The `git ls-remote` command is used to list references in a remote repository along with their corresponding commit IDs, allowing you to see what branches and tags are available without cloning the entire repository.
git ls-remote https://github.com/user/repo.git
What is `git ls-remote`?
`git ls-remote` is a Git command that allows you to query and list references (like branches and tags) in a remote repository without needing to clone the entire repository. This flexibility makes it particularly valuable for inspecting the state of remote repositories, especially when you want to check for updates, new branches, or tags without actually downloading files.
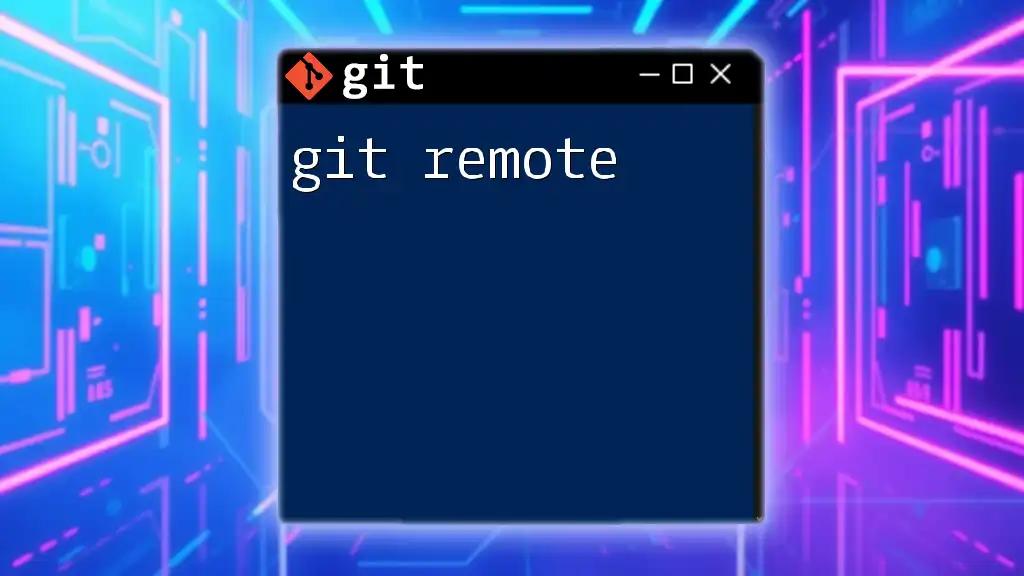
Why is `git ls-remote` important?
Using `git ls-remote` is essential in several scenarios:
- Collaboration: It helps you verify what branches and tags are available in a shared repository, ensuring your team is aligned.
- Workflow: By quickly accessing reference information, you can make informed decisions about your next steps in the development process.
- Automation: This command is often used in scripts to automate tasks related to Git repository management.
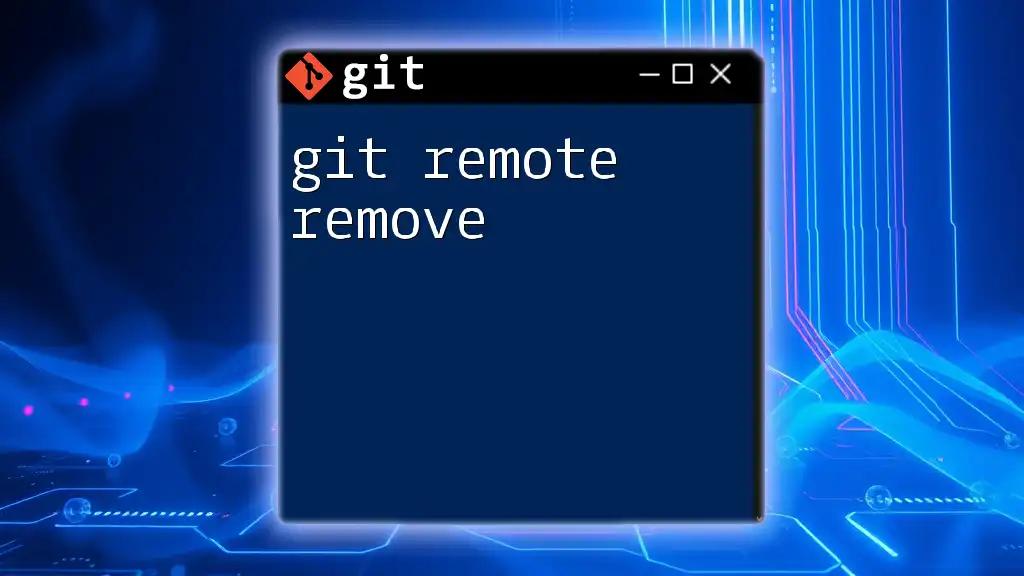
Understanding Git Repositories
What are Git Repositories?
