The command `git rm` is used to remove files from the staging area and the working directory, while `git add` adds changes in your working directory to the staging area for the next commit.
Here's the code snippet in markdown format:
# Remove a file and stage the removal for the next commit
git rm filename.txt
# Add changes from a file to the staging area
git add filename.txt
Understanding Git Commands
What is a Git Command?
A Git command is a fundamental part of using Git, a version control system that helps manage changes in code. The basic structure of a command consists of the command itself followed by its various options and arguments. Understanding these commands is crucial for effective version control, allowing developers to streamline the process of tracking changes, handling multiple project versions, and collaborating with teams.
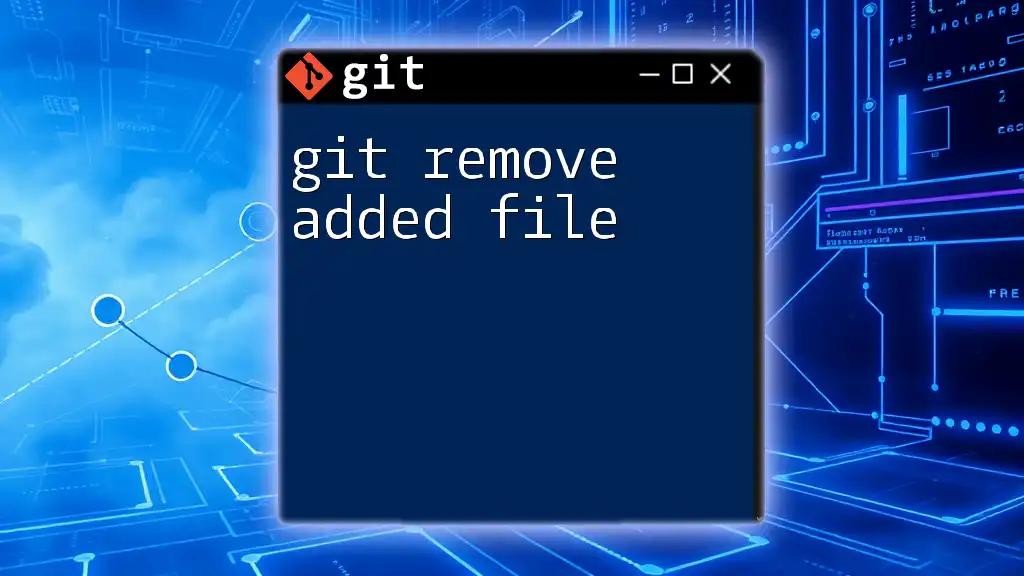
The `git add` Command
Overview of `git add`
The `git add` command plays a pivotal role in the Git workflow. It stages changes in your working directory, preparing them for the next commit. Without executing `git add`, none of your modifications will be saved in your version history. This command serves as a bridge between modifying files in your workspace and committing those changes to your repository.
How to Use `git add`
The basic syntax for the `git add` command is as follows:
git add <file_path>
Replace `<file_path>` with the actual file you want to add.
Examples of `git add`
Adding a Single File
To add a single file, the command would look like this:
git add example.txt
When you run this command, Git stages `example.txt`. If you check the status using `git status`, you will see that `example.txt` is now in the staging area, ready to be committed.
Adding Multiple Files
If you want to add multiple files simultaneously, you can do this:
git add file1.txt file2.txt
This command stages both `file1.txt` and `file2.txt`. It's an efficient way to handle multiple additions at once, but always double-check that the files you are adding are the ones you intend to track.
Adding All Changes
To stage all modified files in your working directory, you can use:
git add .
Using the dot (`.`) as an argument tells Git to include everything in the current directory. However, be cautious, as this will stage all changes, including possibly unintentional modifications. It's a good idea to run `git status` before executing this command to avoid surprises.
