To remove a tag in Git, you can use the following command which deletes the specified tag from your local repository:
git tag -d <tagname>
Understanding Git Tags
What is a Git Tag?
A Git tag is a reference to a specific commit in your repository's history. It is often used to mark particular points in development, such as releases or milestones. Tags are beneficial because they provide easy access and identification to important versions of your code.
There are two main types of tags in Git: lightweight and annotated.
-
Lightweight Tags are essentially pointers to a specific commit, similar to bookmarks. They don’t store any extra information about the project or the author.
-
Annotated Tags are more like full objects in the Git database. They contain additional information, such as the tagger’s name, email, date, and even a message. Annotated tags are typically used for releases since they offer more context.
Why Would You Want to Remove a Tag?
There are various situations where you may wish to remove a tag:
- Incorrect Tagging: You accidentally created a tag with the wrong name or on the wrong commit.
- Duplicate Tags: Two similar tags might have been created unintentionally.
- Releases or Versions that Need Updates: A tag that references a version that has bugs might need to be removed to avoid confusion.
Removing a tag does not just delete a reference; it can also impact the clarity of your project’s history and the collaboration within your team. Therefore, understanding how to do it properly is essential.
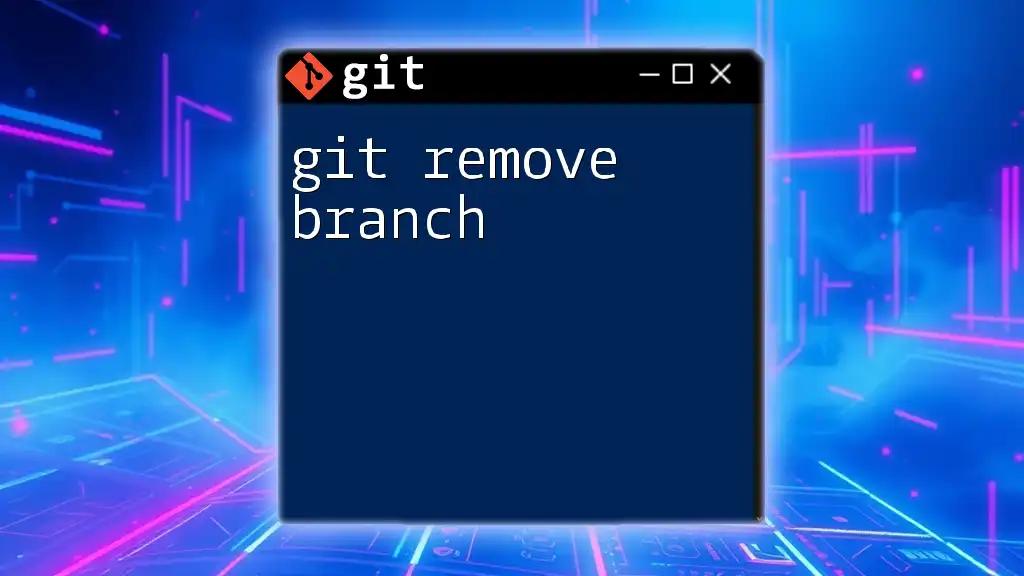
How to Remove a Git Tag Locally
Checking Existing Tags
Before you remove a tag, it's essential to know which tags exist in your local repository. You can easily do this with the command:
git tag
This command will list all the tags currently in your repository, allowing you to identify the tag you want to remove.
Removing a Local Tag
To remove a tag locally, you will use the `git tag -d` command. The syntax is straightforward:
git tag -d <tagname>
For example, if you want to remove a tag named `v1.0`, you would execute:
git tag -d v1.0
After executing the command, you will see an output confirming the deletion, such as:
Deleted tag 'v1.0' (was a1b2c3d).
Confirming Removal of Local Tags
To ensure that the tag has been removed, you can recheck the existing tags with:
git tag
You should no longer see `v1.0` in the list, confirming that the tag has been successfully deleted.
