The `git remote set-url` command is used to change the URL of an existing remote repository in your local Git configuration.
git remote set-url <remote-name> <new-url>
Understanding Git Remotes
What is a Remote?
In Git, a remote refers to a version of your repository that is hosted on the Internet or another network. Remotes enable you to collaborate with others, share your work, and backup your local repositories. Essentially, they allow multiple users to work together on a project without conflicts.
Common Use Cases
The use of remotes is critical in several scenarios:
- Collaborating with Teams: When working with a team, you can push your changes to a shared remote repository, allowing teammates to pull updates and contribute.
- Backing Up Local Repositories: Storing your Git repositories on a remote server helps ensure that your work isn't lost, providing an additional layer of security.
- Managing Multiple Versions of a Project: Remotes let you manage different versions or branches of your projects. For instance, you can have upstream repositories from which you pull changes and keep your own fork.
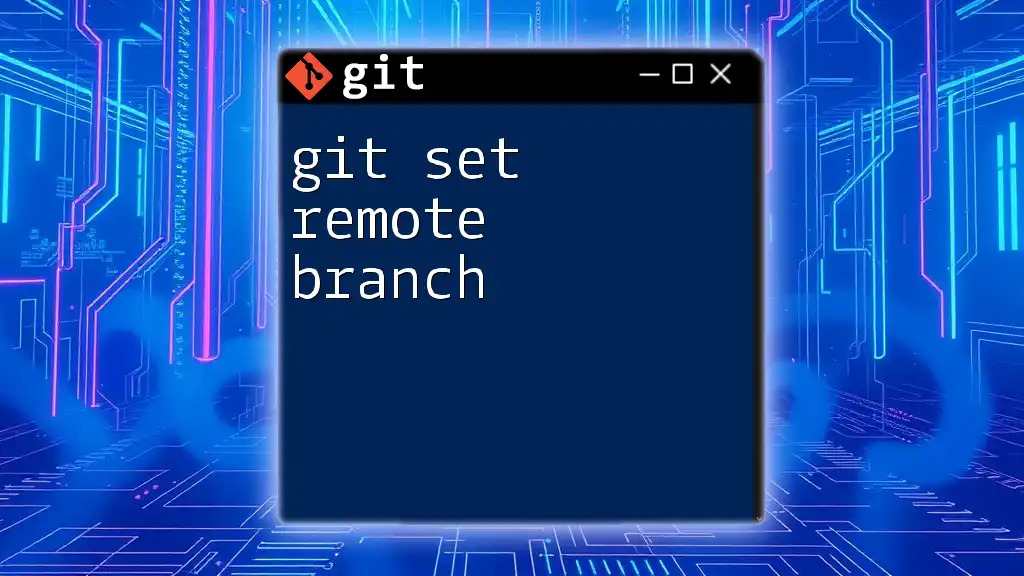
Setting Up a Remote Repository
Creating a Remote Repository
To get started with remotes, you first need a remote repository. Most popular platforms like GitHub, GitLab, and Bitbucket provide easy interfaces for repository creation.
When creating a repository:
- Navigate to your preferred platform.
- Create a new repository, but do not initialize it with a README file unless you want to merge it later.
- After creating the repository, you will usually see a URL that points to your new remote repo.
Adding a Remote
Once your remote repository is set up, you can link your local repository to it using the `git remote add` command. This command allows your local Git environment to communicate with the remote.
The syntax for this command is:
git remote add [name] [url]
For example, if your remote repository URL is `https://github.com/username/repo.git`, you would use:
git remote add origin https://github.com/username/repo.git
Here, `origin` is the default name assigned to your main remote repository. It is a convention in Git, but you can name it whatever you prefer.
