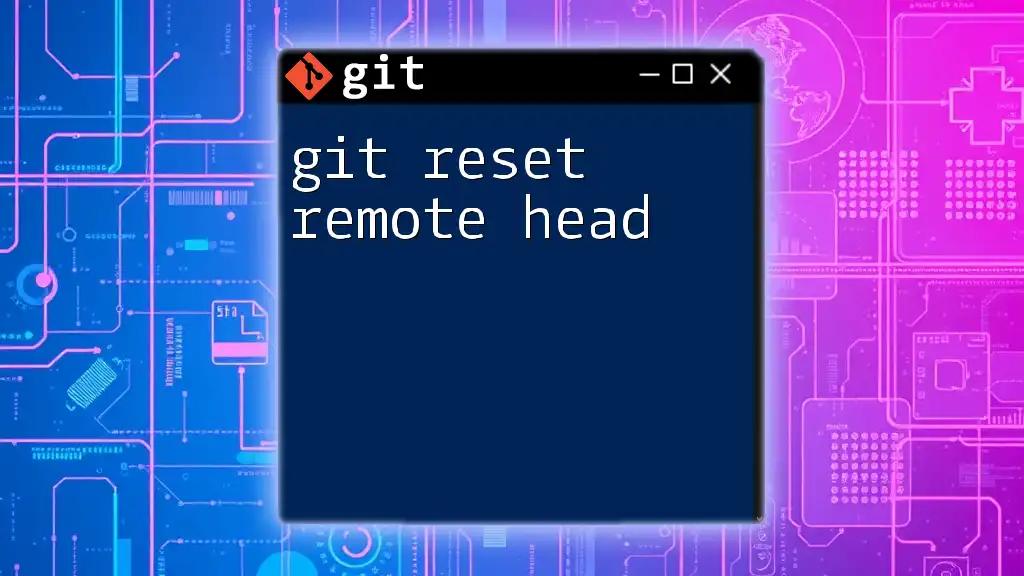
Best Practices
When to Use git reset soft HEAD
Understanding when to use `git reset soft HEAD` is key to harnessing its power correctly. Consider using it in scenarios like:
- Correcting minor issues in the most recent commit.
- Adjusting your staging area to refine your commit before pushing it to the repository.
Avoiding Common Mistakes
To mitigate risks, here are common mistakes to avoid with `git reset soft HEAD`:
- Failing to communicate with your team about changes made to commit history.
- Forgetting to ensure all important changes have been captured before performing a reset.
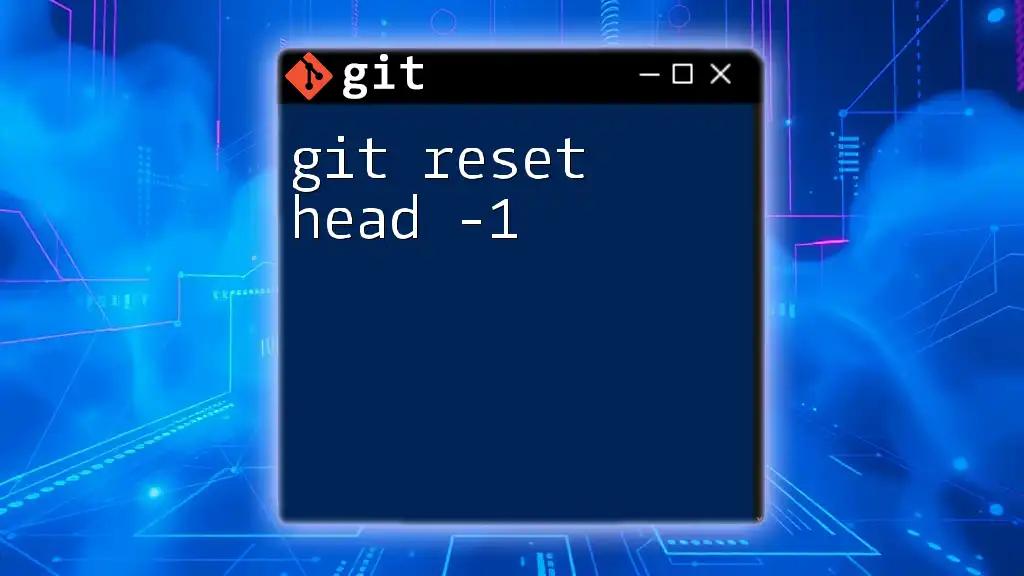
Conclusion
In summary, mastering `git reset soft HEAD` opens up a versatile approach for managing your commit history without sacrificing work. Practicing this command can enhance your control over project modifications and contribute to maintaining a clear, informative log of your development process. Don’t hesitate to experiment with the command in a safe environment to grow more comfortable with its implications.
