To reset your Git repository to a specific commit, use the command `git reset --hard <commit-hash>`, which will discard any changes after the specified commit and move the HEAD pointer back to it.
git reset --hard <commit-hash>
What is Git Reset?
Git reset is a powerful command that allows you to move the current HEAD of your repository to a specified state. It essentially changes the nature of your history by allowing you to "uncommit" or alter the state of your code at a specific point in time. Understanding how to use `git reset` effectively is fundamental when managing changes in a Git repository.
There are three primary modes of `git reset`, each serving a different purpose:
-
Soft Reset: This mode moves the HEAD to a specified commit while leaving your working directory and staging area intact. It is often used when you want to uncommit changes but keep them staged for further editing.
-
Mixed Reset: This is the default behavior of `git reset` and removes commits while moving changes to your working directory. Staged files from the previous commit will be un-staged and changes will remain in your working directory.
-
Hard Reset: This option not only resets the HEAD to a specified commit but also clears any changes in your working directory and staging area. This is powerful but should be used with caution as it can lead to irreversible loss of data.
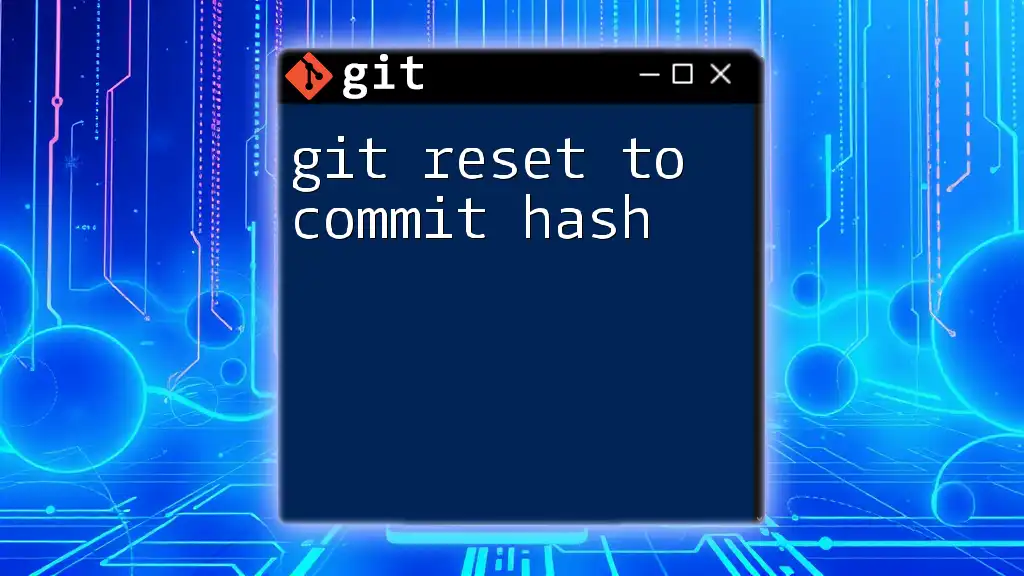
When to Use Git Reset
Resetting to a previous commit can be beneficial in various scenarios:
-
Undoing Mistakes: If you've made a commit with errors, `git reset` allows you to go back to a previous state without cluttering your history with additional "fix" commits.
-
Cleaning Up Commit History: If you realize that you’ve made several commits that should have been part of one cohesive change, `git reset` helps you refine your commit history.
-
Reverting Changes: Sometimes you might want to test new features or code but may decide to revert back when they don't work out. `git reset` provides a straightforward way to achieve this.