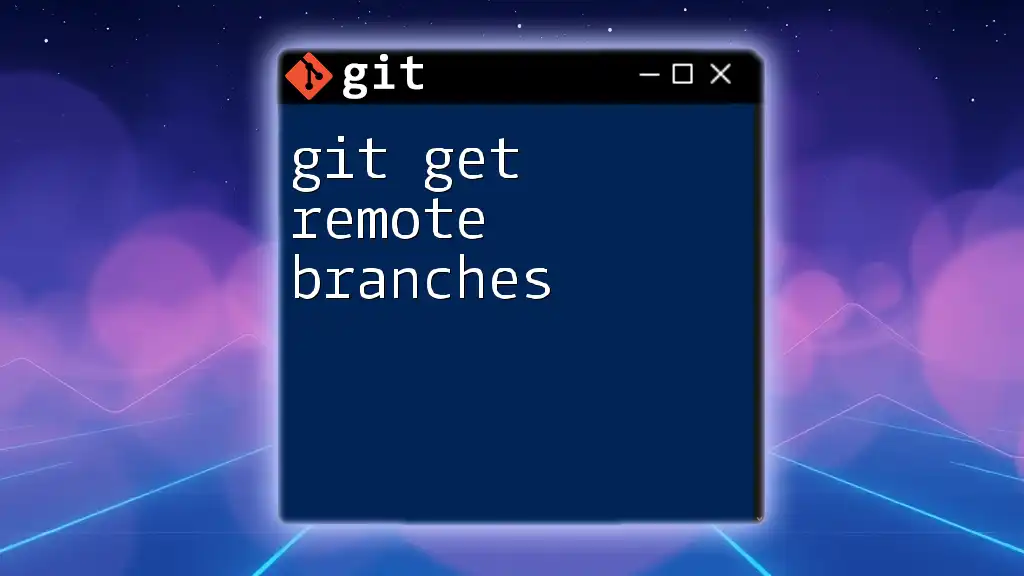
Checking Remote Configuration
Viewing Remotes
To view your configured remotes, you can execute the command `git remote -v`. This command is useful for confirming that your remote is set up correctly. The output will list the remotes for both fetching and pushing.
Example:
git remote -v
You might see an output similar to:
origin https://github.com/username/repo.git (fetch)
origin https://github.com/username/repo.git (push)
This indicates that your remote named origin is linked to the specified URL for both fetching (downloading changes) and pushing (uploading changes).
Inspecting Remote Settings
For a more detailed view of a specific remote's configuration, you can run:
git remote show [name]
For example:
git remote show origin
This will display a summary that includes the remote URL and the branches tracked. Each field in the output provides comprehensible insights into the remote’s setup, making it easier to understand how your local branches relate to the remote branches.
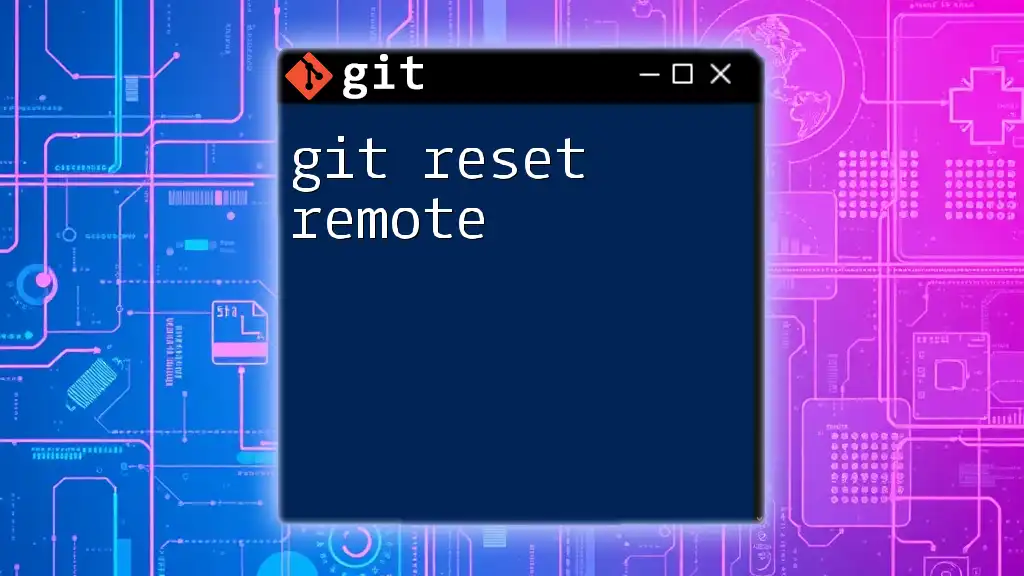
Modifying Remote Configurations
Changing a Remote URL
As the needs of your project evolve, you may need to change the URL associated with a remote repository. This can be easily done with the `git remote set-url` command.
The syntax is as follows:
git remote set-url [name] [newurl]
If you need to change the URL of your remote named origin to a new repository, you'd do:
git remote set-url origin https://github.com/username/new-repo.git
This command updates the URL associated with origin to the new repository, allowing you to push and pull from a different location.
Removing a Remote
If you decide that a remote is no longer needed or relevant, you can remove it using the `git remote remove` command. The syntax is straightforward:
git remote remove [name]
For example, to remove the origin remote, you would execute:
git remote remove origin
This command cleans up your configuration, ensuring you don’t have unnecessary remotes cluttering your setup.