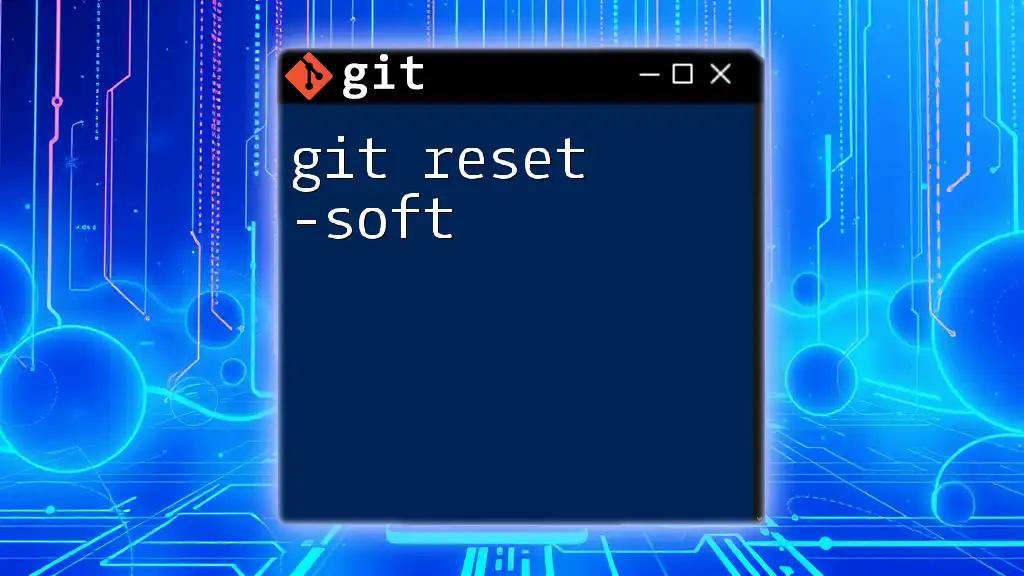
Additional Resources
For further exploration, consider checking out the official Git documentation, which provides in-depth information and advanced use cases. Additionally, books and online courses on Git can offer a deeper dive into effective version control practices.
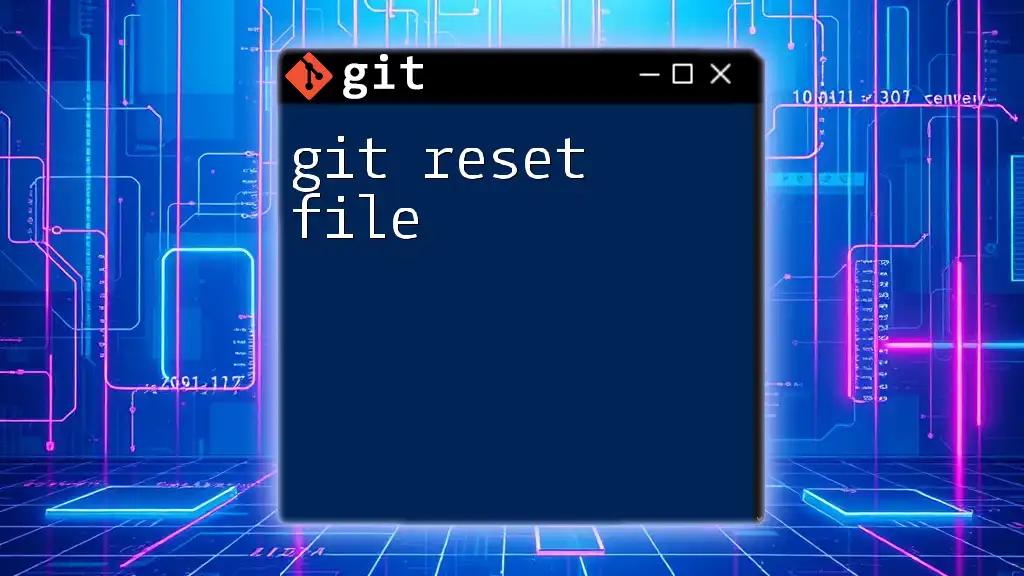
Call to Action
Stay tuned for more concise Git tutorials by subscribing to our newsletter, and don't miss out on our upcoming classes about mastering Git and version control in collaborative environments!