Git repositories are storage spaces that hold all code associated with a project, including its history, branches, and tags. There are two types of repositories:
- Local Repositories: These reside on your local machine and contain the full data of the project.
- Remote Repositories: Hosted on a server, these repositories allow collaboration among multiple developers.
Importance of Remote Repositories
Remote repositories are essential for centralized collaboration as they provide a common platform for teams to access project history, share updates, and contribute to the project simultaneously. When working with remote repositories, understanding their structure and content is crucial, which is where `git ls-remote` comes into play.
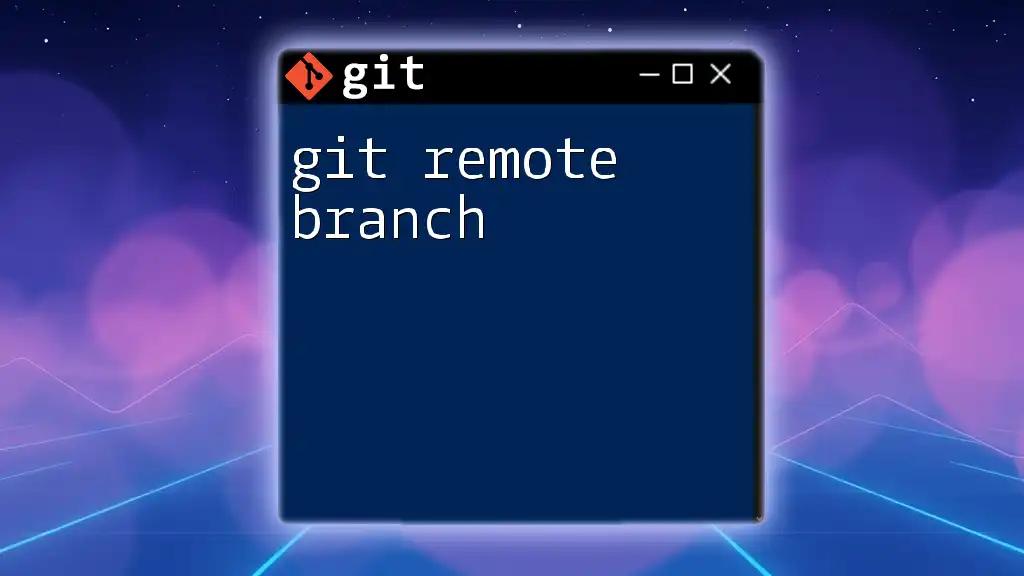
Overview of `git ls-remote`
Basic Syntax
The basic format for using `git ls-remote` is simple. You just need to specify the remote repository:
git ls-remote <repository>
For example:
git ls-remote https://github.com/user/repo.git
Common Options for `git ls-remote`
Using optional parameters can help refine the information you retrieve.
-
`-h` or `--heads`: This option allows you to list only the heads (branches) in the remote repository.
- Example:
git ls-remote -h https://github.com/user/repo.git
-
`-t` or `--tags`: Use this option to display only the tags.
