The `git rebase -i HEAD` command initiates an interactive rebase, allowing you to modify, squash, or reorder your last commits in a clean and efficient way.
git rebase -i HEAD~n # Replace 'n' with the number of commits you want to rebase
Understanding Git Rebase
What is Git Rebase?
Git rebase is a powerful command used to integrate changes from one branch into another. Unlike merging, which creates a new commit that combines both histories, rebasing rewrites the commit history. This allows developers to maintain a cleaner, more linear project history.
Benefits of using rebase:
- Ensures a linear history which improves readability.
- Helps reduce the complexity of the commit graph.
- Allows for the ability to showcase a more cohesive change structure when reviewed by others.
When to Use Git Rebase
Rebase is particularly useful in situations where you want to integrate changes from a base branch (like `main` or `develop`) into your feature branch without creating a merge commit. This can be flattering for handling various changes and working in a team setting. For instance, if multiple developers are working on the same feature, rebasing helps keep the history tidy and minimizes conflicts during integration.
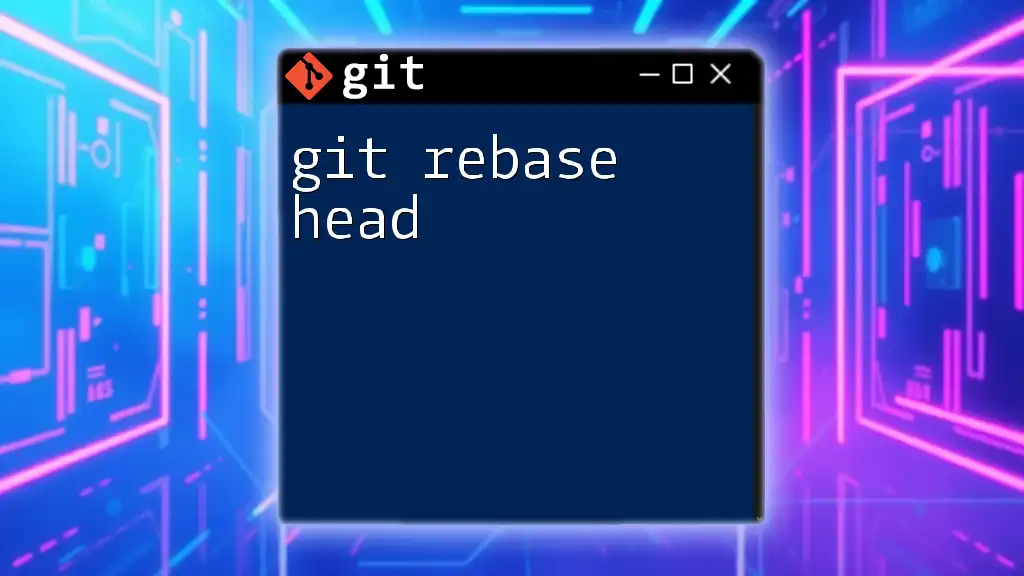
Introduction to Interactive Rebase
What is Interactive Rebase?
Interactive rebase is a more nuanced version of the standard rebase command, allowing developers to edit multiple commits in a single command. You can choose actions like renaming commits, reordering them, squashing them together, or dropping them entirely.
Git Rebase -i HEAD Explained
The command `git rebase -i HEAD` launches the interactive rebase interface at the point specified by HEAD. Here, HEAD refers to the latest commit on your current branch. In practice, when you use `git rebase -i HEAD~n`, you are specifying the last `n` commits to manipulate.
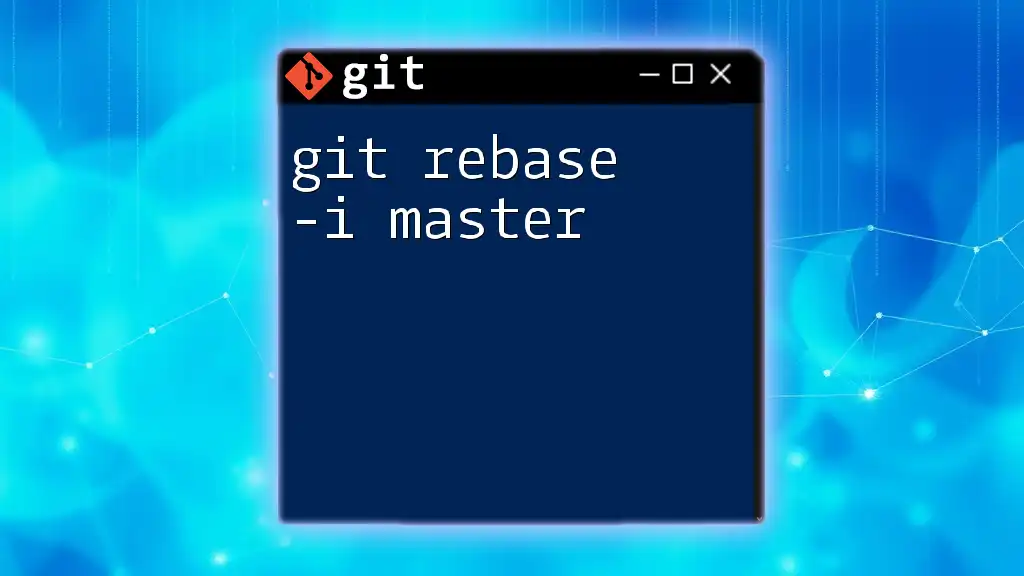
Setting Up for an Interactive Rebase
Preparing Your Branch
Before you begin, make sure you are on the correct branch where you want to perform the interactive rebase. You can ascertain your current branch using:
git branch
Viewing Commit History
Having a clear view of your commit history is essential. Use the following command to display the last few commits in a simple format:
git log --oneline
This will give you a snapshot of the commit history, which is crucial for determining which commits to modify during the rebase.
