The command `git rebase -i main` initiates an interactive rebase of the current branch onto the latest commit of the `main` branch, allowing you to edit, squash, or reorder commits as needed.
git rebase -i main
Understanding Rebase
What is Git Rebase?
Git Rebase is a powerful command in version control systems that allows developers to integrate changes from one branch into another. The primary purpose of a rebase is to create a cleaner, more linear project history.
Unlike merging, which consolidates the changes from different branches but retains their independent histories, rebase rewrites history by applying changes from a feature branch directly onto the target branch. This makes it an invaluable tool, particularly when you want to maintain a tidy commit log.
Benefits of Using Git Rebase
There are several advantages to using git rebase -i main:
-
Cleaner Project History: By eliminating merge commits and applying changes directly on top of the target branch, your project's history remains straightforward and easy to follow.
-
Easier to Navigate with `git log`: A linear history simplifies the process of searching through past commits. You can go through commit messages without wading through complex branching structures.
-
Enhanced Collaboration: It helps in minimizing conflicts when multiple developers work on the same codebase. By rebasing regularly, you can ensure that your feature branch stays up-to-date with the latest changes.
Common Scenarios for Using Rebase
Rebasing is especially useful in scenarios like:
-
Keeping Feature Branches Up to Date: By regularly rebasing your own changes onto the main branch, you can incorporate the latest changes made by others.
-
Resolving Conflicts Among Multiple Branches: When two branches have divergent histories, rebasing helps in applying one branch's changes onto another, resolving conflicts in a structured way.
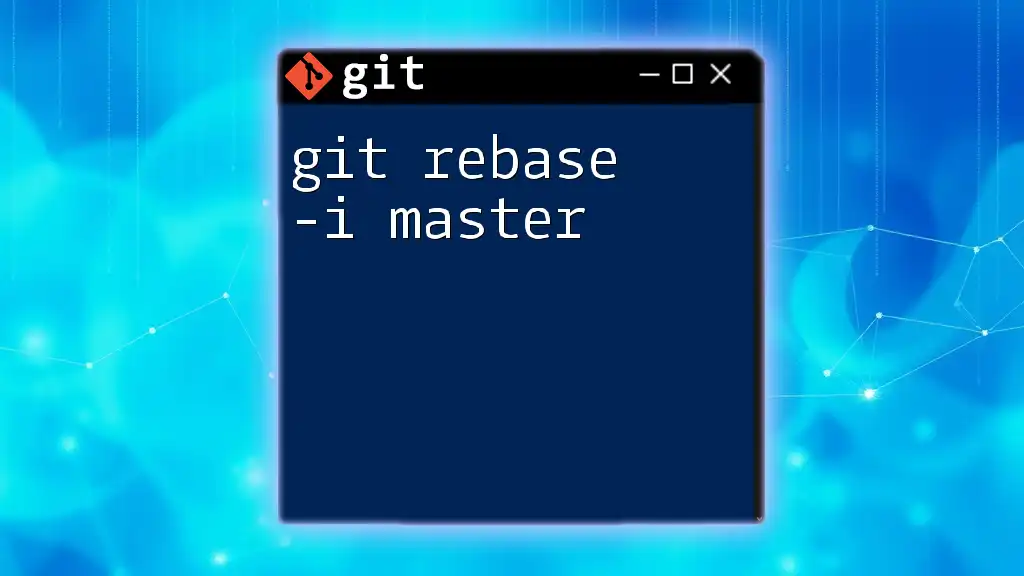
Introduction to Interactive Rebase
What is Interactive Rebase?
Interactive rebase allows you to modify commits in your branch’s history in a more granular fashion. Unlike standard rebase, which is strictly linear, interactive rebase lets you choose how to handle each commit.
Use Cases for Interactive Rebase
Here are a few scenarios where interactive rebase proves beneficial:
-
Squashing Commits: You can combine multiple commits into a single coherent one, which cleans up your commit history.
-
Reordering Commits: You can rearrange the order in which commits appear, which may aid in logical progression or collaboration.
-
Modifying Commit Messages: If you have typos or want to clarify a commit message, interactive rebase allows you to edit those messages.