- Example:
git ls-remote -t https://github.com/user/repo.git
Understanding the Output
The output of `git ls-remote` consists of pairs of SHA-1 hashes and references. Each reference represents either a branch or a tag.
- SHA-1 hash: A unique identifier for a specific commit in the repository.
- Reference: Indicates whether the SHA-1 is connected to a branch or tag.
For instance:
d9134b80008d9b70fea55031080d895a54fbd448 refs/heads/main
a4cdec196e80f5f1c3f10e5dba7d63baebfc3151 refs/tags/v1.0
In this example, we see two references: the main branch and a version tag v1.0.
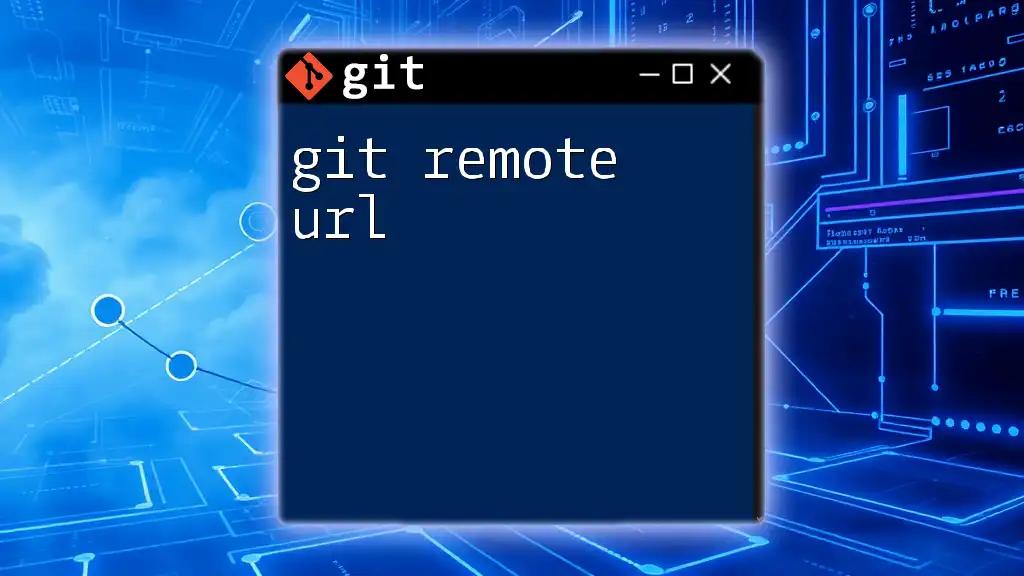
Practical Applications of `git ls-remote`
Checking Available Branches
To see all branches available in a remote repository, you can run:
git ls-remote -h https://github.com/user/repo.git
This command will list all branches, helping you verify which branches exist without cloning the repository.
Retrieving Tags
Tags are often used in version control to mark specific points in history as significant. To retrieve tag information, you would use:
git ls-remote -t https://github.com/user/repo.git
This command will provide a list of all the tags, assisting in version management and deployment processes.
Verifying Remote Connections
One common use case of `git ls-remote` is ensuring that your connection to a remote repository is functional. When this command runs successfully, it provides confirmation that you have access to the specified repository.
Running:
git ls-remote https://github.com/user/repo.git
Without any errors indicates that your setup is correct and the repository is reachable.
