The `git remote url` command allows you to view and configure the remote repository URL associated with your local Git repository.
You can use the following command to see the current remote URL:
git remote -v
To set or change the remote URL, use:
git remote set-url origin <new-url>
Understanding Git Remote
What is a Remote Repository?
A remote repository is simply a version of your project that is hosted on an external server, often in a cloud environment. This enables multiple developers to collaborate on the same project from various locations. Remote repositories serve as centralized storage for your code, making it easier to share, backup, and manage your work.
In contrast, a local repository exists only on your personal machine. While you can make changes and commit them locally, the power of Git comes into play when you synchronize these changes with a remote repository.
Types of Remotes
In Git, remotes can have various names, with origin being the most common. This name refers to your default remote repository, usually the one from which you cloned your project. In larger projects, you might encounter additional remotes, such as upstream. This is typically used in forking scenarios, allowing you to pull in changes from the original repository while still contributing your own modifications.
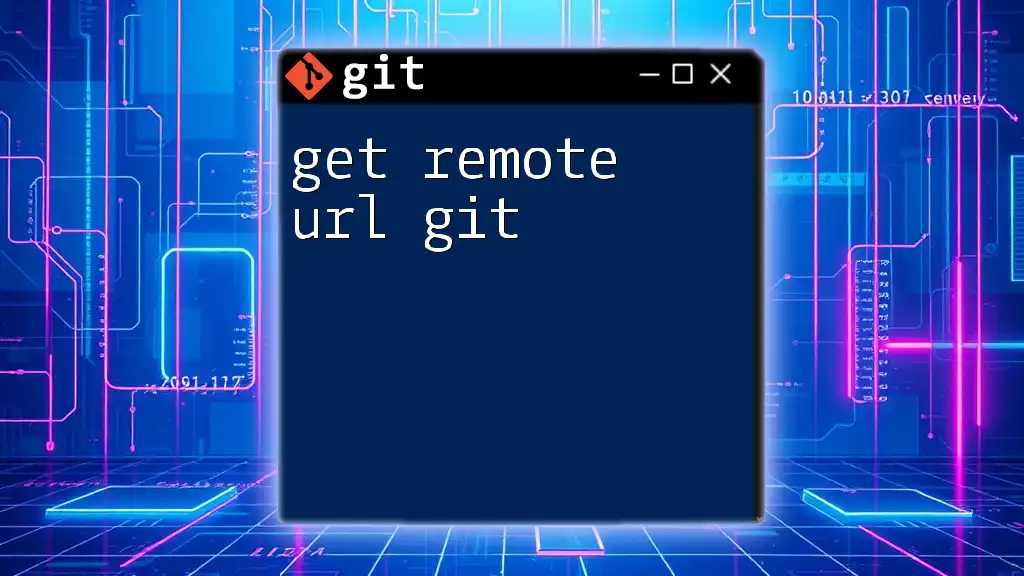
Setting Up Remote URLs
Initializing a Git Repository
Before you can work with remote URLs, you'll need to have a Git repository set up. You can initialize a new repository with the following command:
git init
This command creates a new, empty Git repository in your current directory. If you want to link your local repository to a remote one, you'll need to provide the remote's URL.
Adding a Remote URL
Once your repository is initialized, you can add a remote URL using the following syntax:
git remote add <remote-name> <remote-url>
For example, if you're setting the primary remote to be hosted on GitHub, you would use:
git remote add origin https://github.com/user/repo.git
Here, origin is the name you're giving the remote, while the URL points to your GitHub repository. This command establishes a connection between your local repository and the remote one.
Verifying Your Remote URLs
To check your current remote URLs, you can use the command:
git remote -v
This will display a list of all configured remotes, showing you both the fetch and push URLs. Understanding this output is crucial because it helps confirm that your configuration is set up correctly and that Git knows where to send your changes.