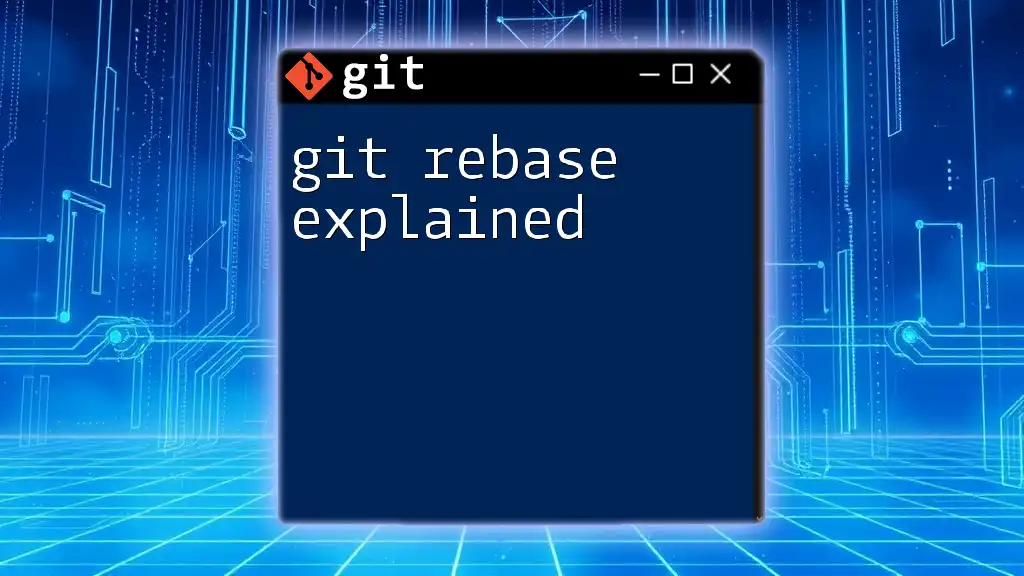
How to Use `git rebase -i main`
Preparing for the Rebase
Before you start a rebase, ensure you are on the target branch (the feature branch you want to rebase) and that your local repository is updated:
git checkout feature-branch
git fetch origin
This ensures you have the latest changes from your remote repository.
Executing the Command
To initiate the interactive rebase, execute the following command:
git rebase -i main
This command opens a text editor with a list of commits to be rebased. You’ll see something like this:
pick 1a2b3c4 First commit message
pick 2b3c4d5 Second commit message
pick 3c4d5e6 Third commit message
The Interactive Rebase Interface
In the text editor, every commit is prefixed with the word "pick". You can modify these lines to control how each commit will be handled:
- pick: Use this commit
- squash: Combine this commit with the previous one
- edit: Pause to amend this commit
- reword: Change the commit message
Example Scenarios
Squashing Commits
If you have multiple small commits that could be cleaner as a single commit, you can opt to squash them. Here’s a step-by-step:
- Execute the command:
git rebase -i main
- Change the second and third commits from “pick” to “squash”:
pick 1a2b3c4 First commit message
squash 2b3c4d5 Second commit message
squash 3c4d5e6 Third commit message
- Save and exit the editor. Git will prompt you to combine the commit messages.
Final Outcome: After executing these steps, your commit history will show a single commit with a combined message.
Reordering Commits
To reorder commits, simply change their order in the list.
Before Rebase:
pick 1a2b3c4 First commit message
pick 3c4d5e6 Third commit message
pick 2b3c4d5 Second commit message
To reorder, just change the lines:
pick 3c4d5e6 Third commit message
pick 1a2b3c4 First commit message
pick 2b3c4d5 Second commit message
After executing the command, the latest commit will appear at the top.
Changing Commit Messages
If you want to modify a commit message, use the “reword” action in the interactive rebase:
- Use the command to get started:
git rebase -i main
-
Change “pick” to “reword” for the target commit.
-
After saving and exiting, you will be prompted to change the commit message.