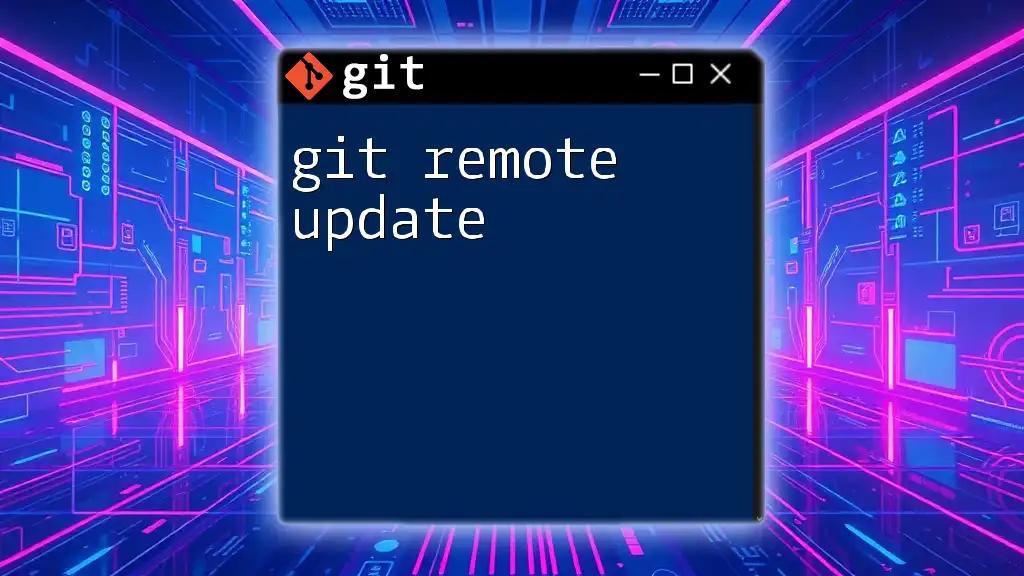
Working with `git ls-remote` in Scripts
Automation with Shell Scripts
The capabilities of `git ls-remote` lend themselves well to automation in shell scripts. For example, you might want to check for new tags added to a remote repository regularly. Here is a simple script illustrating how to do this:
#!/bin/bash
REPO_URL="https://github.com/user/repo.git"
git ls-remote -t $REPO_URL | awk '{print $2}' | grep -E '^refs/tags/' | cut -d'/' -f3
This script retrieves and lists all tag names from the specified remote repository.
Integrating with Continuous Integration/Continuous Deployment (CI/CD)
In CI/CD workflows, `git ls-remote` is integral for fetching updates and ensuring that the build process incorporates the latest changes. By checking remote branches or tags, the pipeline can deploy or build only when necessary, saving resources and time.
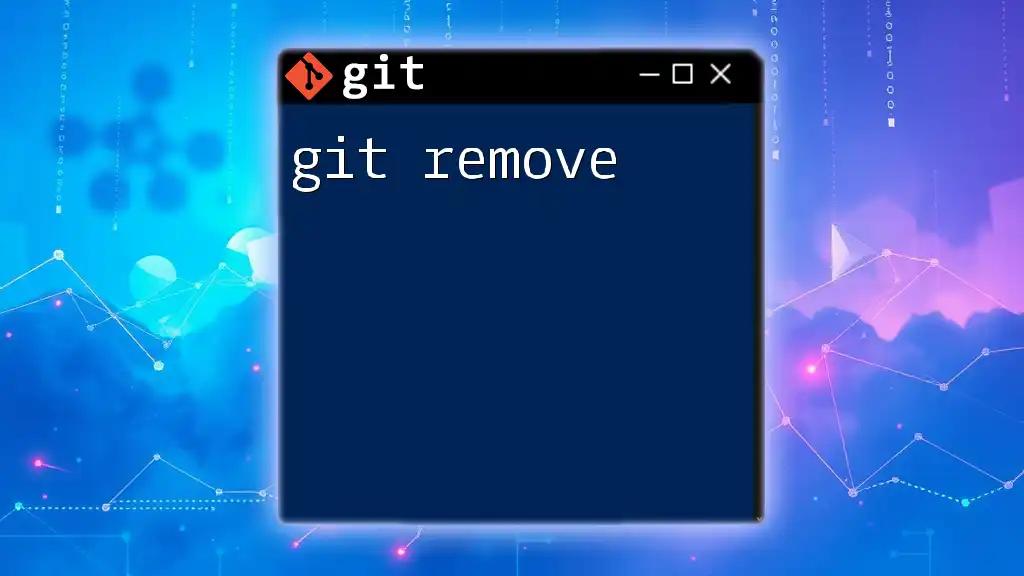
Troubleshooting Common Issues
Unable to Access Remote Repository
If you encounter issues accessing a remote repository, consider the following potential solutions:
- Check the URL: Ensure that the repository URL is correct.