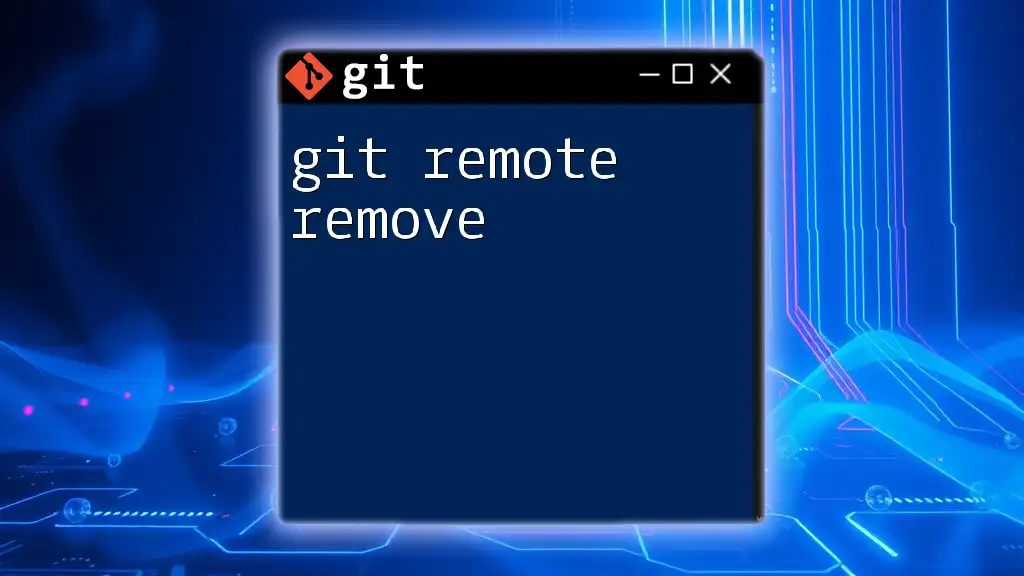
Managing Remote URLs
Changing a Remote URL
If you need to change the URL of an existing remote (perhaps the repository has moved), you can use the following syntax:
git remote set-url <remote-name> <new-remote-url>
For instance, if the repository URL has changed, you would execute:
git remote set-url origin https://github.com/user/new-repo.git
This updates the origin URL to the new address, ensuring your local repository points to the correct remote location.
Removing a Remote URL
In some cases, you may need to remove a remote reference completely. You can do this using the command:
git remote remove <remote-name>
For example, if you want to remove the origin remote, the command would be:
git remote remove origin
This effectively deletes the reference from your local Git configuration.
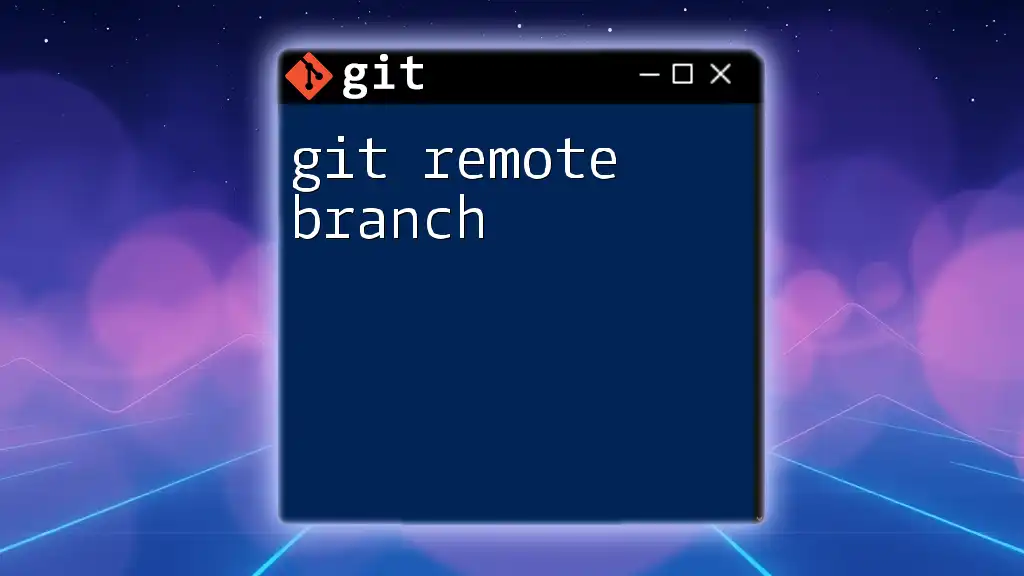
Common Use Cases
Cloning a Repository with a Remote URL
One of the most frequent tasks is cloning a repository. You can clone a remote repository directly to your local machine using:
git clone <remote-url>
For example:
git clone https://github.com/user/repo.git
This command downloads the entire repository from the specified URL, including all its history and branches, allowing you to start working on it immediately.
Collaborating with Multiple Remote Repositories
In larger projects or open-source contributions, you may need to interact with multiple remotes. This is especially common when you fork a repository or need to sync changes from an upstream source. To add an additional remote, simply run:
git remote add upstream https://github.com/another-user/repo.git
Now both origin (your forked repository) and upstream (the original repository) are accessible, facilitating easier collaboration.