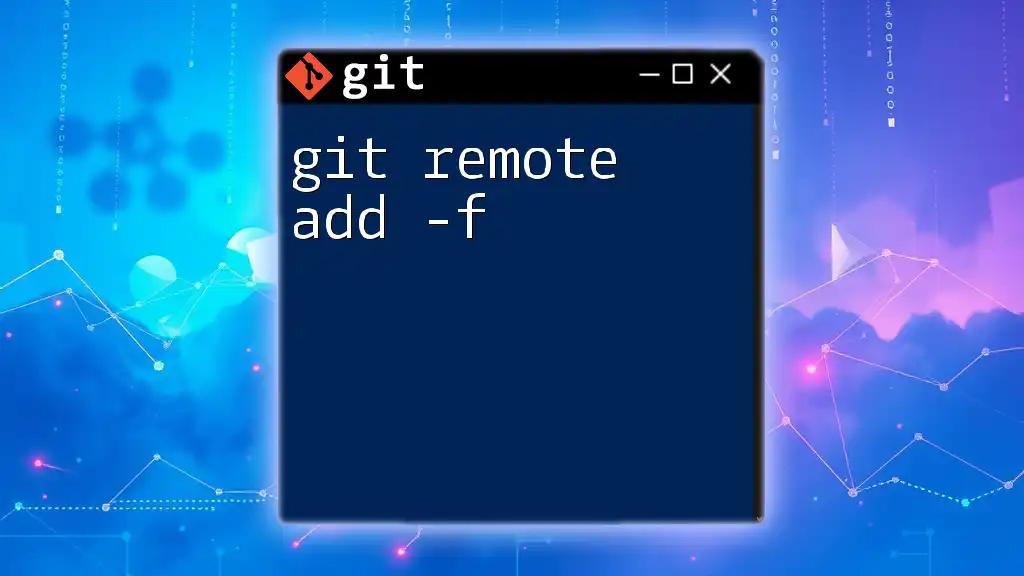
The `git remove` Command
Overview of `git remove`
It's common to confuse `git remove` with the `rm` command found in Unix-like systems. In Git, the correct command to use is usually `git rm`. This command is designed to remove files not just from your working directory but also from the staging area entirely.
How to Use `git remove`
The basic syntax for the command is as follows:
git rm <file_path>
Examples of `git remove`
Removing a Single File
To remove a file from your Git repository, you’d use:
git rm example.txt
In this case, `example.txt` is removed from both the working directory and the staging area. If you check the status now, you'll notice that Git recognizes the file as deleted, ready to be committed.
Removing Multiple Files
Just like adding files, you can also remove multiple files at once:
git rm file1.txt file2.txt
This removes `file1.txt` and `file2.txt` both from the working directory and the index. Exercise caution here, as this can lead to losing vital files if not double-checked.
Removing Files from the Index Only
Suppose you want to keep the file in your working directory but remove its staged status. In that case, you can use the following command:
git rm --cached example.txt
This command un-stages `example.txt`, but the file will still exist in your working directory. It’s particularly useful if you’ve added a file that you later decide doesn't need to be tracked by Git, like logs or compiled binaries.
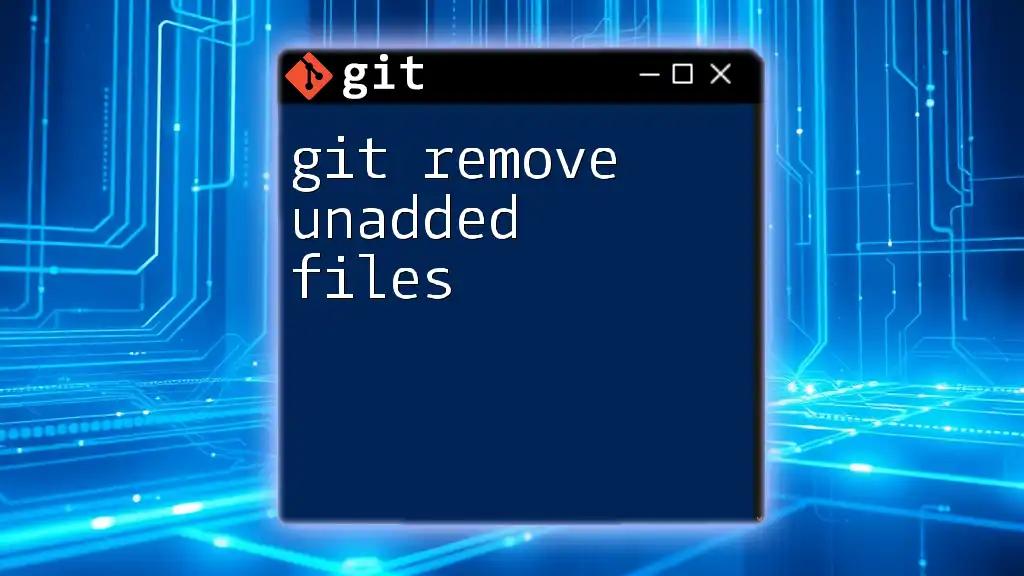
The Relationship Between `git add` and `git remove`
Importance of Understanding Both Commands
Mastering `git add` and `git remove` is essential for managing your version control effectively. Understanding when to use `add` versus `remove` allows you to maintain a clear and organized project history.