Starting the Interactive Rebase
To start the interactive rebase process, use the command that specifies how many commits back you want to manipulate. For example, if you want to interact with the last three commits, the command would be:
git rebase -i HEAD~3
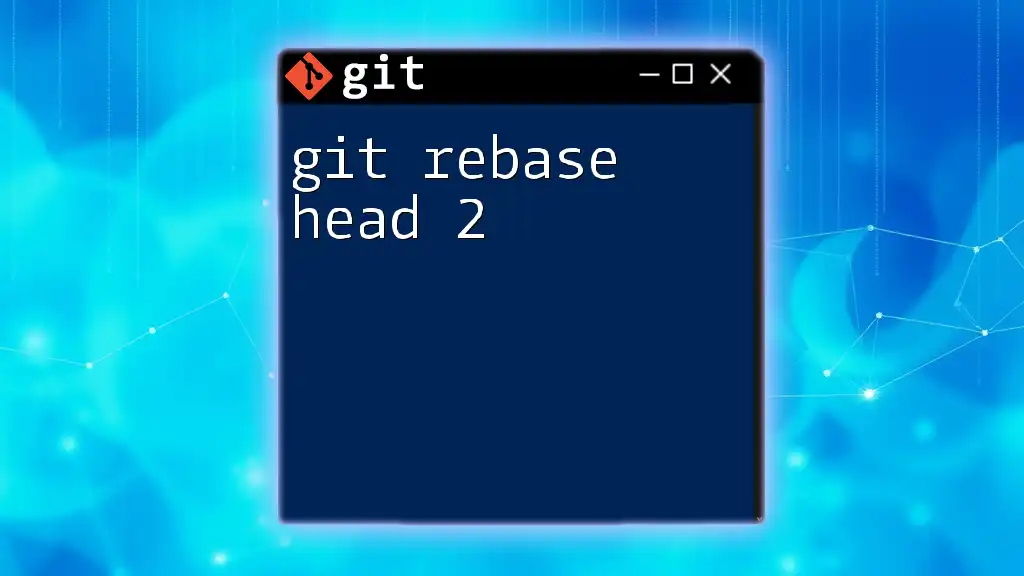
Interactive Rebase Workflow
The Rebase Interface
Upon executing the rebase command, Git will open your default text editor with a list of the last `n` commits and their associated actions. The default action for each commit will be `pick`, indicating that Git will include these commits in the final history.
Modifying Commits
Picking Commits
The `pick` command means you want to include that particular commit in the rebase process. If all you want is simply to retain the commits without changes, you can leave them as they are in the rebase interface.
Squashing Commits
One significant advantage of the interactive rebase is the ability to squash commits. Squashing allows you to combine multiple commit messages into one, which can help simplify the history. To squash a commit, change `pick` to `squash` (or simply `s`) for the commits you want to merge into the previous commit. This could look like:
pick 123abc Commit 1
squash 456def Commit 2
squash 789ghi Commit 3
In this example, Commit 2 and Commit 3 will be combined into Commit 1, creating a cleaner commit history.
Editing Commits
If you need to change the contents or messages of a previous commit, replace `pick` with `edit`. After saving and closing the editor, Git will pause the rebase after that commit allowing you to make changes. To amend the commit, use:
git commit --amend
After editing, to continue the rebase, execute:
git rebase --continue
Reordering Commits
Changing Commit Order
You can change the order of commits simply by rearranging the lines in the rebase interface. For instance, if you have:
pick 123abc Commit 1
pick 456def Commit 2
pick 789ghi Commit 3
And you want `Commit 3` to come first, adjust to:
pick 789ghi Commit 3
pick 123abc Commit 1
pick 456def Commit 2
Aborting and Continuing Rebase
Aborting a Rebase
Sometimes, you may encounter issues or decide not to proceed with the rebase. In such cases, aborting the rebase restores your branch to its original state. Use the following command:
git rebase --abort
Continuing a Rebase
If conflicts arise while rebasing, Git will pause for you to fix them. After resolving any conflicts, use:
git rebase --continue
This command helps you move forward with the rebase process.