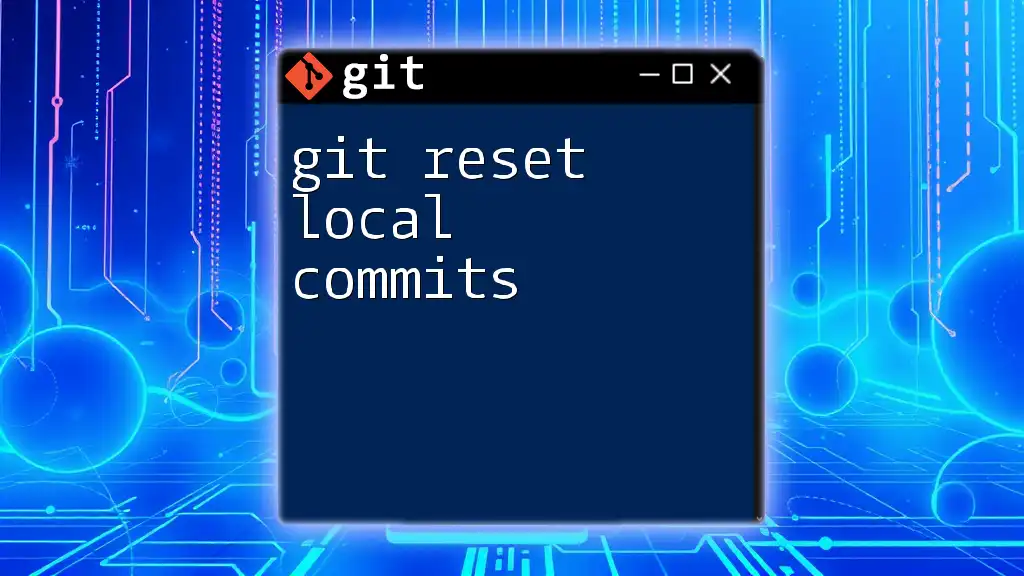
Understanding Commit IDs
Every commit in a Git repository is identified by a unique hash known as a commit ID. This ID is a string of hexadecimal digits that refers specifically to that commit, making it crucial when needing to reset or revert changes.
To find commit IDs, you can use the following command:
git log
This command provides a history of commits, displaying the commit ID, author, date, and commit message. Familiarizing yourself with this log will streamline the process of using `git reset to a commit`.
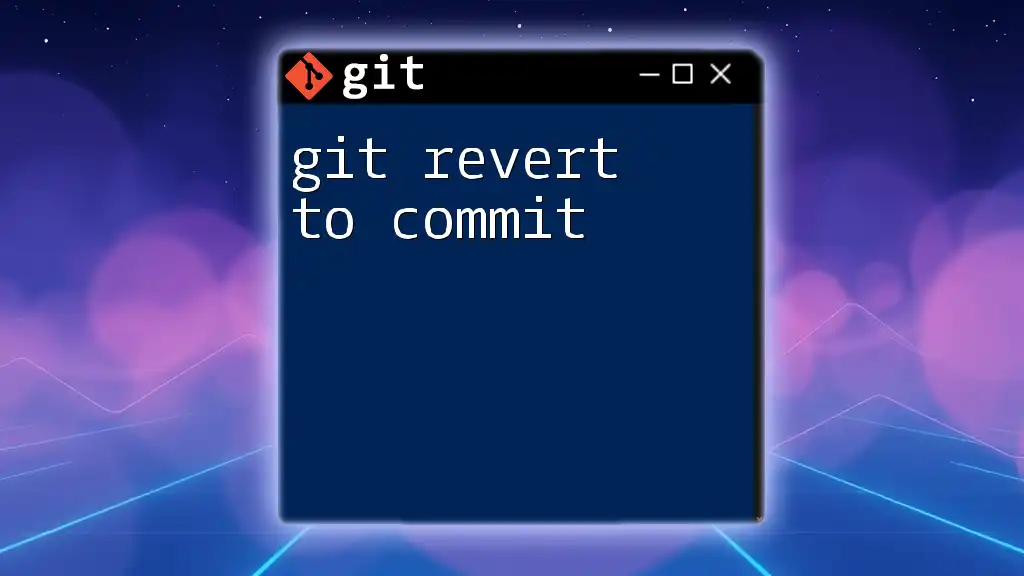
How to Use Git Reset
The basic syntax for the `git reset` command is as follows:
git reset <mode> <commit-id>
Git Reset Types
Soft Reset
When using a soft reset, you tell Git to reset the state to the specified commit while preserving the changes in your staging area. This is particularly useful if you want to make further modifications before recommitting.
Example:
git reset --soft <commit-id>
In this case, the specified changes will remain staged, allowing you to adjust them as needed. For instance, if you accidentally committed a file that should not have been included, you can perform a soft reset to remove that commit, fix the issue, and recommit.