- Permissions: Verify that you have proper permissions to access the repository.
Understanding Authentication Errors
Authentication problems may arise, especially when using private repositories. If you see an error related to authentication, check your credentials, SSH keys, or access tokens to ensure they are valid and configured correctly.
Interpreting Unexpected Output
Sometimes the output might be confusing, especially if there are no references listed. If you run:
git ls-remote https://github.com/user/repo.git
and receive no output, this may indicate that there are no branches or tags published in the repository, or that you are referencing it incorrectly.
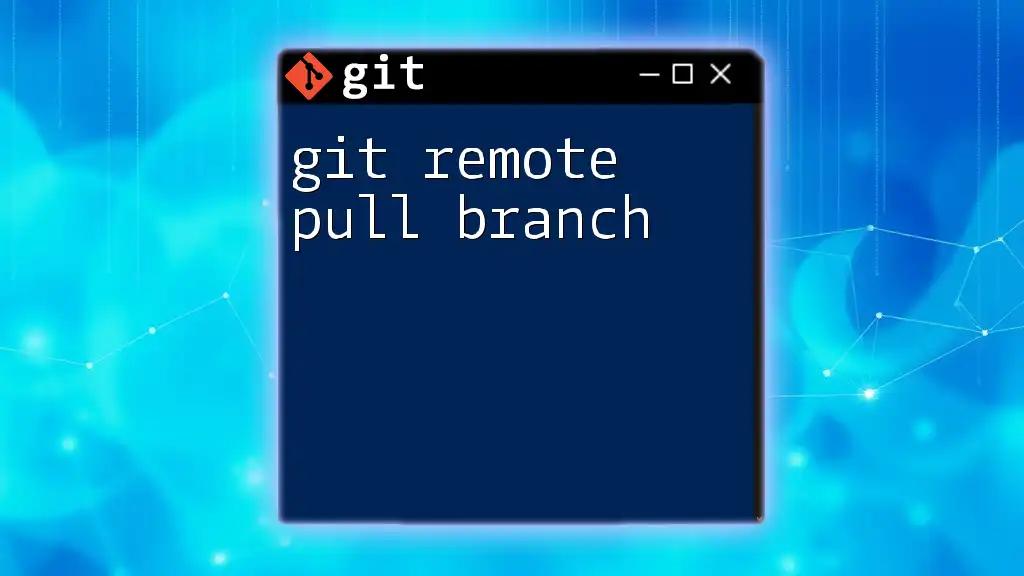
Best Practices for Using `git ls-remote`
Regular Monitoring of Remote Branches and Tags
Make it a habit to periodically run `git ls-remote` commands, especially before significant changes. This vigilant practice can help you avoid conflicts and stay informed about updates from team members.
Combining with Other Git Commands
For a holistic approach to working with Git, combine `git ls-remote` with other commands like `git fetch` and `git pull`. While `git fetch` updates your local repository with changes from a remote, `git ls-remote` provides context about what is available to fetch, making your operations more efficient.