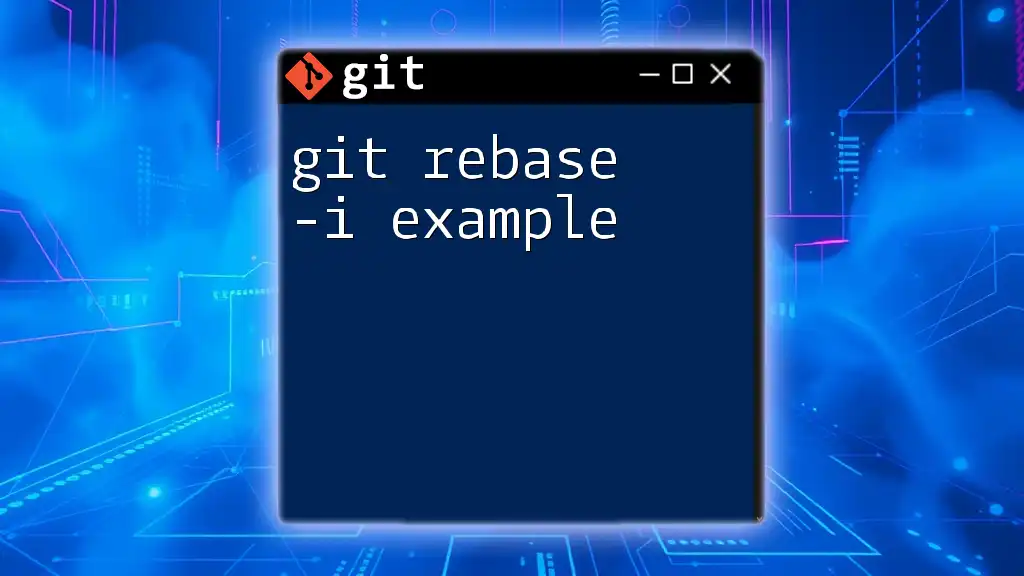
Handling Merge Conflicts
Identifying Merge Conflicts
While rebasing, you may encounter merge conflicts that need resolution. You can check which files are conflicting using:
git status
Resolving Conflicts
To resolve conflicts, open the files listed as having conflicts. Git marks the conflicting parts. Edit the file to remove conflicts, then save the changes. Follow this by staging the resolved files:
git add <filename>
Then continue the rebase.
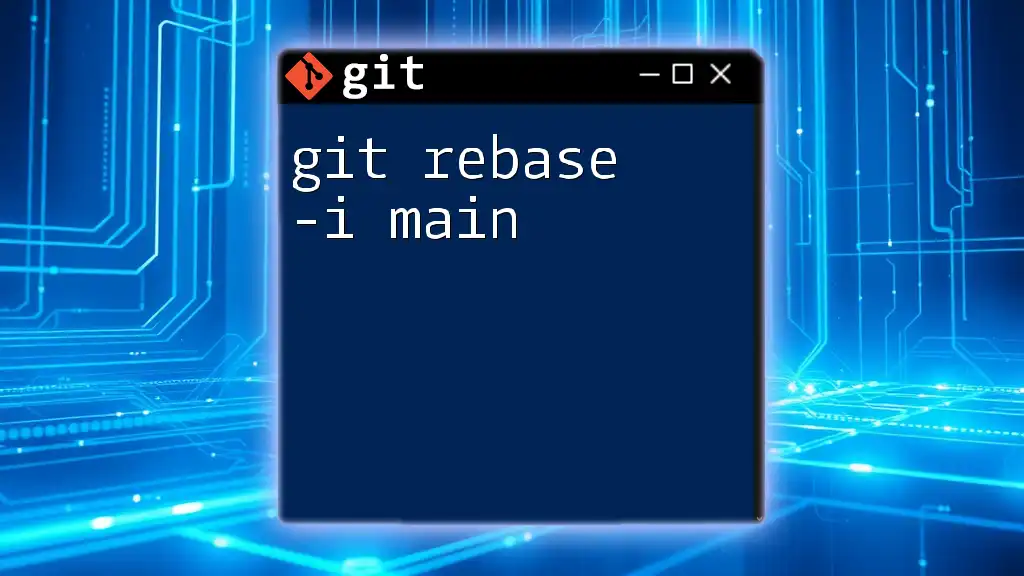
Finalizing the Rebase
Completing the Interactive Rebase
Once all conflicts are resolved and all modifications are complete, finish the interactive rebase process by running:
git rebase --continue
Confirm that your commit history looks clean and structured.