Mixed Reset
The mixed reset (default mode) does the opposite by un-staging any changes but keeps them in your working directory. This allows you to track modifications without losing any work.
Example:
git reset --mixed <commit-id>
This is helpful when you need to clear your staging area for a fresh commit while keeping the changes to work on. It’s a common approach when selectively refining which changes should be committed.
Hard Reset
A hard reset is much more decisive as it resets both the index and working directory to the state of the specified commit. This results in the loss of all changes made after that commit, so it should be used with caution.
Example:
git reset --hard <commit-id>
Use this when you are sure you no longer need the changes made after the specified commit. A practical scenario could involve a corrupt state of your project where you want to roll everything back to a stable commit.
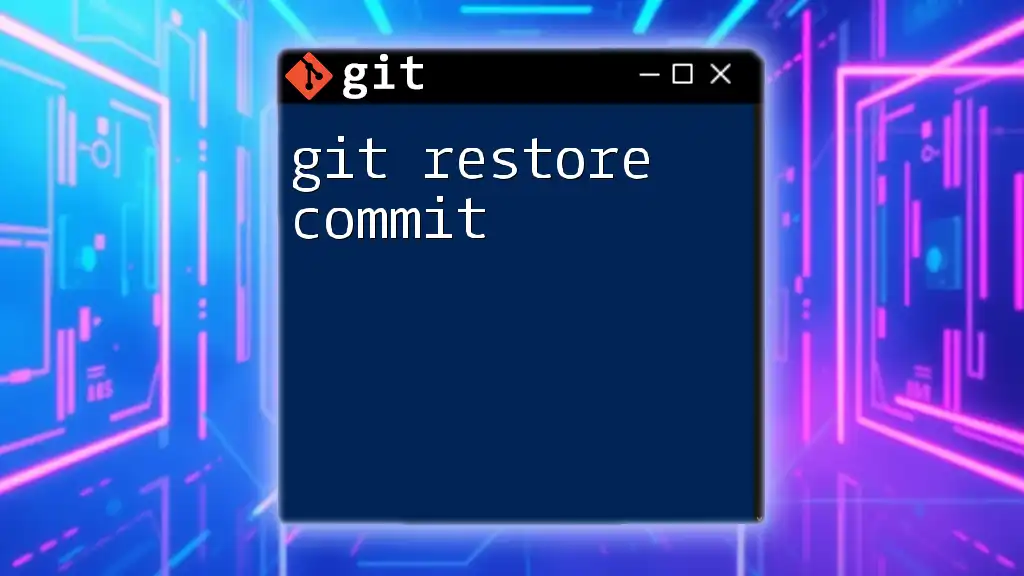
Examples and Use Cases
Resetting to a Specific Commit: Step-by-Step
-
Find the Commit ID: Start by checking your commit history to identify the commit you want to revert to. You can do this with:
git log
-
Perform the Desired Reset: Depending on your needs, choose your mode and execute the corresponding command.
- If you want to amend your last commit while keeping changes staged, use soft reset:
git reset --soft <commit-id>
- If you want to keep changes but unstage them, go for mixed:
git reset --mixed <commit-id>
- If you're looking to completely discard your recent changes, use hard reset:
git reset --hard <commit-id>
Troubleshooting Common Issues
One common issue, especially after a soft or mixed reset, is merging conflicts. It's important to review your changes carefully before merging. If you do mistakenly lose changes after a hard reset, you might still have a chance to recover them using the `git reflog` command, which shows a history of your HEAD's movements, potentially allowing you to find lost commits.