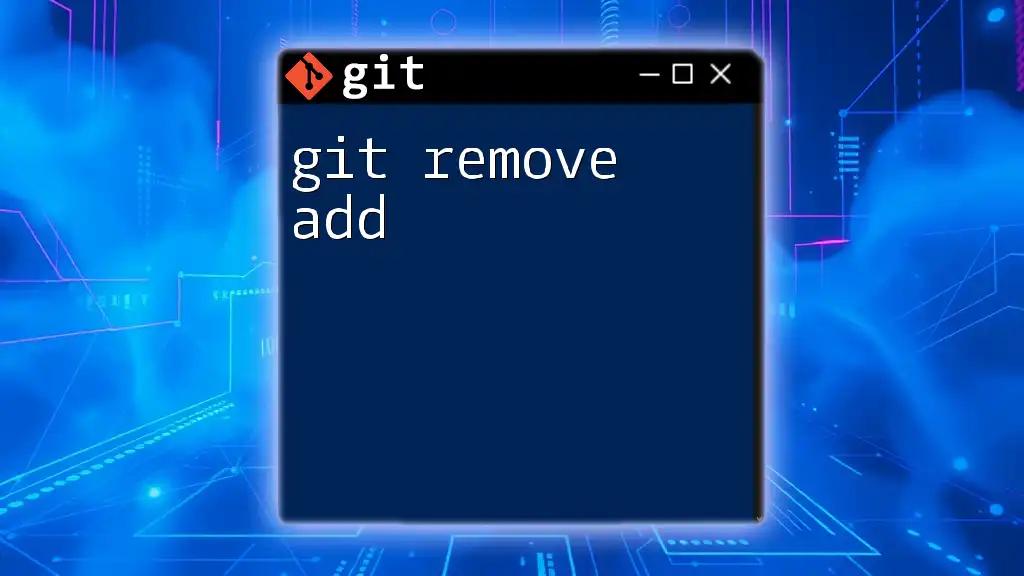
How to Remove a Git Tag from a Remote Repository
Understanding Remote Tags
Tags function similarly in remote repositories. When you push tags to a remote, they become part of that repository's history as well. Therefore, it’s crucial to synchronize your changes between local and remote repositories.
Deleting a Remote Tag
To remove a tag from a remote repository, you will need to use the following command:
git push --delete <remote> <tagname>
For instance, to remove the `v1.0` tag from the `origin` remote, you would execute:
git push --delete origin v1.0
This command communicates to Git that you want to delete the specified tag from the `origin` remote repository.
Confirming Removal of Remote Tags
After you've executed the command to delete the remote tag, you can confirm that the tag has been removed by executing:
git ls-remote --tags <remote>
In this case, it would look like:
git ls-remote --tags origin
This command will list all the tags available on the remote `origin`, and you should see that `v1.0` is no longer listed.
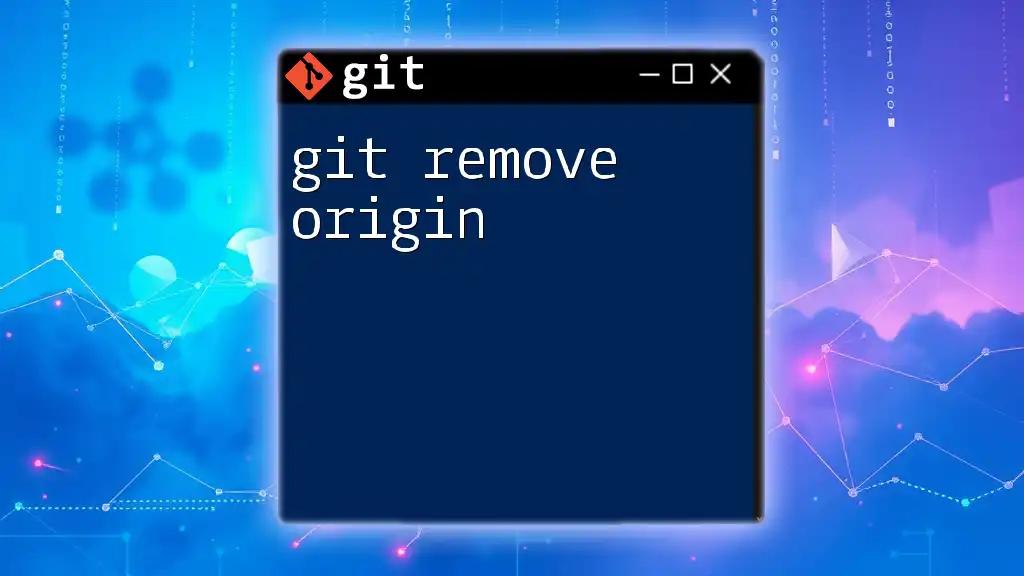
Verifying Tag Deletion
Checking Tags Locally
Recheck your local tags with:
git tag
Make sure to verify that `v1.0` is absent from your local list of tags, confirming local deletion.
Checking Remote Tags
To synchronize and ensure that your remote tags reflect the most recent state, you can run the following commands:
git fetch --tags
git tag
This will update your local tags and allow you to confirm once more that the tag `v1.0` is no longer part of your references.

Additional Considerations
Permanence of Tag Removal
It's crucial to note that once a tag is deleted, it cannot be recovered unless another reference exists. Tags are simply pointers to specific commits, so if you’ve missed a tag that references an important version of your code, it may present challenges in the future. Always double-check before removing a tag to avoid losing valuable references.