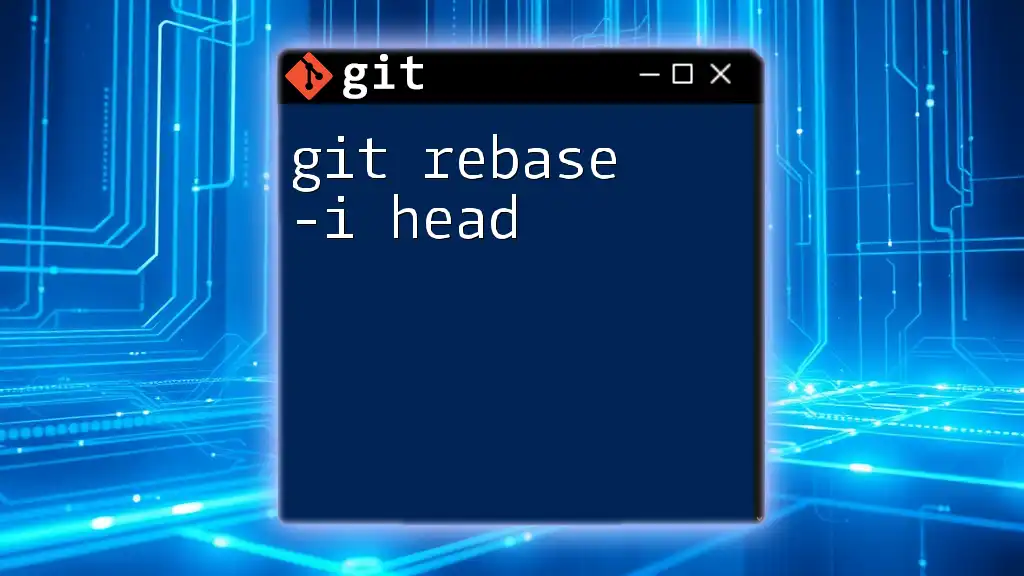
Handling Conflicts While Rebasing
What to Expect
While rebasing is a powerful tool, it may result in conflicts if changes in your branch collide with the main branch. Conflicts will pause the rebase and require you to resolve them manually.
Step-by-Step Guide to Resolving Conflicts
- If conflicts arise, Git will notify you, and you can check the status:
git status
- Edit the conflicted files to resolve the conflicts, then save your changes. Once resolved:
git add <resolved-file>
- Continue the rebase:
git rebase --continue
Git will apply the remaining commits, finishing the rebase process.
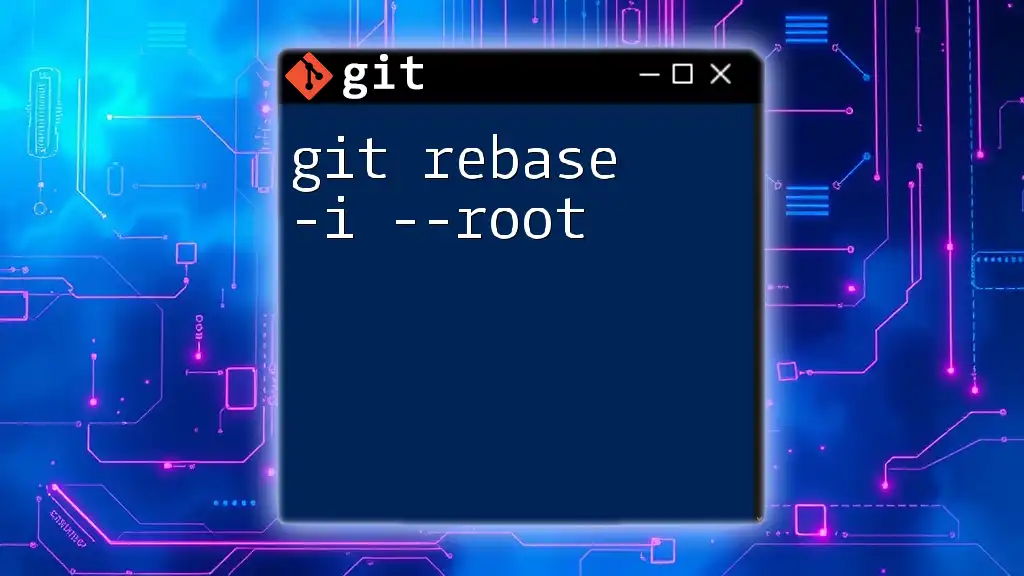
Finalizing the Rebase
Confirming Changes
After completing the rebase, review the new commit history using:
git log
This command lets you confirm that the history looks as expected without any irregular branches.
Pushing Changes After Rebase
Once you are satisfied with the rebase, you can push the changes back to the remote repository. Since the history has been rewritten, you’ll need to use the force option:
git push origin feature-branch --force
Caution: This action can overwrite existing commits on the remote repository, so ensure no one else is working on the same branch.