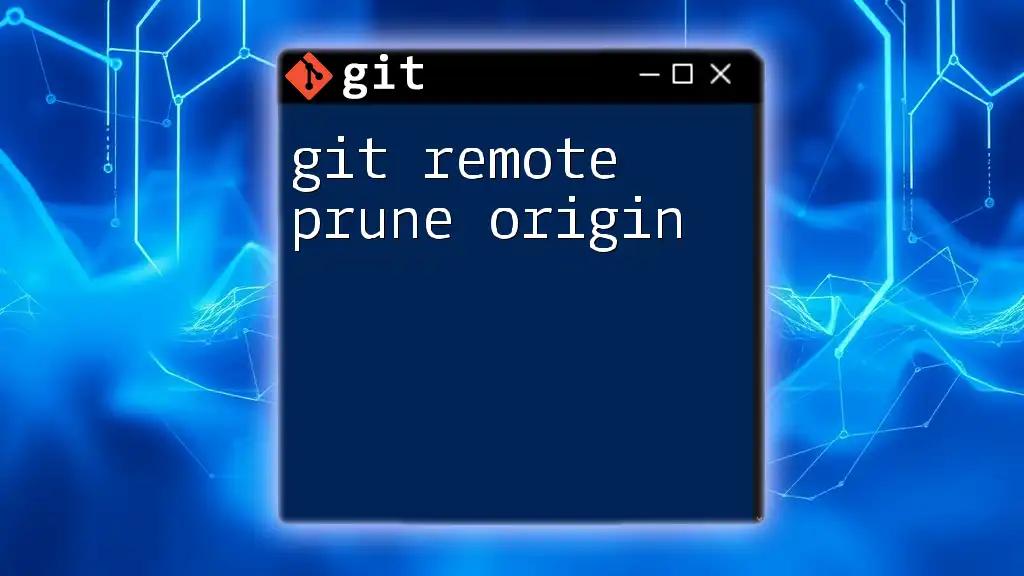
Conclusion
In summary, `git ls-remote` is a powerful tool that provides critical information about remote repositories, aiding in collaboration and version control management. By familiarizing yourself with its capabilities and integrating it into your workflow, you can enhance your efficiency and remain aligned with project changes. Practice using `git ls-remote` and experiment with its options to fully appreciate its usefulness in your development processes.
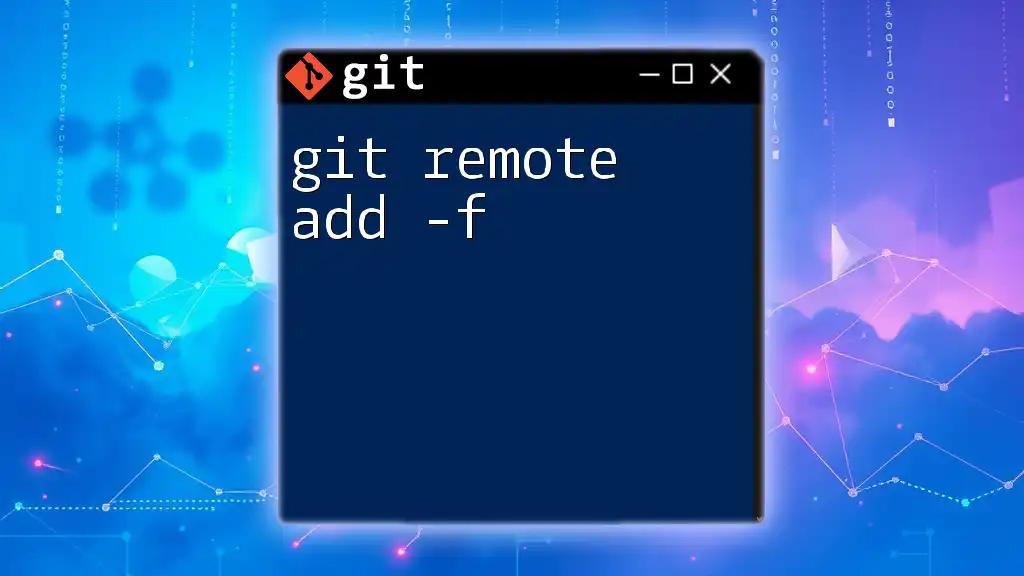
Further Reading
For more in-depth knowledge, consider exploring the official Git documentation, which provides comprehensive information about `git ls-remote` and other commands. Additionally, familiarizing yourself with related git commands, such as `git fetch`, `git pull`, and `git remote`, can further strengthen your understanding of Git as a whole.