Best Practices for Managing Tags
To ensure that your tagging strategy is efficient and error-free, consider the following best practices:
-
Consistent Naming Conventions: Adopt a clear, consistent naming strategy for your tags to reduce the possibility of duplicates or naming errors.
-
Versioning Strategy: Use semantic versioning (such as v1.0.0) along with descriptive tags (like `release-1.0`) to provide clarity on the purpose of each tag.
-
Collaborative Tagging: If you work in a team, communicate changes or deletions to prevent others from facing issues when navigating through tags.
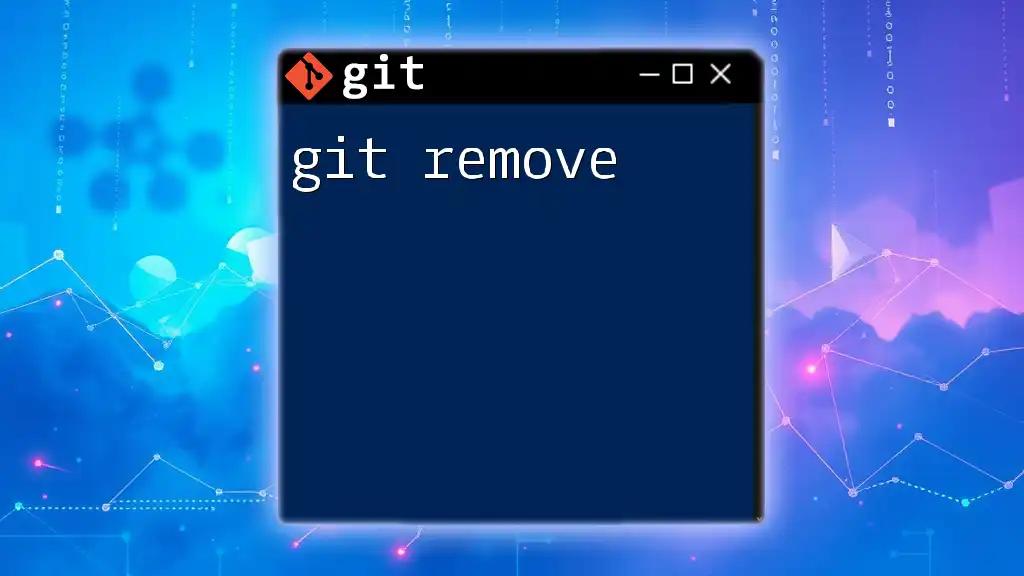
Conclusion
In summary, managing tags in Git is an essential aspect of maintaining clear and effective version control. Knowing how to git remove a tag both locally and remotely offers greater control over your project's history and ensures a smoother development process. By practicing these techniques regularly, you safeguard your project against confusion or downtime caused by incorrect tags.
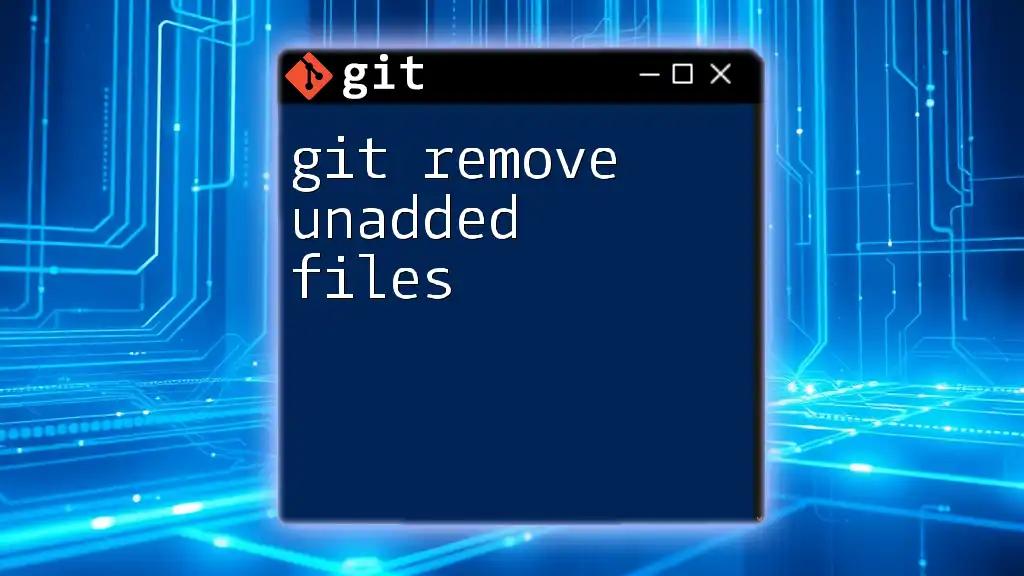
Call to Action
To enhance your Git skills further, subscribe for more tips and tricks related to Git commands. Additionally, explore other resources available that can help you master version control and improve your workflow.