Typical Use Cases
When developing software, you might find yourself frequently adding new features or files that need tracking. Conversely, as your project evolves, you may identify legacy files that no longer serve a purpose. This dual capability of adding and removing keeps your repository clean and manageable.

Common Mistakes and Best Practices
Common Errors with `git add` and `git remove`
One common pitfall is forgetting to commit your changes after adding or removing files. If you skip that step, your changes will remain in the staging area, and your local repository won't reflect these updates.
Best Practices
Staging changes carefully is a best practice that cannot be overstated. Use `git status` regularly to keep track of what has been staged or removed. This habit can save you from accidental commits of incomplete work or unwanted files.
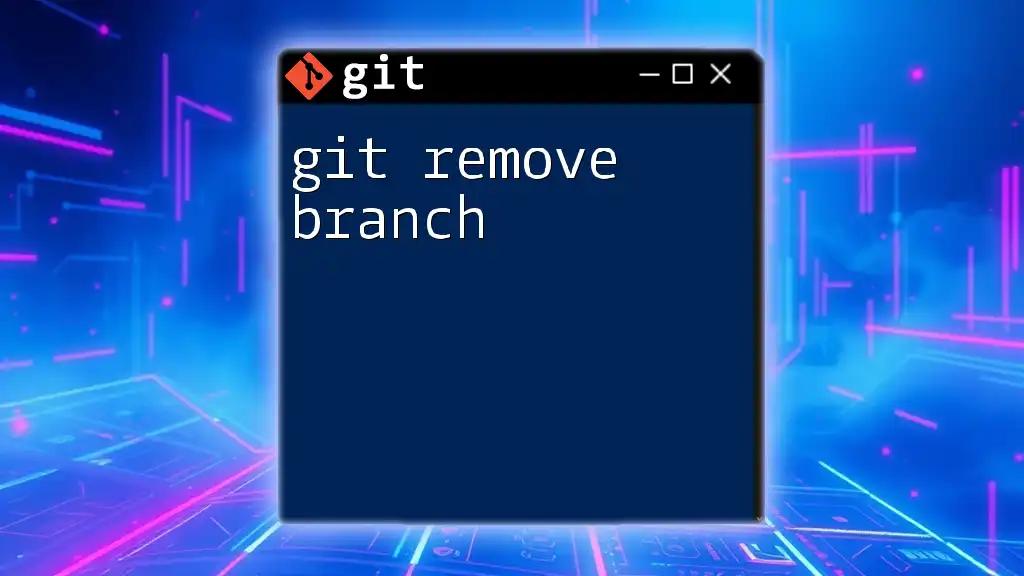
Troubleshooting
Common Issues and Solutions
One frequent issue in the usage of `git remove` is accidentally deleting files you didn’t want to remove. If you find yourself in such a situation, you can often restore deleted files if they haven't yet been committed.
To do so, you may utilize:
git checkout HEAD -- example.txt
This command retrieves the last committed version of `example.txt`, restoring it to your working directory.
Adding Unwanted Files
Should you accidentally stage files you don’t wish to commit, unstage them using:
git reset HEAD <file_path>
This command removes the specified file from the staging area without removing it from your working directory.