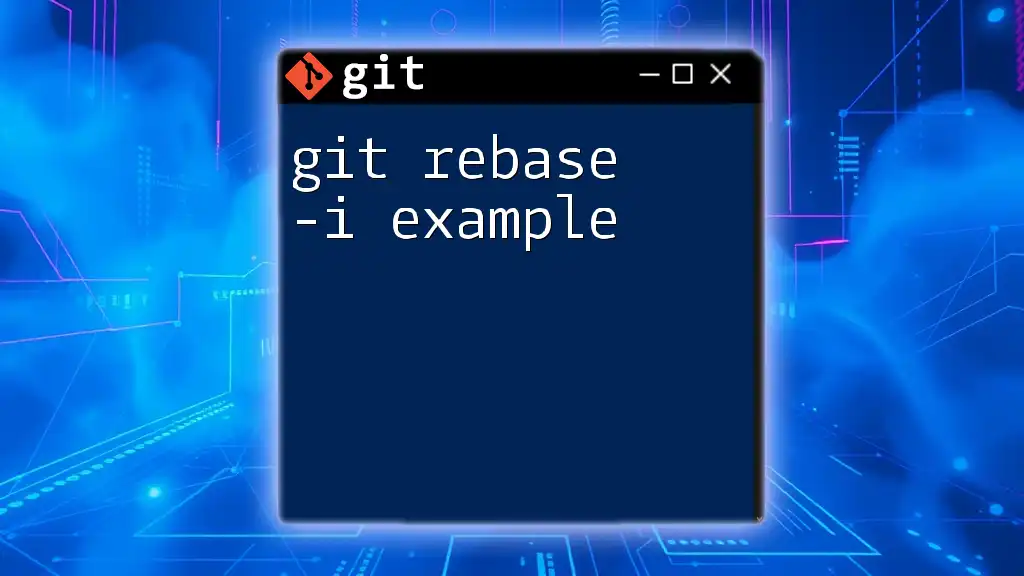
Best Practices for Using `git rebase -i main`
When to Rebase vs. Merge
-
Use Rebase when you want to maintain a cleaner history or when working with local branches that haven’t been shared publicly.
-
Use Merge when collaborating with others to retain the complete history and context of how features were developed.
Maintaining a Clean Commit History
A clean commit history with logical commits makes project maintenance easier. You might opt for commits that correspond to meaningful units of work rather than every trivial change.
Avoid Rebasing Public Branches
Rebasing branches that others are using can lead to confusion and loss of work. It is advisable only to rebase local branches that haven’t been pushed to a shared repository.
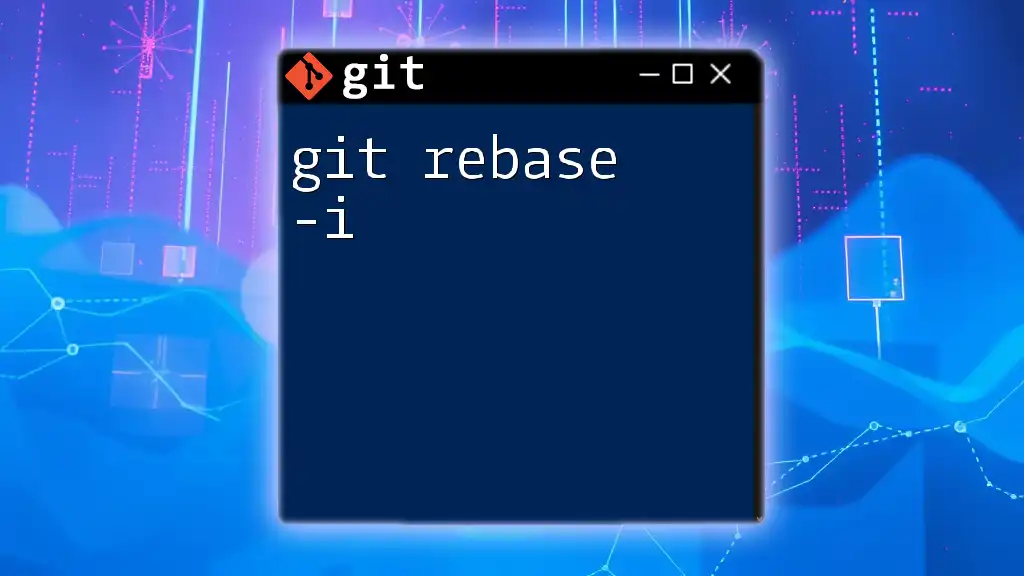
Conclusion
In conclusion, mastering the git rebase -i main command can significantly improve your workflow in collaborative software development. By understanding how to effectively use interactive rebase, you can maintain a clean, organized commit history while smoothly integrating your changes.
Consistent practice will build confidence and speed in using Git commands, making you a more efficient developer.