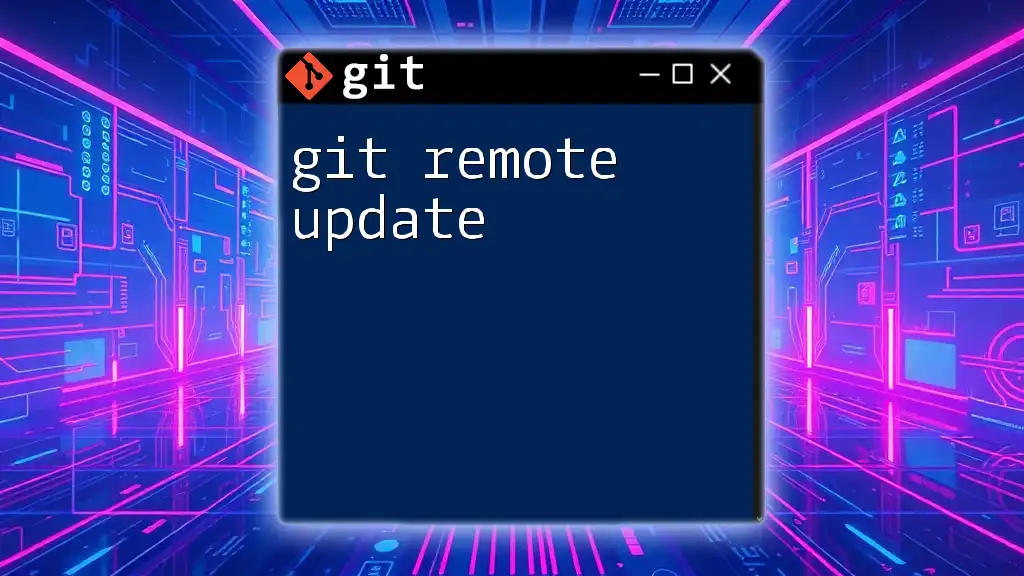
Troubleshooting Remote URL Issues
Common Errors and Solutions
Despite its power, working with remote URLs can lead to errors. One common mistake is encountering the message:
fatal: 'origin' does not appear to be a git repository.
This usually means that you haven't added a remote named origin. You can fix this by re-adding the remote URL with the correct command.
Another prevalent issue is:
Unable to access '<remote-url>'
If you see this error, it's often due to permission issues, such as lack of access rights to the repository. Make sure you have proper permissions or that you're authenticated correctly.
When to Use SSH vs. HTTPS
Choosing between SSH and HTTPS when dealing with remote URLs is crucial for security and ease of use. SSH (e.g., `git@github.com:user/repo.git`) requires setting up SSH keys, which can streamline authentication but does have a slightly steeper initial setup. On the other hand, HTTPS URLs (e.g., `https://github.com/user/repo.git`) are straightforward as they typically require a username and password for each push or pull, which can become cumbersome.
In summary, understanding when and how to use each method is essential for effective collaboration.
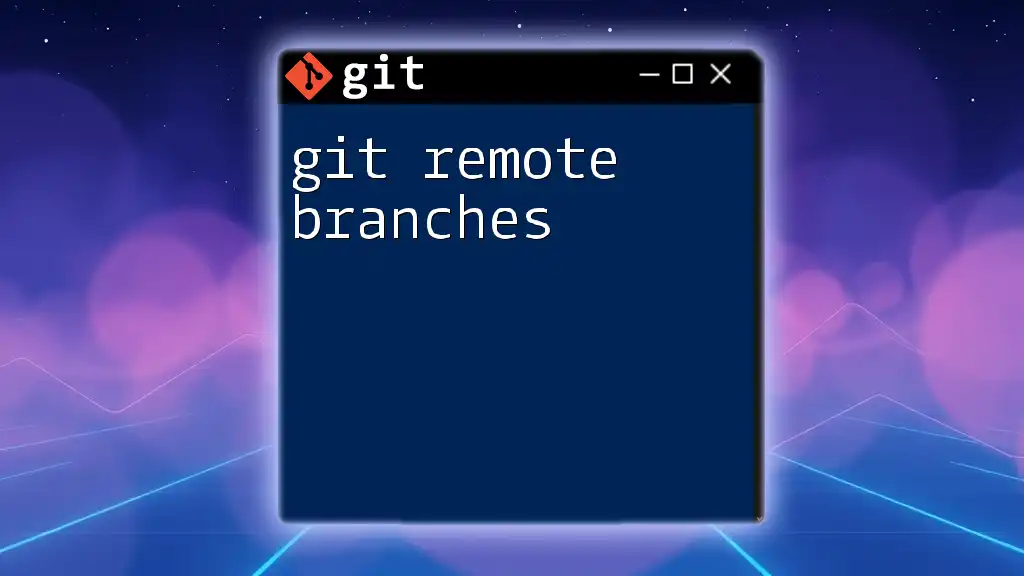
Conclusion
Understanding git remote url is vital for leveraging Git's collaboration features effectively. By mastering the commands for setting up, modifying, and troubleshooting remote URLs, you'll pave the way for smoother teamwork and project management in your development endeavors. Whether you're a beginner or looking to polish your skills, regular practice with these commands will enhance your workflow efficiency in collective environments.