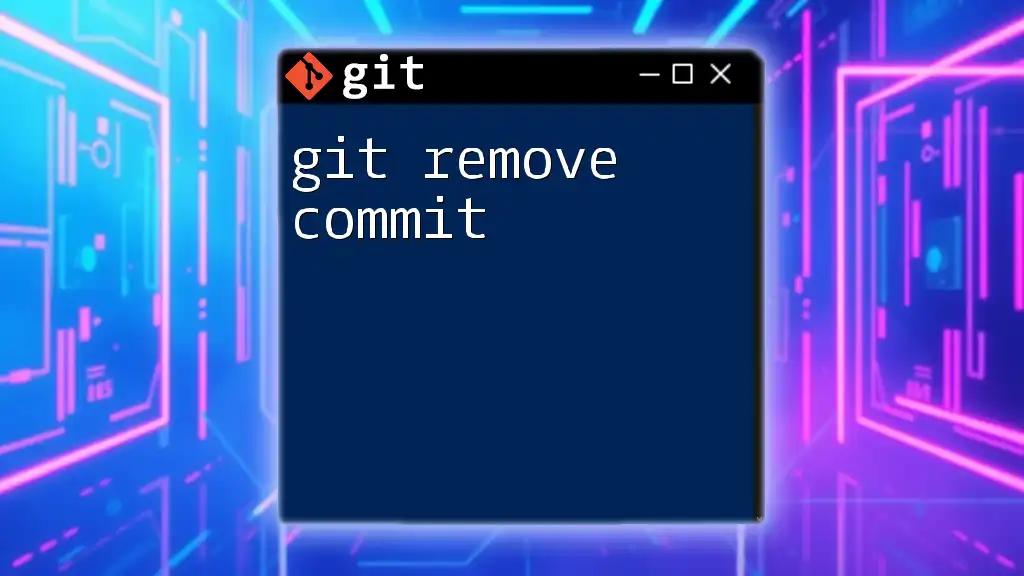
Conclusion
In closing, both `git add` and `git remove` are fundamental commands that every Git user must master. The efficient application of these commands is critical for maintaining a clean and manageable project repository. Practicing these commands in a dedicated test repository will help reinforce your understanding, making Git an invaluable tool in your development toolkit.
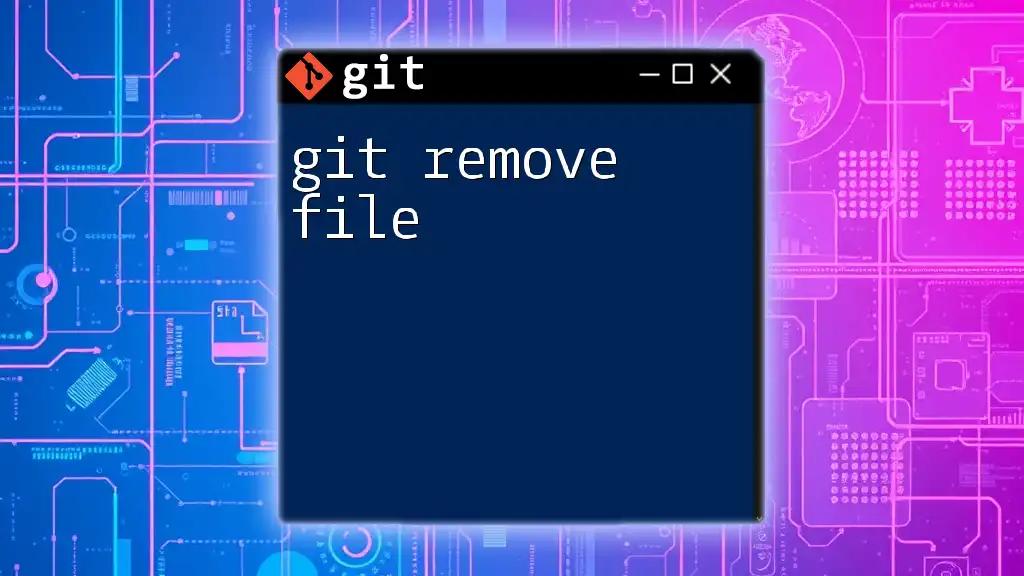
Additional Resources
To further enhance your understanding, consider exploring the official Git documentation. Additionally, platforms such as Codecademy and freeCodeCamp offer extensive tutorials and courses that can deepen your knowledge of Git commands.