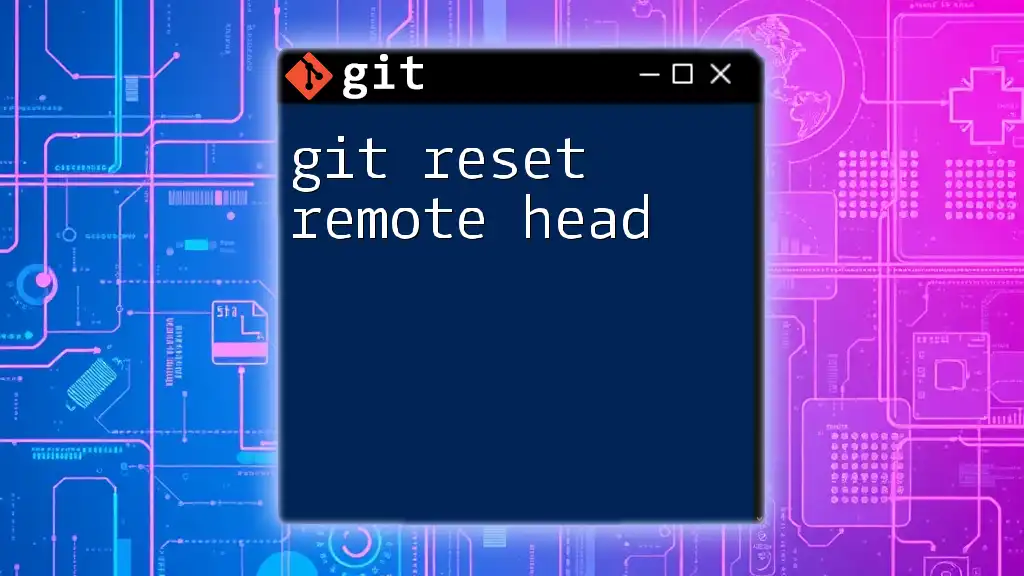
Best Practices for Managing Remotes
Naming Conventions
When adding remotes, it's essential to follow best practices in naming. While origin is the conventional default name for a primary remote, consider using descriptive names for additional remotes. For instance, if you fork a repository and have a remote for the original project, you might name it upstream. This practice enhances clarity, especially in collaborative environments.
Staying Organized
For projects involving multiple contributors or collaborators, managing multiple remotes becomes crucial. It's common to fork repositories, collaborate, and fetch changes from both the original project and your forked project. Being organized with your remote names will help avoid confusion and ensure smoother workflows.
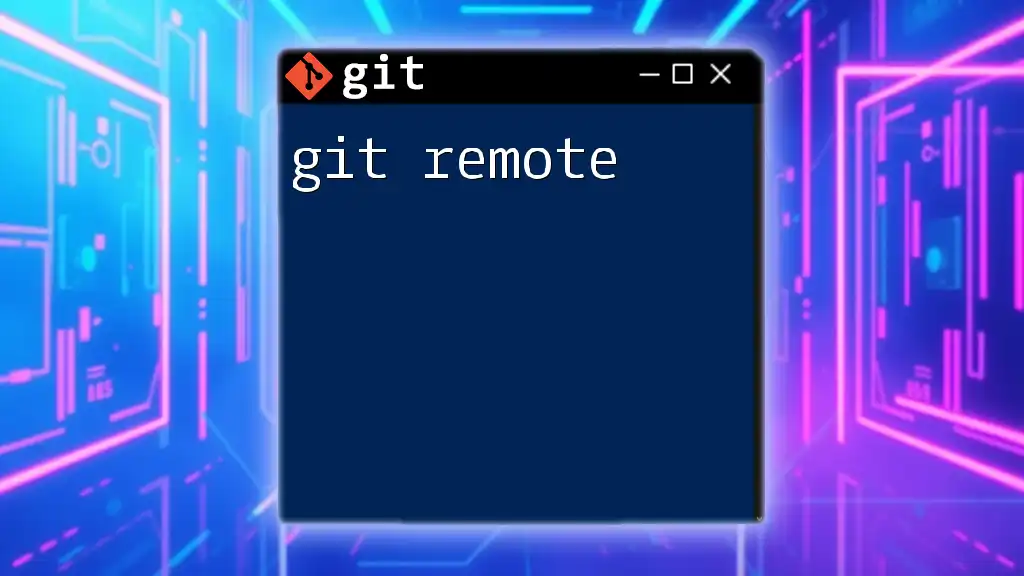
Common Issues and Troubleshooting
Remote Connection Failures
When working with remotes, you may encounter connection issues. Common reasons include:
- Network Problems: Ensure your internet connection is stable.
- Authentication Failures: Check your SSH keys or access tokens if using HTTPS.
- Repository URL Errors: Verify that the URL is correct and accessible.
If you encounter errors when pushing or pulling, carefully reviewing your remote configuration using `git remote -v` can clarify if the URLs are correct.