Verifying Your Changes
To verify the new state of your commit history post-rebase, use:
git log --oneline
This command will show you the updated linear history, confirming that the rebase has been successful.
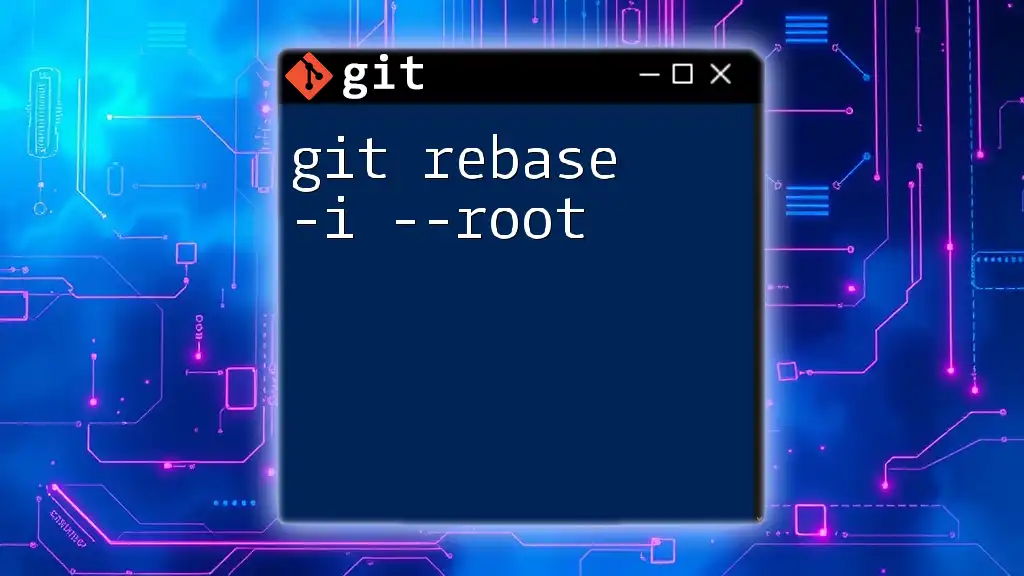
Conclusion
The `git rebase -i HEAD` command is a versatile tool that allows you to effectively manage your commit history. The ability to modify, squash, and reorder commits helps create a refined project history that can enhance collaboration and clarity within your development team. Practice utilizing this command, and consider integrating it into your daily Git workflow to improve your version control skills.
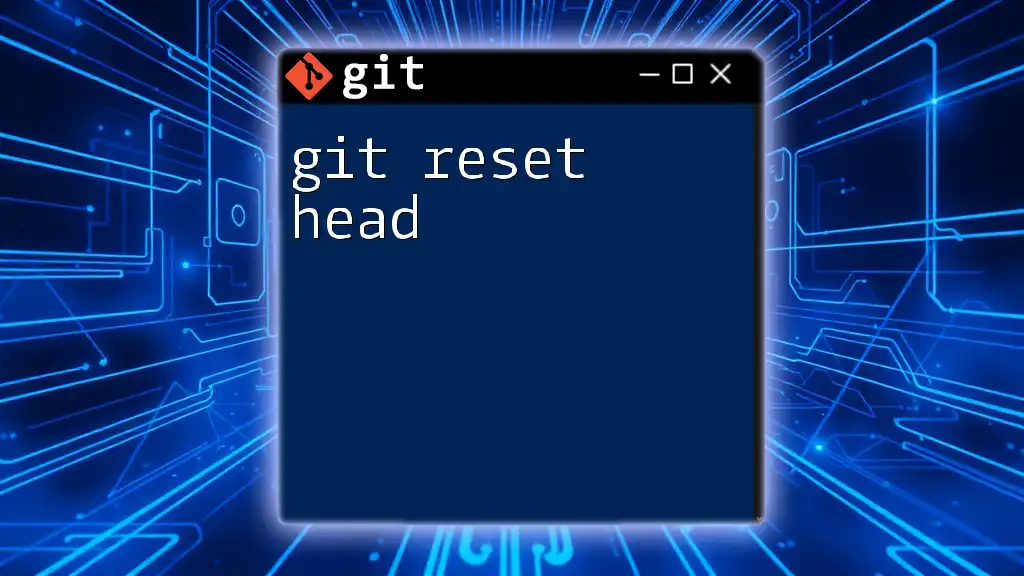
Additional Resources
For those eager to explore beyond this guide, consider checking out the official Git documentation for in-depth knowledge. Additional tutorials and courses are also available online to further enhance your proficiency with Git. Always remember to keep a checklist of Git commands related to rebasing handy for quick reference!