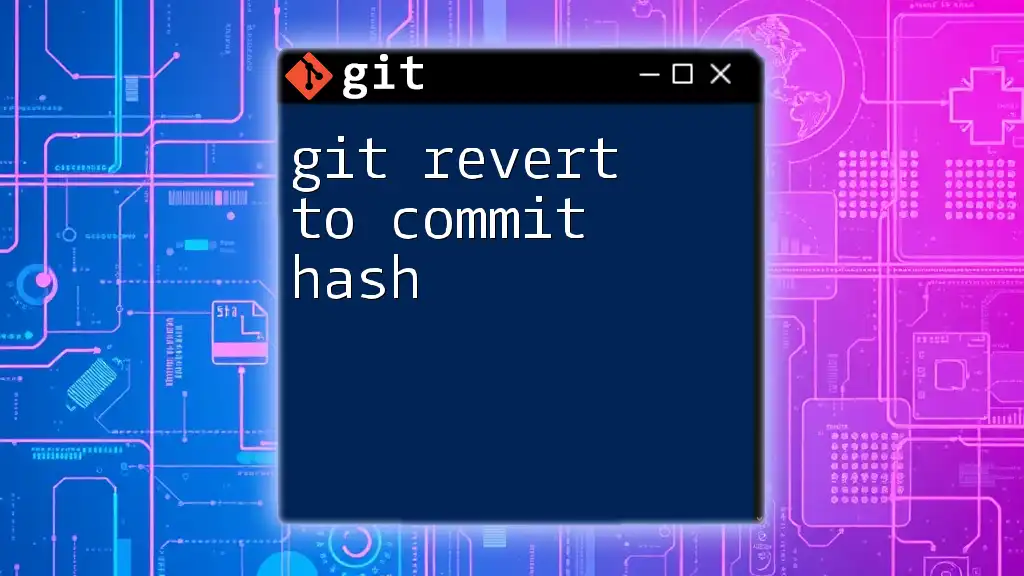
Best Practices for Using Git Reset
While `git reset` can be extremely useful, its power also implies potential pitfalls.
-
Use git reset cautiously: Avoid hard resets on shared branches, as this can lead to confusion among collaborators.
-
Consider using git revert: If you want to undo commits safely on a shared branch, consider using `git revert`, which creates a new commit that reverses the specified changes.
-
Commit Often with Descriptive Messages: Frequent commits with clear messages make it easier to identify commits for resetting.
-
Backup Branches: Always create a backup branch before using dangerous commands that could lead to data loss. You can easily branch off before resetting:
git checkout -b backup-branch
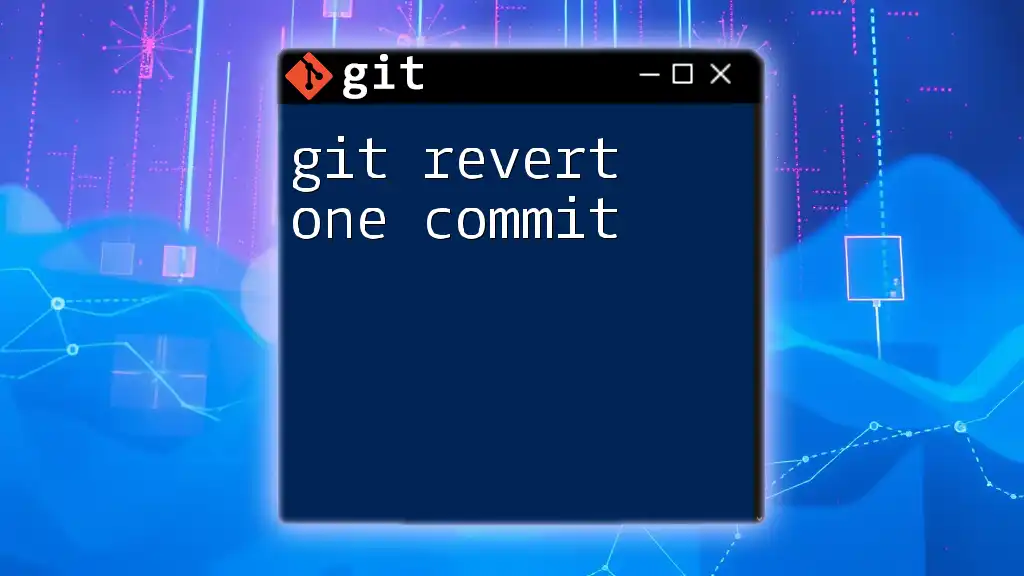
Conclusion
Understanding how to use `git reset to a commit` is essential for effective version control in any development workflow. This powerful command allows you to retrace your steps and clean up your changes, making it a valuable tool to have in your Git arsenal. Practice these commands in your repositories, and you will be better equipped to handle the complexities of version control with Git.