Cloning vs. Adding Remotes
It's crucial to differentiate between cloning a repository and adding a remote. Cloning is the process of creating a local copy of an entire repository, including its remotes. You usually do this when starting a new project collaboration. On the other hand, adding a remote is used to link your existing local repository to a new remote location.
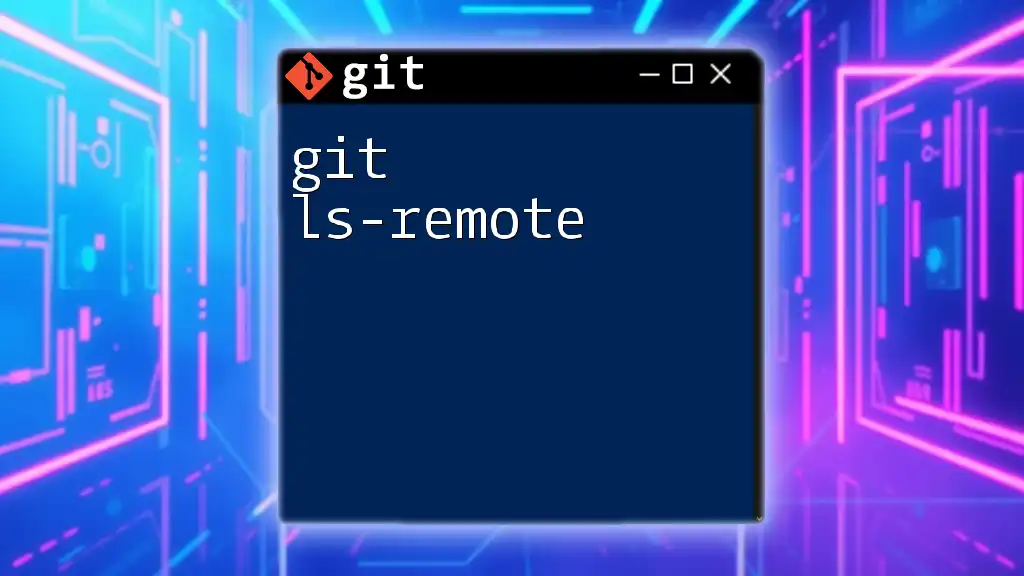
Conclusion
Setting up and managing remotes in Git is a fundamental skill for anyone working on collaborative projects. By understanding how to use commands like `git remote add`, `git remote set-url`, and `git remote remove`, you can navigate your version control with confidence.
Remember to practice these commands and use them in real projects to become proficient. Explore further into Git's documentation for a more in-depth understanding of all available commands and features. By mastering remotes, you are well on your way to leveraging Git for effective collaboration and version control in your development projects.
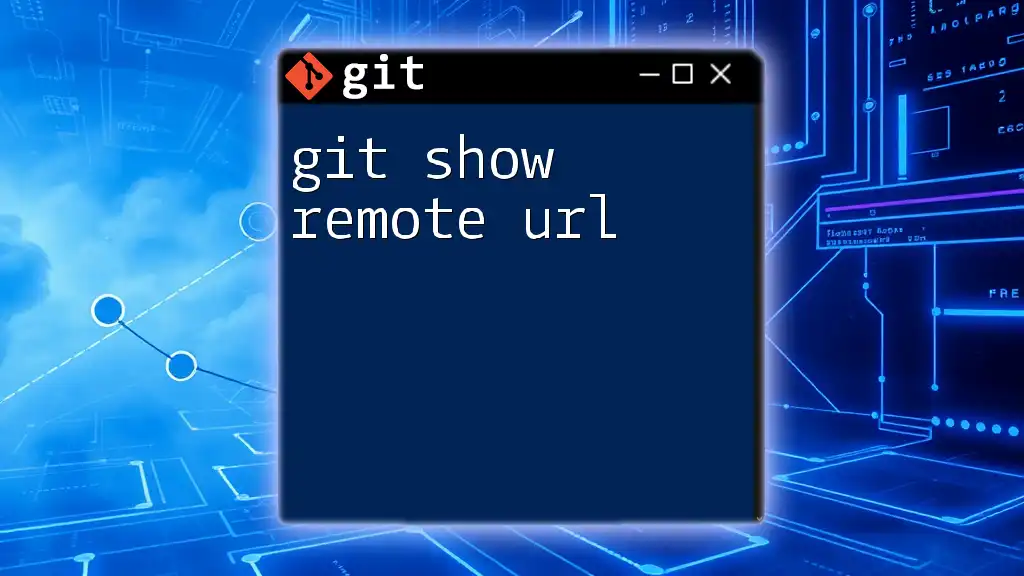
Additional Resources
For more extensive learning, you can refer to official Git documentation and various online tutorials that focus on advanced Git commands and best practices. Engaging with a community or forums can also provide support and insight as you continue to improve your Git skills.